A C++ concurrent queue is a thread-safe data structure that allows multiple threads to enqueue and dequeue items safely without the need for external locking mechanisms.
Here's a simple code snippet demonstrating a concurrent queue using `std::queue` and `std::mutex` in C++:
#include <queue>
#include <mutex>
#include <condition_variable>
template <typename T>
class ConcurrentQueue {
public:
void enqueue(T value) {
std::lock_guard<std::mutex> lock(mutex_);
queue_.push(value);
cond_var_.notify_one();
}
T dequeue() {
std::unique_lock<std::mutex> lock(mutex_);
cond_var_.wait(lock, [this] { return !queue_.empty(); });
T value = queue_.front();
queue_.pop();
return value;
}
private:
std::queue<T> queue_;
std::mutex mutex_;
std::condition_variable cond_var_;
};
Understanding Concurrent Queues
What is a Concurrent Queue?
A concurrent queue is a specialized data structure designed to support multiple threads accessing it simultaneously without causing race conditions. In contrast to standard queues, where one thread can disrupt another's operation, concurrent queues ensure thread safety through synchronization mechanisms.
Use Cases for Concurrent Queues
Concurrent queues are vital in various scenarios:
- Multithreading Applications: When multiple threads need to share data efficiently, concurrent queues enable safe data exchange.
- Producer-Consumer Problem: A classic concurrency issue where one or more producers generate data and one or more consumers process it. Concurrent queues effectively manage the data flow between these threads.
- Load Balancing and Task Distribution: In server applications where tasks must be distributed amongst workers, concurrent queues play a crucial role in coordinating and managing those tasks.
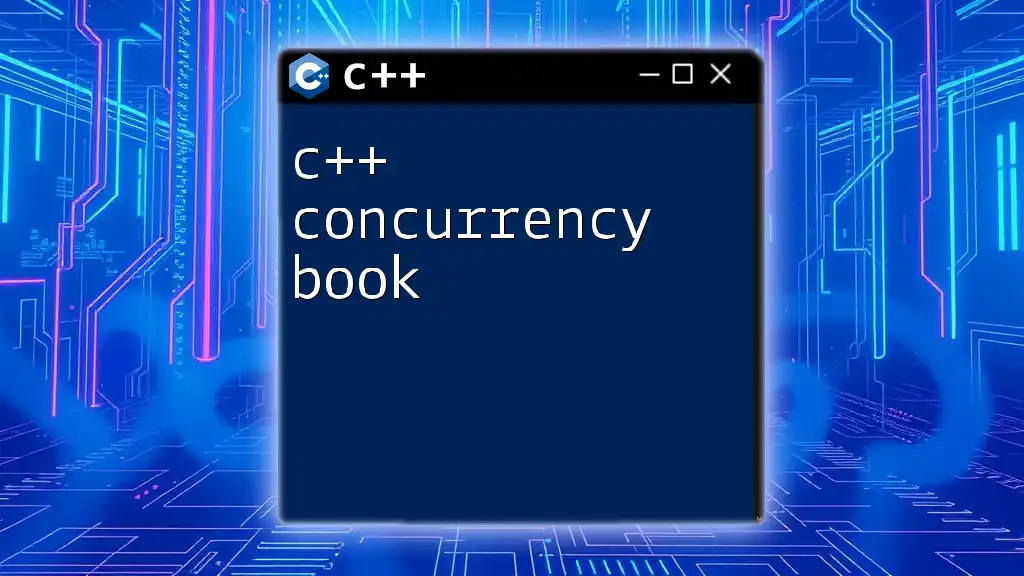
Basic Concepts in Concurrency
Multithreading in C++
Multithreading allows a program to execute multiple sequences of instructions independently. In C++, multithreading can be achieved using the `<thread>` library. However, this introduces complexities such as thread safety and synchronization. Developers must ensure that shared resources are protected to prevent issues like race conditions, where two threads attempt to change the same data simultaneously, leading to unpredictable outcomes.
Mutexes and Locks
A mutex (mutual exclusion) is a synchronization primitive that protects shared resources by allowing only one thread to access the resource at a time. There are various types of locks:
- std::mutex: A basic mutex type that offers exclusive access to a resource.
- std::unique_lock: Provides a mechanism to lock and unlock mutexes, allowing more complex ownership semantics.
- std::shared_lock: This allows multiple threads to acquire shared access without exclusive ownership, beneficial in read-heavy scenarios.
Understanding how to use these tools is essential to avoid common pitfalls in multithreading, such as deadlocks, which occur when two or more threads are waiting indefinitely for resources held by each other.
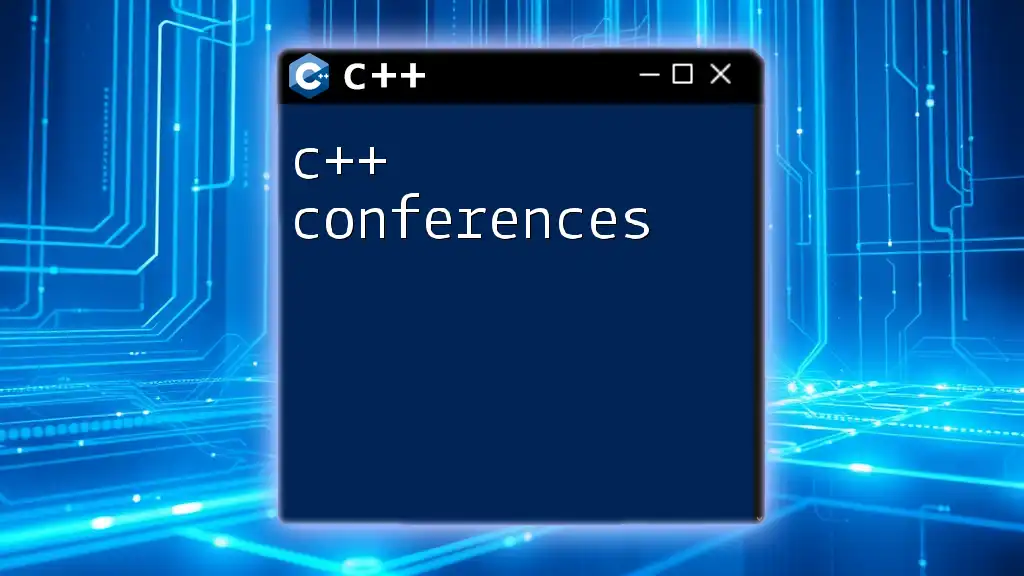
Implementing a Concurrent Queue in C++
Designing a Concurrent Queue Class
To create a robust concurrent queue, you must consider the following key components:
- Data Structure: Choosing the right underlying data structure (e.g., `std::queue`, `std::deque`, or even a custom implementation) is crucial for performance and functionality.
- Synchronization Primitives: Implementing mutexes and condition variables to ensure safe access.
Basic Operations of a Concurrent Queue
Implementing basic operations such as enqueue and dequeue is at the heart of a concurrent queue.
- Enqueue Operation
template <typename T>
class ConcurrentQueue {
private:
std::queue<T> queue_;
std::mutex mutex_;
std::condition_variable condition_;
public:
void enqueue(T value) {
std::lock_guard<std::mutex> lock(mutex_);
queue_.push(value);
condition_.notify_one(); // Notify waiting threads
}
};
In the enqueue method, we first lock the mutex to ensure thread safety. After pushing the new value onto the queue, we call `notify_one()`, allowing one waiting thread (if any) to proceed.
- Dequeue Operation
template <typename T>
T dequeue() {
std::unique_lock<std::mutex> lock(mutex_);
condition_.wait(lock, [this] { return !queue_.empty(); });
T value = queue_.front();
queue_.pop();
return value;
}
When a thread calls dequeue, it secures the mutex and waits on the condition variable until the queue has elements to process. Once an item is available, the thread retrieves and removes it from the queue.
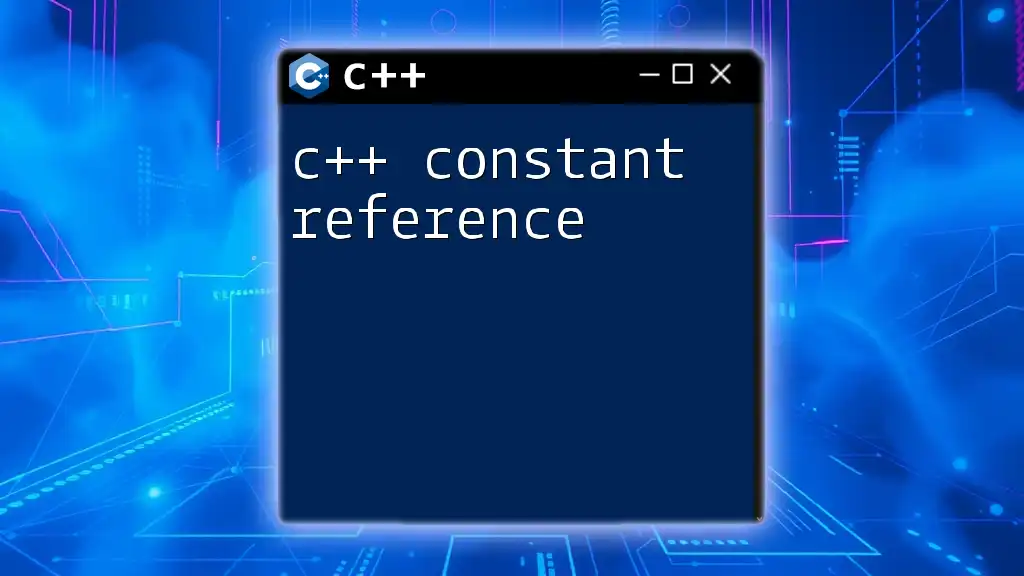
Advanced Features of Concurrent Queues
Condition Variables
Condition variables are essential for managing the signaling between threads, especially when one thread needs to wait for a condition to be met (e.g., when the queue is empty or full). They work alongside mutexes to facilitate this communication without busy-waiting, which is resource-intensive.
Fairness and Performance Considerations
In a concurrent queue, it's vital to ensure fairness, meaning that no thread should starve while waiting to access the queue. Prioritizing requests can help achieve balance. Additionally, performance optimization techniques are crucial for high-load scenarios:
- Consider implementations that leverage lock-free programming to minimize contention and enhance throughput.
- Use atomic operations whenever possible to reduce the overhead of locking.
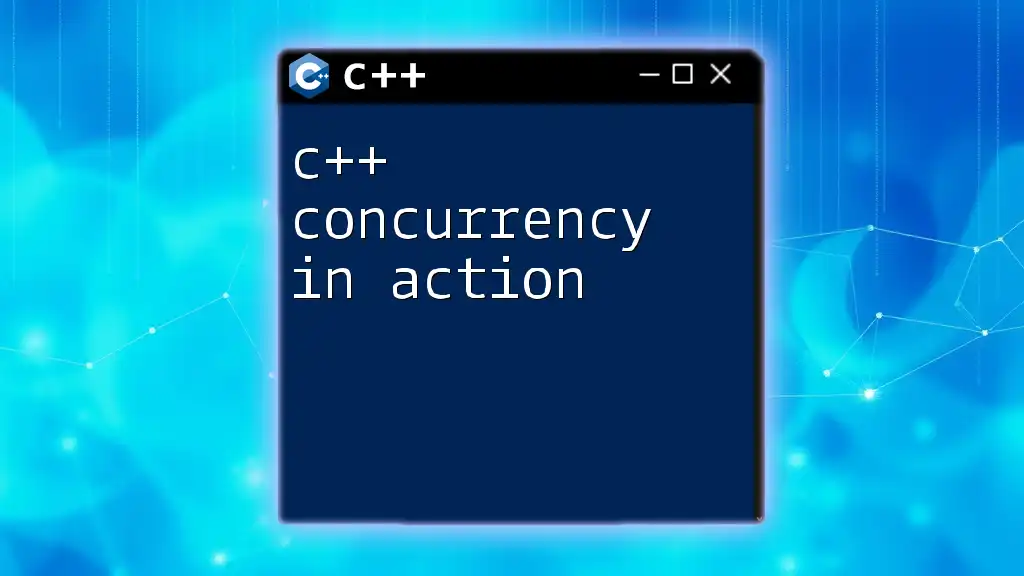
Testing and Debugging Concurrent Queues
Common Issues in Concurrent Programming
Concurrency introduces unique challenges such as race conditions (where the outcome depends on the sequence of thread executions) and deadlocks. Identifying and addressing these issues is critical to ensure reliability. Debugging multithreaded applications can be challenging, but tools such as `Valgrind` and thread analyzers can help.
Unit Testing Concurrent Queues
Testing a concurrent queue is fundamental to validating its behavior under concurrent access. Consider creating unit tests that simulate multiple threads interacting with the queue. This includes checking edge cases like empty queue handling during dequeue operations.
TEST(ConcurrentQueueTest, EnqueueDequeue) {
ConcurrentQueue<int> queue;
std::thread producer([&]() {
for (int i = 0; i < 10; ++i) {
queue.enqueue(i);
}
});
std::thread consumer([&]() {
for (int i = 0; i < 10; ++i) {
int value = queue.dequeue();
// Checks to verify correctness can be added here
}
});
producer.join();
consumer.join();
}
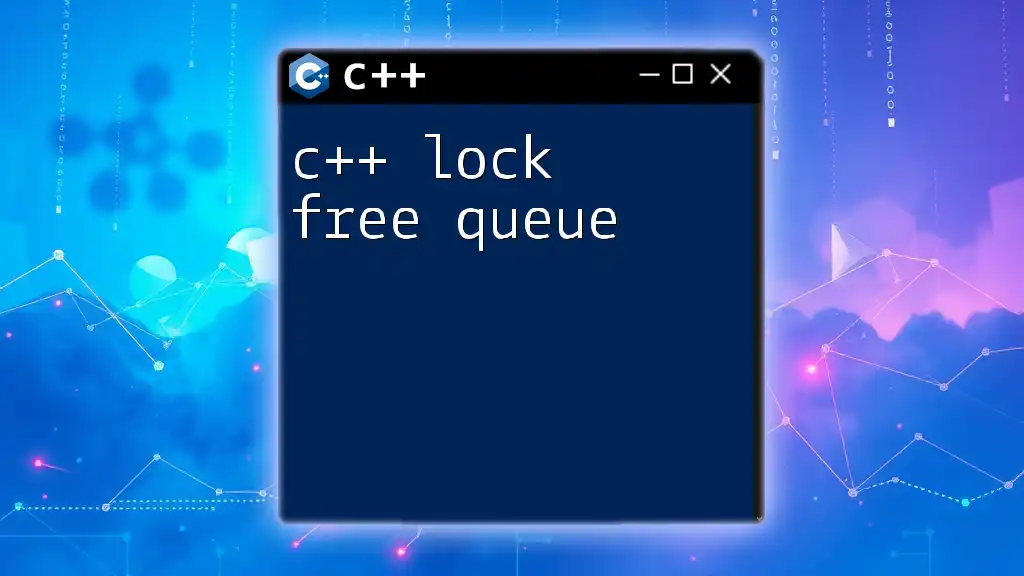
Real-world Applications and Examples
Use Cases of Concurrent Queues
Concurrent queues find their applications in various industries, particularly in areas requiring high performance and responsiveness. For instance, web servers that manage concurrent requests can utilize concurrent queues to handle incoming requests, distributing the workload effectively across multiple threads.
Integrating Concurrent Queues with Other C++ Features
A C++ concurrent queue can be seamlessly integrated with other parts of the C++ standard library. Utilizing `std::async` and `std::future` can enhance the scalability of an application. These features allow threads to execute functions asynchronously, further improving responsiveness and performance.
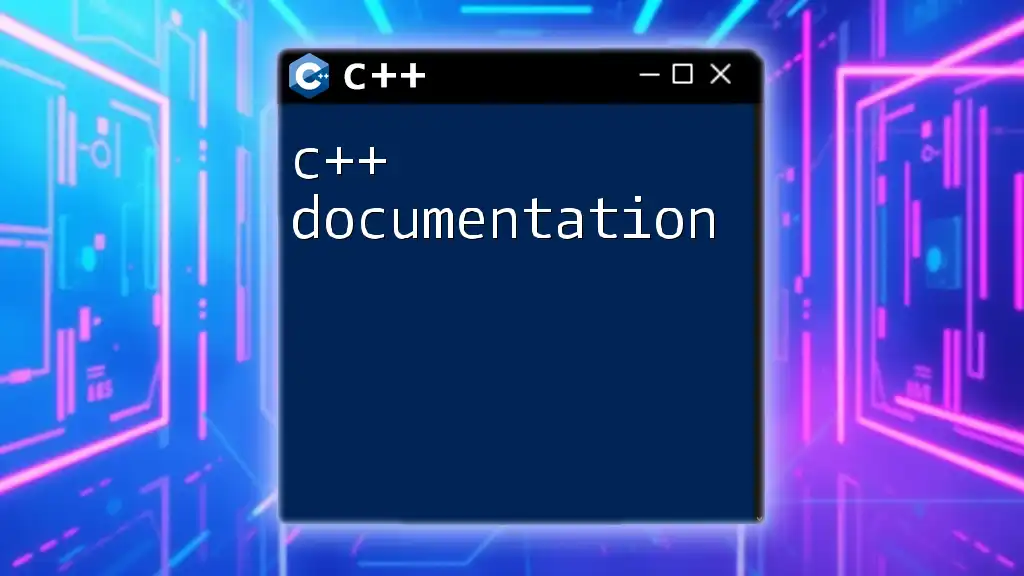
Conclusion
The mastery of C++ concurrent queues equips developers with the tools necessary to write efficient, thread-safe applications. Understanding the underlying principles of concurrency, mutexes, and condition variables will not only enhance your programming skills but also open doors to more advanced concepts.
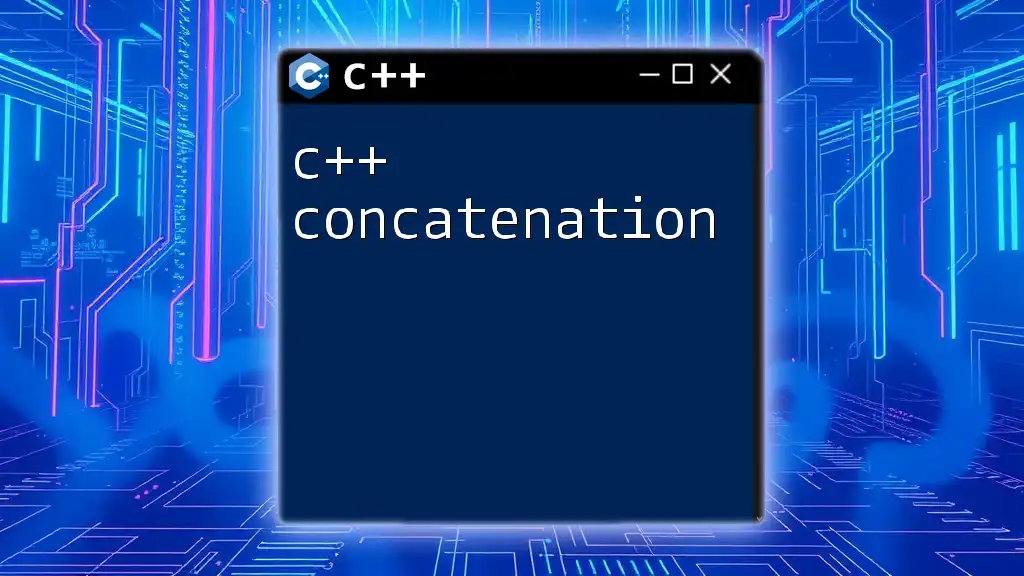
Resources and Further Reading
For those eager to deepen their knowledge further, consider exploring reputable books on concurrency in C++ and engaging with community forums where you can discuss and resolve specific challenges related to concurrent programming.
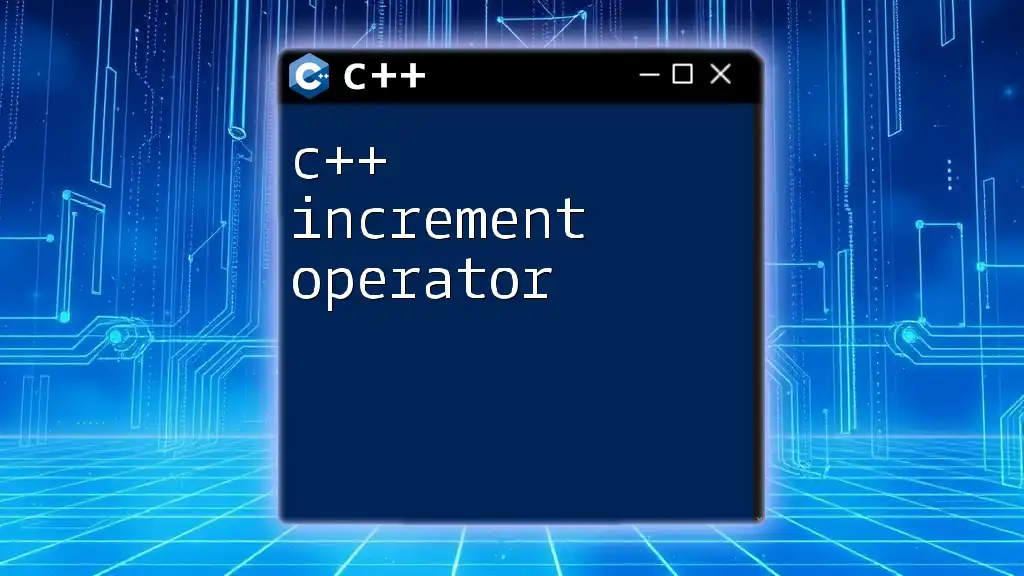
Call to Action
It's time to implement your own concurrent queue! Start coding and experimenting with different features and optimizations. Subscribe to our platform for more C++ content and updates designed to boost your programming skills!