The C++ Standard Template Library (STL) provides a set of generic algorithms that work with containers, allowing for efficient manipulation and processing of data; for example, the `std::sort` algorithm can be used to sort a vector of integers.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {4, 2, 5, 1, 3};
std::sort(numbers.begin(), numbers.end());
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
Understanding STL Algorithms
What are STL Algorithms?
STL algorithms refer to a set of pre-defined functions in the C++ Standard Template Library (STL) that perform various operations on containers like vectors, lists, and arrays. These algorithms embody common tasks—including searching, sorting, and transforming—that can be executed efficiently and succinctly.
The significance of STL algorithms lies in their ability to improve code efficiency and reduce development time. By leveraging these established functions, programmers can avoid rewriting common functionalities and focus on more complex tasks, thereby enhancing productivity.
Types of STL Algorithms
STL algorithms can be broadly categorized into four types:
- Non-modifying sequence algorithms: These do not alter the content of the container but may provide useful information derived from it.
- Modifying sequence algorithms: These change the content of the container. They can manipulate, remove, or insert data.
- Sorting algorithms: As the name implies, these algorithms organize data in a specific order, either ascending or descending.
- Numeric algorithms: These conduct mathematical operations on the elements within the container, such as summation or the computation of products.
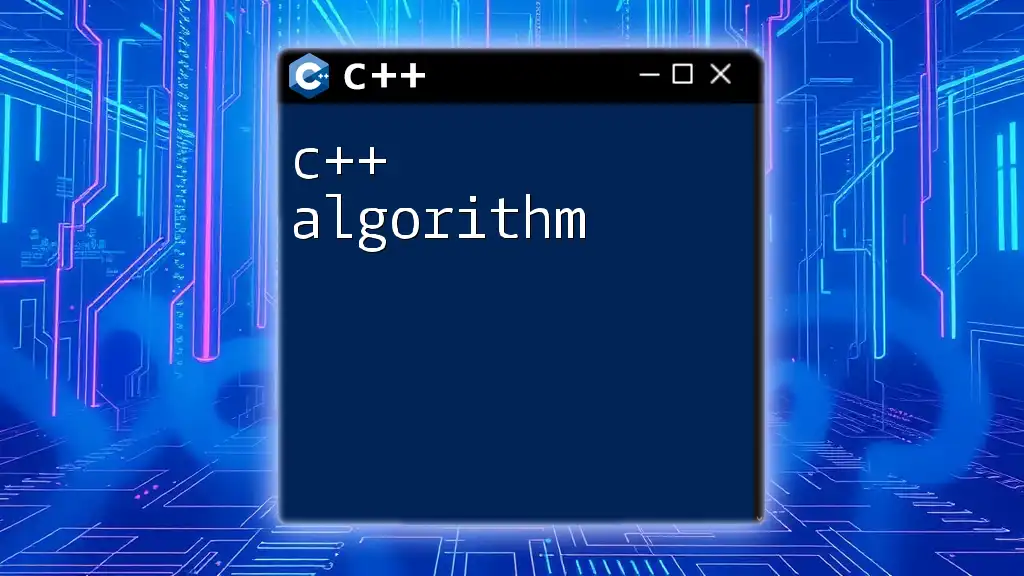
Common STL Algorithms and Their Usage
for_each
The `for_each` algorithm applies a specified function to each element in a given range. This is particularly useful for performing operations without writing boilerplate code to iterate through containers.
Code Example:
#include <iostream>
#include <vector>
#include <algorithm>
void print(int n) {
std::cout << n << " ";
}
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::for_each(vec.begin(), vec.end(), print);
return 0;
}
In this example, `for_each` traverses through the vector `vec` and applies the `print` function to each element, resulting in the output `1 2 3 4 5`. This illustrates the power of STL algorithms to simplify tedious iterative processes.
transform
The `transform` algorithm is designed to apply a function to a range of elements and store the result in a different range. This is useful for modifying data in a straightforward manner.
Code Example:
#include <iostream>
#include <vector>
#include <algorithm>
int square(int n) {
return n * n;
}
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int> squared_vec(vec.size());
std::transform(vec.begin(), vec.end(), squared_vec.begin(), square);
for (int n : squared_vec) {
std::cout << n << " ";
}
return 0;
}
Here, `transform` is used to square each element of `vec` and store the results in `squared_vec`. The output will be `1 4 9 16 25`, effectively demonstrating how `transform` enables clear manipulations of data.
sort
The `sort` algorithm arranges the elements of a container in ascending order by default, but custom orderings can also be specified.
Code Example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {5, 3, 4, 1, 2};
std::sort(vec.begin(), vec.end());
for (int n : vec) {
std::cout << n << " ";
}
return 0;
}
In this example, invoking `sort` on the vector `vec` sorts the elements from least to greatest, yielding the output `1 2 3 4 5`. Sorting is fundamental in many applications, and using STL algorithms ensures efficiency in performance.
find
The `find` algorithm searches for a specified element in a range and returns an iterator pointing to that element, or `end` if it is not found.
Code Example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Element found: " << *it;
} else {
std::cout << "Element not found.";
}
return 0;
}
In the code above, the `find` algorithm looks for the number `3` in `vec`, printing "Element found: 3" if successful. This highlights how STL algorithms can simplify searching tasks while maintaining code readability.
accumulate
The `accumulate` function, part of numeric algorithms, computes the sum of a range of values, providing a convenient way to perform basic arithmetic operations.
Code Example:
#include <iostream>
#include <vector>
#include <numeric>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int sum = std::accumulate(vec.begin(), vec.end(), 0);
std::cout << "Sum: " << sum;
return 0;
}
In this case, `accumulate` calculates the total of the elements in `vec`, producing the output `Sum: 15`. This demonstrates how numeric algorithms can be seamlessly integrated into everyday C++ coding.
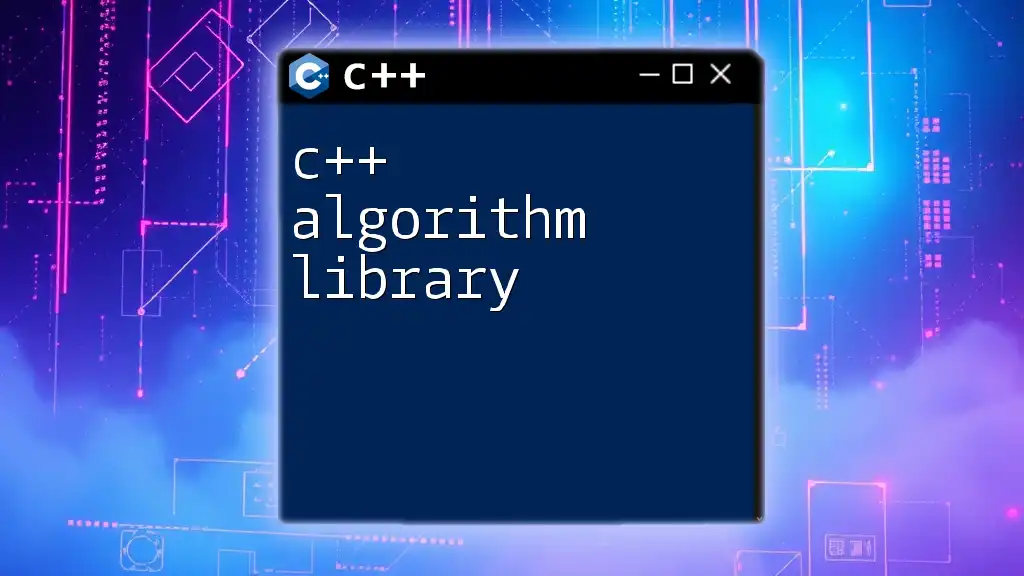
Combining Algorithms for Enhanced Functionality
One of the key strengths of STL algorithms is their ability to be combined to achieve more complex behaviors without compromising code clarity. For instance, one might sort a vector and then apply a transformation in a single workflow.
Example workflow:
std::sort(vec.begin(), vec.end());
std::transform(vec.begin(), vec.end(), vec.begin(), square);
This combination first sorts the data in `vec` and subsequently squares each element. By chaining algorithms like this, programmers can create powerful and concise solutions.
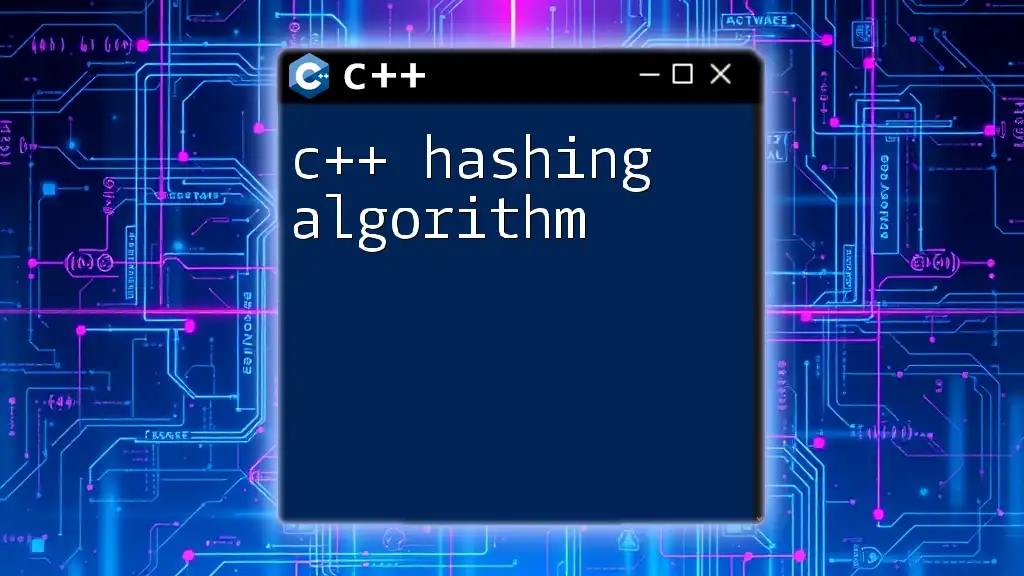
Best Practices for using STL Algorithms
Performance Considerations
When utilizing STL algorithms, it is important to consider their time complexity and overall efficiency. Most STL algorithms are designed with optimal performance in mind, often adhering to O(n log n) for sorting and O(n) for searching and transforming, making them suitable for a variety of applications.
Readability and Maintainability
Using STL algorithms contributes significantly to code readability. By employing well-known function names such as `sort`, `accumulate`, or `find`, the intention behind the code becomes immediately clear. It’s equally important to use meaningful names for any lambda functions created, ensuring that even complex functionality remains easy to understand.
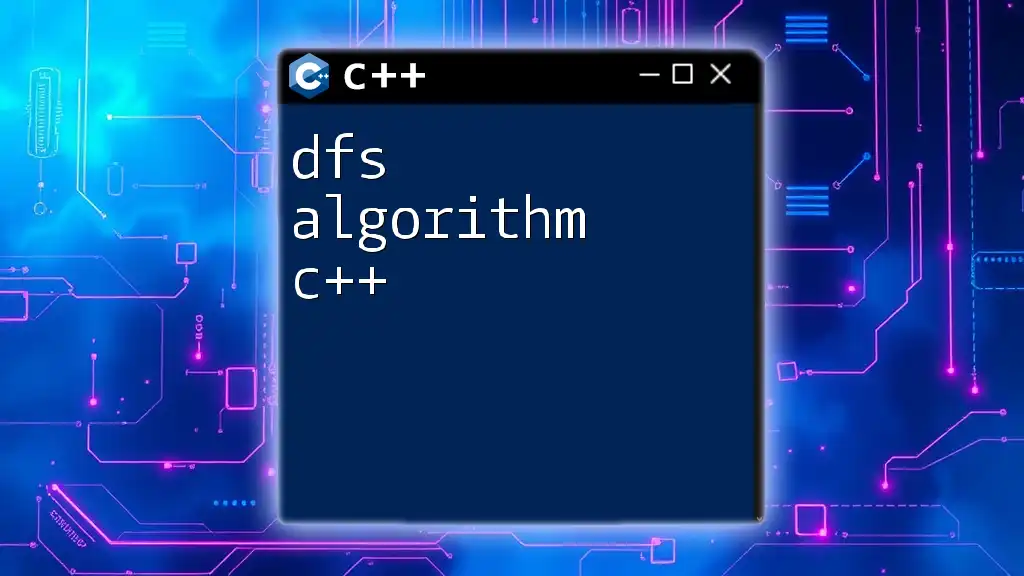
Conclusion
C++ STL algorithms play a vital role in enhancing programming efficiency, offering ready-made solutions for repeatedly necessary tasks. By understanding and applying these algorithms, programmers can write cleaner, more maintainable code while significantly boosting performance. Engaging with STL algorithms opens horizons for innovative programming practices—encouraging further exploration and experimentation in C++. Consider challenging yourself with practice exercises or real-world projects to cement your understanding of C++ STL algorithms.