A C++ stack trace provides a summary of the active function calls at a specific point in the program, which is useful for debugging errors and understanding the flow of execution.
#include <execinfo.h>
#include <iostream>
#include <stdlib.h>
void printStackTrace() {
void *array[10];
size_t size;
// get void*'s for all entries in the call stack
size = backtrace(array, 10);
// print the call stack
backtrace_symbols_fd(array, size, STDOUT_FILENO);
}
void testFunction() {
printStackTrace();
}
int main() {
testFunction();
return 0;
}
What is a Stack Trace?
A stack trace is a report of the active stack frames at a certain point in time during the execution of a program. It serves as a snapshot of the call stack, which is an essential part of understanding the flow of a program. When an error occurs, such as an exception or segmentation fault, the stack trace can help identify where the error originated and the sequence of function calls that led to it.
Importance in C++ Development
Understanding C++ stack traces is critical for debugging and improving code quality. They provide developers with insights into function calls that were executed prior to an error, making it easier to trace back the flow of the application and determine the cause of the problem.
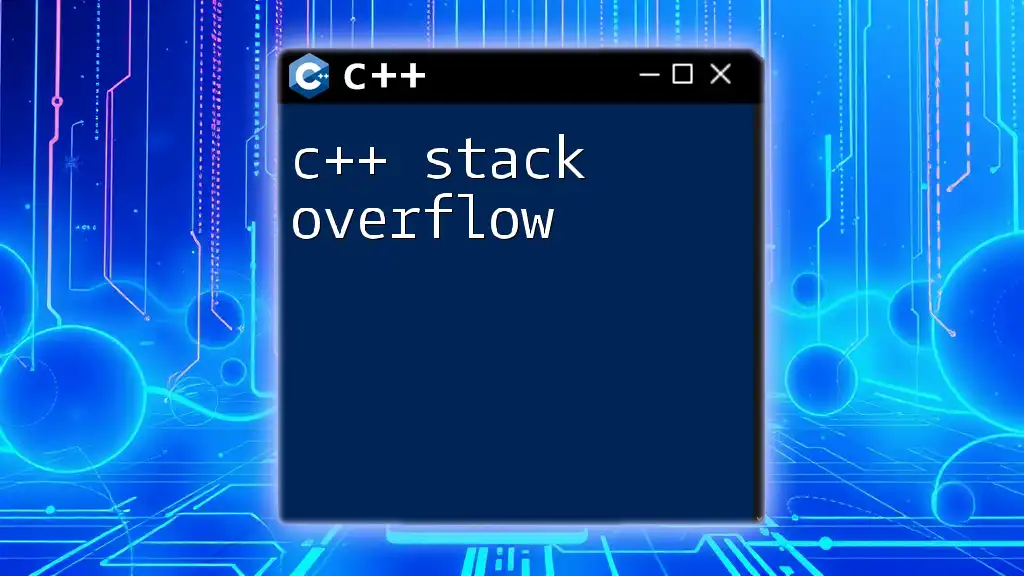
Understanding Stack Traces in C++
How Stack Traces Work
The stack trace reflects the call stack, which is a data structure that keeps track of active subroutines in a program. Each time a function is called, a new stack frame is created and added to the stack. Conversely, when a function exits, its stack frame is removed from the stack.
Stack Frame Structure
A stack frame contains crucial information, such as:
- Return Address: The address to return to after the function execution.
- Local Variables: Any variables that were declared within the function.
- Parameters: Values passed to the function.
Understanding the stack frame helps in recognizing how C++ stack traces are constructed and used.
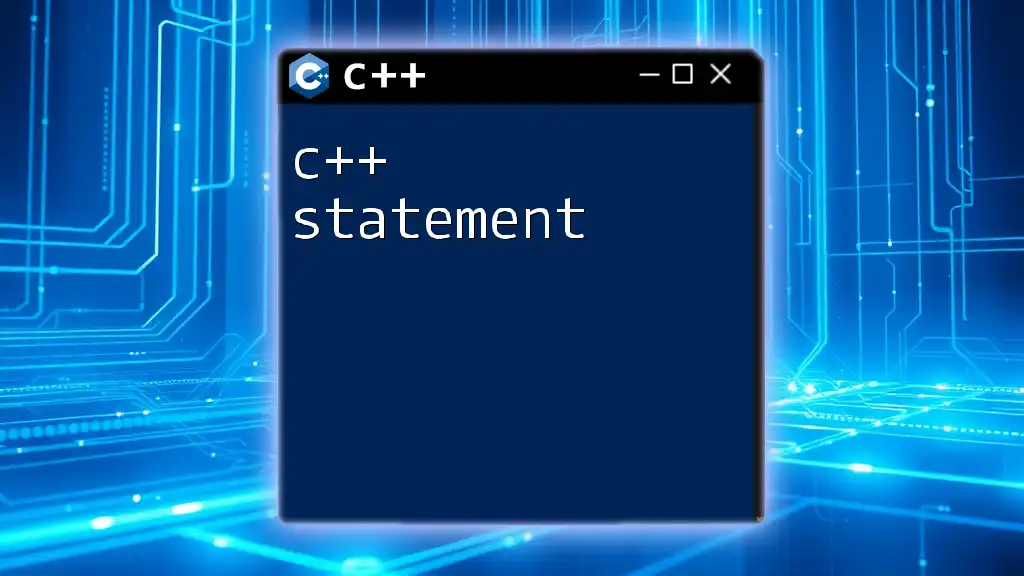
Generating Stack Traces in C++
Basic Methods for Generating a Stack Trace
C++ does not provide built-in support for generating stack traces. However, there are various ways to obtain stack traces using system functions or third-party libraries. One common method is by integrating platform-specific functions available in operating systems like Linux and Windows.
Using Third-Party Libraries
Using third-party libraries simplifies the process of generating and managing stack traces. Here are two popular libraries:
Google Breakpad
Google Breakpad handles crash reporting and provides a robust framework for capturing stack traces.
Boost.Stacktrace
Boost.Stacktrace is an easy-to-use library that can provide rich stack trace information.
To install Boost.Stacktrace, you can use a package manager like vcpkg:
vcpkg install boost-stacktrace
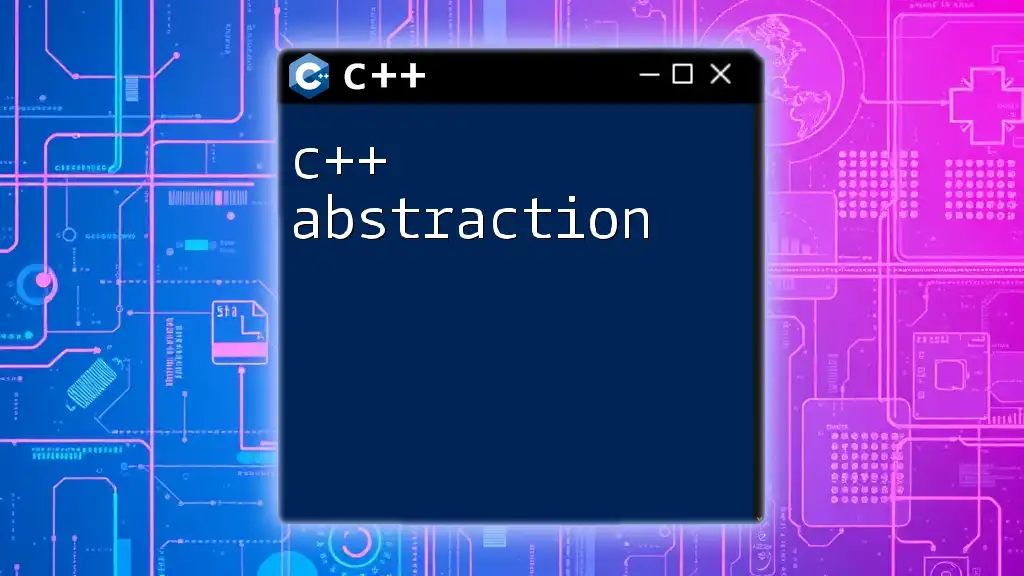
Printing a Stack Trace in C++
How to Print Stack Trace Using Built-in Functions
In some environments, you can use system-specific functions to generate a stack trace. For example, on Linux, the `backtrace` function can be utilized:
#include <execinfo.h>
#include <iostream>
#include <signal.h>
#include <stdlib.h>
void signalHandler(int signal) {
void *array[10];
size_t size;
// Get void*'s for all entries currently on the stack
size = backtrace(array, 10);
// Print out all the frames to stderr
std::cerr << "Error: signal " << signal << "\n";
backtrace_symbols_fd(array, size, STDERR_FILENO);
exit(1);
}
This function captures the stack at the time a signal is received, and uses `backtrace_symbols_fd` to print the symbols to the standard error.
Using Third-Party Libraries to Print Stack Trace
Using Boost.Stacktrace enhances the readability and functionality of stack traces. Here’s how to use it:
#include <boost/stacktrace.hpp>
#include <iostream>
void throwError() {
throw std::runtime_error("An error occurred!");
}
void print_stack_trace() {
std::cout << "Stack trace:\n" << boost::stacktrace::stacktrace() << std::endl;
}
int main() {
try {
throwError();
} catch (const std::exception &e) {
std::cout << "Caught exception: " << e.what() << std::endl;
print_stack_trace();
}
return 0;
}
In this example, an exception is thrown, caught, and the stack trace is printed, providing clear insight into the call history that led to the exception.
Error Handling and Stack Trace Printing
Integrating stack trace printing in your error handling can greatly enhance your debugging capabilities. When an error occurs, ensure to capture a stack trace before handling the exception. This allows you to examine the state of your application at the time of the error, leading to faster diagnosis and resolution.
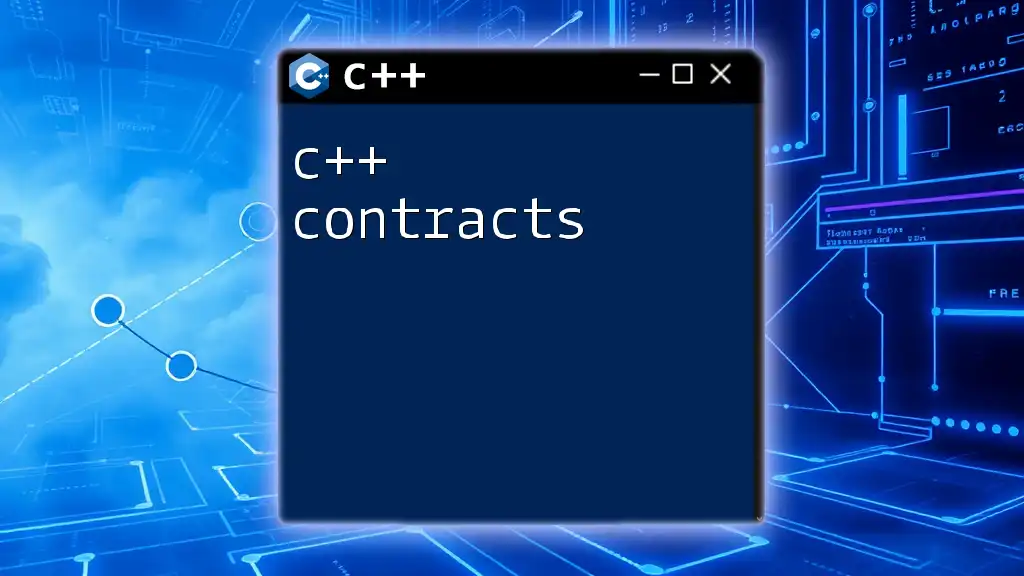
Best Practices for Analyzing Stack Traces
Common Tricks for Debugging with Stack Traces
When analyzing stack traces, look for:
- Patterns: Identify if errors are occurring in specific modules or functions, which can help pinpoint the problem areas.
- Trace Depth: Assess whether stack trace depth is consistent or varies significantly, as this may indicate recursion issues or a complex flow.
Using Stack Traces for Performance Analysis
Stack traces can also be invaluable in performance profiling. Identifying parts of the application that frequently appear in the call stack can lead to optimizations, resulting in overall improved performance.
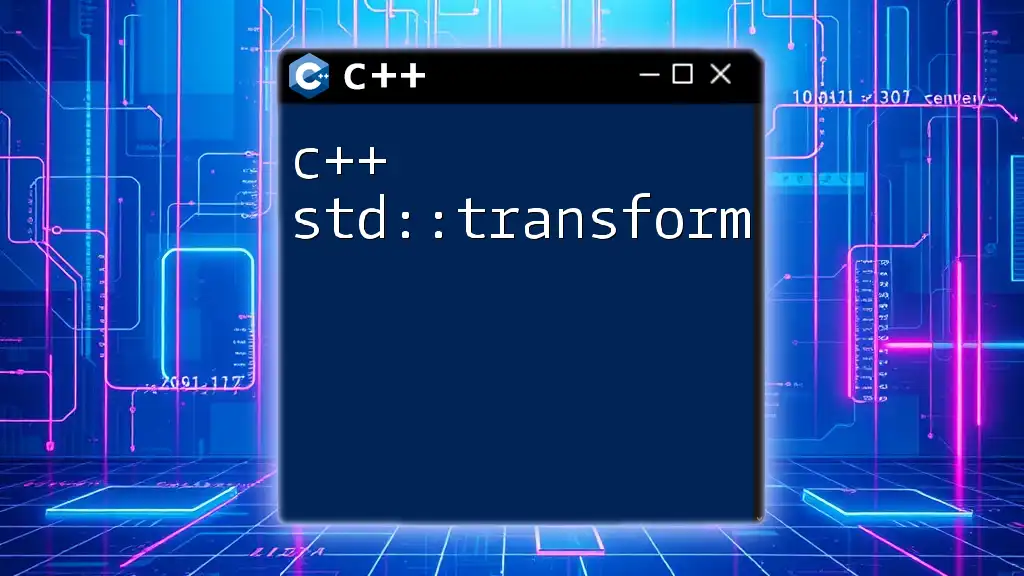
Real-World Use Cases
Case Study: Debugging a Memory Leak
Consider an application that encounters a memory leak. By utilizing stack traces, you can analyze functions that allocate memory. Properly examining each function in the call stack can reveal forgotten `delete` statements or improper resource management.
Case Study: Identifying Recursion Depth Issues
When faced with a function that isn't terminating as expected due to excessive recursion, stack traces help identify how deep the recursion goes.
void recursiveFunction(int depth) {
if (depth == 0) return;
recursiveFunction(depth - 1);
}
Running the above code with a stack trace will highlight how many times the function is called, allowing developers to adjust their logic to avoid deep recursion.
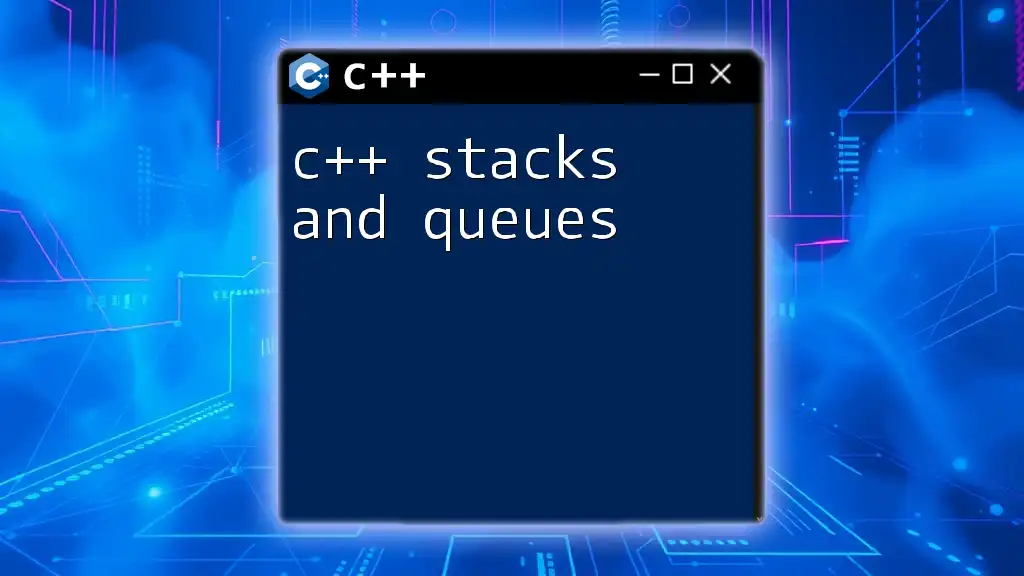
Conclusion
Understanding and utilizing C++ stack traces is crucial for efficient debugging and performance tuning. They map out the sequence of function calls leading up to an error, enabling developers to diagnose problems swiftly. Practicing stack trace analysis will significantly enhance your skills in managing exceptions and improving the quality of your C++ applications.
Encouragement to Practice Stack Trace Analysis
To improve your C++ debugging capabilities, start incorporating stack trace analysis into your projects today. Exploring additional resources and tutorials will further fine-tune your understanding and expertise in managing C++ stack traces effectively.