In this post, we'll explore essential C++ data structures and algorithms through practical LeetCode exercises to enhance your problem-solving skills.
Here’s a simple example of using a vector in C++ to store integers and print them:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Data Structures
What are Data Structures?
Data structures are specialized formats that define how to store, organize, and manage data. They play a crucial role in programming, helping developers efficiently handle data during computational tasks. Choosing the right data structure can mean the difference between an efficient solution and one that is prohibitively slow or memory-intensive.
Common Data Structures in C++
Arrays
Arrays are one of the simplest and most widely used data structures. They consist of a fixed-size sequence of elements, all of which must be of the same type. An array allows for efficient access to its elements via indexing.
Example: Declaring an Array
int arr[5] = {1, 2, 3, 4, 5};
This declaration creates an array of five integers. While arrays allow for fast access (O(1) time complexity), they have fixed sizes, which can be a limitation in dynamic scenarios.
Linked Lists
A linked list is a data structure that consists of nodes, where each node contains data and a pointer to the next node in the sequence. This structure allows for efficient insertions and deletions compared to arrays.
Types of Linked Lists:
- Singly Linked List: Nodes point to the next node only.
- Doubly Linked List: Nodes point to both the next and previous nodes.
- Circular Linked List: The last node points back to the first node.
Example: Simple Linked List Implementation
struct Node {
int data;
Node* next;
};
Node* head = nullptr; // Start with an empty list
When to Use Linked Lists: Use linked lists when frequent insertions and deletions occur, especially when the size of the list is unknown.
Stacks
Stacks are collections that adhere to the Last In First Out (LIFO) principle. This means that the last element added to the stack will be the first to be removed. Stacks are useful in scenarios like reversing strings or implementing recursive functions.
Operations on Stacks:
- Push: Add an element to the top.
- Pop: Remove the top element.
- Peek: Get the top element without removing it.
Code Example: Implementing a Stack
#include <stack>
std::stack<int> myStack;
// Push elements
myStack.push(10);
myStack.push(20);
// Pop an element
myStack.pop(); // Removes 20
Applications of Stacks: Stacks are commonly used in algorithm implementations for function calls and backtracking.
Queues
Queues are collections that utilize the First In First Out (FIFO) principle. The first element added is the first to be removed. They are essential for managing tasks in order of arrival.
Operations on Queues:
- Enqueue: Add an element to the back.
- Dequeue: Remove the front element.
- Front: Retrieve the front element without removing it.
Code Example: Implementing a Queue
#include <queue>
std::queue<int> myQueue;
// Enqueue elements
myQueue.push(1);
myQueue.push(2);
// Dequeue an element
myQueue.pop(); // Removes 1
Uses of Queues: They are vital in task scheduling and buffering mechanisms, such as IO buffering in operating systems.
Trees
Trees are hierarchical data structures with a root value and subtrees. They provide efficient ways to represent data that have a natural hierarchical relationship.
Types of Trees:
- Binary Tree: Each node has two children at most.
- Binary Search Tree (BST): A binary tree in which the left child contains values less than the parent, and the right child contains values greater.
- AVL Tree: A self-balancing binary search tree.
Example: Simple Binary Tree Implementation
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
};
TreeNode* root = nullptr; // Start with an empty tree
When to Use Trees: Trees are optimal for representing hierarchical data, conducting searches, and sorting.
Graphs
Graphs are collections of nodes connected by edges. They form a powerful basis for modeling relationships between entities, such as social networks.
Representation of Graphs:
- Adjacency List: A list where each element points to a list of connected vertices.
- Adjacency Matrix: A 2D array that uses rows and columns to represent connections.
Code Example: Simple Graph Representation Using Adjacency List
#include <vector>
std::vector<std::vector<int>> graph(5); // A graph with 5 nodes
When to Use Graphs: Graphs are used in networking, route planning, and relationship mapping in data.

Mastering Algorithms
What are Algorithms?
Algorithms are sequential steps or procedures used for solving computational problems. They are crucial in deriving an efficient solution and optimizing performance. The choice of algorithm depends on the problem type and constraints.
Common Algorithmic Techniques
Sorting Algorithms
Sorting algorithms arrange elements in a specific order. The choice of sorting algorithm can significantly impact performance based on data size and initial order.
Types of Sorting Algorithms:
- Bubble Sort: Simple but inefficient for large datasets.
- Merge Sort: A divide-and-conquer algorithm with a time complexity of O(n log n).
- Quick Sort: Also follows a divide-and-conquer strategy with an average time complexity of O(n log n).
Code Example: Basic Implementation of Quick Sort
void quickSort(int arr[], int low, int high) {
// Implementation of quick sort algorithm
}
Time Complexity: Understanding the time and space complexities of sorting algorithms is vital for optimizing applications.
Searching Algorithms
Searching algorithms are designed to retrieve an item from a data structure. The efficiency of these algorithms can drastically affect performance based on data size and organization.
Types of Searching Algorithms:
- Linear Search: Checks each element one by one (O(n) complexity).
- Binary Search: Requires sorted data and divides the search space by half at each step (O(log n) complexity).
Code Example: Implementing Binary Search
int binarySearch(int arr[], int size, int target) {
int low = 0, high = size - 1;
while (low <= high) {
int mid = low + (high - low) / 2;
if (arr[mid] == target) return mid; // Target found
if (arr[mid] < target) low = mid + 1; // Target is in the right half
else high = mid - 1; // Target is in the left half
}
return -1; // Target not found
}
Optimal Use Cases: Choosing the right algorithm depends on whether your dataset is small, large, or sorted.
Dynamic Programming
Dynamic programming is a mathematical optimization technique used extensively in algorithm design. It breaks problems down into simpler overlapping subproblems and stores their results to avoid redundant calculations.
Example Problem: Fibonacci Sequence Calculating Fibonacci numbers can be done efficiently using dynamic programming (memoization) instead of simple recursion, which has exponential time complexity.
int fibonacci(int n) {
if (n <= 1) return n;
int fib[n + 1];
fib[0] = 0;
fib[1] = 1;
for (int i = 2; i <= n; i++)
fib[i] = fib[i - 1] + fib[i - 2]; // Memoization step
return fib[n];
}
Use Cases in Competitive Programming: Employed for problems involving optimization and resource allocation.

Integrating LeetCode Exercises
Why LeetCode?
LeetCode is a widely recognized platform dedicated to algorithm and data structure problems. It provides an extensive library of questions that help sharpen your coding skills and prepare for technical interviews.
Recommended Data Structure Problems on LeetCode
LeetCode offers numerous problems specifically aimed at honing your understanding of data structures. These problems often mimic real-world scenarios, which are beneficial for preparing for coding interviews.
Example: "Add Two Numbers" is a classic problem that requires the use of linked lists. Mastering such problems is essential in solidifying your understanding of how to manipulate data structures effectively.
Recommended Algorithmic Problems on LeetCode
Alongside data structure problems, LeetCode offers a plethora of algorithmic challenges. These can range from sorting and searching to dynamic programming.
Example: "Two Sum" is a popular problem that requires utilizing arrays effectively to find pairs of numbers that add up to a given target. This problem emphasizes the importance of optimal searching techniques.
Tips for Solving LeetCode Problems
- Understand the Problem Statement: Ensure you grasp what’s being asked by reading carefully.
- Break Down the Problem: Start with small cases to formulate your approach.
- Optimize Your Solution: Always analyze the time and space complexity of your solution for potential improvements.
How to Approach a LeetCode Problem
- Choose the Right Data Structure: Analyze the problem and select a suitable data structure to represent the data.
- Outline the Algorithm: Before you start coding, outline the steps you intend to take to solve the problem.
- Implement and Test Edge Cases: Implement your solution and rigorously test it against various edge cases to ensure its robustness.
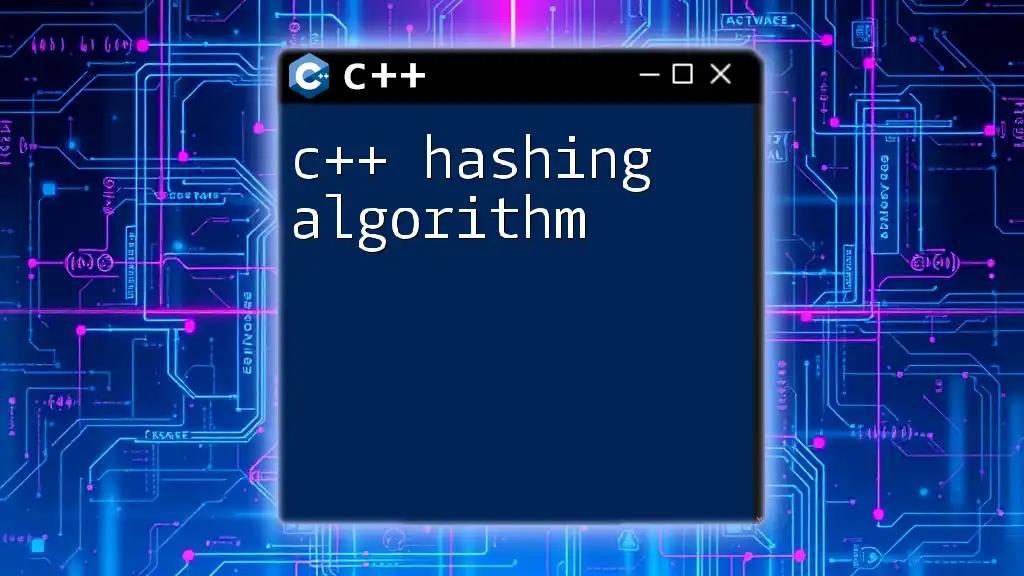
Conclusion
Mastering C++ data structures & algorithms + LeetCode exercises is vital for becoming an adept programmer. With a solid foundation in understanding these concepts, you are poised to tackle complex programming challenges and improve your coding skills. Engaging with platforms like LeetCode will not only enhance your ability to solve problems but also prepare you for technical interviews.
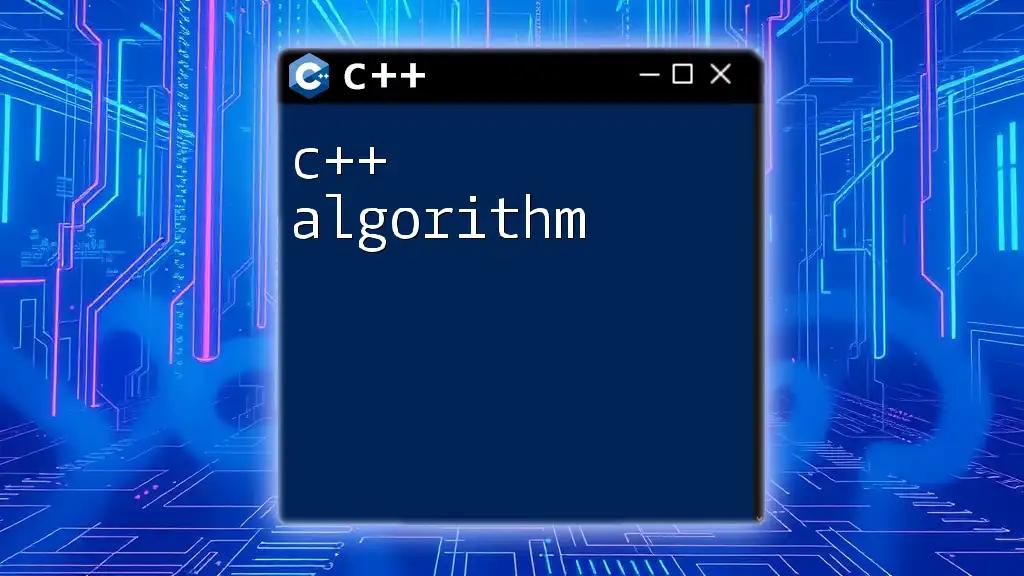
Additional Resources
To expand your knowledge further, consider delving into recommended books, online courses, and tutorials about data structures and algorithms. Joining coding communities and contributing to open-source projects can also provide valuable practical experience.

FAQs
Many aspiring programmers often have questions regarding the best practices for learning data structures and algorithms, how to effectively prepare for coding interviews, and which resources to use for optimal learning. Address these common queries for a more comprehensive understanding and guidance as you navigate the world of programming.