DS Malik's "C++ Programming" provides a comprehensive overview of C++ concepts and commands, making it an essential resource for beginners and experienced programmers alike. Here’s a simple code snippet demonstrating a basic C++ command to output "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding DS Malik's Approach to C++
DS Malik's philosophy on programming education emphasizes a problem-solving approach that breaks down complex programming concepts into easily digestible components. This method is particularly useful for beginners, as it allows them to grasp the fundamentals before moving on to more intricate topics.
Malik's C++ programming books are structured to guide learners from the very basics through to advanced concepts, ensuring that students not only learn how to write code but also understand the underlying principles that govern effective programming. His step-by-step guidance from problem analysis to design promotes critical thinking and encourages students to develop their problem-solving skills.
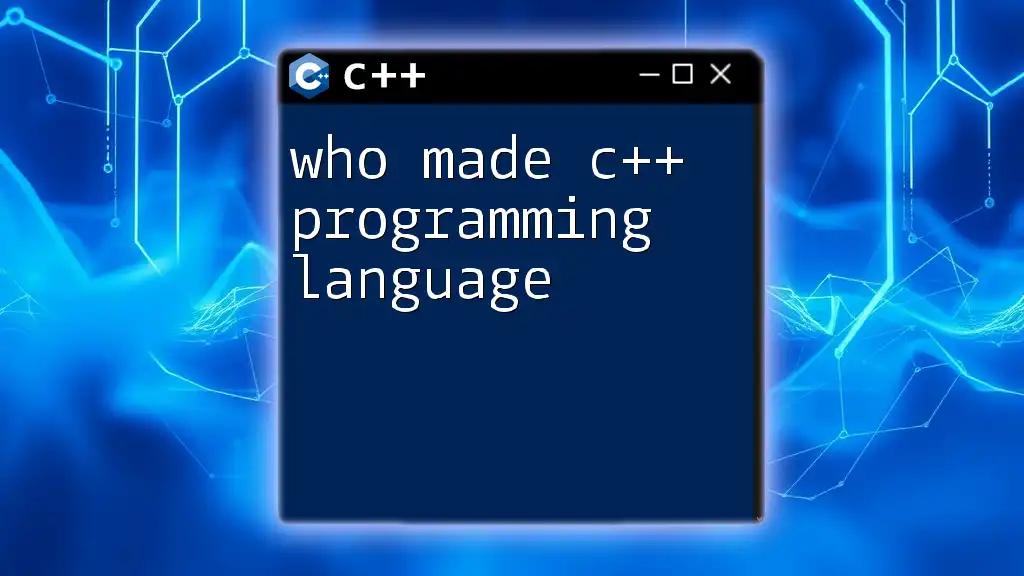
The Core Concepts of C++ Programming
Basic Constructs in C++
C++ programming begins with an understanding of its basic constructs.
Variables and Data Types are essential components of any C++ program. Common data types include:
- int: Represents integers.
- float: Represents floating-point numbers.
- char: Represents single characters.
A simple example of variable declaration is as follows:
int main() {
int age = 25; // integer variable
float salary = 1000.50; // floating point variable
char grade = 'A'; // character variable
return 0;
}
Control Structures such as if-else statements and switch-case constructions allow programmers to dictate the flow of execution based on specific conditions. Here's an example of an if-else statement:
int num = 10;
if (num > 0) {
cout << "Positive Number";
} else {
cout << "Negative Number";
}
Functions in C++
Functions play a crucial role in C++ programming by allowing code to be modularized, making it reusable and easier to manage.
Types of functions include:
- Standard Functions: Predefined functions provided by libraries.
- User-Defined Functions: Functions created by the programmer to perform specific tasks.
- Inline Functions: Functions defined with the keyword "inline" that suggest to the compiler to insert the function's code directly into the calling code.
Here’s a simple example of a user-defined function:
int add(int a, int b) {
return a + b; // returns the sum
}
int main() {
int result = add(5, 7); // calls the function
cout << "Sum: " << result;
return 0;
}
Object-Oriented Programming (OOP) in C++
Principles of OOP
C++ is a multi-paradigm programming language, but its object-oriented programming (OOP) capabilities make it particularly powerful. The four pillars of OOP are:
- Encapsulation: Bundling data with methods that operate on that data.
- Abstraction: Hiding complex implementation details and exposing only what is necessary.
- Inheritance: Enabling new classes to adopt properties from existing classes.
- Polymorphism: Allowing functions or objects to behave differently based on context.
Here’s a brief example illustrating encapsulation:
class Box {
private:
int length; // data member
public:
void setLength(int len) { // setter function
length = len;
}
int getLength() { // getter function
return length;
}
};
int main() {
Box box;
box.setLength(10);
cout << "Length: " << box.getLength();
return 0;
}
And here’s how inheritance can be demonstrated:
class Shape {
public:
void display() {
cout << "I am a shape!";
}
};
class Circle : public Shape {
public:
void display() {
cout << "I am a circle!";
}
};
int main() {
Circle c;
c.display(); // This will call Circle's display
return 0;
}
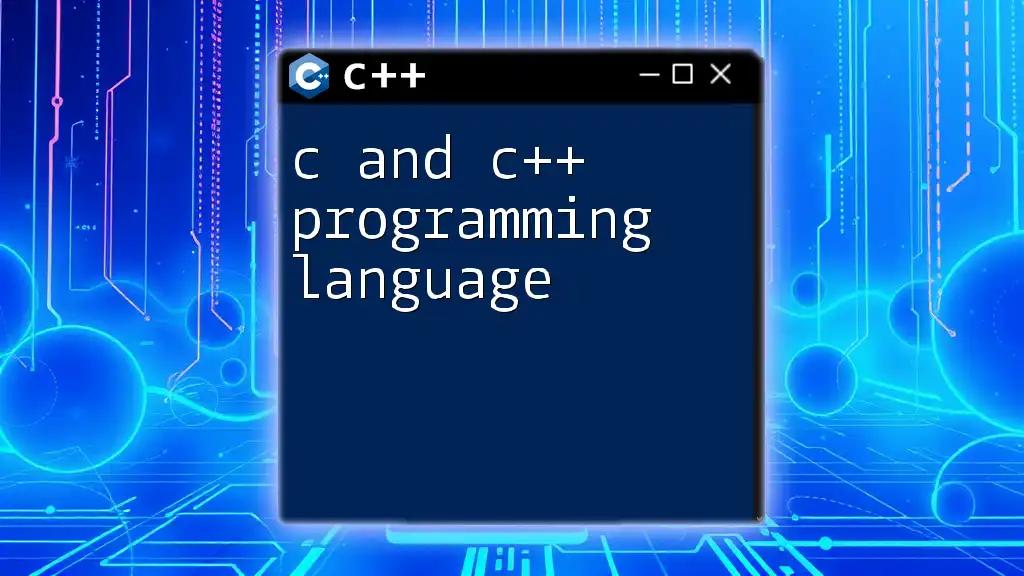
From Problem Analysis to Program Design
Problem Analysis
Effective problem analysis is crucial in developing any software. It involves understanding the requirements and defining objectives clearly. Techniques for analyzing a problem include using flowcharts and pseudocode, which help in mapping out the logic and flow of the program before actual coding begins.
For example, in analyzing a simple problem like calculating the area of a rectangle, you might start with a flowchart that shows the steps to input length and width, calculate the area, and present the output.
Program Design
Once the problem is analyzed, transitioning to program design is essential. UML (Unified Modeling Language) diagrams can be an excellent tool for visualizing the structure of the software application. For instance, you may create a class diagram to outline how various objects interact within your program.
Here, the focus is on defining how components will work together as per the design before moving to the implementation stage.
Implementation
In the implementation phase, the structured design translates into actual C++ code. This is where clear organization and meaningful comments in the code become indispensable. Here’s an example of implementing the rectangle area calculation discussed earlier:
#include <iostream>
using namespace std;
int main() {
float length, width, area;
// User input
cout << "Enter length: ";
cin >> length;
cout << "Enter width: ";
cin >> width;
// Area calculation
area = length * width;
// Output
cout << "Area of Rectangle: " << area;
return 0;
}
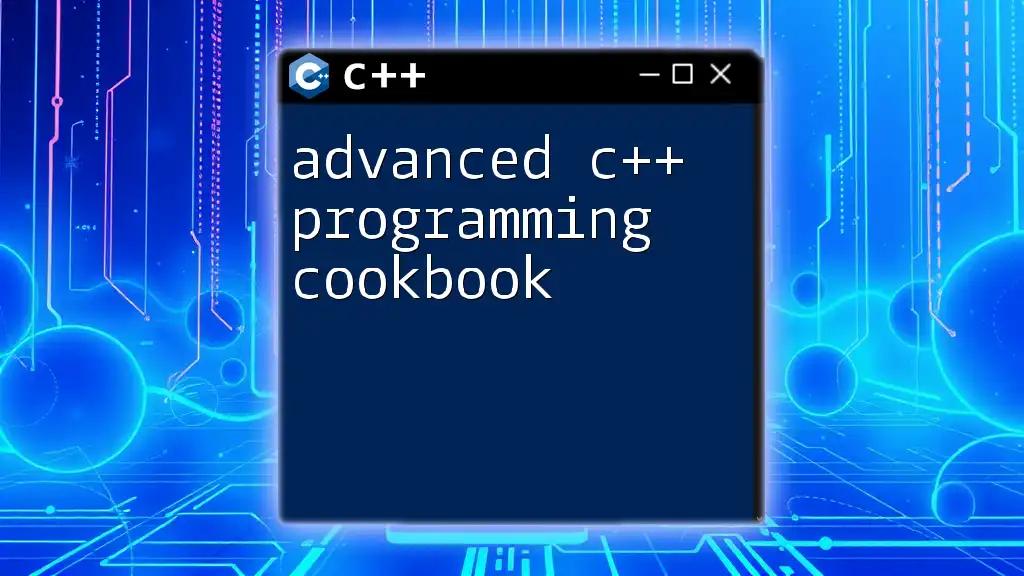
Testing and Debugging in C++
Importance of Testing
Testing is a fundamental aspect of software development, as it ensures that the application functions as intended. Different types of testing practices are crucial:
- Unit Testing: Testing individual components for correctness.
- Integration Testing: Testing how multiple components work together.
- System Testing: Testing the complete, integrated system for overall functionality.
Utilizing testing frameworks for C++ can streamline this process, enhancing code reliability.
Debugging Techniques
Debugging is essential for identifying and resolving bugs in code. Common strategies include:
- Print statements: Adding output statements throughout the code to track variable values.
- Using Debugging Tools: Many IDEs come with integrated debugging tools to help navigate through the code execution process.
Here’s an example of a potential bug in code and how you might resolve it:
int main() {
int a = 5, b = 0;
// Potential division by zero error
cout << "Result: " << (a / b); // Error here
return 0;
}
To resolve this, you would first check if `b` is zero before performing the division to avoid runtime exceptions.
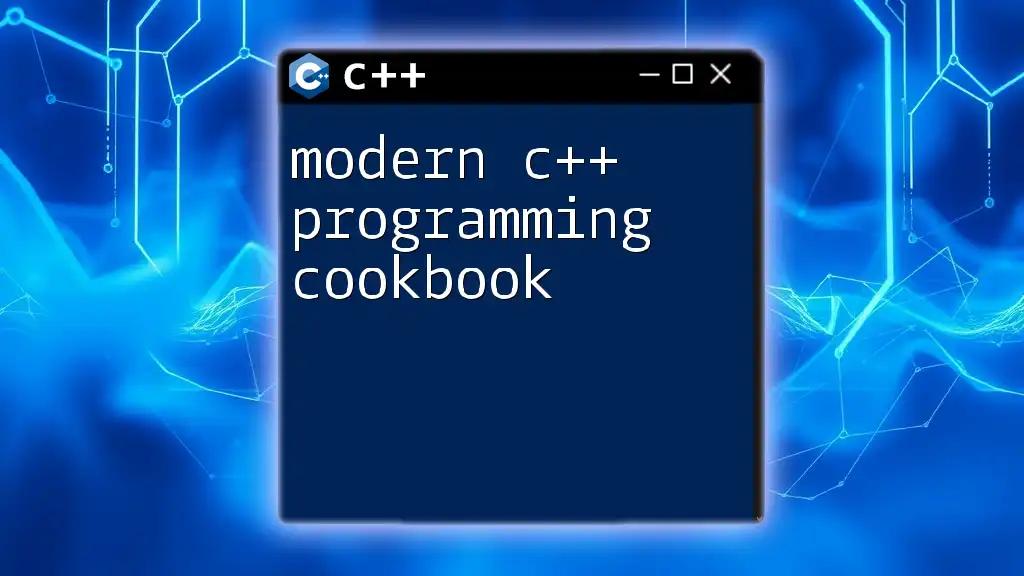
Conclusion
Mastering DS Malik C++ programming provides a structured approach to learning C++. By understanding the core concepts, transitioning through problem analysis to program design, and employing effective testing and debugging techniques, you can develop robust applications. Adopting Malik's methodology equips learners with vital skills that extend beyond merely writing code, instilling the confidence needed to tackle complex programming challenges.
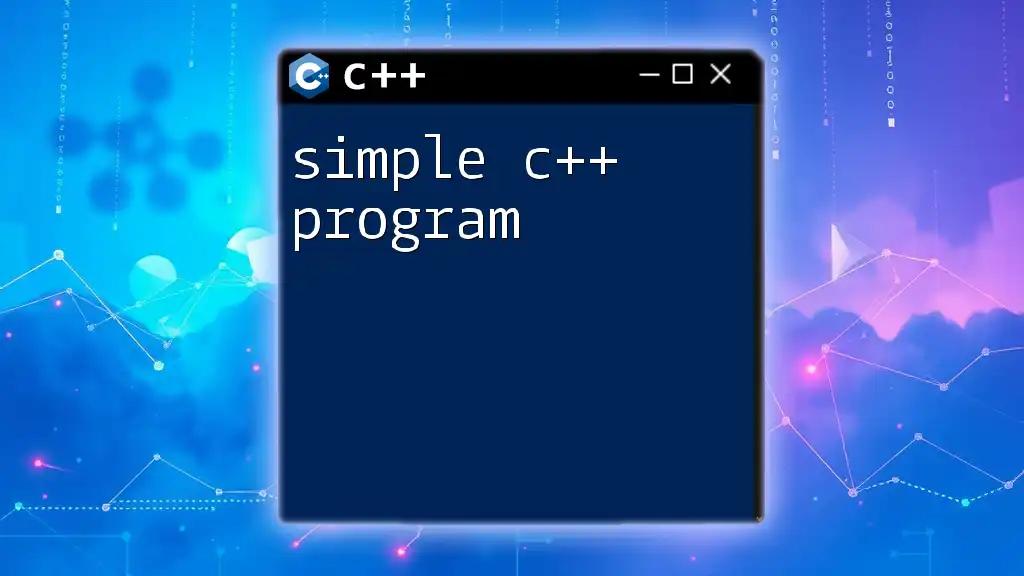
Additional Resources
For those looking to deepen their knowledge, consider exploring DS Malik's recommended books on C++ programming and engaging with online courses that provide further learning opportunities. Joining forums and communities can also be invaluable for networking and problem-solving with fellow programmers.