"The C++ Programming Language Edition 4" is a comprehensive guide authored by Bjarne Stroustrup that serves as an essential resource for understanding and mastering C++ concepts and programming techniques.
Here's a simple C++ code snippet demonstrating a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Why Choose the C++ Programming Language Fourth Edition
The C++ programming language has undergone significant evolution, making it a favored tool in various programming arenas. With the release of the C++ programming language edition 4, developers and students alike are equipped with a resource that encapsulates both foundational concepts and contemporary practices. One of the critical motivations for choosing this edition lies in its comprehensive coverage of new features and enhancements that resonate with modern software development paradigms.
New Features and Improvements
This fourth edition introduces several new features that enrich the C++ programming experience. It delves into crucial aspects such as:
-
Enhanced types and type safety: The edition emphasizes the importance of strong typing, leading to fewer runtime errors. Features such as `const` and `constexpr` are more rigorously explained.
-
Streamlined Standard Template Library (STL): The STL is a game-changer in C++, offering a wealth of well-tested algorithms and data structures. This edition details how to implement STLs effectively to create dynamic and efficient code.
-
Better examples and exercises: Practical examples throughout the chapters help solidify understanding, making complex concepts accessible.
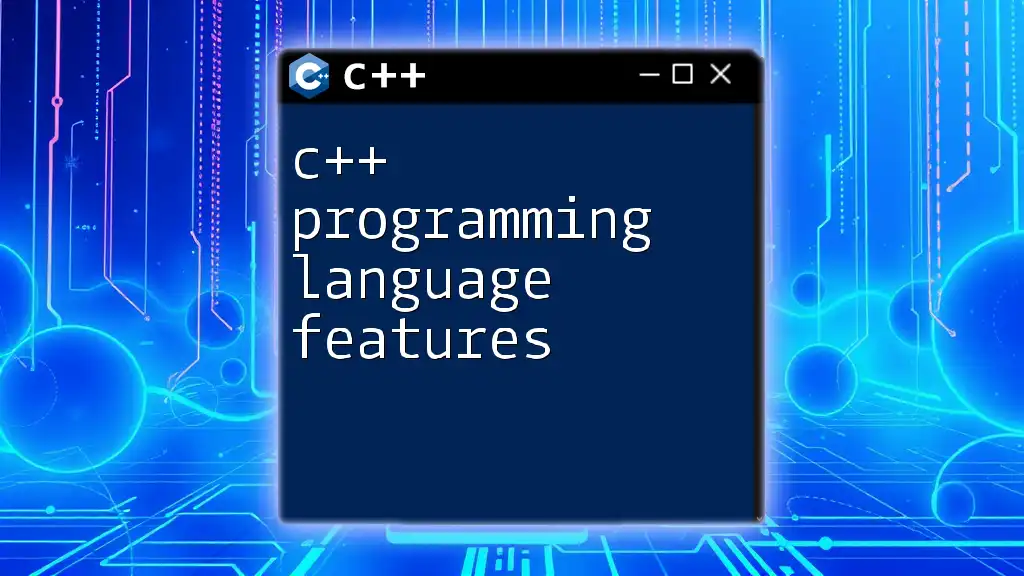
Key Highlights of the C++ Programming Language 4th Ed
Comprehensive Coverage of C++ Concepts
In this edition, readers are guided through the essential building blocks of C++. Topics such as variables, control structures, functions, and data types are thoroughly examined. The rich explanations serve as a foundation for new programmers, while also providing seasoned developers with a refresher on best practices.
Understanding these fundamentals is vital for programmers since they not only form the core of the language but also represent common patterns in software development.
In-Depth Exploration of Standard Library
The C++ Standard Library is one of the language’s most significant assets. It contains a plethora of tools that simplify programming tasks. This edition dives into the key components of STL, including vectors, lists, maps, and queues.
For example, with the following code snippet, one can create a simple vector and iterate through its elements:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
This example showcases the ease of use and flexibility that STL provides, allowing developers to focus on solving problems rather than managing data structures manually.
Advanced Topics in the Fourth Edition
Moving beyond the basics, the C++ programming language edition 4 intricately explores advanced topics that are crucial for professional development. This includes:
-
Object-Oriented Programming (OOP): The concepts of encapsulation, inheritance, and polymorphism are examined in detail. These principles allow developers to create organized and reusable code.
-
Template Programming and Generic Programming: This edition introduces templates, which are fundamental in creating functions that work with any data type, leading to code that is both flexible and efficient.
-
Exception Handling: The explanation of error management techniques is critical. Exception handling ensures that your program can gracefully recover from unexpected issues, enhancing robustness.
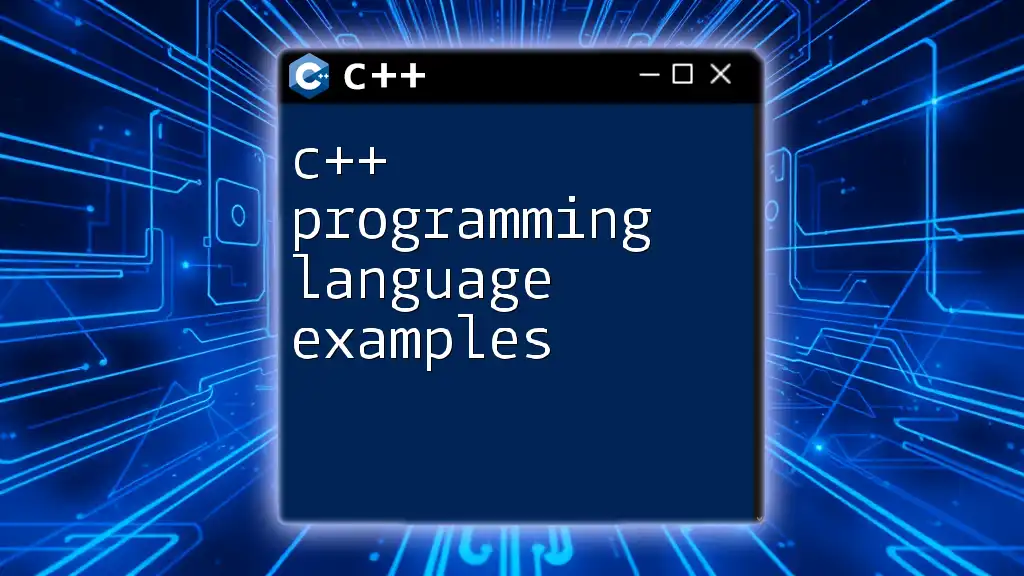
Practical Applications of C++
Developing Efficient Software with C++
C++ stands as a cornerstone in several domains including game development, system programming, and application development. One of the standout features of the C++ programming language edition 4 is its focus on performance, enabling developers to write code that runs efficiently, even in resource-constrained environments.
C++ in Real-World Projects
Numerous renowned applications, including major software suites and gaming engines, are built with C++. The edition emphasizes notable projects and showcases case studies that highlight the robustness and adaptability of C#. Understanding these real-world applications exposes learners to the diverse possibilities and encourages them to apply their knowledge in practical scenarios.
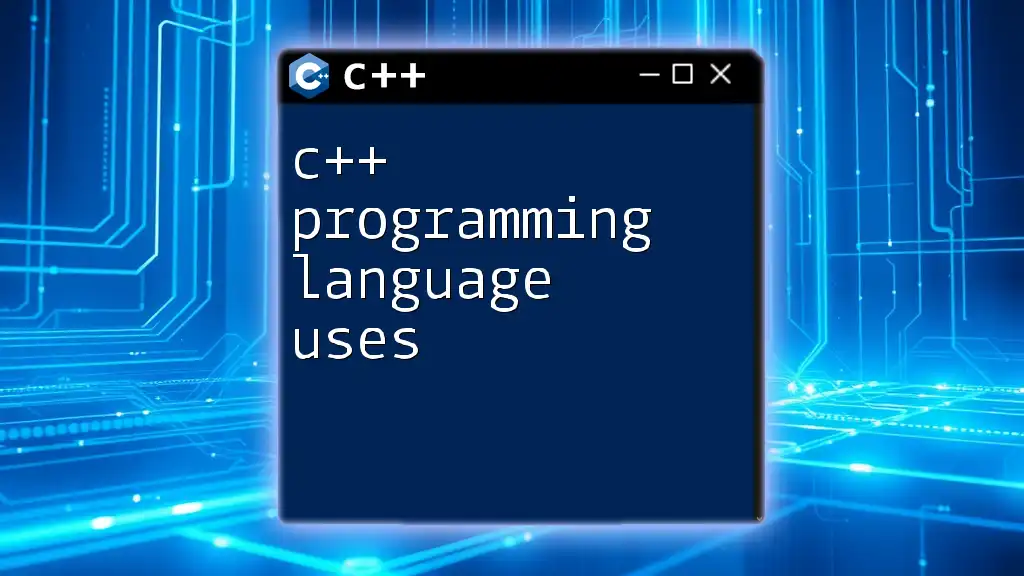
Understanding the Structure of the 4th Edition
Organization of Content
The fourth edition is meticulously organized, allowing readers to navigate through complex topics with ease. The chapters build on each other logically, leading learners from fundamental principles to advanced techniques.
Learning Techniques Emphasized
Designed not just as a textbook but a comprehensive learning tool, this edition employs various learning mechanisms. It incorporates practical examples, exercises, and self-assessment quizzes that enable readers to test their knowledge and practice coding regularly. This method of learning ensures that concepts are not just memorized, but understood and applied effectively.
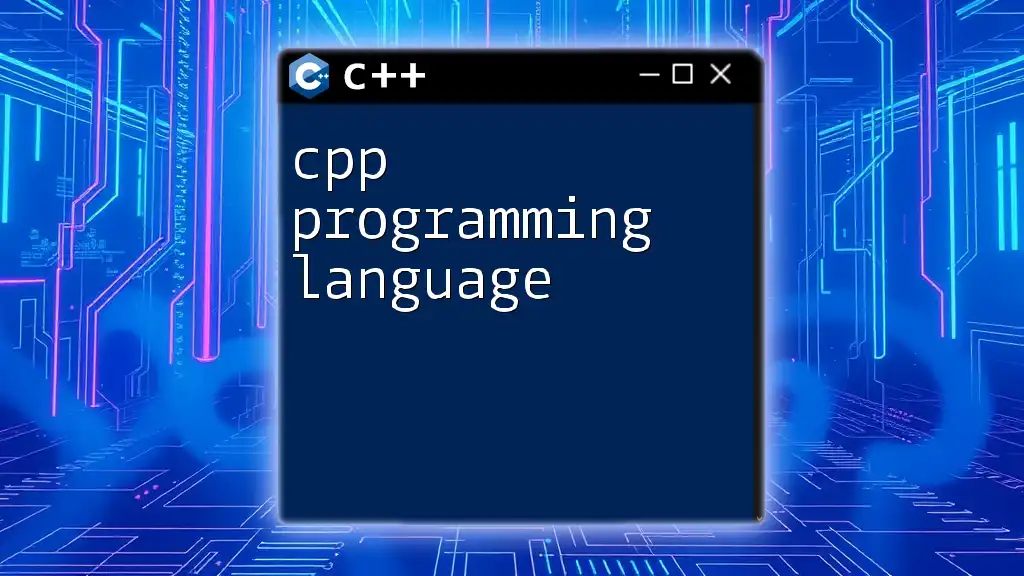
Getting the Most Out of the C++ Programming Language Fourth Edition
Recommended Resources
To complement the readings from the C++ programming language edition 4, learners can turn to various online platforms and forums. Engaging in communities such as Stack Overflow or participating in code review sites provide invaluable feedback and insights.
Building a Learning Routine
Creating a study plan that incorporates reading chapters, completing exercises, and developing small projects can significantly enhance understanding. As the C++ programming language edition 4 advocates, practice is key to mastering programming concepts, and consistent engagement with the material leads to better retention and skill enhancement.
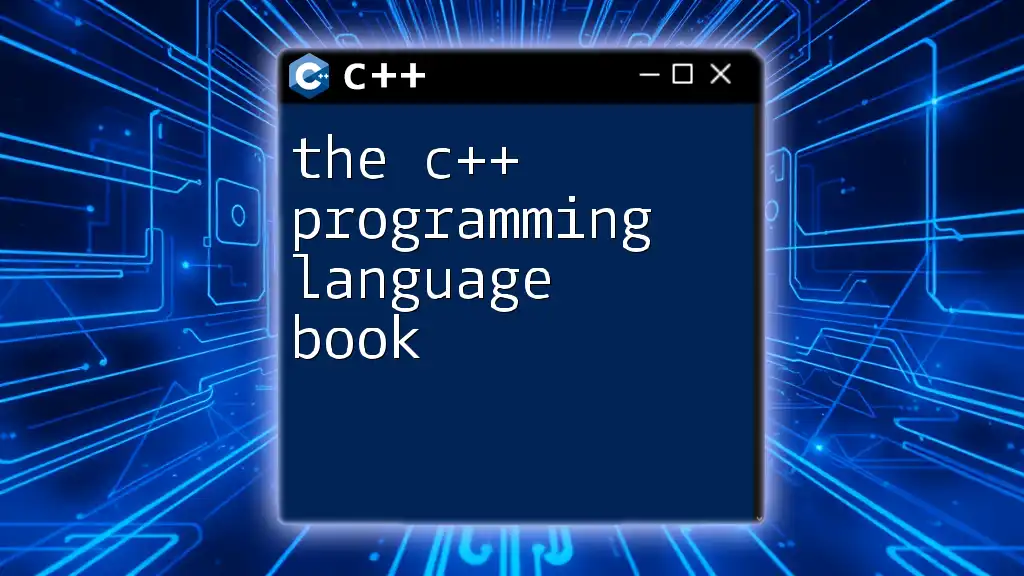
Conclusion: Embracing the C++ Programming Language Fourth Edition
With its in-depth coverage and practical approach, the C++ programming language edition 4 stands as an essential resource for anyone looking to delve into the world of C++. The blend of theory and practice throughout the edition empowers readers to tackle real-world programming challenges effectively.
Starting your journey with this edition provides not just the knowledge but also the confidence needed to excel in the vibrant landscape of C++ programming. Engage deeply, experiment continuously, and above all, embrace the challenges that come with learning a powerful language like C++.