The C++ Standard Template Library (STL) provides a collection of powerful data structures and algorithms that simplify programming tasks such as sorting, searching, and managing data collections efficiently.
Here's a simple example demonstrating a vector and sorting algorithm in C++:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 2, 8, 3, 1};
std::sort(numbers.begin(), numbers.end());
for(int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is C++ STL?
C++ STL, which stands for Standard Template Library, is a powerful set of C++ template classes and functions aimed at providing generic classes for various data structures and algorithms. It is an invaluable resource for developers, significantly simplifying the process of building and managing collections of data.
Advantages of Using STL
Utilizing the STL brings several advantages to programmers:
- Efficiency: The STL is designed to reduce coding time considerably. Many data structures and algorithms have been pre-implemented, allowing developers to leverage these solutions without the need to write code from scratch.
- Performance: The STL offers highly optimized implementations, ensuring that operations on containers are performed with maximum efficiency. This performance advantage becomes particularly apparent when dealing with large datasets.
- Ease of Use: With ready-to-use templates, programmers can focus more on solving their particular problems rather than worrying about the intricacies of data structure implementation.

Key Components of C++ STL
Containers
Containers are essential building blocks in STL; they store and manage collections of data. They come in various types, each tailored for specific tasks.
Sequence Containers
Sequence containers maintain the order of elements and allow easy insertion and removal.
- Vector: A dynamic array that can resize itself, offering fast access by index.
- List: A doubly linked list, ideal for frequent insertions and deletions.
- Deque: A double-ended queue facilitating the addition or removal of elements from both ends.
Associative Containers
Associative containers store elements in a specific order based on keys, providing efficient access to elements.
- Set: A collection of unique elements, enabling quick lookups.
- Map: Stores key-value pairs, allowing retrieval of specific values based on their corresponding keys.
- Multiset: Similar to a set but allows multiple occurrences of elements.
- Multimap: Functions like a map but allows duplicate keys.
Unordered Containers
Unordered containers store elements in an unpredictable order, enabling fast access via hashing.
- Unordered Set: A collection of unique elements without a specific order.
- Unordered Map: Similar to a map but uses a hash table for quick access.
Algorithms
The STL also includes a variety of algorithms designed to operate on these containers. They greatly enhance the capabilities of STL, allowing complex operations to be performed effortlessly.
Searching Algorithms
Searching algorithms, such as binary search, are used to find specific values within a collection. For example, consider the following code snippet demonstrating a binary search:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int target = 3;
bool found = std::binary_search(numbers.begin(), numbers.end(), target);
std::cout << "Target " << (found ? "found" : "not found") << std::endl;
return 0;
}
This example checks for the presence of the number 3 within a sorted vector. The `std::binary_search` algorithm efficiently determines if the target exists.
Sorting Algorithms
Sorting algorithms facilitate organizing data in a particular order. The STL provides a straightforward method for sorting container contents:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 1, 4, 2};
std::sort(numbers.begin(), numbers.end());
for (int num : numbers) {
std::cout << num << " "; // Output sorted numbers
}
return 0;
}
In this example, the `std::sort` function organizes the numbers in ascending order.
Iterators
Iterators act as a bridge between algorithms and containers, enabling flexible data navigation. They provide a uniform method for accessing elements in various container types. Here are the main types of iterators found in the STL:
- Input Iterators: Read-only access to elements from the container.
- Output Iterators: Write-only access for inserting elements into a container.
- Forward Iterators: Allow traversing elements in one direction.
- Bidirectional Iterators: Enable movement through elements in both directions.
- Random Access Iterators: Provide the capability to access any element directly by using an index.

Practical Application of C++ STL Data Structures
Using Vectors
Vectors are one of the most versatile sequence containers in the STL. They provide dynamic size and fast access:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
for (int i : vec) {
std::cout << i << " ";
}
return 0;
}
This code snippet initializes a vector with some integer values and iterates through them, demonstrating the ease of use of vectors. Vectors are preferred when random access to elements is crucial, but frequent insertions and deletions in the middle can degrade performance.
Using Maps
Maps allow the storage of key-value pairs, where each key must be unique. Here’s a practical example:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
std::cout << "Alice's age: " << ageMap["Alice"] << std::endl;
return 0;
}
In this snippet, a map is created to store the ages of different individuals. Maps are incredibly useful for quick data retrieval based on specific keys, making them ideal for scenarios where associations between keys and values are needed.
Using Sets
Sets provide a way to store unique elements efficiently. Here’s how a set can be utilized:
#include <iostream>
#include <set>
int main() {
std::set<int> uniqueNumbers = {1, 2, 3, 2, 4};
for (int num : uniqueNumbers) {
std::cout << num << " "; // Output will be unique numbers
}
return 0;
}
In this example, duplicate numbers are automatically eliminated when stored in a set. Sets are particularly effective for applications where maintaining unique elements is necessary.

Understanding C++ STL Algorithms
Implementing Custom Data Structures
The STL containers can also serve as the foundation for developing custom data structures. This technique allows developers to implement higher-level abstractions while taking advantage of STL's efficiency. Here’s a simple class that utilizes a vector:
#include <iostream>
#include <vector>
class MyContainer {
private:
std::vector<int> data;
public:
void add(int value) {
data.push_back(value);
}
void display() {
for (int i : data) {
std::cout << i << " ";
}
}
};
int main() {
MyContainer container;
container.add(10);
container.add(20);
container.display();
return 0;
}
In this code, a custom class `MyContainer` encapsulates a vector, which can add and display numbers. This demonstrates how STL containers can be embedded within user-defined classes, enhancing modularity and code organization.
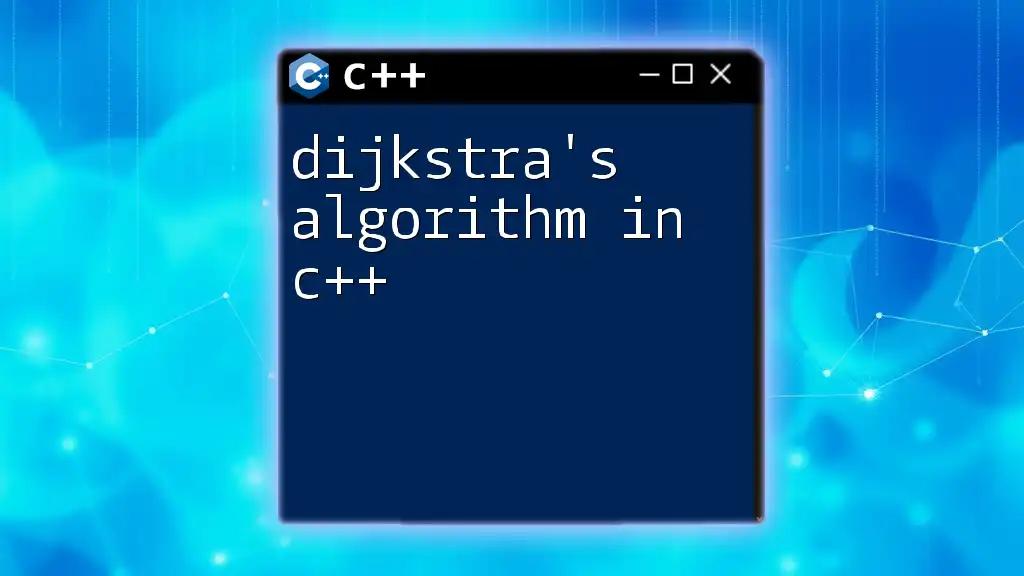
Conclusion
In conclusion, data structures and algorithms with the C++ STL can significantly enhance productivity and reduce complexity in programming. The STL offers a rich set of features through its collection of containers, algorithms, and iterators that not only promote code reuse but also lead to high-performance applications. Programmers are encouraged to dive deeper into STL, experiment with various data structures, and push the boundaries of what they can achieve using this powerful library. Embracing STL is a step toward writing clean, efficient, and maintainable code.