C++ programming progresses from fundamental control structures that manage the flow of the program, to advanced concepts such as classes and objects that encapsulate data and behavior, enabling better organization and modularity in code development.
#include <iostream>
using namespace std;
void controlStructuresExample(int x) {
// Control Structure: If-Else
if (x > 0) {
cout << "Positive number" << endl;
} else {
cout << "Negative number or zero" << endl;
}
}
class ObjectExample {
public:
void displayMessage() {
cout << "Hello from Object!" << endl;
}
};
int main() {
controlStructuresExample(10); // Control structure in action
ObjectExample obj; // Creating an object
obj.displayMessage(); // Calling a method on the object
return 0;
}
Understanding Control Structures
What are Control Structures?
Control structures are essential components in programming that dictate the flow of execution of a program based on certain conditions. They allow developers to control the order in which statements are executed, which is crucial for developing logic in code. There are three primary types of control structures:
- Sequential: This is the default mode of execution where statements are processed one after another.
- Selection: These structures allow the program to select different paths of execution based on certain conditions (e.g., `if`, `switch`).
- Iteration: Also known as looping, iteration structures repeat a block of code as long as a specified condition is true (e.g., `for`, `while`).
Selection Control Structures
If Statements
An `if` statement evaluates a condition and executes a block of code if the condition is true. This is a foundational control structure that allows decision-making within a program.
Example:
int age = 18;
if (age >= 18) {
std::cout << "You are eligible to vote." << std::endl;
}
In this example, the statement checks if the variable `age` is greater than or equal to 18. If true, it prints a message indicating that the user is eligible to vote.
Switch Statements
A `switch` statement provides a cleaner way to handle multiple conditions compared to multiple `if` statements. It evaluates a variable against several possible values (cases).
Example:
int day = 3;
switch (day) {
case 1: std::cout << "Monday"; break;
case 2: std::cout << "Tuesday"; break;
case 3: std::cout << "Wednesday"; break;
default: std::cout << "Invalid day"; break;
}
Here, the `switch` statement checks the value of `day` and executes the corresponding block of code. If none of the cases match, it executes the `default` case.
Iteration Control Structures
For Loops
`For` loops are used when the number of iterations is known beforehand. They consist of an initialization, a condition, and an increment/decrement expression.
Example:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
In this example, the loop iterates five times, printing the current iteration number each time.
While Loops
`While` loops are used when the number of iterations is not known, and the loop continues until a specified condition becomes false.
Example:
int count = 0;
while (count < 5) {
std::cout << "Count: " << count << std::endl;
count++;
}
This code block continues to execute as long as `count` is less than 5, incrementing `count` with each iteration.
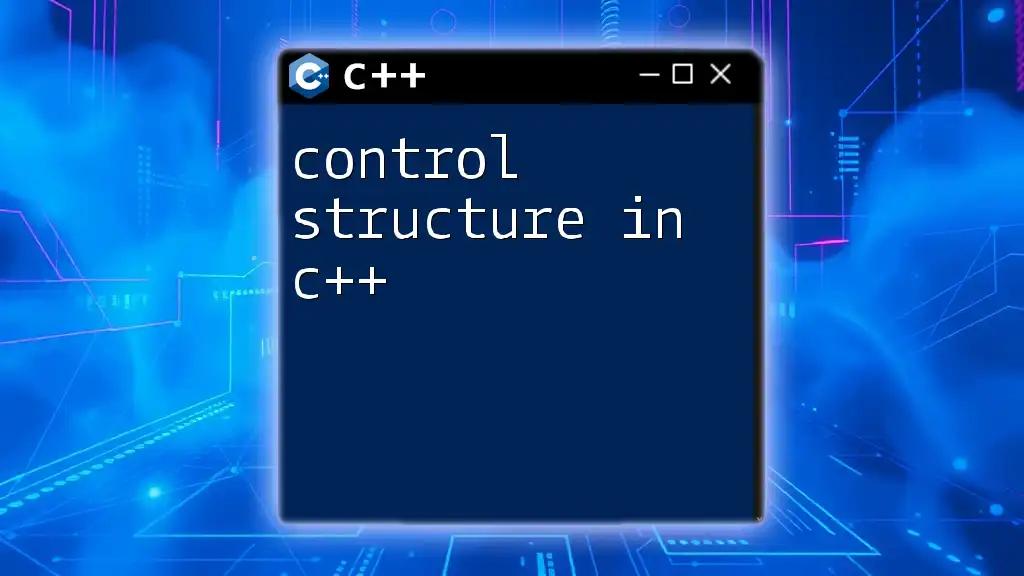
Introduction to Functions
What are Functions?
Functions are reusable blocks of code designed to perform a specific task. A function reduces code duplication and improves modularity and readability. They can take parameters and often return values.
Creating and Using Functions
To create a function, you provide a return type, a name, and any required parameters.
Example:
int add(int a, int b) {
return a + b;
}
Here, the function `add` takes two integer parameters and returns their sum.
Calling Functions:
int result = add(5, 3);
std::cout << "Result: " << result << std::endl;
This snippet calls the `add` function with arguments 5 and 3 and stores the return value in `result`, which is then printed.

From Control Structures to Object-Oriented Programming
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm centered around objects, encapsulating data, and operations on that data. The four fundamental concepts of OOP are:
- Classes: Blueprints for creating objects.
- Objects: Instances of classes.
- Encapsulation: Bundling data and methods that operate on the data within one unit.
- Inheritance: Mechanism to create new classes based on existing classes, promoting code reuse.
- Polymorphism: The ability to call the same method on different objects, allowing for dynamic behavior.
Creating Classes and Objects
Defining a Class
A class is defined using the `class` keyword, and it encapsulates attributes (variables) and methods (functions).
Example:
class Car {
public:
std::string model;
int year;
void display() {
std::cout << "Model: " << model << ", Year: " << year << std::endl;
}
};
In this class definition, `Car` has two public attributes (`model` and `year`) and a method `display` to print the car details.
Instantiating an Object
To create an object of a class, simply declare an instance of the class. Accessing attributes and methods is straightforward.
Example:
Car myCar;
myCar.model = "Toyota";
myCar.year = 2020;
myCar.display();
In this example, we create an object `myCar` of type `Car`, set its attributes, and call the `display` method.
Concept of Inheritance
Inheritance allows a new class (derived class) to inherit attributes and methods from an existing class (base class). This promotes code reuse and establishes a relationship between classes.
Example:
class Vehicle {
public:
void start() {
std::cout << "Vehicle is starting." << std::endl;
}
};
class Bike : public Vehicle {
public:
void ringBell() {
std::cout << "Bike bell rings!" << std::endl;
}
};
Here, `Bike` inherits from `Vehicle`, allowing it to use the `start` method while also having its own unique method, `ringBell`.

Practical Applications
Building a Simple C++ Application
Building a robust application involves combining various elements of C++, including control structures, functions, and objects. Start by defining the overall structure of your program, followed by creating classes for encapsulating data and logic. Functions will help modularize code, while control structures guide the flow.
Integrating Control Structures and Objects
In real-world scenarios, combining control structures with objects can enhance functionality. For instance, an application managing a library could use classes for `Book` and `User` while employing control structures for checking availability or handling user interactions.
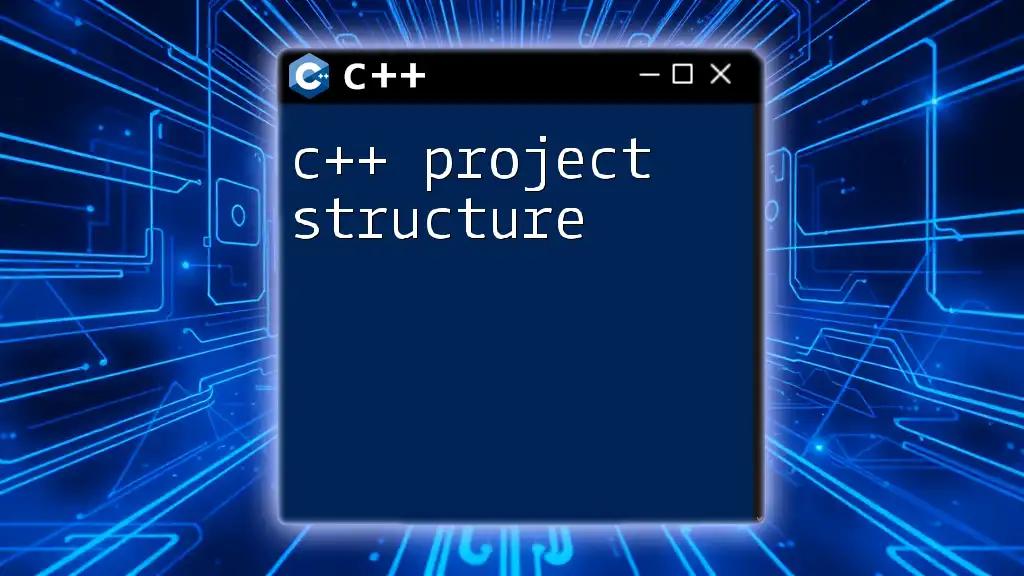
Conclusion
In summary, understanding C++ from control structures through objects is vital for developing any practical application. Control structures provide fundamental decision-making capabilities; functions enhance modularization, and object-oriented programming offers a robust framework for building complex systems. To deepen your knowledge, keep practicing, explore advanced topics, and consider joining learning platforms that specialize in C++. Together, these elements lay a solid foundation for your journey in C++.

Additional Resources
If you’re looking to further your understanding of C++, consider exploring respected texts like “Starting Out with C++” by Gaddis or participating in online programming communities for additional guidance and support.