In C++, stacks and queues are abstract data types that follow specific orders for adding and removing elements, with stacks using Last-In-First-Out (LIFO) and queues using First-In-First-Out (FIFO) principles.
Here's a simple example demonstrating both:
#include <iostream>
#include <stack>
#include <queue>
int main() {
// Stack example
std::stack<int> s;
s.push(1);
s.push(2);
std::cout << "Top of stack: " << s.top() << std::endl; // Outputs 2
s.pop();
// Queue example
std::queue<int> q;
q.push(1);
q.push(2);
std::cout << "Front of queue: " << q.front() << std::endl; // Outputs 1
q.pop();
return 0;
}
What Are Stacks and Queues?
C++ stacks and queues are fundamental data structures essential for efficient data handling. A stack operates on the principle of Last In, First Out (LIFO), where the most recently added element is the first one to be removed. Conversely, a queue is based on First In, First Out (FIFO), meaning the earliest added element will be removed first.
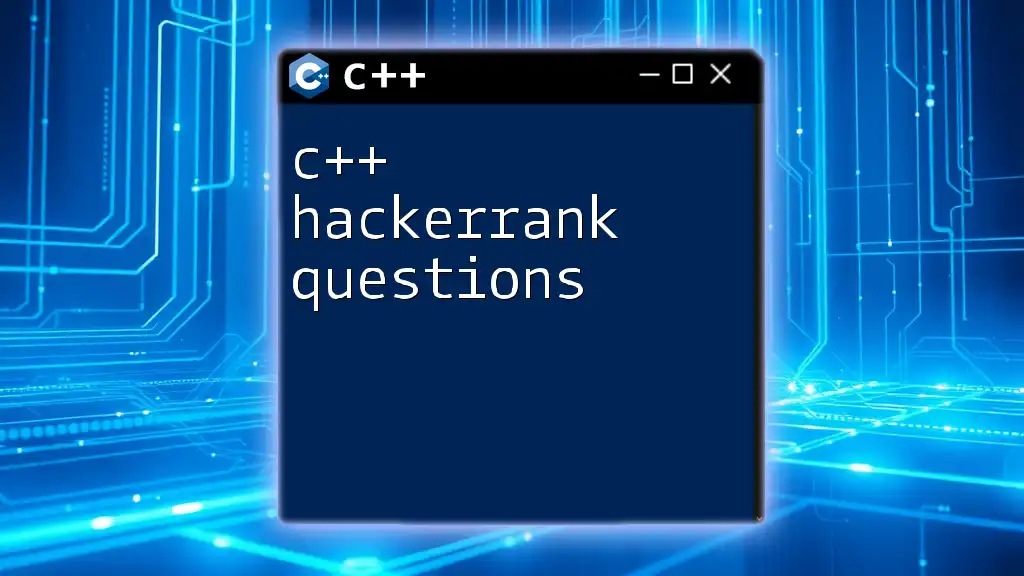
Why Use Stacks and Queues in C++?
The use of stacks and queues in programming is not only practical but also crucial for certain algorithm designs. Stacks are widely employed in scenarios such as function call management, where tracking the execution context is vital. Queues, on the other hand, are integral to managing tasks in scheduling systems, enabling orderly processing of events or data.
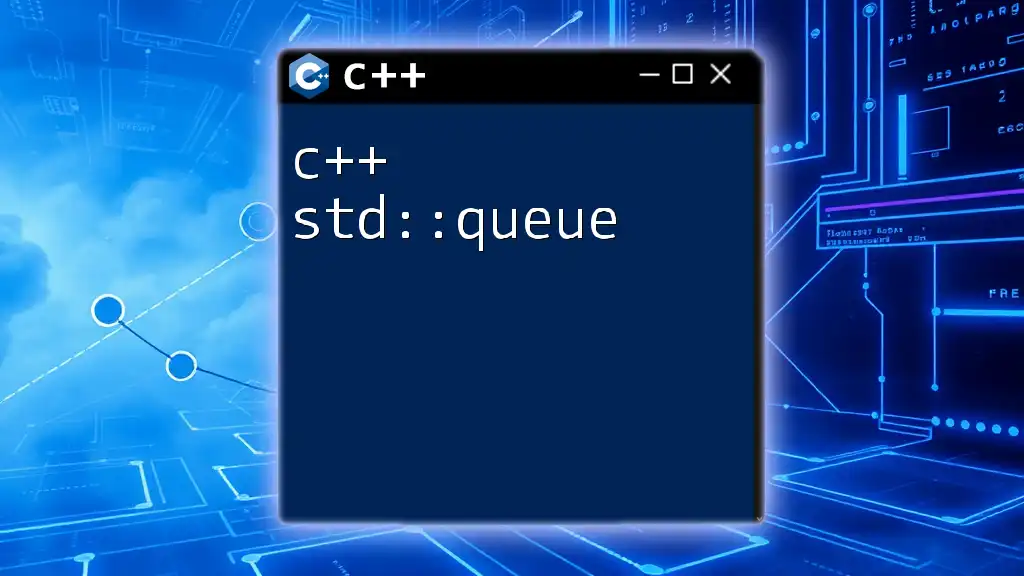
Understanding Stacks in C++
Definition of a Stack
A stack can be defined as a collection of elements with a restricted access policy. With its LIFO structure, any interaction with the stack (like adding or removing elements) occurs at one end, often referred to as the "top" of the stack.
Basic Operations of Stacks
Push Operation
The push operation allows you to add an element to the top of the stack. This operation is crucial as it builds the stack layer by layer, with each new element being placed above the previous one.
Code Snippet: Adding Elements to a Stack
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
myStack.push(1);
myStack.push(2);
myStack.push(3);
return 0;
}
Pop Operation
The pop operation removes the top element from the stack. It's essential to ensure that the stack is not empty before attempting to pop an element to prevent runtime errors.
Code Snippet: Removing the Top Element
myStack.pop(); // removes the top element, which is 3
Top Operation
The top operation allows you to view the element currently on the top of the stack without removing it. This operation is particularly useful when you need to access the most recently added element without modifying the stack's current state.
Code Snippet: Accessing the Top Element
int topElement = myStack.top(); // retrieves the top element, which is 3
Implementing Stacks in C++
Using Standard Template Library (STL)
The C++ Standard Template Library (STL) provides a flexible and efficient implementation of stacks through the `std::stack` template. Using STL not only saves time but also leverages optimizations performed internally.
Code Example: Using std::stack
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
myStack.push(10);
std::cout << "Top element: " << myStack.top() << std::endl; // Output: 10
myStack.pop(); // Removes 10
return 0;
}
Custom Stack Implementation
For educational purposes, it can be insightful to implement a basic stack class from scratch. This exercise can enhance your understanding of how stacks operate under the hood.
Code Example: Basic Stack Class
#include <iostream>
#include <vector>
class Stack {
private:
std::vector<int> elements;
public:
void push(int element) {
elements.push_back(element);
}
void pop() {
if (!isEmpty()) {
elements.pop_back();
}
}
int top() {
if (!isEmpty()) {
return elements.back();
}
throw std::out_of_range("Stack is empty");
}
bool isEmpty() {
return elements.empty();
}
};
Use Cases of Stacks in C++
Stacks are indispensable in various real-world applications. They are fundamental to function call management, where they maintain the order of function calls in a program's execution. Stacks are also widely used in undo functionalities of applications, where recent actions need to be retraced in reverse order.
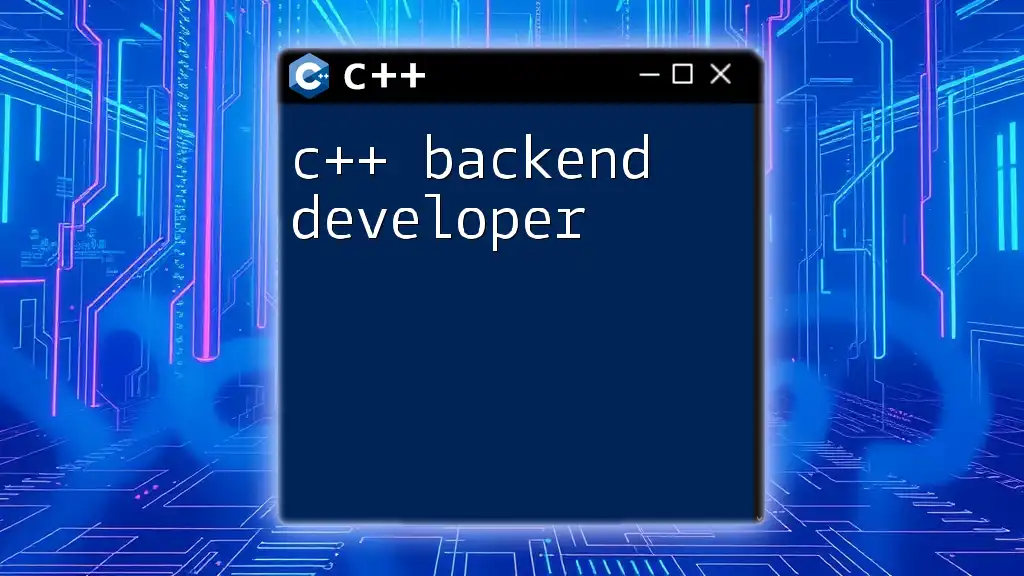
Understanding Queues in C++
Definition of a Queue
A queue is defined as a collection of elements that follows a FIFO method for adding and removing elements. Essentially, items are added at the back of the queue and removed from the front.
Basic Operations of Queues
Enqueue Operation
The enqueue operation allows you to add an element to the back of the queue, maintaining the order of elements as they are processed.
Code Snippet: Adding Elements to a Queue
#include <iostream>
#include <queue>
int main() {
std::queue<int> myQueue;
myQueue.push(1);
myQueue.push(2);
myQueue.push(3);
return 0;
}
Dequeue Operation
The dequeue operation removes the front element from the queue. It’s crucial to ensure that the queue is non-empty before attempting to remove an item.
Code Snippet: Removing the Front Element
myQueue.pop(); // removes the front element, which is 1
Front Operation
The front operation allows you to access the front element of the queue without removing it. This is useful in scenarios where you need to know what the next item to process is without altering the queue.
Code Snippet: Accessing the Front Element
int frontElement = myQueue.front(); // retrieves the front element, which is 1
Implementing Queues in C++
Using Standard Template Library (STL)
C++ STL also provides a robust queue implementation via `std::queue`. This feature allows for straightforward queue operations while benefiting from optimized performance.
Code Example: Using std::queue
#include <iostream>
#include <queue>
int main() {
std::queue<int> myQueue;
myQueue.push(10);
std::cout << "Front element: " << myQueue.front() << std::endl; // Output: 10
myQueue.pop(); // Removes 10
return 0;
}
Custom Queue Implementation
Understanding how to build a queue from scratch can provide deeper insights into its mechanics. This exercise involves creating a simple queue class.
Code Example: Basic Queue Class
#include <iostream>
#include <vector>
class Queue {
private:
std::vector<int> elements;
public:
void enqueue(int element) {
elements.push_back(element);
}
void dequeue() {
if (!isEmpty()) {
elements.erase(elements.begin());
}
}
int front() {
if (!isEmpty()) {
return elements.front();
}
throw std::out_of_range("Queue is empty");
}
bool isEmpty() {
return elements.empty();
}
};
Use Cases of Queues in C++
Queues play a pivotal role in many systems. They are commonly utilized in task scheduling, where processes need to be handled in the order they arrive. Queues are also vital in buffering mechanisms for input/output data processing, managing data flow based on arrival time.
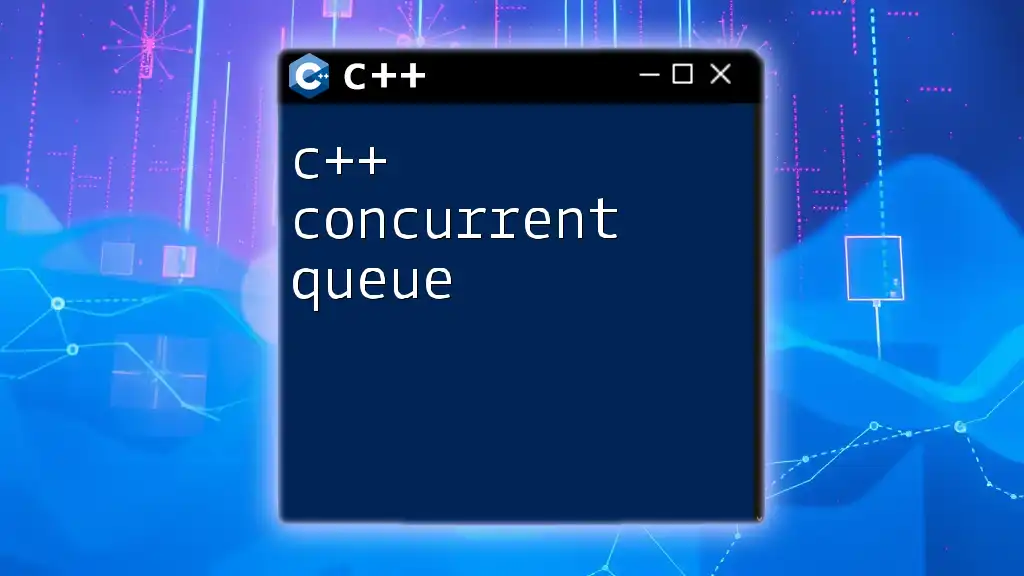
Comparing Stacks and Queues
Key Differences Between Stacks and Queues
The primary difference between stacks and queues lies in their operational principles. Stacks utilize a LIFO approach, where the last element added is the first to be removed. In contrast, queues operate on a FIFO principle, ensuring that the first element added is processed first. Understanding these differences is crucial for choosing the appropriate data structure for specific tasks.
Choosing the Right Data Structure
When deciding between stacks and queues, consider the problem context. If you need to access the most recent information first, stacks are your best bet. On the other hand, if your processing requires maintaining sequential order, queues should be utilized. Real-world scenarios can further illuminate these choices, as seen in function calls (using stacks) versus task management (using queues).
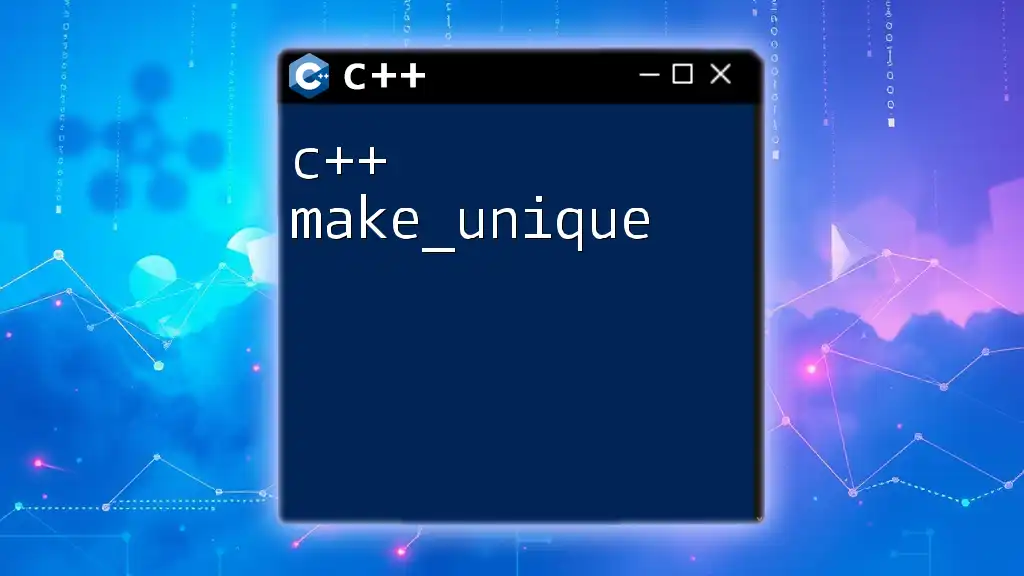
Conclusion
In conclusion, C++ stacks and queues are powerful constructs that every programmer should master. Their unique capabilities allow for effective data manipulation and organization, supporting various applications across different domains. Understanding their operations, implementations, and use cases will enhance your programming toolkit and improve efficiency in your coding tasks.
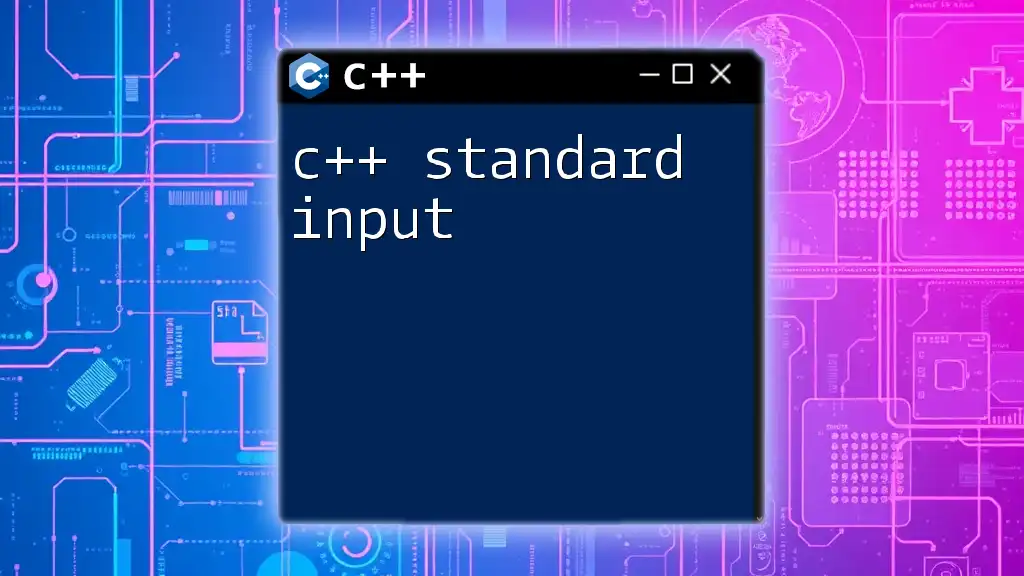
Further Learning Resources
For those eager to deepen their knowledge, several excellent resources are available. Books such as "Data Structures and Algorithms in C++" and online platforms like Coursera and Udacity offer courses specifically focusing on data structures. Participating in community forums, reading official documentation, and experimenting with code are also effective ways to expand your understanding of C++ stacks and queues.
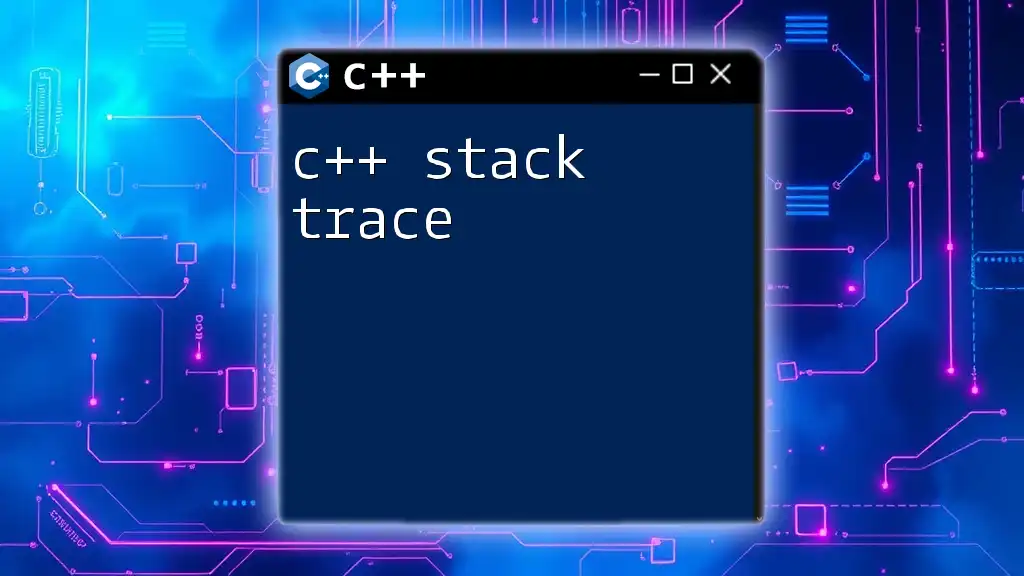
FAQs About C++ Stacks and Queues
What is a stack overflow?
A stack overflow occurs when a stack exceeds its capacity, typically due to excessive pushing of elements without adequate popping.
Can you implement a stack using a queue?
Yes, you can implement a stack using one or more queues by manipulating the enqueue and dequeue operations to reverse the order of elements.
What are the performance implications of using stacks and queues?
Both stacks and queues generally offer O(1) performance for their primary operations (push, pop, enqueue, dequeue), making them efficient choices for their specific use cases.