C++ futures and promises are a part of the C++ standard library that allow for asynchronous programming by enabling a thread to produce a value (promise) and another thread to retrieve that value (future) once it's ready.
Here's a simple code snippet demonstrating their usage:
#include <iostream>
#include <future>
#include <thread>
int computeSquare(int x) {
return x * x;
}
int main() {
std::promise<int> prom;
std::future<int> fut = prom.get_future();
std::thread t([&prom]() {
prom.set_value(computeSquare(5));
});
std::cout << "Result: " << fut.get() << std::endl;
t.join();
return 0;
}
Understanding C++ Futures and Promises
What is a Promise?
A promise in C++ serves as a mechanism for managing asynchronous operations. It acts as a placeholder for a value that will eventually be available after some computation. When a promise is created, it is initially "empty," but it can be filled with a value later or marked with an error.
Promises are essential in cases where you wish to decouple the task of results computation from the task that consumes the results. By setting up promises properly, you can manage complex asynchronous workflows efficiently.
Creating and Using a Promise
To create a promise, use the `std::promise` class from the `<future>` header. Here’s an example:
#include <iostream>
#include <thread>
#include <future>
void exampleFunction(std::promise<int>& promiseObj) {
// Simulate long computation
std::this_thread::sleep_for(std::chrono::seconds(1));
promiseObj.set_value(42); // Set the result in promise
}
int main() {
std::promise<int> myPromise;
std::thread myThread(exampleFunction, std::ref(myPromise));
myThread.join();
return 0;
}
In this snippet, the promise is created, and a worker thread is spawned to fulfill the promise. The `set_value()` function is called once the computation is completed (in this case, it simply returns the integer 42). Using `std::ref()` ensures that the promise is passed by reference, preventing any undesired copying.
Error Handling with Promises
Another crucial function is `set_exception()`, which allows you to indicate an error condition in the promise. If the computation fails, you can capture that failure through an exception.
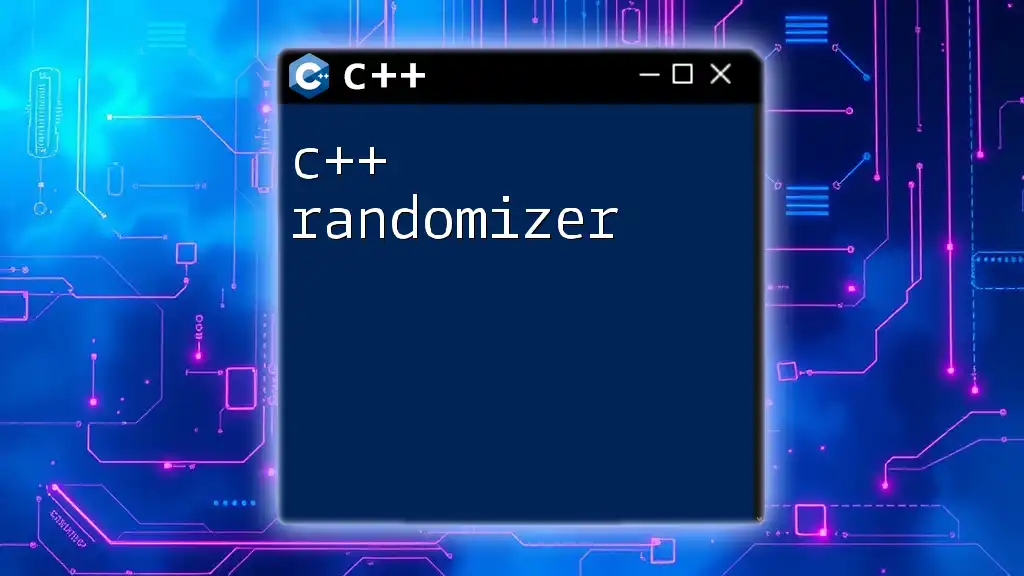
Understanding Futures in C++
What is a Future?
A future in C++ is an object tied to a promise that allows you to retrieve the value of an asynchronous result. Simply put, it can be thought of as a ticket for the eventual computation that provides a means to track its execution state.
Creating and Using a Future
To use a future, one commonly promotes a task into asynchronous operation using `std::async`. Here’s how it works:
#include <iostream>
#include <thread>
#include <future>
int calculate() {
std::this_thread::sleep_for(std::chrono::seconds(2));
return 12;
}
int main() {
std::future<int> myFuture = std::async(std::launch::async, calculate);
std::cout << "Calculating..." << std::endl;
std::cout << "Result: " << myFuture.get() << std::endl; // Blocks until result is ready
return 0;
}
In this code, `std::async` launches the `calculate` function in a new thread. The `myFuture.get()` call blocks the main thread until the future value is ready. If the task fails, exceptions thrown are propagated to where `get()` is called.
Handling Exceptions with Futures
Using futures allows for graceful error management in asynchronous functions. Wrapping your future retrieval in a try-catch block can ensure that any exceptions occurring within your asynchronous task can be handled effectively.
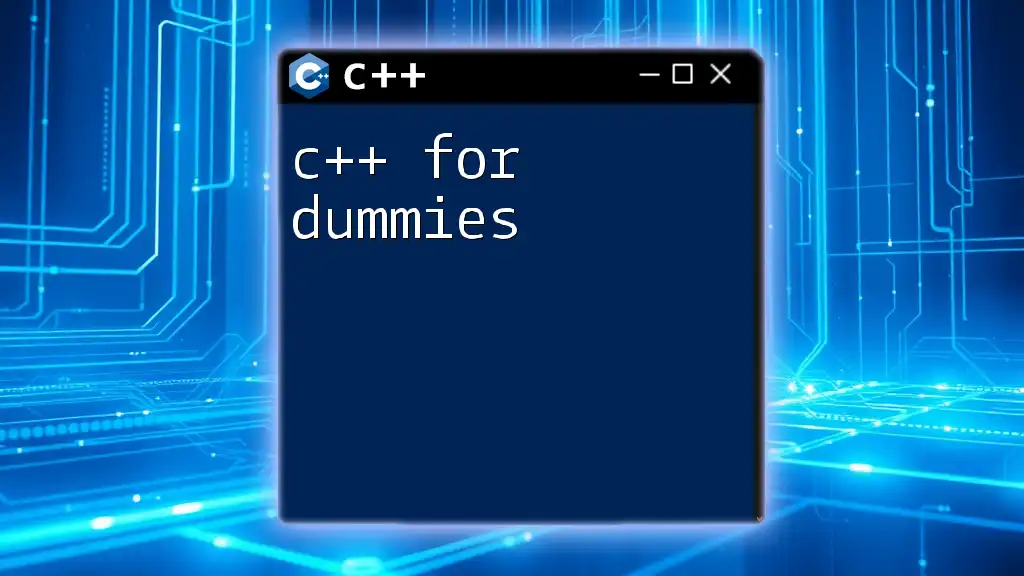
Relationship Between Futures and Promises
How Promises and Futures Work Together
The promise and future provide an elegant means of managing concurrency. When you create a promise, it’s linked to a future which retrieves the result.
This relationship is essential when separating task execution from result consumption. For instance, the promise sets the computed value, and the future retrieves this value at a later stage. It's a handy system for ensuring that values can be computed in the background while the program continues executing other tasks.
Example: Using Promise with Future
A practical example illustrates how futures and promises work together. Consider this code:
#include <iostream>
#include <thread>
#include <future>
void asyncTask(std::promise<int> promiseObj) {
std::this_thread::sleep_for(std::chrono::seconds(1));
promiseObj.set_value(10); // Setting the value
}
int main() {
std::promise<int> myPromise;
std::future<int> myFuture = myPromise.get_future(); // Getting the future
std::thread t(asyncTask, std::move(myPromise)); // Passing promise to thread
std::cout << "The answer is: " << myFuture.get() << std::endl; // Retrieves the value
t.join();
return 0;
}
In this example, a new thread is created to perform a task. The promise is passed to the thread, where the value is computed, and it can then be retrieved through the future. The key point is to observe how the `myFuture.get()` method retrieves the computed value only when it is ready, blocking the call until the promise fulfills.
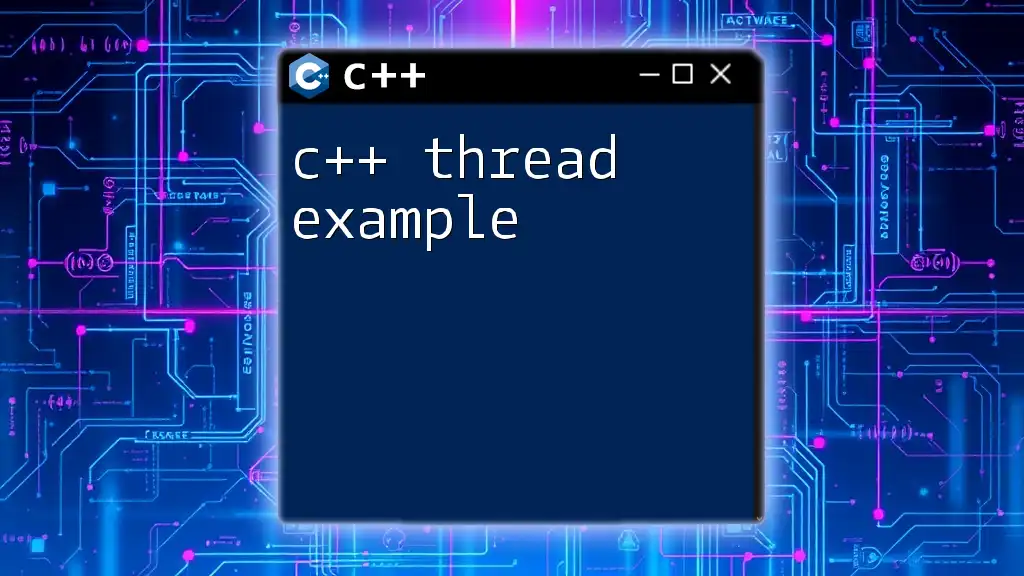
Best Practices Utilizing Futures and Promises
Managing Resource Efficiency
When using C++ futures and promises, you should be mindful of resource management. Avoid blocking operations when possible by leveraging asynchronous models properly. Using `std::async` allows you to invoke background tasks with better resource management.
Exception Handling in Asynchronous Tasks
An effective approach in handling exceptions is to include try-catch blocks around the call to `get()`. This strategy safeguards against unexpected issues during asynchronous operations and allows you to manage control flow even when things go wrong.
Real-World Applications
C++ futures and promises serve numerous use cases, particularly in high-performance applications such as web servers, where handling requests concurrently can significantly enhance performance. They are also valuable in data processing tasks that exploit parallel algorithms to improve computational speed and efficiency.
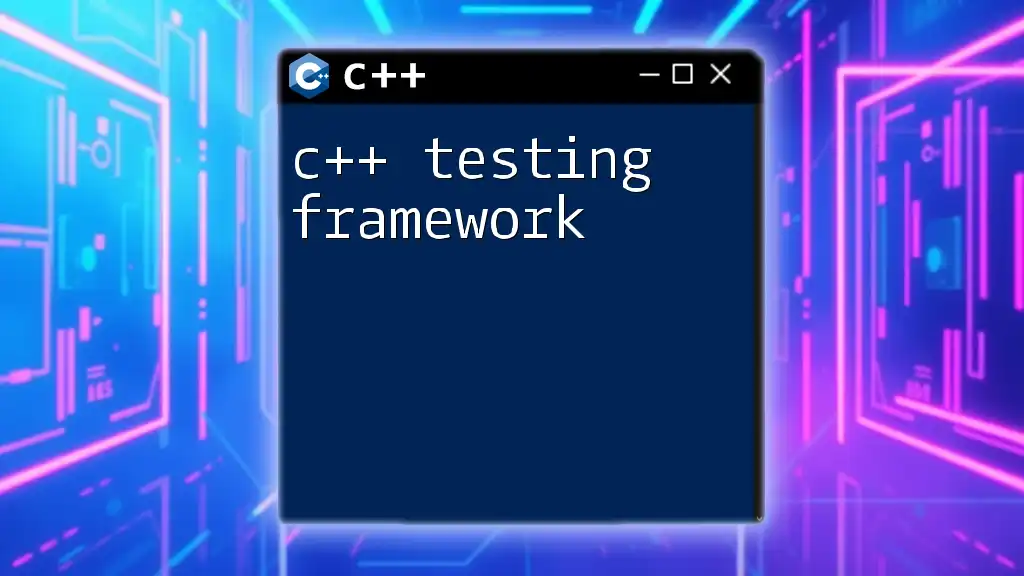
Comparing C++ Futures and Promises with Other Languages
Futures and Promises in Other Languages
When juxtaposing C++ futures and promises with similar constructs in other programming languages, some common differences arise. For example, JavaScript promises work on a fundamentally similar principle but use the event loop mechanism of JavaScript for asynchronous task handling. In contrast, C++ offers a more flexible model utilizing threads and asynchronous function calls.
How C++ Implementation Benefits Performance
C++ futures and promises are designed for high-performance applications, minimizing the need for mutex locks and thread handling overhead. This efficiency is crucial in systems where performance is paramount, allowing fine-grained control over thread execution and resource management.
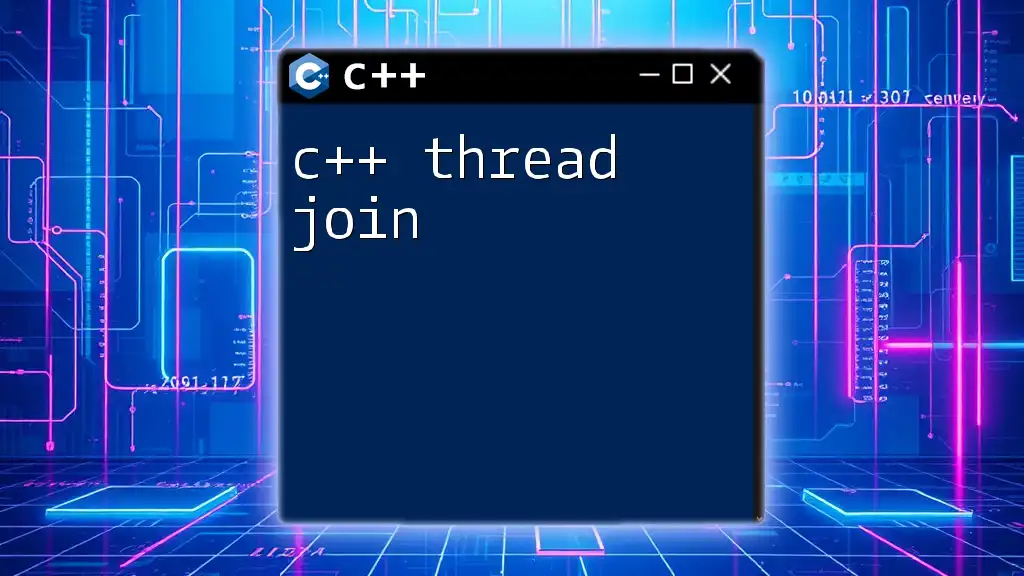
Conclusion
C++ futures and promises are powerful constructs enabling logical and efficient asynchronous programming. They help manage concurrency in a sophisticated manner, separating the concerns of computation and result retrieval. By understanding and mastering these elements, you open avenues for developing high-performance applications that can operate smoothly and efficiently, making asynchronous programming accessible and effective.