C++ was created by Bjarne Stroustrup at Bell Labs in the early 1980s as an extension of the C programming language, introducing object-oriented features.
Here’s a simple example of a C++ program that demonstrates the basic structure:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Origins of C++
The Need for a New Language
The programming landscape in the late 1970s was heavily dominated by the C programming language. While C was powerful and efficient, it did present several limitations that hindered developers. Memory management was a significant challenge, often leading to errors and memory leaks. Moreover, C lacked built-in support for the increasingly popular object-oriented programming (OOP) paradigms, which made large applications difficult to manage and scale.
These limitations sparked the need for a new language that could combine the efficiency of C with the features of OOP, paving the way for the creation of C++.
Bjarne Stroustrup: The Man Behind C++
Bjarne Stroustrup, a computer scientist from Denmark, embarked on his journey to create C++ during his time at Bell Labs in Murray Hill, New Jersey. His academic background in computer science and his keen interest in programming languages laid the groundwork for what would become one of the most influential languages in software development.
Stroustrup was motivated by a desire to extend the capabilities of C by incorporating features from Simula67, the first object-oriented programming language. His goal was to develop a language that allowed programmers to write efficient code while enabling better management of large software projects.
Inception of C++
C++ was born in the late 1970s, when Stroustrup began working on “C with Classes” as an extension to the C language. The initial version introduced classes, basic inheritance, and basic polymorphism—key features of OOP. By 1983, the language evolved into what we now know as C++, with “++” symbolizing the evolution from C. Stroustrup’s innovative vision combined efficiency with abstraction, enabling developers to create sophisticated and maintainable applications.
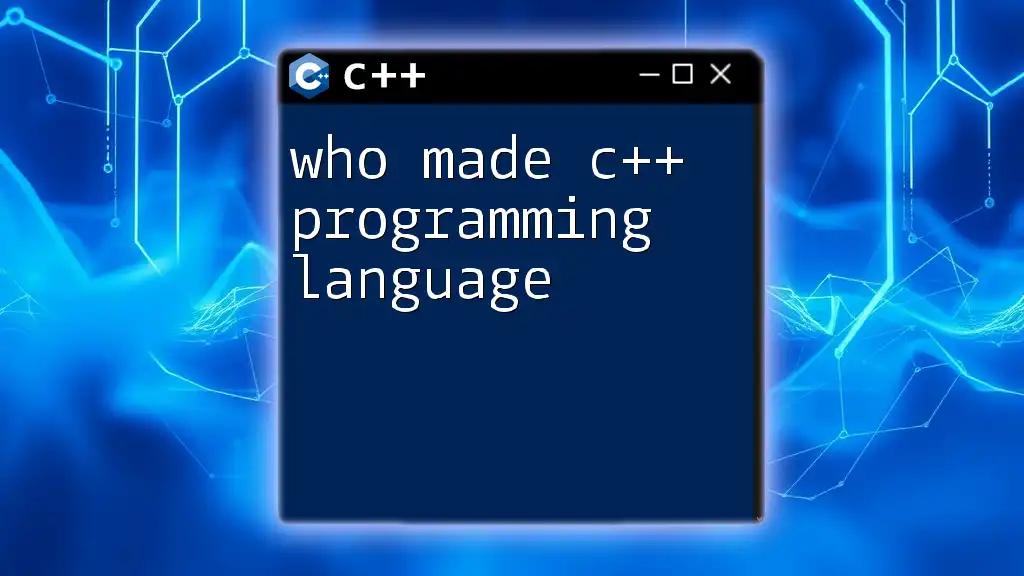
Key Features Introduced with C++
Object-Oriented Programming
What is OOP?
Object-oriented programming is a programming paradigm centered around the concepts of objects and classes. An object represents a real-world entity, while a class is a blueprint for creating objects. Key OOP concepts include:
- Encapsulation: Bundling the data (attributes) and methods (functions) that operate on the data within a single unit, restricting access to some of the object's components.
- Inheritance: Mechanism for creating a new class that inherits properties and behaviors from an existing class.
- Polymorphism: The ability of different classes to be treated as instances of the same class through a common interface.
C++ OOP Implementation
In C++, these core OOP principles are finely integrated. Here's an example of a simple class and inheritance structure:
class Animal {
public:
void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
class Dog : public Animal {
public:
void speak() {
std::cout << "Bark!" << std::endl;
}
};
In the code snippet above, `Dog` inherits from `Animal`, showcasing the inheritance feature. When the `speak()` method is called on a `Dog` object, it overrides the base class's `speak()` method.
Other Innovative Features
Templates in C++
Templates in C++ provide a powerful mechanism to create generic functions and classes. This feature allows for code reusability and the ability to work with any data type. Here’s an example of a basic template function:
template <typename T>
T sum(T a, T b) {
return a + b;
}
In this example, the `sum` function can accept parameters of any type, offering flexibility and versatility across different data types. This ability to create functions that are agnostic to the data types they operate on is a cornerstone of modern C++ programming.
The Standard Template Library (STL)
The Standard Template Library (STL) is a powerful aspect of C++, providing a collection of ready-to-use classes and functions for data structures and algorithms. STL includes components such as:
- Containers: Data structures like vectors, lists, and maps that hold collections of data.
- Algorithms: Functionalities for sorting, searching, and manipulating data in containers.
- Iterators: Mechanisms that allow for traversal of elements in containers seamlessly.
Incorporating STL allows developers to create efficient and high-performance applications while focusing on solving problems rather than implementing foundational structures from scratch.

Evolution of C++
Major Versions and Improvements
Since its creation, C++ has undergone significant revisions to enhance its functionality and to incorporate modern programming practices.
C++98, introduced in 1998, provided the first standardized version of the language known officially. Subsequent versions such as C++11 brought numerous innovative features including lambda expressions, auto keyword, move semantics, and much more. Each version, including C++14 and C++17, further refined the language and introduced new capabilities, making C++ more robust and applicable to a wider range of programming tasks.
Ongoing Development
The ISO C++ Committee, tasked with developing and maintaining the C++ standard, plays a crucial role in the linguistics' ongoing evolution. It comprises experts who gather to discuss and propose enhancements and new features. The community’s efforts have led to rapid advancements, ensuring C++ remains competitive in the face of emerging programming languages and paradigms.
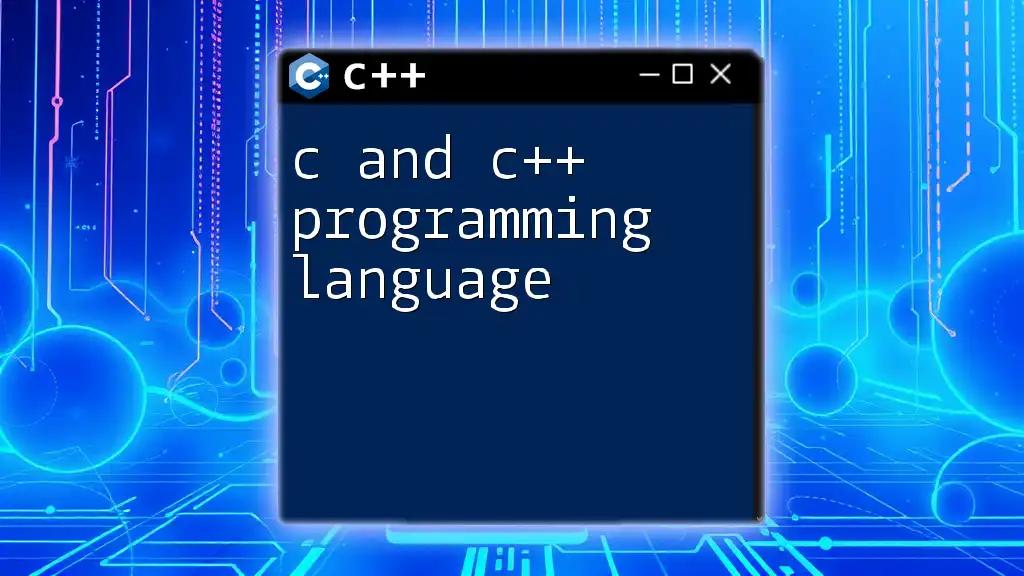
Conclusion
Bjarne Stroustrup's creation of C++ has left a profound mark on programming and software development. By seamlessly blending the efficiency of C with the features of object-oriented programming, C++ has empowered countless developers worldwide to build complex software systems.
As technology continues to evolve, the relevance of C++ remains unwavering. Its legacy, marked by the principles laid out by Stroustrup, continues to influence modern programming practices.
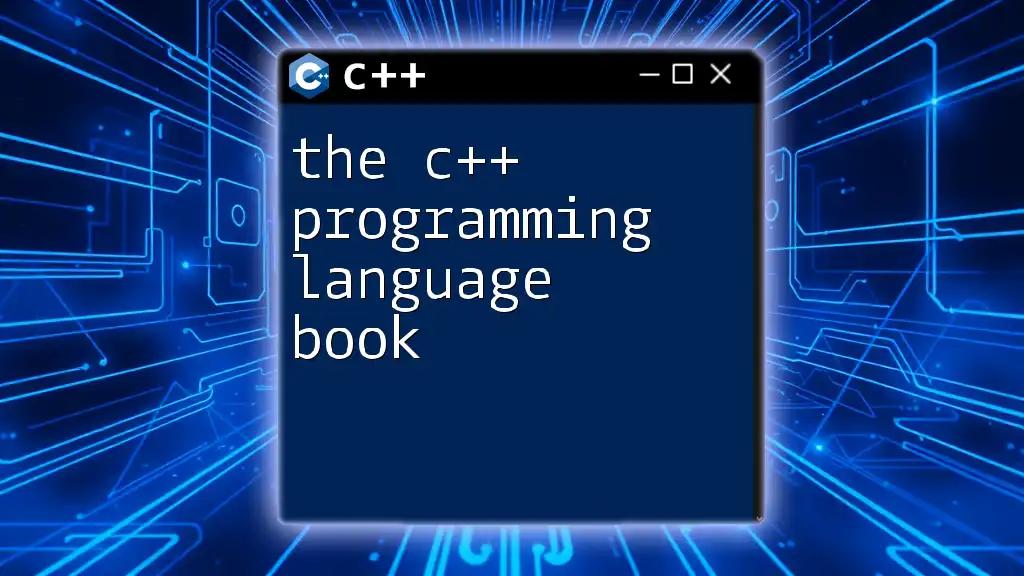
Further Resources for Learning C++
As you embark on your journey to master C++, resources abound—books, online courses, and supportive communities are available. Explore these avenues to deepen your understanding and proficiency in this powerful programming language.
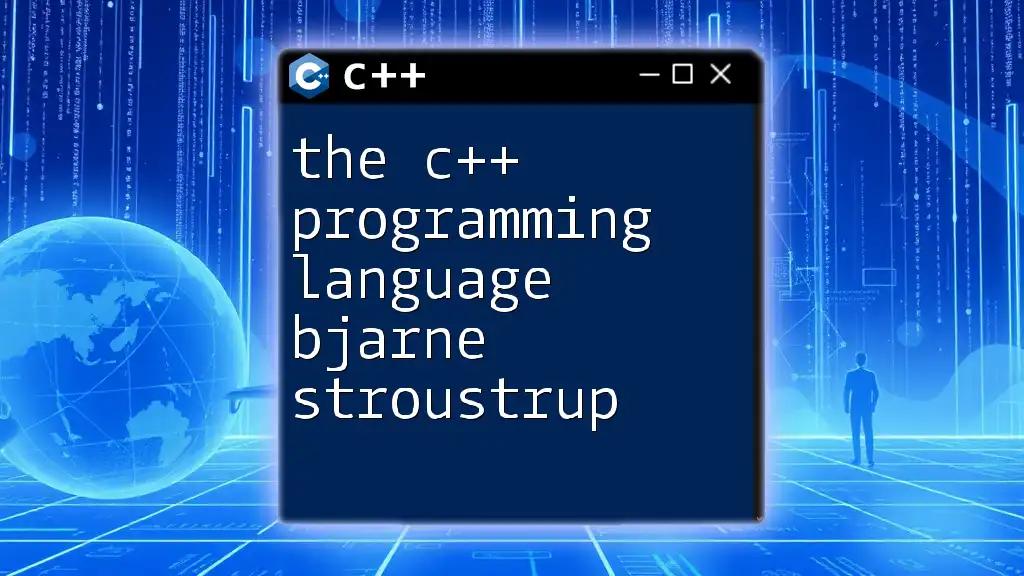
Call to Action
Join our C++ learning community to excel in your understanding of C++ commands and programming concepts. Your journey to becoming a proficient C++ programmer starts here. Feel free to reach out with any questions or thoughts; your engagement is always welcome!