CLion is a powerful IDE for C++ development that offers intelligent coding assistance, navigation, and integrated tools to streamline the coding process.
Here's a simple example of a C++ program in CLion:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is CLion?
Overview of CLion
CLion is a powerful Integrated Development Environment (IDE) developed by JetBrains, specifically designed for C and C++ programmers. Launched in 2015, it quickly became popular due to its extensive feature set and user-friendly interface.
Some of the key features of CLion include intelligent code assistance, CMake support, and a robust debugging toolset. It provides an advantage over traditional text editors, offering real-time feedback and enhanced productivity tools that aid developers in building complex applications efficiently.
System Requirements
To utilize CLion, ensure your system meets the following minimum requirements:
- Operating Systems: Windows (10 or later), macOS (10.13 or later), or Linux (Ubuntu, Fedora, etc.)
- Hardware Specifications: A minimum of 2 GB RAM (8 GB is recommended) and 1.5 GB of disk space, with additional space for your projects.
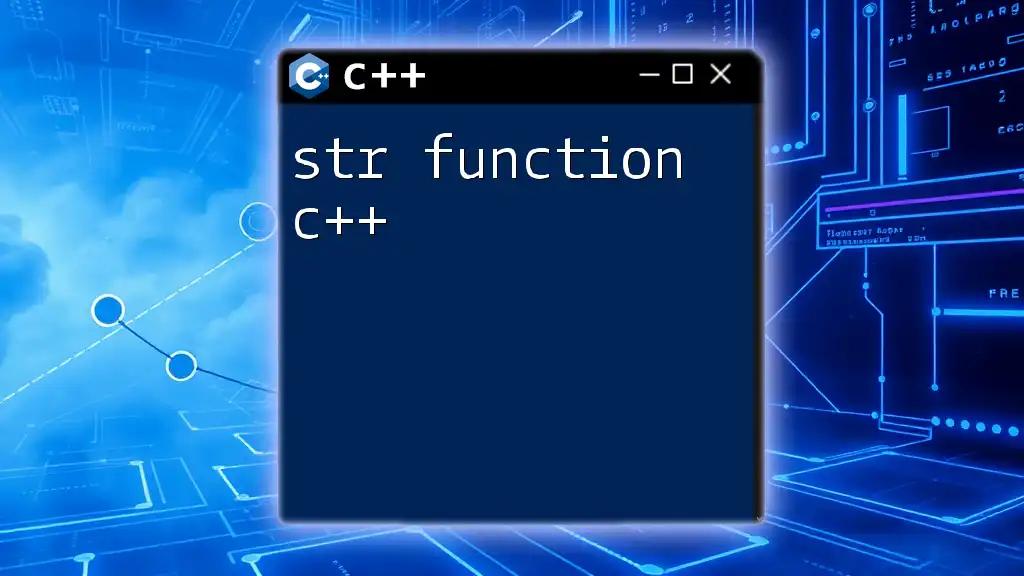
Getting Started with CLion
Installation Process
Installing CLion is straightforward. Here’s a quick guide:
- Visit the JetBrains website and download the installer for your operating system.
- Run the installer and follow the on-screen instructions.
- Once installed, launch CLion and complete the initial configuration, which includes choosing the UI theme and plugins.
During the initial setup, make sure to install any necessary toolchains, such as compilers and debuggers, so you can start coding right away.
Interface Walkthrough
Upon launching CLion, you will encounter a well-organized user interface that consists of several key components:
- Editor Window: This is where you write and edit your C++ code.
- Project Tree: Located on the left, this panel displays your project structure and files, making navigation easy.
- Navigation Bar: At the top, this bar allows quick access to different parts of your project.
- Tool Windows: These windows provide various functionalities like version control, debugging, and terminal access, all accessible via the sidebar.
Customizing the UI can significantly enhance your coding experience. You can rearrange the layout, resize panels, and adjust themes to make it more comfortable for your workflow.
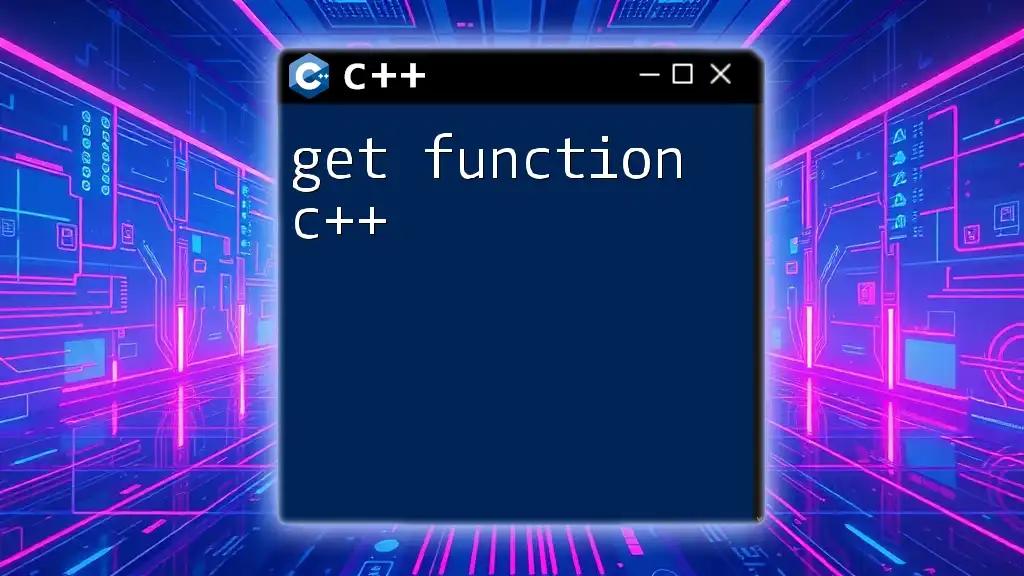
Creating Your First C++ Project in CLion
Setting Up a New Project
To create a new C++ project, follow these steps:
- On the welcome screen, click on “New Project”.
- Select “C++ Executable” from the list of project types.
- Choose your toolchain (e.g., GCC or Clang) and click “Create”.
CLion will generate a standard project layout, including a `CMakeLists.txt` file essential for managing project settings.
Writing C++ Code in CLion
Writing C++ code in CLion is a breeze, thanks to its intelligent coding features. Here’s a simple example to get started:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, we're including the `iostream` library, which is necessary for input and output operations. The `main` function executes the program, printing "Hello, World!" to the console.
CLion enhances your coding experience with syntax highlighting and code formatting, which can help avoid common programming mistakes.
Building and Running Your Application
To build and run your application:
- Click on the green "Run" button in the top-right corner.
- If there are no errors, the program will compile and execute, displaying the output in the console at the bottom of the IDE.
Understanding the output window is crucial, as it displays build errors, warnings, and runtime output, allowing you to debug any issues effectively.
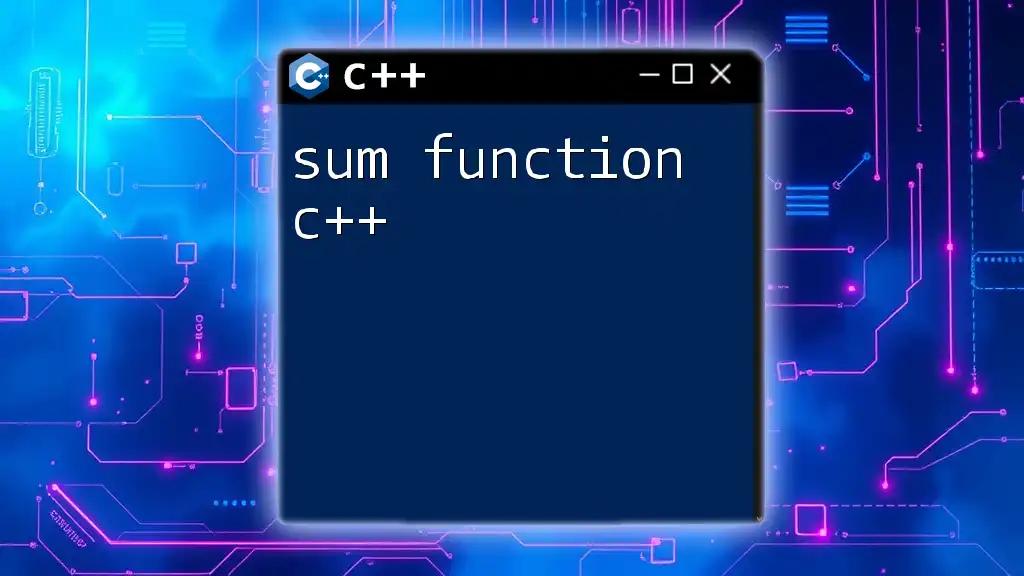
Basic Features of CLion
Code Completion and Suggestions
One of the standout features of CLion is its code completion functionality. As you type, CLion offers context-aware suggestions, which helps reduce the time spent coding by completing common patterns and providing relevant options. This feature can significantly boost your productivity and help you avoid syntax errors.
Refactoring Tools
Refactoring is an essential practice for maintaining clean code. CLion includes powerful refactoring tools that allow you to:
- Rename variables, functions, and classes effortlessly.
- Move files and classes.
- Change method signatures.
These tools help ensure that your codebase remains tidy and organized, making it easier to maintain over time.
Debugging in CLion
Debugging is simplified with CLion's integrated tools. You can set breakpoints by clicking in the gutter next to the line numbers, allowing you to pause execution and inspect your program's state. During a debugging session, you can:
- Step through your code line-by-line.
- Inspect variable values and watch expressions to monitor changes.
- Analyze the call stack to trace the function calls leading to the current execution point.
This rich debugging environment helps identify and fix issues quickly and efficiently.
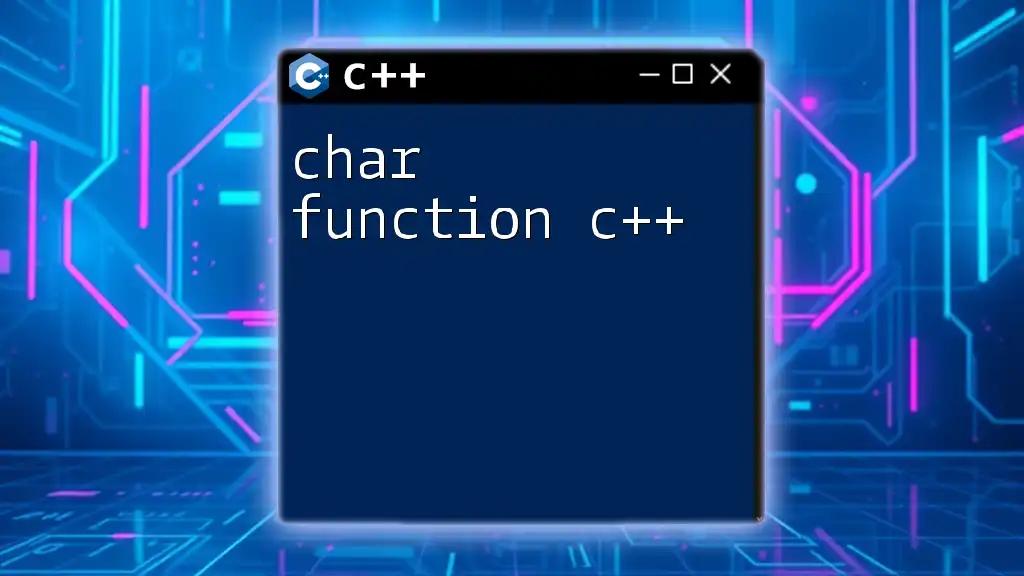
Advanced Features
Integrated Tools and Plugins
CLion is not just a C++ IDE; it comes with integrated tools such as:
- CMake: A powerful build system that allows you to manage complex builds seamlessly.
- Git integration: Built-in version control support that simplifies collaboration and version management.
In addition, CLion supports plugins to extend its functionality, enhancing your development experience. You can browse and install plugins directly from the IDE under the "Plugins" menu.
Code Analysis and Inspections
CLion offers real-time code analysis, providing immediate insights into code quality. Inspections can highlight potential issues and suggest improvements, ensuring your code adheres to best practices. This feature fosters a disciplined coding style and can help prevent future bugs.
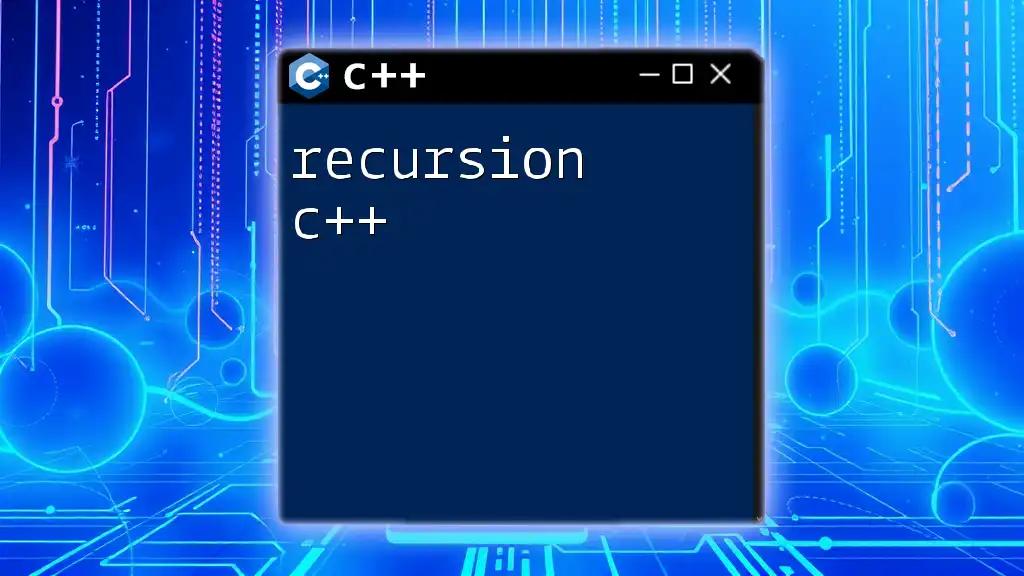
Customizing CLion for C++ Development
Configuring Compiler and Toolchains
To maximize your productivity with C++, properly configuring the compiler and toolchain is essential. You can specify different compilers such as GCC or Clang and configure multiple toolchains for various platforms directly from the settings menu. This flexibility is particularly useful for cross-platform development.
Editor Settings and Preferences
Customizing your editor settings can enhance your coding efficiency. You can adjust code styles to match your preferences, set keymap shortcuts to speed up your workflow, and tweak other settings to create an IDE environment that works best for you.

Frequently Asked Questions
Common Issues and Their Solutions
As you navigate using CLion, you might encounter some common issues, such as installation problems or compiler errors. Many solutions can be found in the extensive documentation provided by JetBrains or through community forums.
CLion vs Other IDEs
CLion stands out against other C++ IDEs like Visual Studio or Eclipse due to its intuitive interface and powerful features. While some IDEs may excel in specific areas, CLion’s integrated tools and support for modern C++ standards make it a robust choice for many developers.

Conclusion
CLion is a comprehensive IDE that simplifies C++ development through its array of features, making it easier to write, debug, and manage code. By leveraging its intelligent coding assistance, debugging tools, and integration with popular build systems, developers can achieve efficient, high-quality programming.

Additional Resources
Official Documentation
For further reading and detailed guidance, visit the [JetBrains official CLion documentation](https://www.jetbrains.com/help/clion/).
Community Forums
Connecting with other CLion users can provide valuable insights. Consider joining forums such as the JetBrains Community or Stack Overflow to share experiences and tips.
Recommended Tutorials
Explore additional tutorials and courses available online to deepen your understanding of both C++ and CLion for even more effective programming.

Call to Action
If you found this guide helpful, consider subscribing for more C++ tips and tricks. We welcome your feedback and encourage you to share your experiences using CLion!