A C++ lock-free queue is a concurrent data structure that allows multiple threads to enqueue and dequeue items without the need for mutex locks, thereby avoiding contention and potential delays.
Here's a simple implementation of a lock-free queue using atomic operations:
#include <atomic>
#include <memory>
template<typename T>
class LockFreeQueue {
private:
struct Node {
T data;
std::shared_ptr<Node> next;
Node(const T& data) : data(data), next(nullptr) {}
};
std::atomic<std::shared_ptr<Node>> head;
std::atomic<std::shared_ptr<Node>> tail;
public:
LockFreeQueue() : head(new Node(T())), tail(head.load()) {}
void enqueue(const T& value) {
auto new_node = std::make_shared<Node>(value);
while (true) {
auto tail_node = tail.load();
auto next_node = tail_node->next;
if (tail_node == tail.load()) {
if (next_node == nullptr) {
if (tail_node->next.compare_exchange_strong(next_node, new_node)) {
tail.compare_exchange_strong(tail_node, new_node);
return;
}
} else {
tail.compare_exchange_strong(tail_node, next_node);
}
}
}
}
std::shared_ptr<T> dequeue() {
while (true) {
auto head_node = head.load();
auto tail_node = tail.load();
auto next_node = head_node->next;
if (head_node == head.load()) {
if (head_node == tail_node) {
if (next_node == nullptr) return nullptr; // Queue is empty
tail.compare_exchange_strong(tail_node, next_node);
} else {
if (next_node == nullptr) continue; // Queue is empty
head.compare_exchange_strong(head_node, next_node);
return std::make_shared<T>(next_node->data);
}
}
}
}
};
This C++ code defines a simple lock-free queue template that utilizes atomic operations for thread-safe enqueue and dequeue operations.
What is a Lock-Free Queue?
A lock-free queue is a data structure designed for concurrent programming that allows multiple threads to operate on it without the need for locks. This approach provides a significant advantage in terms of performance and scalability. While traditional queues require locks to manage access, leading to contention and potential bottlenecks, lock-free queues utilize atomic operations to ensure that threads can operate independently with minimal interference.
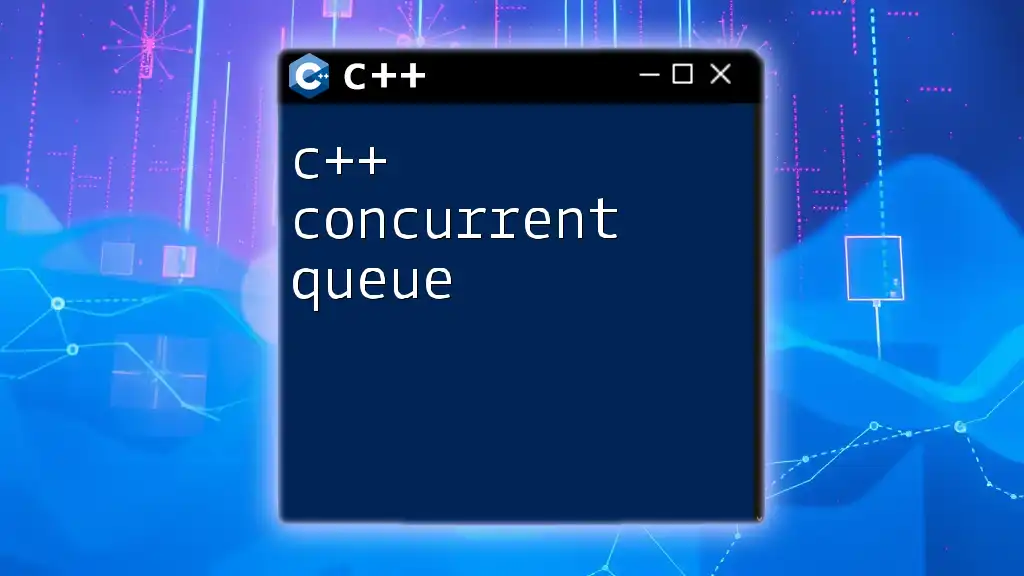
Why Use Lock-Free Queues?
Lock-free queues deliver impressive performance benefits. When multiple threads access a queue simultaneously, traditional locking mechanisms can create contention, significantly reducing throughput. In contrast, lock-free queues enable threads to execute concurrently, which enhances efficiency and responsiveness in multi-threaded applications.
Use Cases for lock-free queues include high-frequency trading, real-time data processing, and scenarios where latency is critical. In these contexts, the ability to handle operations without blocking can be a defining factor in the performance of an application.
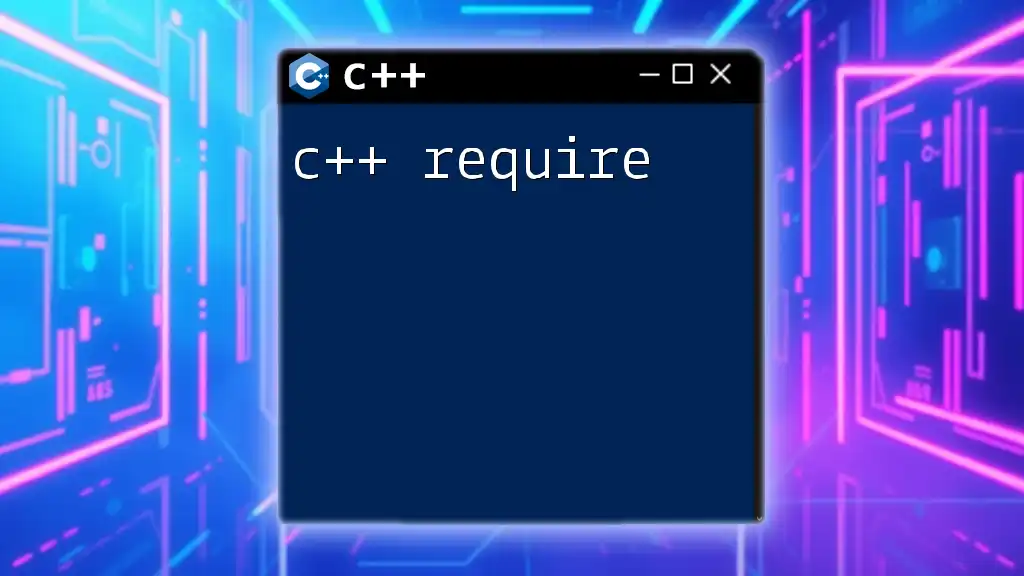
Understanding Lock-Free Data Structures
Basics of Lock-Free Programming
The key to lock-free data structures is a deep understanding of atomic operations and memory models. While some might assume that lock-free programming is inherently more complex, it is grounded in principles that can be mastered with some study and practice.
Common Misconceptions about lock-free programming primarily revolve around the belief that it is always harder to implement than traditional locking techniques. In many cases, once understood, the principles behind atomic operations can lead to cleaner and more maintainable code.
The Concept of Non-Blocking Algorithms
Lock-free queues are a prime example of non-blocking algorithms. These algorithms do not cause threads to wait, preventing the typical pitfalls that can arise from blocking operations.
The terms blocking and non-blocking distinguish how threads interact with shared resources. Non-blocking algorithms allow a thread to continue executing, even if it cannot immediately perform its desired operation, thereby avoiding the typical slowdowns associated with traditional locks.
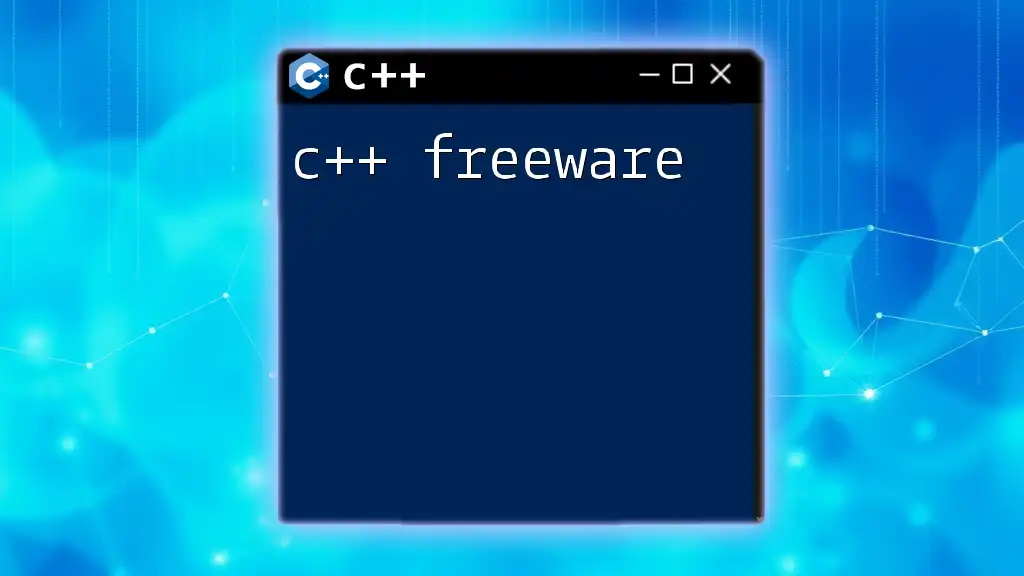
The Mechanics of C++ Lock-Free Queues
Implementing a Simple Lock-Free Queue
A lock-free queue typically relies on an algorithm that uses pointers instead of locks to manage enqueue and dequeue operations. The two primary components are the head and tail pointers, which denote the front and end of the queue.
Here's a basic structure for a lock-free queue:
template <typename T>
class LockFreeQueue {
// Implementation details
};
Key Components of Lock-Free Queues
Node Structure
Each node in a lock-free queue usually contains the data and a pointer to the next node. This structure is critical, as it forms the backbone of the queue's behavior.
struct Node {
T data;
Node* next;
};
Head Pointer Management
Managing the head pointer is crucial for the correct functioning of the queue. When an item is dequeued, the head pointer must be updated to point to the next node in the queue. This operation must be performed atomically to prevent race conditions.
Tail Pointer Management
The tail pointer serves a similar purpose for enqueue operations. It points to the end of the queue and must also be updated atomically. Here's how enqueue operations might look:
void enqueue(T value) {
Node* newNode = new Node{value, nullptr};
Node* oldTail;
// Atomically update the tail pointer
// Ensure oldTail is updated correctly to point to the new node
}
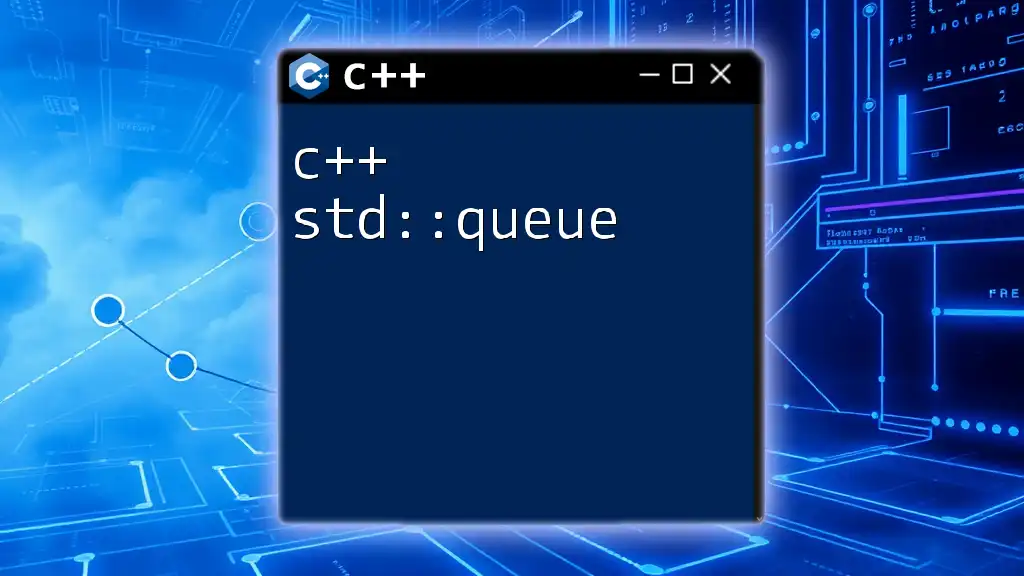
Real-World Implementation of Lock-Free Queue in C++
Complete Code Example
Offering a complete implementation provides a clear understanding of how a lock-free queue may function in practice. Below is an illustrative example of a simple lock-free queue in C++:
#include <atomic>
template <typename T>
class LockFreeQueue {
private:
struct Node {
T data;
Node* next;
};
std::atomic<Node*> head;
std::atomic<Node*> tail;
public:
LockFreeQueue() {
Node* dummy = new Node{T{}, nullptr};
head.store(dummy);
tail.store(dummy);
}
void enqueue(T value) {
Node* newNode = new Node{value, nullptr};
Node* oldTail = tail.load();
while (!tail.compare_exchange_weak(oldTail, newNode)) {
// Continue trying to update tail
}
oldTail->next = newNode;
}
bool dequeue(T& result) {
Node* oldHead = head.load();
if (oldHead == tail.load()) {
return false; // Queue is empty
}
result = oldHead->next->data;
head.store(oldHead->next);
delete oldHead; // Free memory
return true;
}
~LockFreeQueue() {
// Clean up remaining nodes
T temp;
while (dequeue(temp));
}
};
How to Use the Lock-Free Queue?
To demonstrate practical usage of the lock-free queue, consider the following producer-consumer model:
void producer(LockFreeQueue<int>& queue) {
for (int i = 0; i < 100; ++i) {
queue.enqueue(i);
}
}
void consumer(LockFreeQueue<int>& queue) {
int value;
while (queue.dequeue(value)) {
// Process value
}
}
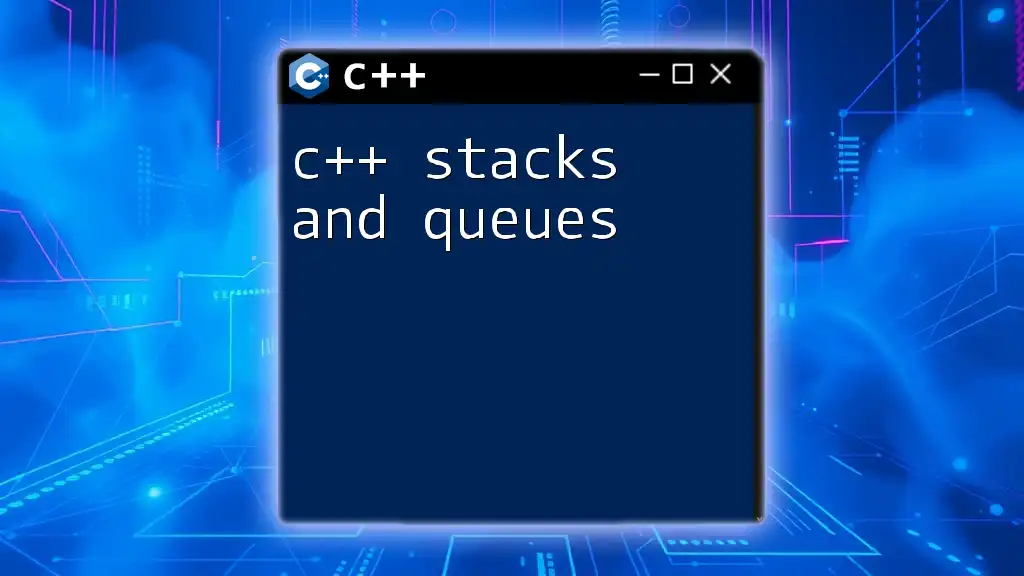
Error Handling and Memory Management
Common Issues in Lock-Free Queue Implementation
When implementing a lock-free queue, developers may encounter memory leaks and race conditions. Since no locks are used, the correct management of shared resources becomes crucial.
Encountering a race condition can lead to unpredictable behavior, where two threads might attempt to update the same node simultaneously. This can corrupt the state of the queue if not managed correctly.
Guidelines for Safe Memory Management
To help mitigate these issues, one solid approach is to use smart pointers, which automatically manage memory and prevent leaks. In a lock-free context, they can simplify ownership issues when a node is dequeued or deleted.
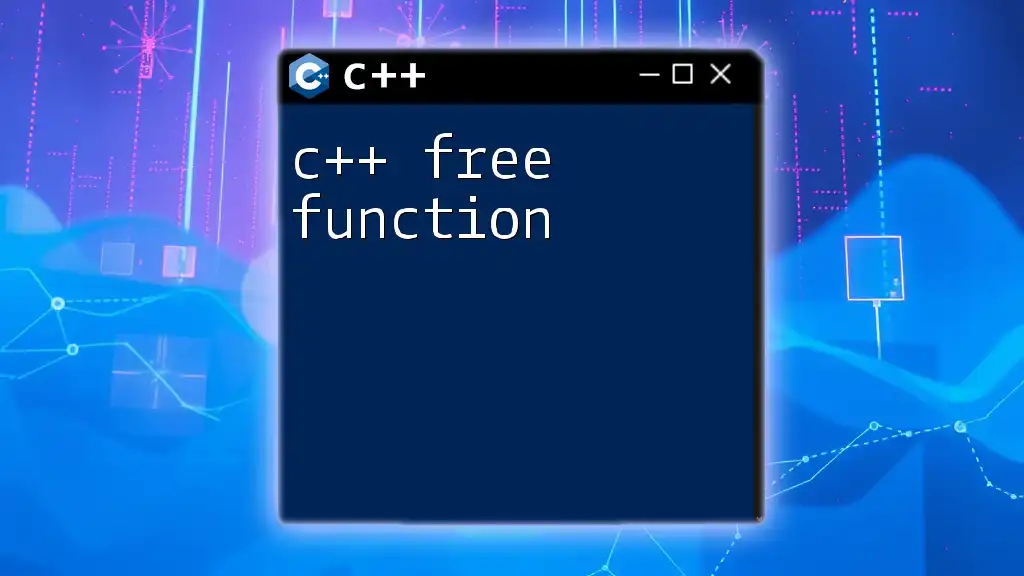
Performance Considerations
Benchmarks: Lock-Free Queue vs. Traditional Queues
When it comes to performance metrics, lock-free queues often outperform traditional queues, especially in scenarios with high contention and many concurrent threads. Benchmarks reveal that latency can be significantly reduced, leading to faster overall processing times.
Limitations of Lock-Free Queues
It's important to acknowledge that lock-free queues may not always be the best choice. In scenarios with low contention, the overhead associated with managing atomic operations can exceed the performance benefits.
A lock-free queue might also not be the most appropriate choice if the queue's size is expected to grow significantly, as this could lead to problems with memory management.
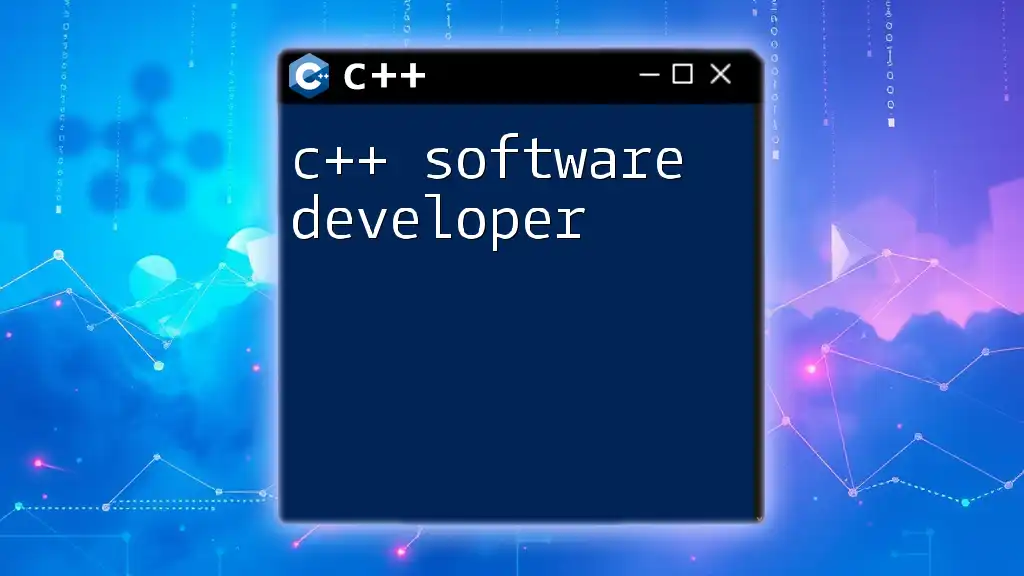
Advanced Topics
Variations of Lock-Free Queues
There are various implementations of lock-free queues tailored for specific use cases:
- Single Producer / Single Consumer (SPSC): Designed for scenarios where a single producer pushes data and a single consumer pulls data.
- Multi-Producer / Multi-Consumer (MPMC): Allows multiple producers and consumers to interact with the queue simultaneously.
Implementing variations requires understanding the specific challenges and optimization techniques relevant to each model.
Future of Lock-Free Queues in C++
As the demand for concurrency and performance continues to grow, lock-free queues are becoming increasingly relevant in modern C++ programming. Future developments in both hardware and software will likely contribute to better and more efficient implementations, keeping pace with evolving technology.
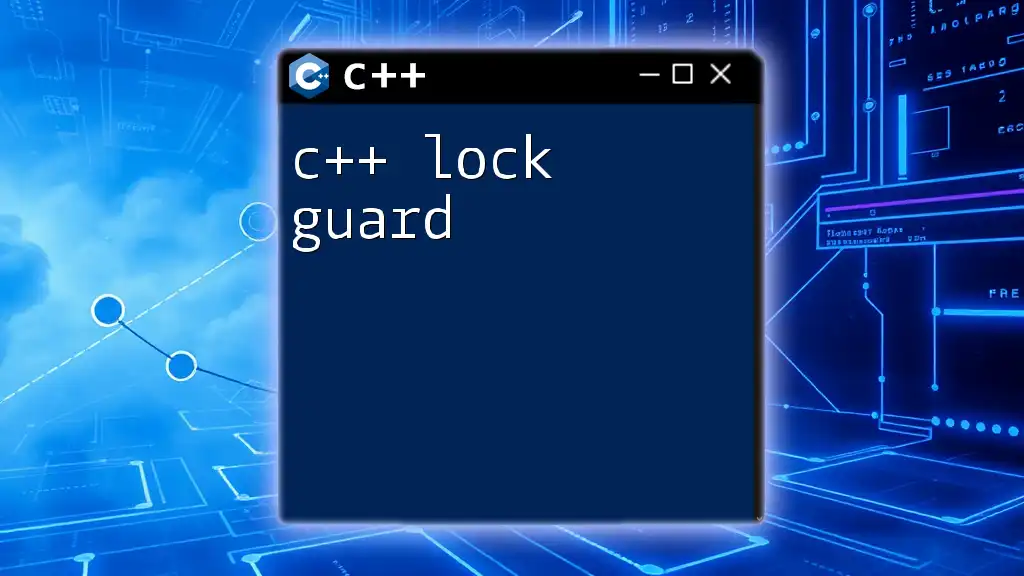
Conclusion
In summary, the C++ lock-free queue presents a compelling option for developers working in multi-threaded environments. While challenging, mastering the concepts behind atomic operations and non-blocking algorithms will yield highly efficient data structures that can enhance application performance.
Investing time into understanding and implementing lock-free queues can lead to cleaner, more efficient code that supports high concurrency. As always, continuous learning and experimentation are key to successful programming in C++.