In C++, you can use the `<chrono>` library to work with date and time, allowing you to measure time intervals efficiently.
Here’s a simple code snippet that demonstrates getting the current date and time:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t currentTime = std::chrono::system_clock::to_time_t(now);
std::cout << "Current date and time: " << std::ctime(¤tTime);
return 0;
}
Understanding Date and Time in C++
C++ provides various functionalities for handling date and time, an essential aspect of many software applications. Whether you're logging events, scheduling tasks, or processing timestamps, understanding how to manage date and time effectively in C++ is vital. The C++ standard encourages the use of the `<chrono>` library, which offers robust facilities for working with time-related data.

Getting Started with the `<chrono>` Library
Overview of `<chrono>`
The `<chrono>` library in C++ is a powerful tool designed for handling time durations, time points, and clocks. It abstracts time manipulation to make it easier and ensures better precision and type safety than manually handling time. The chrono library empowers developers to create applications that can seamlessly manage and operate around various dates and timestamps.
Basic Components of `<chrono>`
-
Time Points: A time point represents a specific point in time, expressed as a duration since a clock's epoch. You can create a time point and manipulate it to accommodate your needs.
Here's an example of how to create a time point representing the current time:
#include <iostream> #include <chrono> int main() { auto now = std::chrono::system_clock::now(); std::time_t now_c = std::chrono::system_clock::to_time_t(now); std::cout << "Current date and time: " << std::ctime(&now_c); return 0; }
-
Duration: A duration refers to the length of time between two points. With chrono, you can directly manipulate durations using arithmetic operations.
This snippet calculates the duration between two dates:
#include <iostream> #include <chrono> int main() { auto start = std::chrono::system_clock::now(); // Simulate work with a sleep duration std::this_thread::sleep_for(std::chrono::seconds(2)); auto end = std::chrono::system_clock::now(); std::chrono::duration<double> elapsed_seconds = end - start; std::cout << "Elapsed time: " << elapsed_seconds.count() << " seconds\n"; return 0; }
-
Clock Types: C++ provides various types of clocks. The system_clock tracks real time, the steady_clock is not affected by system clock adjustments, and the high_resolution_clock offers the highest available precision. Understanding these distinctions is crucial when you need precise timing in your applications.
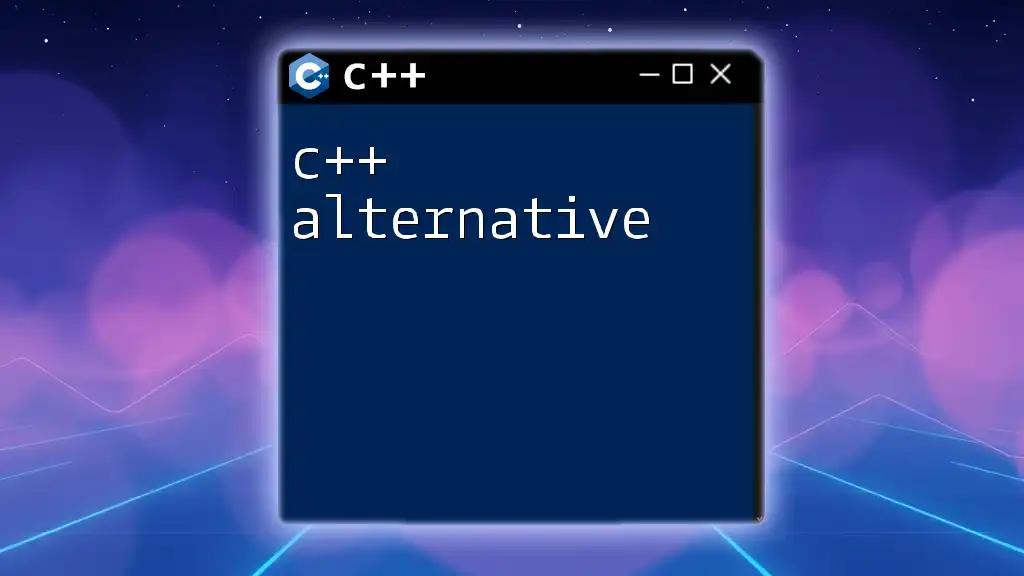
Working with C++ Date and Time
Retrieve Current Date and Time
Fetching the current date and time is essential for many applications. The `std::chrono::system_clock` is the go-to clock for retrieving the current system date and time. Here's a quick example:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
std::chrono::system_clock::time_point now = std::chrono::system_clock::now();
std::time_t current_time = std::chrono::system_clock::to_time_t(now);
std::cout << "Current date and time: " << std::ctime(¤t_time);
return 0;
}
Formatting Date and Time
Formatting date and time is crucial for presenting data in a user-friendly manner. The `std::put_time` function from the `<iomanip>` library allows you to format date and time effectively.
For example:
#include <iostream>
#include <chrono>
#include <iomanip>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_c = std::chrono::system_clock::to_time_t(now);
std::tm *local_tm = std::localtime(&now_c);
std::cout << "Formatted date and time: "
<< std::put_time(local_tm, "%Y-%m-%d %H:%M:%S") << '\n';
return 0;
}
In this snippet, the format used (`"%Y-%m-%d %H:%M:%S"`) breaks down into year, month, day, hours, minutes, and seconds.
Creating Custom Date and Time
Sometimes, you might need custom date functionality in your applications. This can be achieved by designing a simple date class that supports basic operations.
Here’s a simplistic representation of a custom `Date` class:
#include <iostream>
class Date {
public:
int year, month, day;
Date(int y, int m, int d) : year(y), month(m), day(d) {}
void display() const {
std::cout << year << "-" << month << "-" << day << "\n";
}
};
int main() {
Date my_date(2023, 10, 1);
my_date.display(); // Output: 2023-10-01
return 0;
}
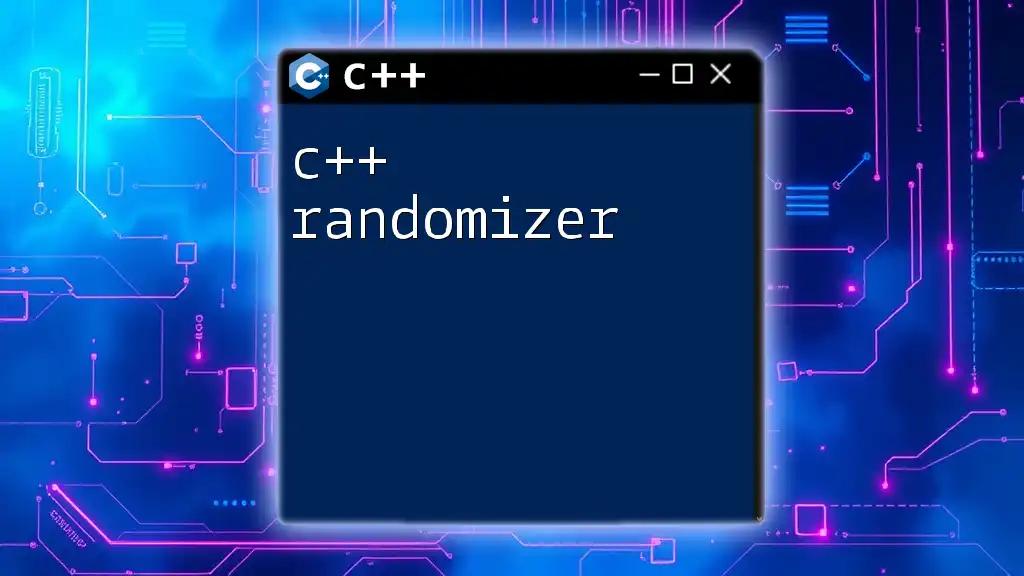
Common Operations with Date and Time in C++
Date Arithmetic
Performing arithmetic operations on dates is a common requirement. You can easily add or subtract durations from existing dates. For instance:
#include <iostream>
#include <chrono>
int main() {
auto today = std::chrono::system_clock::now();
auto tomorrow = today + std::chrono::hours(24);
std::time_t tomorrow_c = std::chrono::system_clock::to_time_t(tomorrow);
std::cout << "Tomorrow's date and time: " << std::ctime(&tomorrow_c);
return 0;
}
In this example, we add 24 hours to the current date, effectively calculating tomorrow's date.
Comparing Dates
The ability to compare dates is also vital. Using comparison operators allows for the comparison of two time points, making it easy to determine which is earlier, later, or if they are identical.
Here’s an example:
#include <iostream>
#include <chrono>
int main() {
auto now = std::chrono::system_clock::now();
auto future_time = now + std::chrono::hours(1);
if (future_time > now) {
std::cout << "Future time is greater than now.\n";
}
return 0;
}
Date & Time Intervals
Determining the duration between two dates can be accomplished with ease using chrono. Here's an example of calculating the number of days between two dates:
#include <iostream>
#include <chrono>
int main() {
auto start_date = std::chrono::system_clock::now();
std::this_thread::sleep_for(std::chrono::hours(48)); // Simulating time passage
auto end_date = std::chrono::system_clock::now();
std::chrono::duration<double, std::ratio<86400>> days_between = end_date - start_date;
std::cout << "Days between: " << days_between.count() << " days.\n";
return 0;
}
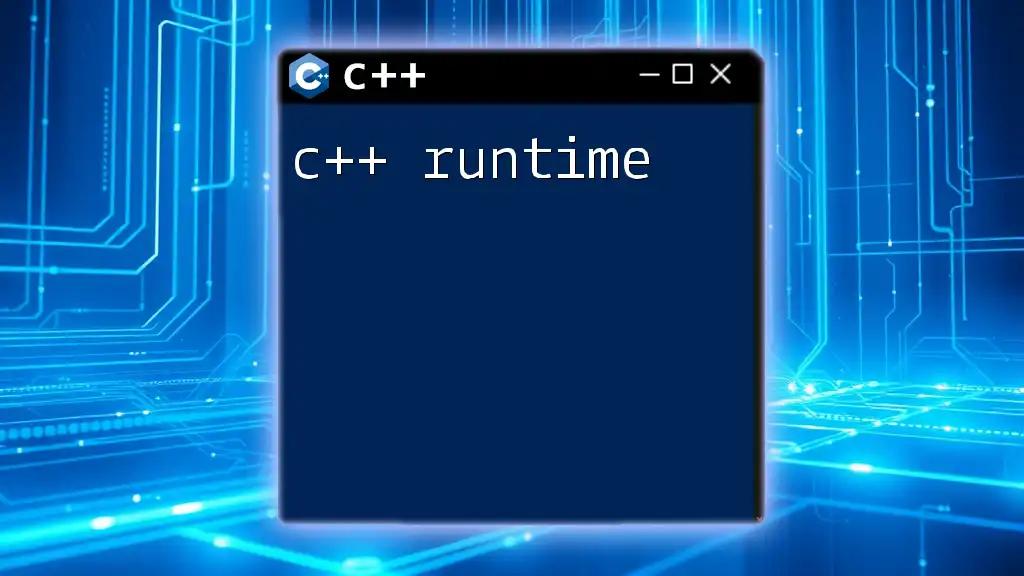
Best Practices for Date and Time Handling in C++
Avoiding Common Pitfalls
When working with C++ date and time, it is crucial to understand the difference between local time and UTC time, especially for applications dealing with users across different time zones.
Always use UTC time for back-end systems to ensure consistency and translate to local time only at the point of user interaction.
Testing and Validating Dates
Date validation is a necessary step to ensure inputs are correct. By implementing checks to confirm that a date is legitimate (e.g., ensuring February 30th is not accepted), you can avoid runtime errors.
Here’s a simple method for validating dates:
bool is_valid_date(int day, int month, int year) {
if (month < 1 || month > 12) return false;
if (day < 1 || day > 31) return false;
// Handle month-end days
if (month == 2) {
bool leap_year = (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
return (day <= (leap_year ? 29 : 28));
}
if (month == 4 || month == 6 || month == 9 || month == 11) {
return (day <= 30);
}
return true;
}
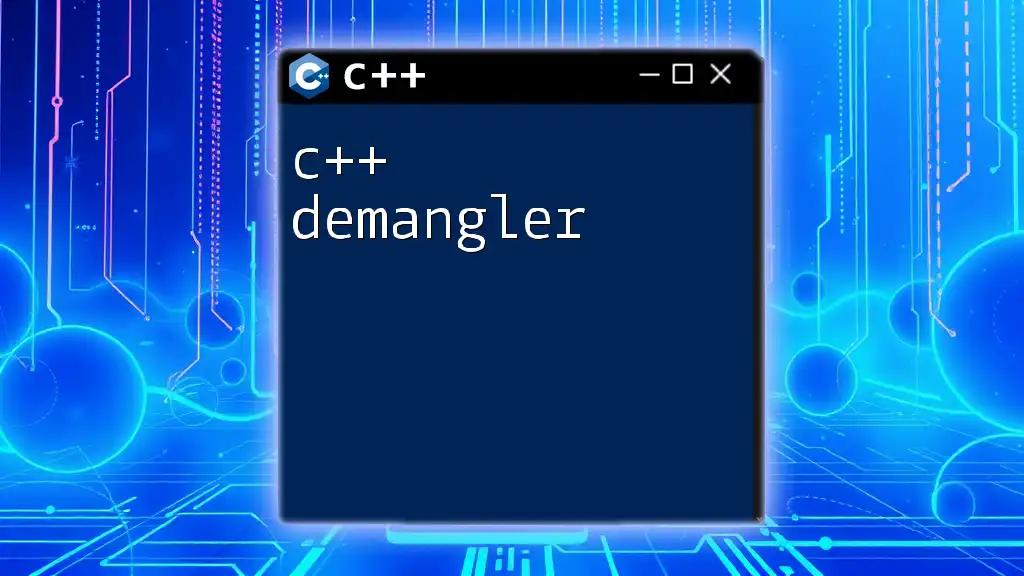
Leveraging Third-Party Libraries for Advanced Date and Time
While the standard library provides robust capabilities, third-party libraries often offer enhanced functionalities. Libraries like Boost.Date_Time or Howard Hinnant’s date library are great alternatives for more complex date and time manipulations. They provide additional features like time zone support and more comprehensive date calculations, which can be beneficial for developing applications that require robust date handling.
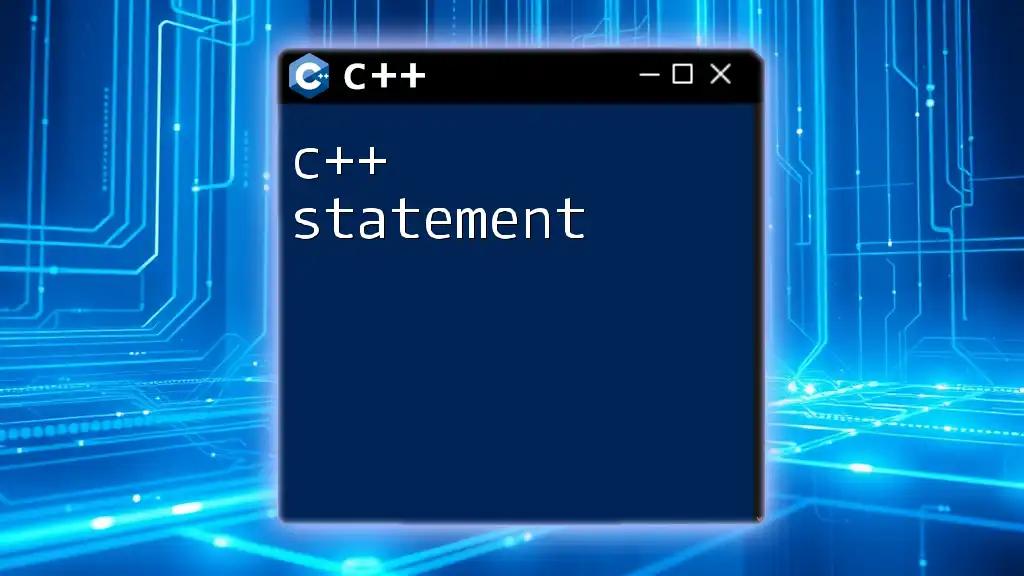
Conclusion
Mastering C++ date and time functionality is essential for developing efficient and accurate applications. By leveraging the capabilities of the `<chrono>` library and understanding the principles of date and time management, programmers can ensure their applications operate smoothly across various scenarios. Practice with the provided code examples and explore how date and time handling can be incorporated into your projects for more effective programming.
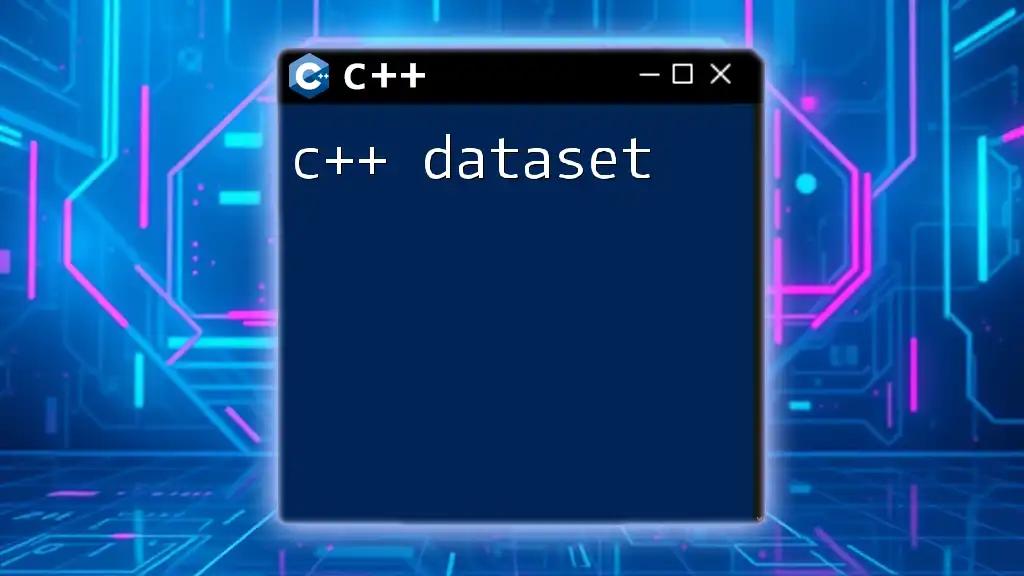
Additional Resources
For further learning and better mastery of C++ date and time, check the official documentation, online tutorials, and forums that specialize in C++ programming and time management techniques. Embrace the opportunity to experiment with these concepts through coding exercises to solidify your knowledge.

Call to Action
If you found this guide helpful, talk to us! Share your thoughts, experiences, or ask questions to improve your journey with C++ date and time handling. Subscribe to our content for more insightful programming tips and resources!