"Data Structures & Algorithm Analysis in C++ (4th Edition) provides a comprehensive guide to implementing efficient data structures and algorithms in C++ through clear explanations and practical examples."
Here’s a simple code snippet demonstrating a basic implementation of a stack using C++:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
// Pushing elements onto the stack
myStack.push(10);
myStack.push(20);
myStack.push(30);
// Accessing the top element
std::cout << "Top element: " << myStack.top() << std::endl;
// Popping the top element
myStack.pop();
std::cout << "New top after popping: " << myStack.top() << std::endl;
return 0;
}
Introduction to Data Structures and Algorithm Analysis
What are Data Structures?
Data structures are specialized formats for organizing, processing, and storing data. They are fundamental to computer science and programming, serving as the backbone for efficient data handling. By understanding data structures, developers can optimize performance and create more efficient applications.
Real-world applications include databases, operating systems, and any software that requires systematic access to data. The choice of data structure can significantly impact the efficiency of an algorithm.
Understanding Algorithm Analysis
Algorithm analysis is the process of determining the computational complexity of algorithms, especially looking at their time complexity and space complexity.
- Time Complexity explains the amount of time an algorithm takes to complete as a function of the size of the input data. We often use Big O notation to describe this.
- Space Complexity reflects the amount of memory an algorithm needs relative to the input size.

Overview of C++ in the Context of Data Structures
Why Use C++?
C++ is a powerful language that provides a mix of low-level and high-level features, making it ideal for implementing complex data structures. It offers fine control over system resources and memory management, which is essential when working with data structures.
When compared to other languages, C++ balances performance with abstraction more effectively, allowing for both efficient execution and easier code maintenance.
C++ Standard Template Library (STL)
The STL is a powerful component of C++ that offers a rich set of template classes and algorithms for data handling. It includes predefined data structures like vectors, stacks, and queues, making it easier to implement complex data structures without starting from scratch.

Fundamental Data Structures in C++
Arrays
Definition and Characteristics
An array is a collection of items stored at adjacent memory locations. They are one of the simplest data structures, providing quick access to elements using an index.
- Static Arrays have a fixed size, determined at compile time.
- Dynamic Arrays can resize, often implemented using pointers.
Code Snippet: Array Creation and Manipulation
#include <iostream>
using namespace std;
int main() {
int arr[5]; // Static array
for (int i = 0; i < 5; i++) {
arr[i] = i + 1; // Assign values
}
cout << "Array elements: ";
for (int i = 0; i < 5; i++) {
cout << arr[i] << " "; // Accessing elements
}
return 0;
}
Linked Lists
Singly Linked Lists vs. Doubly Linked Lists
A linked list is a linear data structure composed of nodes. Each node contains data and a pointer to the next node in the sequence.
- Singly Linked Lists allow traversal in one direction, while Doubly Linked Lists allow traversal in both directions.
Code Snippet: Basic Linked List Operations
#include <iostream>
using namespace std;
struct Node {
int data;
Node* next;
};
void insert(Node*& head, int value) {
Node* newNode = new Node{value, head};
head = newNode;
}
void display(Node* head) {
while (head != nullptr) {
cout << head->data << " ";
head = head->next;
}
}
int main() {
Node* head = nullptr;
insert(head, 1);
insert(head, 2);
insert(head, 3);
cout << "Linked list elements: ";
display(head);
return 0;
}
Stacks
Definition and Applications
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. This means that the last element added is the first one to be removed. Stacks are used commonly in function call management, parsing expressions, and backtracking algorithms.
Code Snippet: Stack Implementation Using Arrays
#include <iostream>
#define MAX 100
using namespace std;
class Stack {
int arr[MAX];
int top;
public:
Stack() : top(-1) {}
void push(int x) {
if (top == MAX - 1) {
cout << "Stack Overflow";
return;
}
arr[++top] = x;
}
int pop() {
if (top == -1) {
cout << "Stack Underflow";
return -1;
}
return arr[top--];
}
};
int main() {
Stack s;
s.push(10);
s.push(20);
cout << "Popped element: " << s.pop() << endl;
return 0;
}
Queues
Definition and Real-World Applications
A queue is a linear data structure adhering to the First In, First Out (FIFO) principle, making it ideal for scenarios like task scheduling and print job management.
Code Snippet: Queue Implementation Using Linked Lists
#include <iostream>
using namespace std;
struct Node {
int data;
Node* next;
};
class Queue {
Node* front;
Node* rear;
public:
Queue() : front(nullptr), rear(nullptr) {}
void enqueue(int x) {
Node* newNode = new Node{x, nullptr};
if (rear) {
rear->next = newNode;
}
rear = newNode;
if (!front) {
front = rear;
}
}
int dequeue() {
if (!front) {
cout << "Queue Underflow";
return -1;
}
int data = front->data;
Node* temp = front;
front = front->next;
delete temp;
return data;
}
};
int main() {
Queue q;
q.enqueue(10);
q.enqueue(20);
cout << "Dequeued element: " << q.dequeue() << endl;
return 0;
}

Advanced Data Structures in C++
Trees
Introduction to Tree Data Structures
Trees are hierarchical structures consisting of nodes, where each node contains data and references to its children. The tree is used extensively in organizing data in a way that enables faster and more efficient search, insertion, and deletion operations.
Binary Trees and Binary Search Trees (BST)
A Binary Tree has two children at most, while a Binary Search Tree is a binary tree where left children are less than the parent node and right children are greater.
Code Snippet: Basic Tree Traversal Techniques
#include <iostream>
using namespace std;
struct Node {
int data;
Node* left;
Node* right;
};
void inorder(Node* node) {
if (node == nullptr) return;
inorder(node->left);
cout << node->data << " ";
inorder(node->right);
}
int main() {
Node* root = new Node{1, nullptr, nullptr};
root->left = new Node{2, nullptr, nullptr};
root->right = new Node{3, nullptr, nullptr};
cout << "Inorder traversal: ";
inorder(root);
// Typically would include cleanup here to delete allocated nodes
return 0;
}
Graphs
Understanding Graphs and Their Representations
A graph is a collection of nodes (vertices) connected by edges. Graphs can be directed or undirected, and they can be represented using adjacency lists or adjacency matrices.
Common Graph Algorithms
Algorithms for traversing graphs include Depth-First Search (DFS), which explores a branch to its deepest point before backtracking, and Breadth-First Search (BFS), which explores neighbors first before moving deeper.
Code Snippet: Graph Implementation and Traversal example
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
class Graph {
int V; // Number of vertices
vector<int>* adj; // Pointer to an array containing adjacency lists
public:
Graph(int V) {
this->V = V;
adj = new vector<int>[V];
}
void addEdge(int v, int w) {
adj[v].push_back(w);
}
void BFS(int s) {
vector<bool> visited(V, false);
queue<int> q;
visited[s] = true; // Mark the current node as visited
q.push(s);
while (!q.empty()) {
s = q.front();
cout << s << " ";
q.pop();
for (int i : adj[s]) {
if (!visited[i]) {
visited[i] = true;
q.push(i);
}
}
}
}
};
int main() {
Graph g(4);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
cout << "Breadth First Traversal starting from vertex 0: ";
g.BFS(0);
return 0;
}

Algorithm Analysis Techniques
Analyzing Algorithms
Understanding Time Complexity
The time complexity of an algorithm evaluates how its duration grows relative to the size of its input. Common cases—Best, Average, and Worst—help developers understand performance under different conditions.
Common Algorithm Strategies
Divide and Conquer
Definition and Examples
The divide and conquer strategy breaks a problem into smaller subproblems, solves each one independently, and then combines their solutions. Classic examples include Merge Sort and Quick Sort.
Code Snippet: Merge Sort Implementation in C++
#include <iostream>
#include <vector>
using namespace std;
void merge(vector<int>& arr, int left, int mid, int right) {
// Code for merging two halves
}
void mergeSort(vector<int>& arr, int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
int main() {
vector<int> arr = {12, 11, 13, 5, 6, 7};
mergeSort(arr, 0, arr.size() - 1);
// Output sorted array
return 0;
}
Dynamic Programming
Principles of Dynamic Programming
Dynamic programming (DP) is a method for solving complex problems by breaking them down into simpler subproblems and storing the results of these subproblems to avoid redundant computations.
Code Snippet: Example of Fibonacci Sequence Calculation
#include <iostream>
#include <vector>
using namespace std;
int fib(int n, vector<int>& memo) {
if (n <= 1) return n;
if (memo[n] != -1) return memo[n];
memo[n] = fib(n - 1, memo) + fib(n - 2, memo);
return memo[n];
}
int main() {
int n = 10;
vector<int> memo(n + 1, -1);
cout << "Fibonacci of " << n << " is: " << fib(n, memo) << endl;
return 0;
}
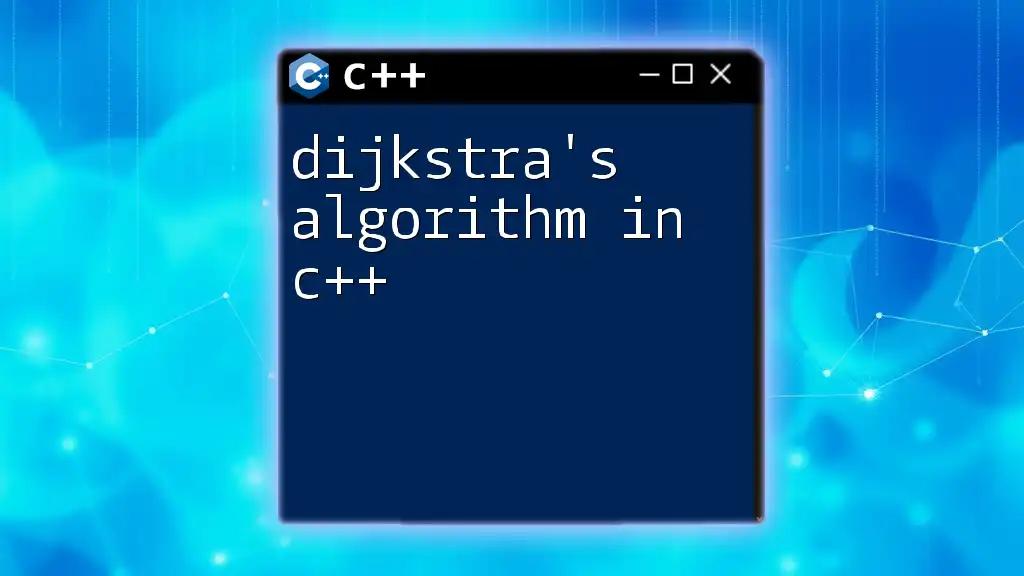
Best Practices for Using C++ with Data Structures
Memory Management and Avoiding Leaks
In C++, manual memory management can lead to leaks and segmentation faults. Using smart pointers (like `std::unique_ptr` and `std::shared_ptr`) from the C++ Standard Library can help manage resources automatically, preventing memory leaks.
Code Efficiency and Optimization Techniques
Focus on optimizing algorithms for better performance. Techniques like profiling—analyzing properties of the code to identify bottlenecks—are crucial. Always aim to minimize time and space complexity.

Conclusion
Mastering data structures and algorithms is crucial for every programmer and forms the foundation of writing efficient programs in C++. By understanding these fundamental components, you will not only enhance your coding skills but also prepare yourself for technical interviews and real-world problem-solving scenarios.
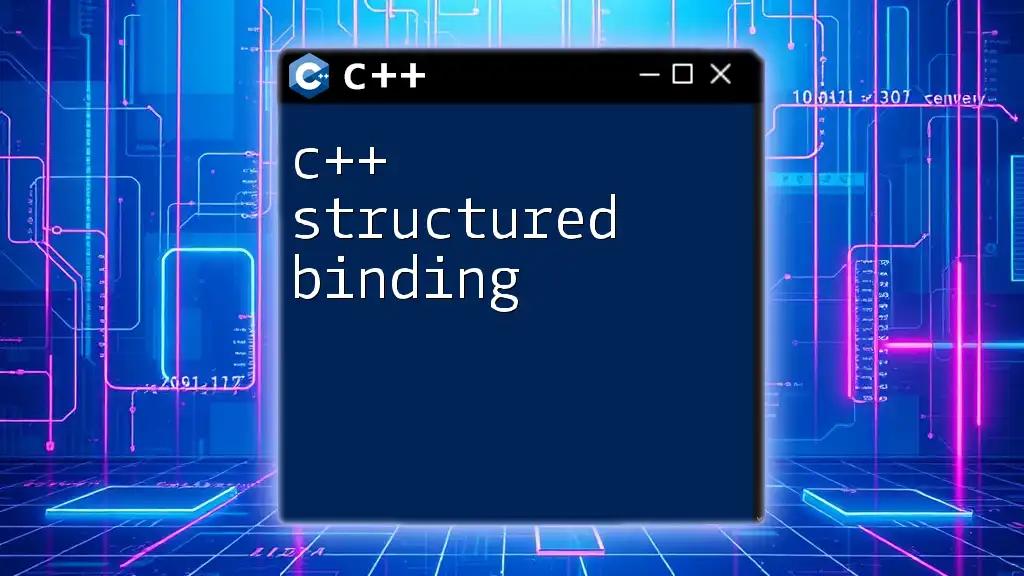
Frequently Asked Questions (FAQs)
What is the best way to learn C++ data structures?
Start by familiarizing yourself with the basic data structures and their implementations. Progress to more advanced structures and algorithms, practicing through coding challenges.
How do I practice implementing data structures in C++?
Utilize online platforms like LeetCode and HackerRank to solve problems that require the use of various data structures.
What are common mistakes to avoid when learning data structures?
Make sure to pay attention to memory management in C++. Avoid mixing static and dynamic allocations without proper handling, and don’t neglect algorithmic complexity when designing your code.

Additional Resources
Books, Online Courses, and Tutorials
Explore resources such as "Data Structures and Algorithm Analysis in C++ 4th Edition" and online platforms like Coursera or Udemy for structured learning paths.
Communities and Forums for C++ Learners
Join forums like Stack Overflow or Reddit's r/cpp for community support, where you can ask questions and share your knowledge with fellow C++ learners.