In C++, you can determine whether a number is odd or even by using the modulus operator `%` to check the remainder when the number is divided by 2.
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter an integer: ";
cin >> number;
if (number % 2 == 0) {
cout << number << " is even." << endl;
} else {
cout << number << " is odd." << endl;
}
return 0;
}
Basic Concepts of Odd and Even Numbers
Odd and even numbers are fundamental to understanding how numbers work in C++. In programming, recognizing whether a number is odd or even is crucial for various algorithms, validations, and game mechanics.
Even Numbers are defined as integers that can be divided by 2 without a remainder (e.g., 0, 2, 4, 6). Conversely, Odd Numbers leave a remainder of 1 when divided by 2 (e.g., 1, 3, 5, 7). Understanding these definitions will serve as the foundation for implementing checks in C++.
Determining whether a number is odd or even in C++ can play an essential role in areas such as input validation, controlling loop iterations, or even simple game logic. For instance, you might want to ensure that a user enters only even numbers for a game that requires even-numbered play, enhancing user experience and input accuracy.
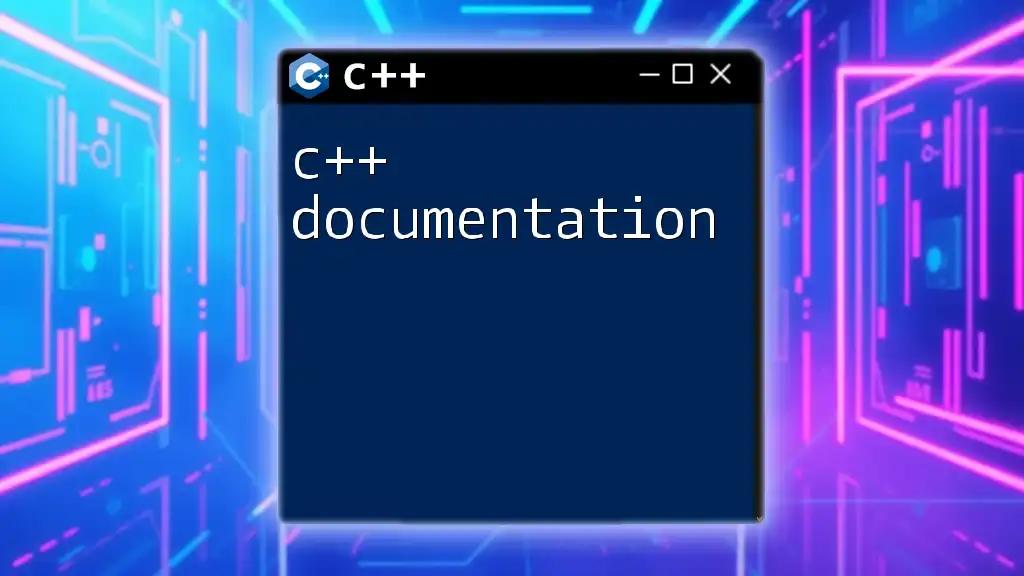
Methods to Check Odd or Even in C++
Using the Modulus Operator
One of the most straightforward methods to determine if a number is odd or even in C++ is by utilizing the modulus operator (`%`). This operator computes the remainder when one number is divided by another.
Here's a simple implementation to check for odd or even:
#include <iostream>
using namespace std;
void checkOddOrEven(int number) {
if (number % 2 == 0) {
cout << number << " is even." << endl;
} else {
cout << number << " is odd." << endl;
}
}
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
checkOddOrEven(num);
return 0;
}
In this code snippet, the `checkOddOrEven` function takes an integer as input. The condition `number % 2 == 0` evaluates whether the number is even. If it is true, the program outputs that the number is even; otherwise, it indicates that the number is odd. This simple method is widely used and quite effective for validating odd and even numbers.
Using Bitwise AND Operator
Another efficient method to determine whether a number is odd or even is to use the bitwise AND operator (`&`). This approach is particularly fast and is utilized at a lower level:
#include <iostream>
using namespace std;
void checkOddOrEven(int number) {
if (number & 1) {
cout << number << " is odd." << endl;
} else {
cout << number << " is even." << endl;
}
}
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
checkOddOrEven(num);
return 0;
}
In this code, the expression `number & 1` checks the least significant bit (LSB) of the number. If the LSB is 1, the number is odd; if it is 0, the number is even. This method is slightly more efficient than using the modulus operator, particularly in performance-critical applications.
Using C++ Standard Library Functions
You can also utilize functions from the C++ Standard Library to check if numbers are odd or even.
By using `std::iota` and `std::for_each`, you can fill and process an array of numbers in a concise manner:
#include <iostream>
#include <vector>
#include <numeric>
using namespace std;
int main() {
vector<int> numbers(10);
iota(numbers.begin(), numbers.end(), 0); // Fills with values 0 to 9
for_each(numbers.begin(), numbers.end(), [](int num) {
cout << num << (num % 2 == 0 ? " is even." : " is odd.") << endl;
});
return 0;
}
This code snippet first initializes a vector containing numbers from 0 to 9. The `std::for_each` function then iterates through this vector, using a lambda function to determine whether each number is odd or even. This demonstrates how using the C++ Standard Library can simplify your code and make it more readable.
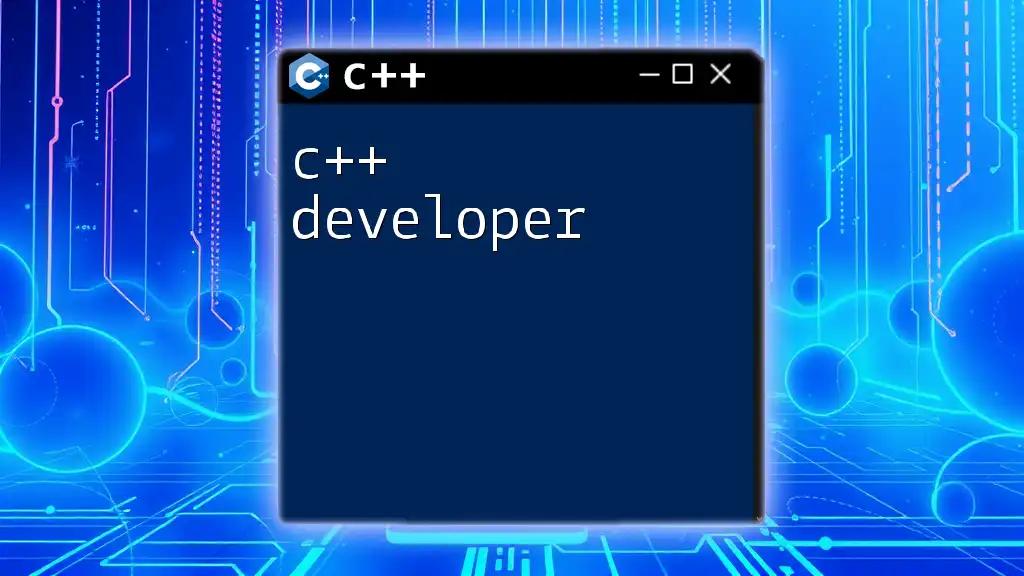
Tips for Effectively Using Odd or Even Checks
To enhance your coding skills and avoid common pitfalls while implementing C++ odd or even checks, consider the following:
- Avoid Common Mistakes: Ensure that you are working with integers when performing checks, as floating-point numbers may yield inaccurate results due to how they are represented in memory.
- Robust Testing: It is essential to test your implementation with various input values, including negative numbers and zero, to ensure accuracy across all scenarios.
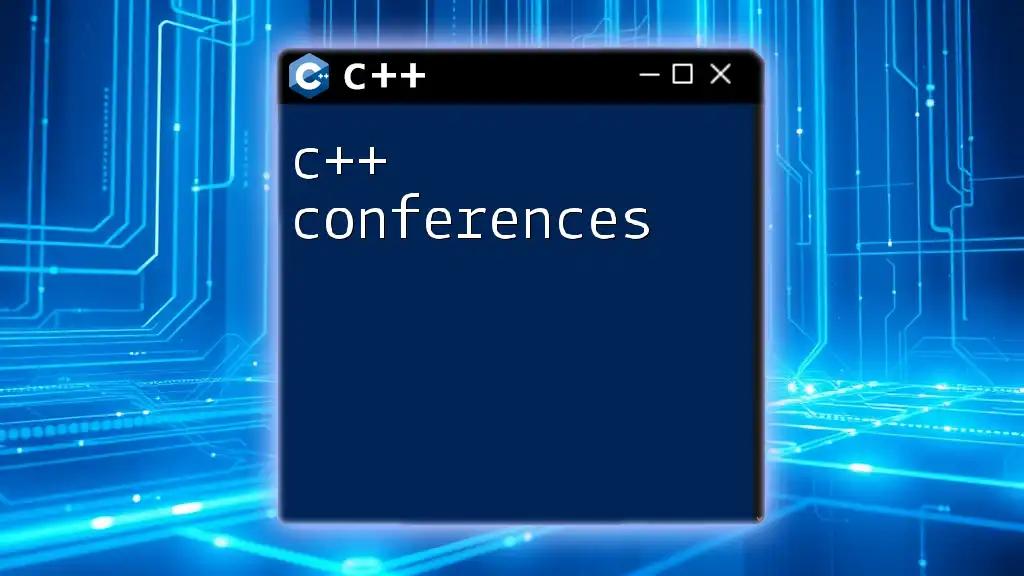
Real-World Applications of Odd or Even Checks in C++
Simple Games
In simple gaming applications, odd or even checks can dictate critical game mechanics. For instance, you can create turn-based games where players take turns based on whether the current round number is odd or even, enhancing interactivity and user engagement.
User Input Validation
In forms or applications requiring user input, implementing odd or even checks can help validate responses—ensuring that users enter only specific types of numbers based on requirements.
Algorithms in Competitive Programming
In competitive programming, checking for odd or even numbers can lead to more efficient algorithms. For instance, when iterating through large datasets, determining whether a number is odd or even can guide the flow of the algorithm and streamline computations.
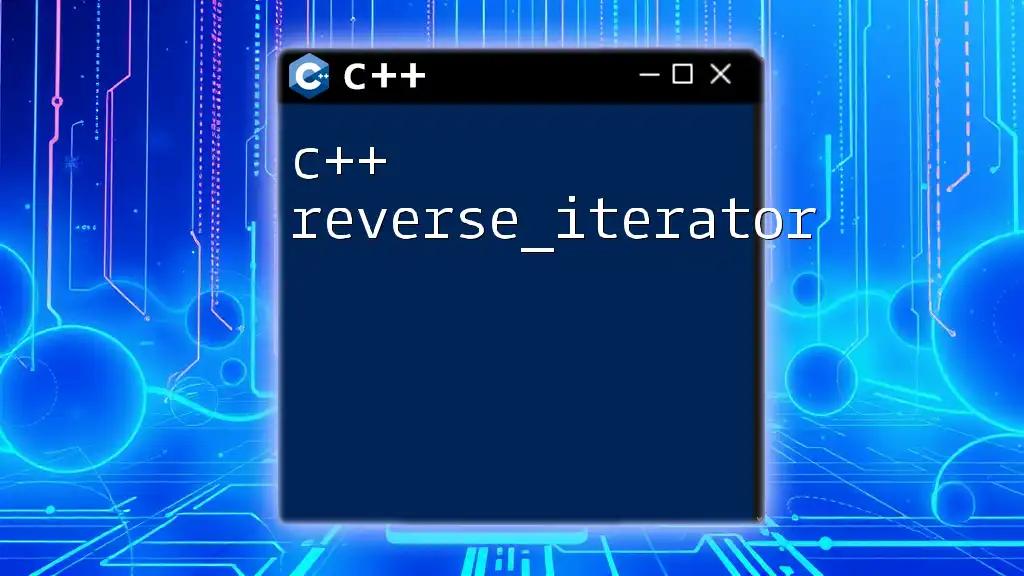
Conclusion
In summary, understanding how to effectively check if a number is odd or even in C++ is an essential programming skill. By utilizing methods such as the modulus operator, bitwise AND operator, or even functions from the C++ Standard Library, you can optimize your code for various applications. As you experiment and implement these techniques, you'll gain a deeper understanding of number operations and enhance your programming capabilities in C++.