The Depth-First Search (DFS) algorithm in C++ is a recursive method used to explore all the vertices of a graph or tree by traversing as far down a branch as possible before backtracking.
Here's a simple code snippet demonstrating a DFS implementation in C++:
#include <iostream>
#include <vector>
using namespace std;
void DFS(int vertex, vector<bool> &visited, const vector<vector<int>> &graph) {
visited[vertex] = true; // Mark the current node as visited
cout << vertex << " "; // Print the current node
for (int neighbor : graph[vertex]) {
if (!visited[neighbor]) {
DFS(neighbor, visited, graph); // Recur for all the vertices adjacent to this vertex
}
}
}
int main() {
vector<vector<int>> graph = {
{1, 2}, // Vertices adjacent to 0
{0, 3}, // Vertices adjacent to 1
{0}, // Vertices adjacent to 2
{1} // Vertices adjacent to 3
};
vector<bool> visited(graph.size(), false);
DFS(0, visited, graph); // Start DFS from vertex 0
return 0;
}
Understanding Graphs: The Backbone of DFS
What is a Graph?
A graph is a fundamental data structure used in computer science to model relationships between entities. A graph comprises two main components:
- Nodes (or Vertices): The individual entities or points in the graph.
- Edges: The connections or relationships between pairs of nodes.
Graphs can be classified into different types:
- Undirected Graphs: The edges do not have a direction. The connection between nodes A and B is the same as the connection between B and A.
- Directed Graphs: The edges have a direction, typically represented as arrows. An edge from A to B does not imply an edge from B to A.
- Weighted Graphs: Each edge has an associated weight or cost, which is often used in optimization problems.
- Unweighted Graphs: Edges are considered equal without any associated weight.
Graph Representation in C++
When representing graphs in C++, two popular methods are commonly used: adjacency lists and adjacency matrices.
Adjacency List
An adjacency list maintains a collection of lists or vectors, where each list corresponds to a node and contains the nodes to which it is connected. This method is space-efficient, especially for sparse graphs.
Example representation using C++:
#include <iostream>
#include <vector>
class Graph {
public:
std::vector<std::vector<int>> adjList;
Graph(int nodes) {
adjList.resize(nodes);
}
void addEdge(int src, int dest) {
adjList[src].push_back(dest); // For directed graph; use both directions for undirected
}
};
Adjacency Matrix
An adjacency matrix consists of a 2D array where the cell at (i, j) indicates whether there is an edge from node i to node j. This representation is beneficial for dense graphs but can consume significant memory for large datasets.
Example representation using C++:
class Graph {
public:
std::vector<std::vector<int>> adjMatrix;
Graph(int nodes) {
adjMatrix.resize(nodes, std::vector<int>(nodes, 0));
}
void addEdge(int src, int dest) {
adjMatrix[src][dest] = 1; // For directed graph; set reciprocal for undirected
}
};
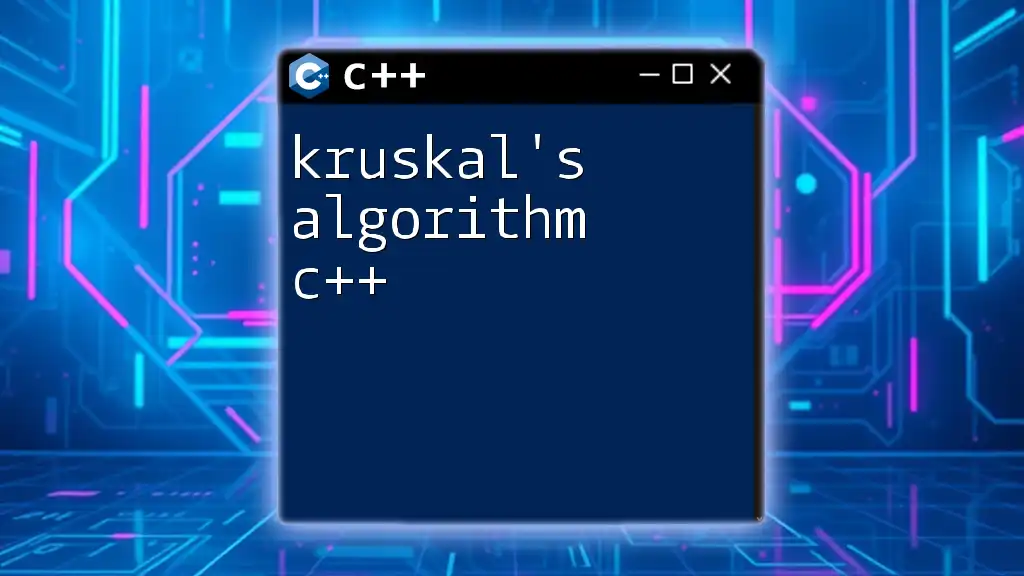
The Depth-First Search Algorithm
How DFS Works
The Depth-First Search (DFS) algorithm is a graph traversal technique that explores as far down a branch as possible before backtracking. This search method can be implemented using recursion or an explicit stack.
When performing DFS, the algorithm follows these steps:
- Start at a selected node (root).
- Mark the current node as visited.
- Explore each adjacent node that has not been visited.
- Recursively apply the same strategy until all nodes are visited.
DFS Algorithm Pseudocode
Below is a pseudocode representation of the DFS algorithm:
function DFS(node)
mark node as visited
for each neighbor in node's adjacency list
if neighbor is not visited
DFS(neighbor)
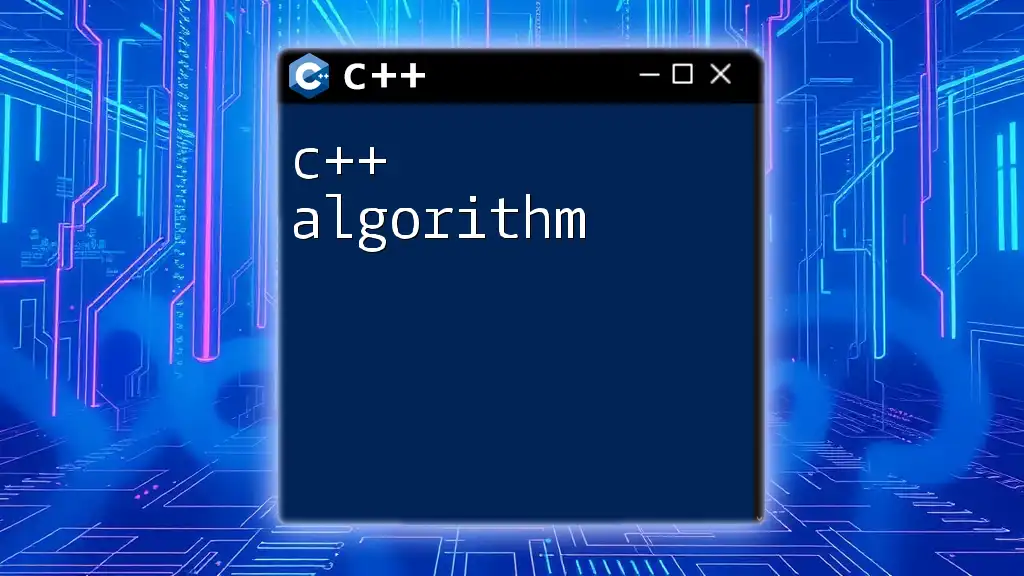
Implementing DFS in C++
Code Structure for DFS in C++
In this section, we will create a simple C++ program that demonstrates the DFS algorithm using an adjacency list.
Including Necessary Libraries
Start by including the necessary C++ headers:
#include <iostream>
#include <vector>
#include <stack>
Defining the Graph Class
Here, we extend our previous graph class with DFS implementation:
class Graph {
public:
std::vector<std::vector<int>> adjList;
Graph(int nodes) {
adjList.resize(nodes);
}
void addEdge(int src, int dest) {
adjList[src].push_back(dest);
}
void DFS(int startNode) {
std::vector<bool> visited(adjList.size(), false);
DFSUtil(startNode, visited);
}
private:
void DFSUtil(int node, std::vector<bool>& visited) {
visited[node] = true; // Mark the current node as visited
std::cout << node << " "; // Output the node (or perform any other operation)
for (int neighbor : adjList[node]) {
if (!visited[neighbor]) {
DFSUtil(neighbor, visited);
}
}
}
};
An Example of DFS Implementation
DFS Recursive Approach
The `DFS` function defined above is an implementation of the recursive approach. Here’s an example of how to use the `Graph` class:
int main() {
Graph g(5); // Create a graph with 5 nodes
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 3);
g.addEdge(1, 4);
std::cout << "DFS starting from node 0:\n";
g.DFS(0);
return 0;
}
This code initializes a graph, adds edges, and performs a DFS traversal starting from node 0.
DFS Iterative Approach
The iterative version of DFS can be implemented using a stack. This method avoids potential issues with recursion depth in case of large graphs. Here’s how to implement it:
void DFSIterative(int startNode) {
std::vector<bool> visited(adjList.size(), false);
std::stack<int> stack;
stack.push(startNode);
while (!stack.empty()) {
int node = stack.top();
stack.pop();
if (!visited[node]) {
visited[node] = true;
std::cout << node << " ";
for (auto neighbor : adjList[node]) {
if (!visited[neighbor]) {
stack.push(neighbor);
}
}
}
}
}
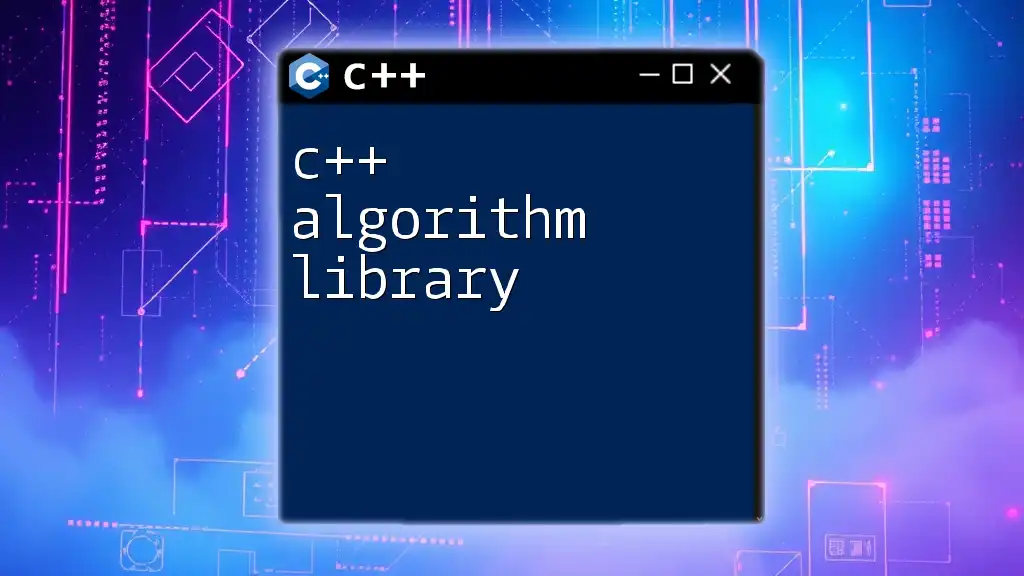
Practical Applications of DFS
Use Cases of DFS in Real-World Problems
DFS finds applications in various real-world scenarios:
- Exploring Paths in Mazes: DFS can be used to navigate through mazes and find paths from start to finish.
- Topological Sorting: In directed acyclic graphs (DAGs), DFS assists in ordering nodes such that for every directed edge from node A to node B, A appears before B in the ordering.
- Finding Connected Components: DFS helps determine different connected components in a graph, which is useful in network connectivity problems.
Complexity Analysis of DFS
The time complexity of the DFS algorithm is O(V + E), where V is the number of vertices and E is the number of edges. This complexity arises because every vertex and edge will be processed once. In terms of space complexity, it is O(V) due to the storage of visited nodes and the stack for recursion.
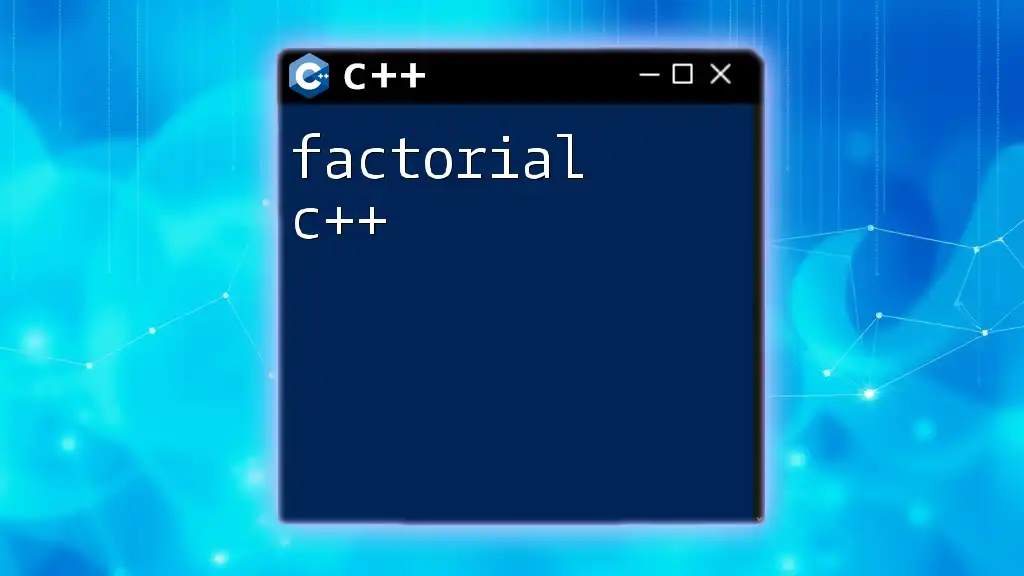
Testing and Debugging Your DFS Implementation
Common Pitfalls and Solutions
When implementing DFS in C++, some common issues include:
- Not marking nodes as visited: This can lead to infinite loops. Always ensure that a node is marked as visited before further exploration.
- Incorrectly defined graph edges: Ensure that edges are defined accurately for your specific use case, especially in directed and undirected graphs.
Unit Testing Your DFS Algorithm
Testing your DFS implementation ensures correctness and robustness. Consider the following test cases:
// Create a graph and add edges for testing
Graph testGraph(5);
testGraph.addEdge(0, 1);
testGraph.addEdge(0, 2);
testGraph.addEdge(1, 3);
testGraph.addEdge(2, 4);
// Expected output for DFS starting from node 0 should cover all nodes
testGraph.DFS(0); // Check if all nodes are visited in the expected order
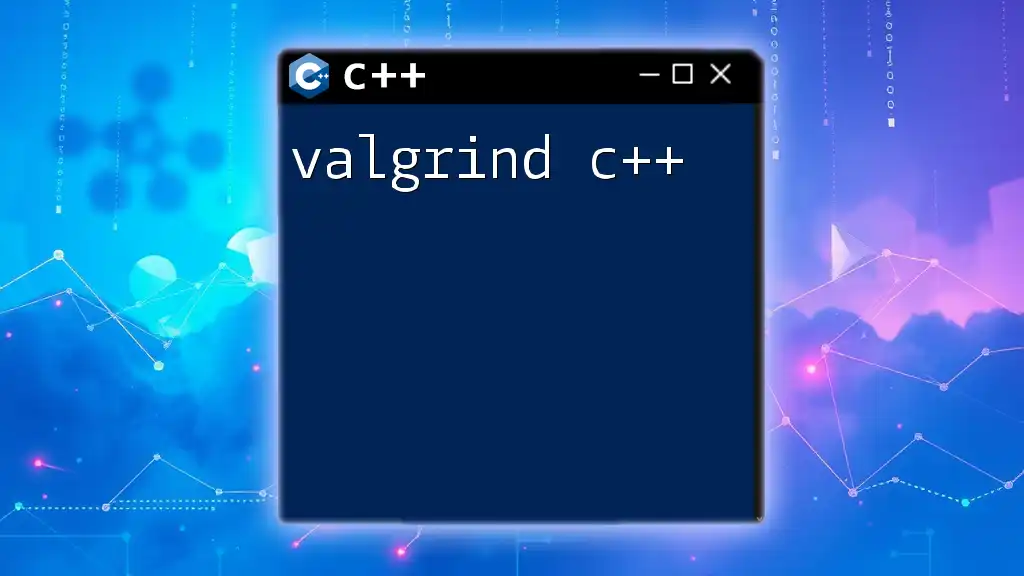
Conclusion
In this guide, we explored the DFS algorithm in C++. We discussed its fundamental concepts, implementation, practical applications, and how to effectively test your solution. Understanding DFS will not only deepen your knowledge of graph algorithms but also enhance your problem-solving skills in various applications. As you continue to journey through the world of C++, consider exploring more complex problems and optimization techniques to further strengthen your grasp on this powerful programming language.