C++ offers powerful data structures like arrays, linked lists, and maps that enable efficient data manipulation and organization, essential for building robust applications.
Here's a basic code snippet demonstrating the use of a vector in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Data Structures
What are Data Structures?
A data structure is a specialized format for organizing and storing data in a computer so that it can be accessed and modified efficiently. Data structures are crucial for developing efficient algorithms and managing data effectively. They can be broadly categorized into primitive and non-primitive structures.
Why Use Data Structures in C++?
Utilizing appropriate data structures can significantly enhance the efficiency and performance of your C++ applications. For instance, a well-chosen data structure can reduce the time complexity of algorithms, enabling faster data processing and retrieval. Common applications range from managing large datasets in databases to optimizing search algorithms in search engines.
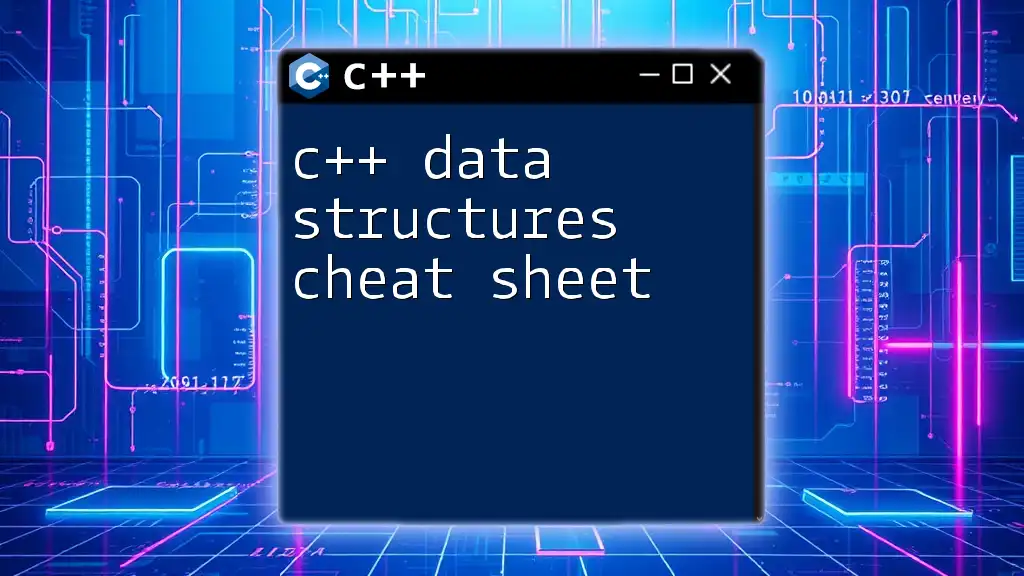
Fundamental Data Structures in C++
Arrays
Arrays are one of the simplest data structures, allowing you to store a collection of elements indexed by contiguous memory locations.
Characteristics:
- Fixed size
- Homogeneous elements (same data type)
Syntax and Example:
int arr[5] = {1, 2, 3, 4, 5};
In this example, `arr` can hold five integers. Accessing an element is straightforward, as you simply use the index, such as `arr[0]` to access the first element. However, it’s important to note that resizing an array after its definition requires allocating a new array and copying elements, which can be inefficient.
Structures
C++ allows the creation of structures, which act as custom data types. Structures can group different types of data together.
Syntax and Definition:
struct Student {
string name;
int age;
};
In this example, we define a `Student` structure with two fields: `name` of type `string` and `age` of type `int`. This helps organize related data and enhances clarity in code.
Linked Lists
A linked list is a dynamic data structure consisting of nodes, where each node contains data and a pointer to the next node.
Types:
- Singly Linked List: Nodes point only to the next node.
- Doubly Linked List: Nodes point to both the next and the previous nodes.
Code Snippet for a Singly Linked List:
struct Node {
int data;
Node* next;
};
In a linked list, you can efficiently insert or remove nodes without reallocating the whole structure. However, unlike arrays, linked lists do not allow direct access to individual elements, which can slow down certain operations.
Stacks
A stack is a linear data structure that follows the Last In First Out (LIFO) principle. That means the last element added is the first to be removed.
Common Operations:
- Push: Add an element to the top of the stack
- Pop: Remove the top element
- Peek: Retrieve the top element without removing it
Implementation Example:
class Stack {
int top;
int arr[MAX];
public:
Stack() { top = -1; }
void push(int x) {
if (top >= MAX - 1) {
cout << "Stack Overflow";
} else {
arr[++top] = x;
}
}
};
In the above class, we create a stack using an array. `top` keeps track of the last element, ensuring stack operations are performed efficiently.
Queues
A queue is another linear data structure that follows the First In First Out (FIFO) principle, where the first element added is the first one to be removed.
Representation:
- Linear Queue: Follows a linear approach.
- Circular Queue: Links the end back to the beginning to facilitate better memory usage.
Example of Basic Queue Implementation:
class Queue {
int front, rear;
int queue[MAX];
public:
Queue() { front = rear = -1; }
void enqueue(int x) {
if (rear == MAX - 1) {
cout << "Queue Overflow";
} else {
queue[++rear] = x;
if (front == -1) front = 0;
}
}
};
This class manages a queue and provides basic operations to manage data flow, commonly required in various applications like scheduling and buffering.
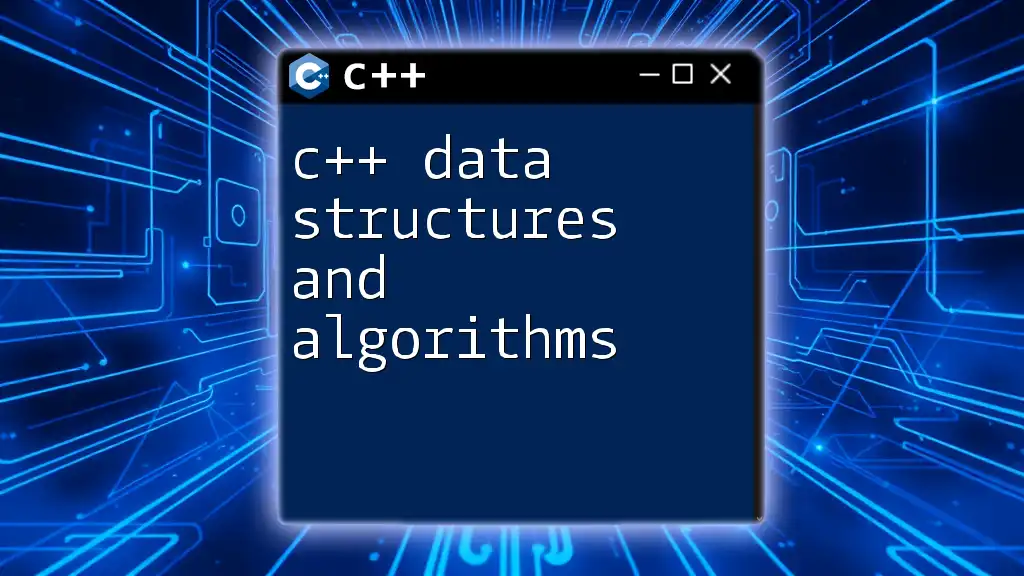
Advanced Data Structures in C++
Trees
Trees are hierarchical data structures that consist of nodes, with a single node as the root from which subtrees branch out. They are pivotal for structuring data hierarchically.
Types:
- Binary Tree: Each node has at most two children.
- Binary Search Tree (BST): A binary tree where the left child is less than the parent and the right child is greater.
- AVL Tree: A self-balancing binary search tree.
Example of a Basic Binary Tree Structure:
class TreeNode {
int value;
TreeNode* left;
TreeNode* right;
};
Trees are advantageous for searching and sorting operations, providing logarithmic time complexity in many cases when balanced. They are widely used in databases and file systems.
Graphs
A graph is a collection of nodes, called vertices, and edges that connect pairs of nodes. They can represent various data structures such as social networks, road maps, etc.
Types:
- Directed Graph: Edges have a direction.
- Undirected Graph: Edges are bidirectional.
Representation Methods:
- Adjacency Matrix: A 2D array indicating whether pairs of vertices are adjacent.
- Adjacency List: A collection of lists or arrays to show all neighbors for each vertex.
Example of Creating a Simple Graph Using Adjacency List:
vector<vector<int>> graph(V);
Graphs offer flexibility in representation and allow complex relationships among data to be modeled effectively.
Hash Tables
A hash table is a data structure that maps keys to values for highly efficient data retrieval. Using a hash function, it converts a given key to an index in an array, where the value corresponding to that key is stored.
Benefits:
- Average-case constant time complexity for search, insert, and delete.
- Handles collisions efficiently with techniques such as chaining or open addressing.
Example of Implementing a Simple Hash Table:
class HashTable {
list<pair<int, string>> table[MAX];
public:
void insert(int key, string value) {
int index = hashFunction(key);
table[index].emplace_back(key, value);
}
};
Hash tables are exceptionally useful in scenarios requiring rapid data access, such as databases and caching mechanisms.
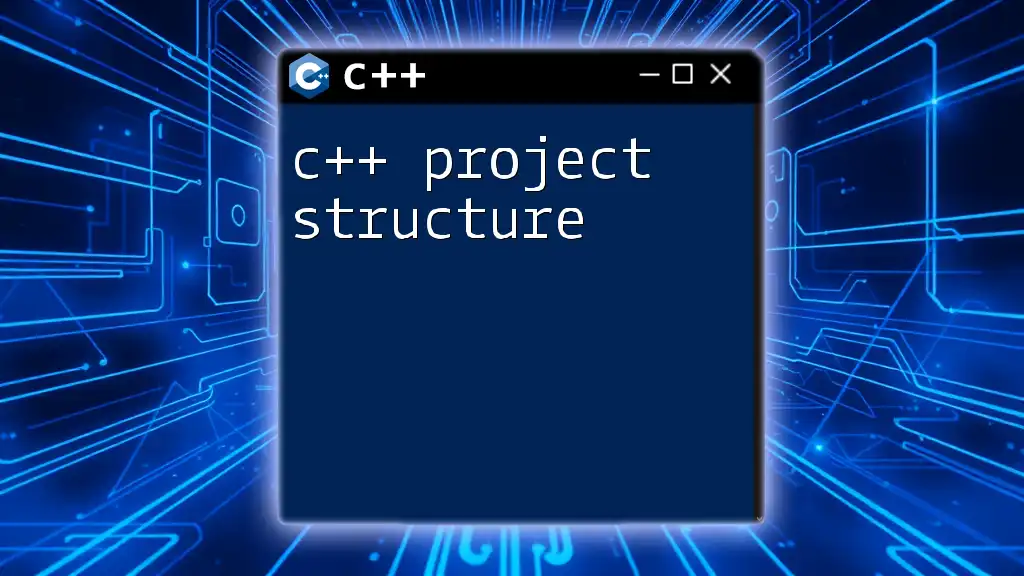
Choosing the Right Data Structure
Factors to Consider
When selecting a data structure, consider factors like:
- Time Complexity: Analyze the efficiency of operations you intend to perform.
- Space Complexity: Ensure your application manages memory efficiently.
- Use Cases: Choose data structures that align best with the problem you're solving; for instance, trees are ideal for hierarchical data, while hash tables excel in fast lookups.
Common Mistakes to Avoid
Avoid using complex data structures for simple tasks. Overengineering your solution can lead to increased coding errors and maintenance difficulties. Always choose the simplest, most effective structure for your use case.
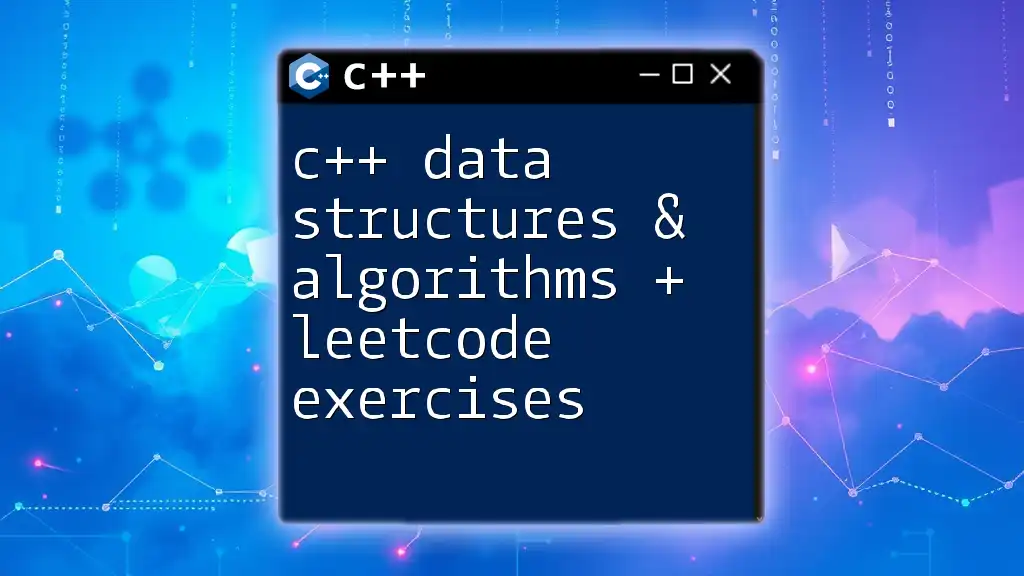
Performance Analysis
Big O Notation
For efficient algorithm design, understanding Big O notation is vital. This notation expresses the relationship between input size and the time or space required for an algorithm. Familiarity with common complexities such as O(1), O(n), O(n log n), and O(n²) can guide you in making informed decisions regarding data structures.
Best Practices for Optimization
To optimize performance:
- Profile your application's bottlenecks and adjust data structures accordingly.
- Favor structures that minimize access times, such as hash tables for quick lookups.
- Regularly review your usage of data structures throughout development to ensure they still meet your application's needs.
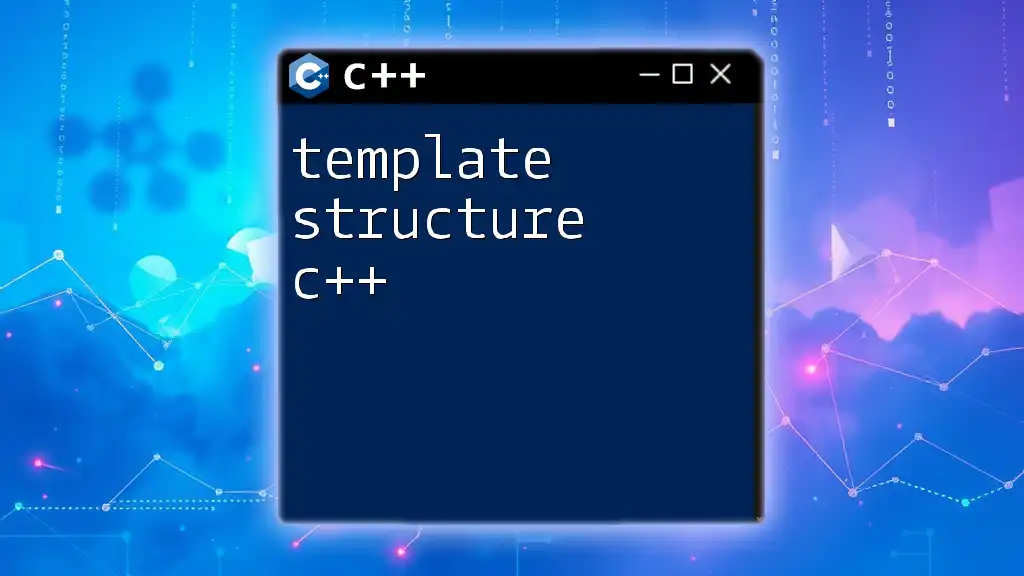
Conclusion
Mastering C++ plus data structures is essential for developing efficient and effective programming solutions. Understanding the various data structures and their applications can greatly enhance your coding capabilities and problem-solving skills.
Call to Action
Dive into practice with the code snippets provided. Implement the data structures discussed and experiment with their operations to strengthen your understanding. For more insights and resources on C++ data structures, consider exploring tutorials, books, and online courses dedicated to this essential topic.
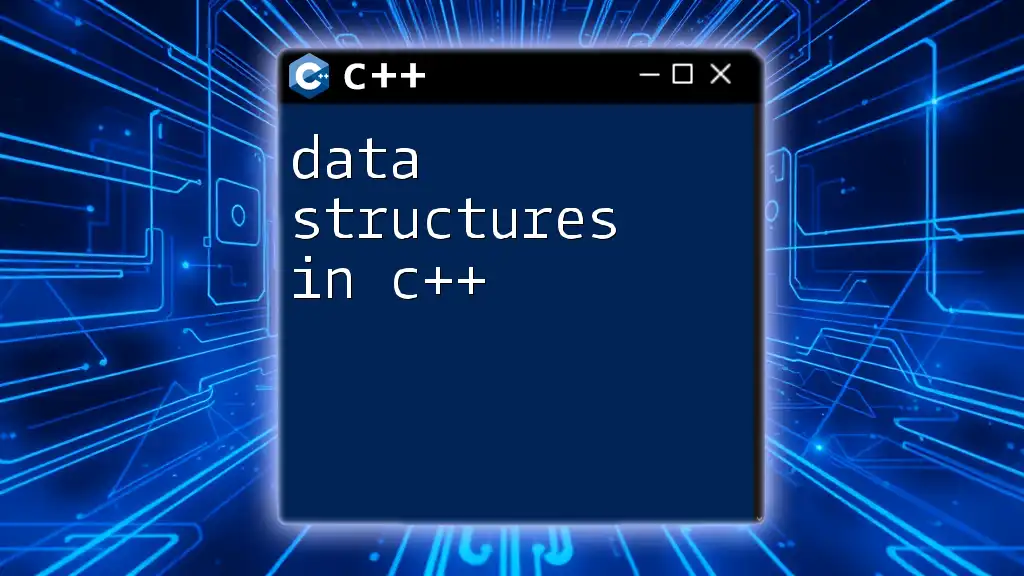
Additional Resources
For further exploration, consider checking out recommended books on data structures and C++, online tutorials, and coding platforms that offer practical exercises. These resources can greatly enhance your learning experience and enhance your mastery of C++ and data structures.