C++ data structures and algorithms are essential components that enable efficient storage, organization, and manipulation of data, utilizing various structures like arrays, linked lists, stacks, and sorting algorithms to optimize performance.
Here’s a simple example of a C++ program that demonstrates the use of a stack data structure:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
// Push elements onto the stack
myStack.push(1);
myStack.push(2);
myStack.push(3);
// Pop an element from the stack
myStack.pop();
// Display the top element
std::cout << "Top element: " << myStack.top() << std::endl;
return 0;
}
What Are Data Structures?
Definition of Data Structures
Data structures are systematic ways to organize and manage data so that it can be accessed and modified efficiently. Understanding C++ data structures is crucial because it allows programmers to handle data more effectively, enhancing performance and reducing complexity in software programs.
Types of Data Structures
Linear Data Structures
Arrays
An array is a collection of elements identified by index or key. It is one of the simplest forms of data structures and provides a way to group multiple elements of the same type.
int arr[5] = {10, 20, 30, 40, 50}; // Declaration and initialization
Arrays are particularly useful for storing a known number of elements. However, they have a fixed size, which can lead to waste of space or difficulty when resizing.
Linked Lists
A linked list consists of nodes, where each node contains data and a pointer to the next node. Linked lists can dynamically grow and shrink, unlike arrays.
struct Node {
int data;
Node* next;
};
Node* head = nullptr; // Starting with an empty list
Linked lists have advantages in terms of insertions and deletions, but they may consume more memory due to overhead from pointers.
Stacks
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means the most recently added element is the first to be removed.
#include <stack>
std::stack<int> myStack;
myStack.push(10); // Add element
myStack.pop(); // Remove last added element
The stack is widely used in function calls and manages to backtrack operations efficiently.
Queues
A queue is another linear data structure that operates on a First In, First Out (FIFO) basis. The first element added is the first one to be removed.
#include <queue>
std::queue<int> myQueue;
myQueue.push(10); // Add element
myQueue.pop(); // Remove the first added element
Queues are essential in scenarios where you need to manage tasks in order, such as in CPU scheduling.
Non-Linear Data Structures
Trees
A tree is a hierarchical data structure consisting of nodes, where the top node is called the root and each node can link to one or more child nodes.
A common type is the Binary Search Tree (BST), where each node has at most two children and maintains sorted order:
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
};
TreeNode* root = nullptr; // Initial root node
Trees are excellent for representing sorted data and providing quick search capabilities.
Graphs
A graph is a collection of nodes (vertices) connected by edges. Graphs can represent a wide range of real-world problems, like social networks or routes on a map.
#include <vector>
#include <iostream>
class Graph {
public:
std::vector<std::vector<int>> adjList;
Graph(int vertices) {
adjList.resize(vertices);
}
};
Graph myGraph(5); // Create a graph with 5 vertices
Graphs can be either directed or undirected, and understanding these structures plays a significant role in dynamic problem-solving.
Choosing the Right Data Structure
Selecting the appropriate data structure is vital for efficient data management. Factors to consider include:
- Type of data: Is it static or dynamic?
- Operations needed: How often will you insert, delete, or access data?
- Memory constraints: Are you limited in space?
A comparison of performance (time and space complexity) is also essential to make informed decisions.
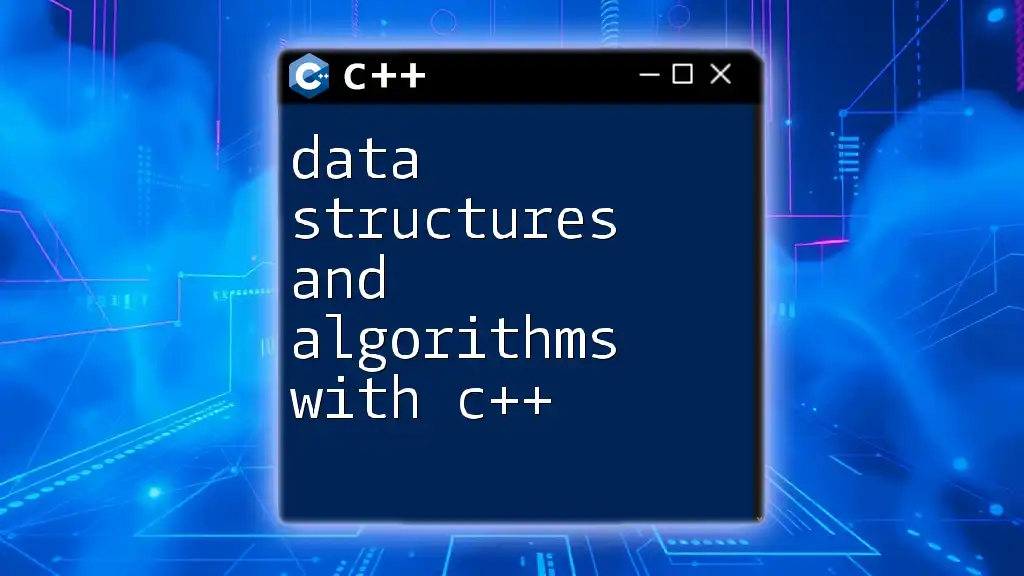
What Are Algorithms?
Definition of Algorithms
An algorithm is a step-by-step procedure or formula for solving a problem. In the context of C++ data structures and algorithms, understanding algorithms is crucial for creating efficient and effective solutions.
Types of Algorithms
Sorting Algorithms
Bubble Sort
Bubble sort is a straightforward sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. Though easy to understand, its efficiency is low in large datasets.
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
std::swap(arr[j], arr[j+1]);
}
}
}
}
Quick Sort
Quick sort is a more efficient sorting algorithm that works on the divide-and-conquer principle. It divides the array into smaller subsets, sorts them and combines them.
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
std::swap(arr[i], arr[j]);
}
}
std::swap(arr[i + 1], arr[high]);
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
Searching Algorithms
Linear Search
Linear search is the simplest searching algorithm that checks each element until it finds a match or reaches the end.
bool linearSearch(int arr[], int size, int key) {
for (int i = 0; i < size; i++) {
if (arr[i] == key) {
return true; // Found
}
}
return false; // Not found
}
Binary Search
Binary search works on sorted arrays, dividing the search interval in half repeatedly.
bool binarySearch(int arr[], int low, int high, int key) {
if (high >= low) {
int mid = low + (high - low) / 2;
if (arr[mid] == key)
return true; // Found
if (arr[mid] > key)
return binarySearch(arr, low, mid - 1, key); // Search left
return binarySearch(arr, mid + 1, high, key); // Search right
}
return false; // Not found
}
Algorithm Complexity
Big O Notation
Big O notation provides a way to classify algorithms according to how their run time or space requirements grow as the input size grows. It is essential for evaluating the efficiency of algorithms.
Common complexities include:
- O(1) - Constant time
- O(n) - Linear time
- O(log n) - Logarithmic time
- O(n^2) - Quadratic time
Understanding these complexities helps in selecting the right algorithm for the job.
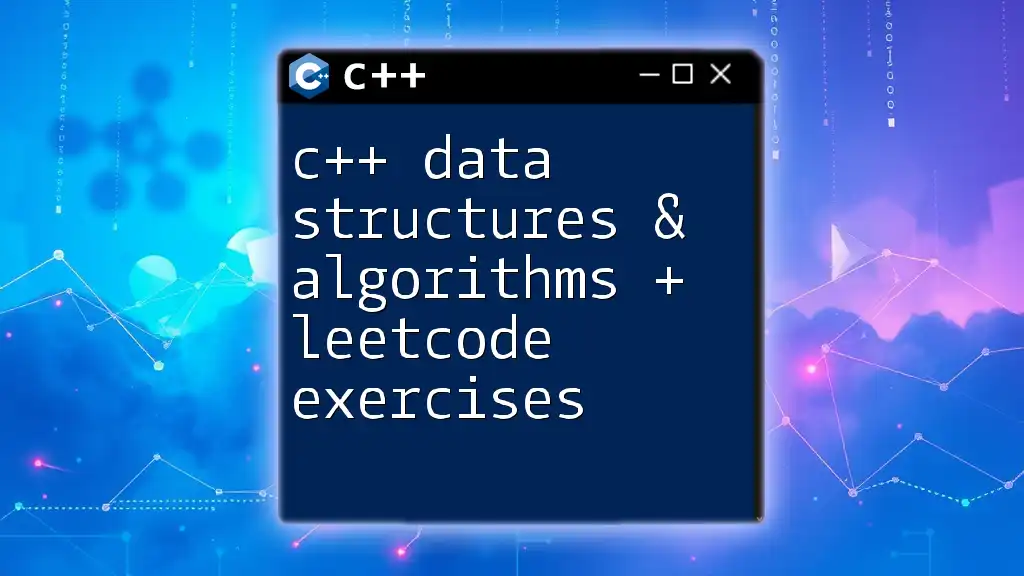
Data Structures and Algorithms in C++
Implementing Data Structures in C++
Using STL (Standard Template Library)
The Standard Template Library in C++ offers a rich set of built-in data structures such as vectors, lists, and maps. Using STL can significantly reduce development time and improve code reliability.
For example, using a vector:
#include <vector>
std::vector<int> myVector = {1, 2, 3, 4, 5};
myVector.push_back(6); // Adding an element at the end
The STL also includes powerful algorithms that work seamlessly with its data structures, showcasing a clear advantage for C++ programmers.
Implementing Algorithms in C++
While STL provides many algorithms, custom implementations may be needed in some scenarios. Knowing C++ data structures and algorithms allows you to optimize solutions for specific problem requirements.
Custom implementations allow greater flexibility and can be fine-tuned for performance based on constraints.
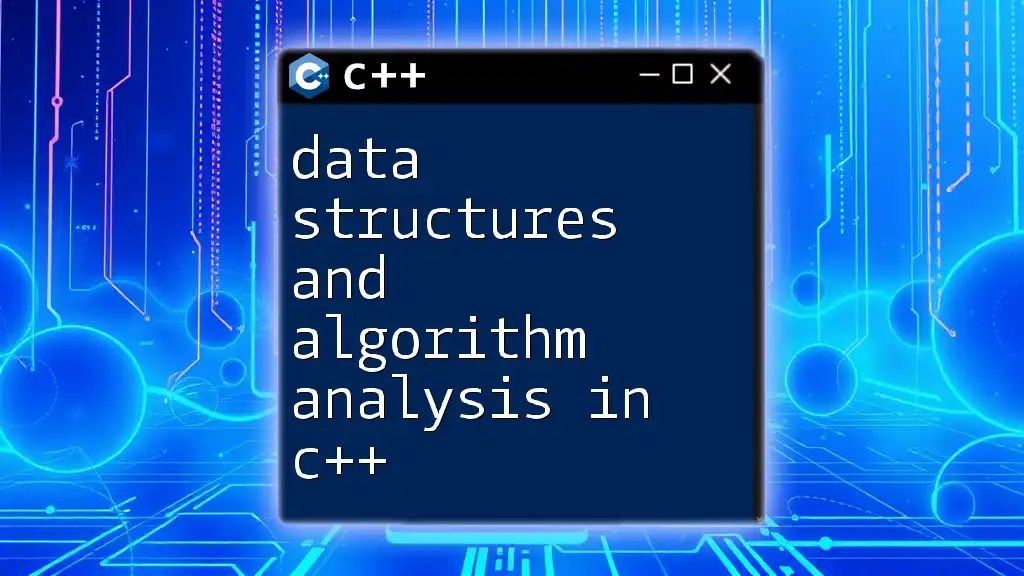
Best Practices When Working with Data Structures and Algorithms
To harness the full power of C++ data structures and algorithms, consider the following best practices:
-
Write Clean Code: Use meaningful variable names and maintain clarity throughout your code. This makes your code easily understandable and maintainable.
-
Test Thoroughly: Always test edge cases to ensure your algorithms handle unexpected input correctly. Testing will help validate your implementations.
-
Analyze Performance: Regularly check the time and space complexities of your algorithms as you develop. This awareness helps you catch potential inefficiencies early.
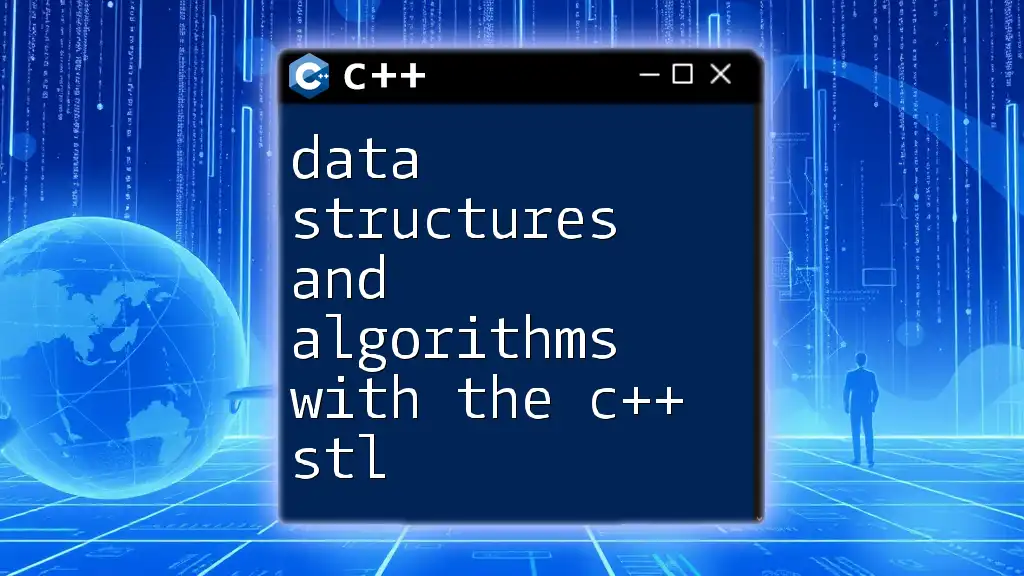
Conclusion
In conclusion, mastering C++ data structures and algorithms is fundamental for any programmer looking to enhance their problem-solving skills and write efficient code. It is imperative to practice these concepts continually, as they form the backbone of software development.
Pursuing advanced topics in data structures and algorithms can further refine your skills, leading to improved design patterns and efficient solutions to complex problems.