C++ is a powerful language for image processing due to its performance and access to various libraries, allowing for efficient manipulation and analysis of images.
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("image.jpg");
cv::Mat grayImage;
cv::cvtColor(image, grayImage, cv::COLOR_BGR2GRAY);
cv::imwrite("gray_image.jpg", grayImage);
return 0;
}
What is Image Processing?
Image processing is a technique that involves the manipulation of images to enhance or extract useful information. It encompasses a wide range of operations, from simple adjustments such as resizing and filtering to complex algorithmic tasks, such as image recognition and segmentation. This field finds its application in various industries, including healthcare (e.g., MRI and CT scans), automotive (e.g., self-driving cars), and entertainment (e.g., video processing).
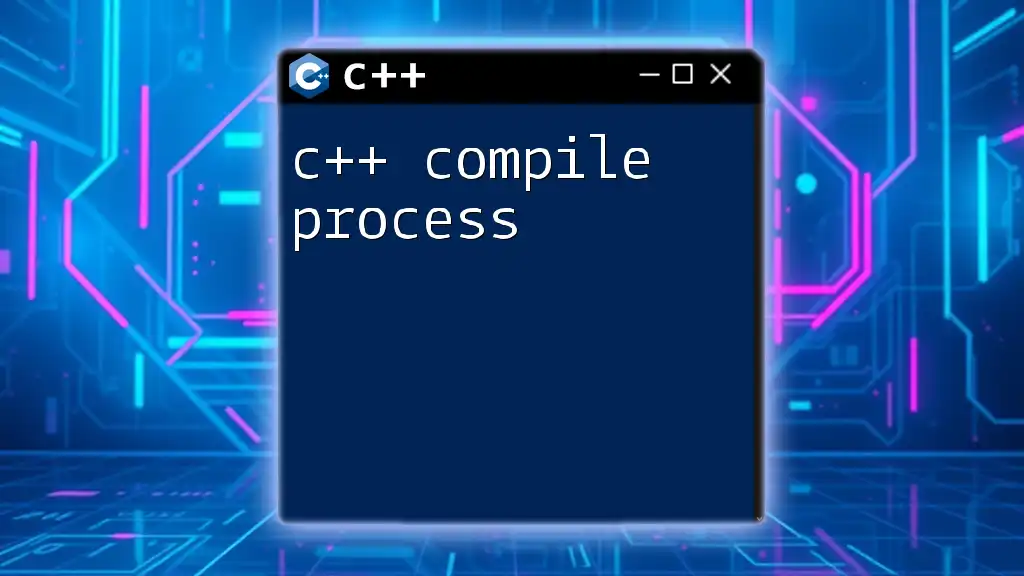
Why Use C++ for Image Processing?
C++ stands out as an excellent choice for image processing tasks due to several key factors. First and foremost, speed and efficiency are critical in image processing, especially when handling large datasets or real-time applications. C++ allows developers to write high-performance code that can utilize system resources effectively.
Another significant advantage is fine control over system resources. C++ provides low-level programming capabilities that allow developers to manage memory efficiently, an important aspect when dealing with images.
Additionally, C++ boasts robust libraries and frameworks dedicated to image processing. One of the most popular is OpenCV, which offers an extensive set of functions that enable developers to implement complex image processing operations with ease.
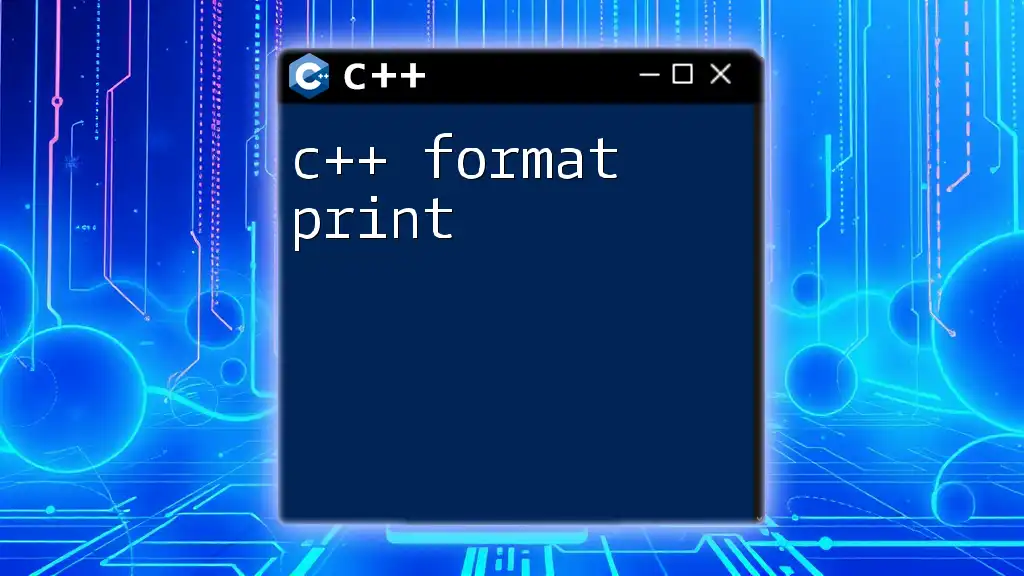
Getting Started with C++ for Image Processing
Prerequisites for Learning
To effectively use C++ for image processing, a basic understanding of C++ syntax and programming concepts is essential. Familiarity with relevant libraries, particularly OpenCV, will greatly enhance the learning curve.
Setting Up Your Environment
Selecting the right Integrated Development Environment (IDE) is crucial for a productive coding experience. Popular choices include Visual Studio, Code::Blocks, and CLion.
Installing necessary libraries is the next step. OpenCV is the go-to library for image processing in C++.
Here’s how to install OpenCV on various platforms:
- Windows: Use vcpkg or download pre-built libraries.
- Linux: Install via the terminal with the command:
sudo apt-get install libopencv-dev
- macOS: You can use Homebrew:
brew install opencv
Following the installation, ensure that your IDE is linked to the OpenCV libraries to access its functionalities seamlessly.
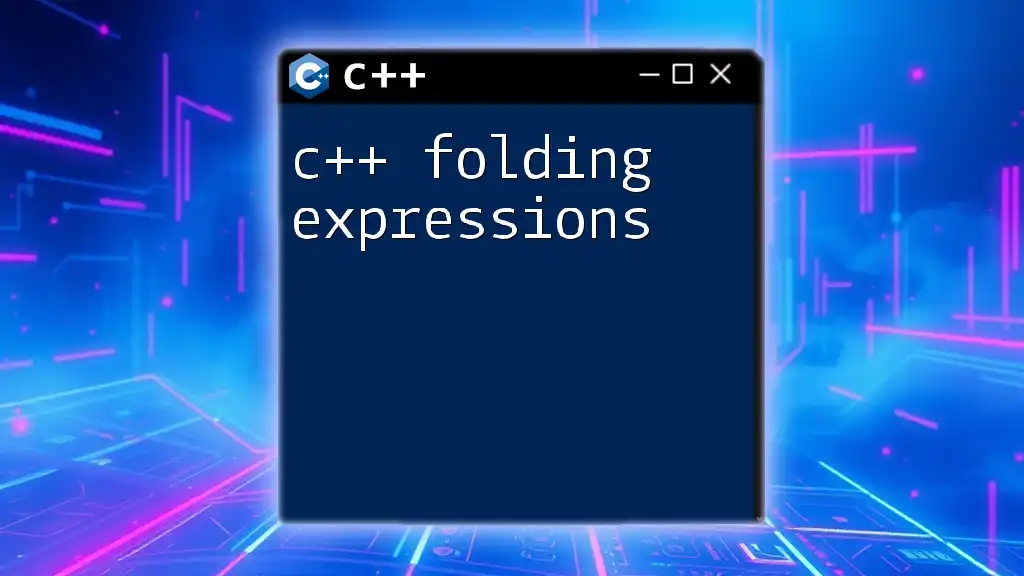
Understanding Image Representation in C++
How Images are Represented
Images are stored as pixel arrays, where each pixel represents color information. Common color representations include RGB (Red, Green, Blue) for colored images and Grayscale for monochrome images. Understanding image formats such as JPEG, PNG, and BMP is also vital, as different formats may require specific handling in C++.
Loading and Displaying Images
Using OpenCV, loading and displaying images is straightforward.
Here’s a simple code example that demonstrates how to load an image:
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
Mat image = imread("path/to/image.jpg");
imshow("Loaded Image", image);
waitKey(0); // Wait for a key press to close the image window
return 0;
}
In this snippet, `imread` loads an image from the specified path, and `imshow` displays it in a window. The program waits for a key press before terminating.
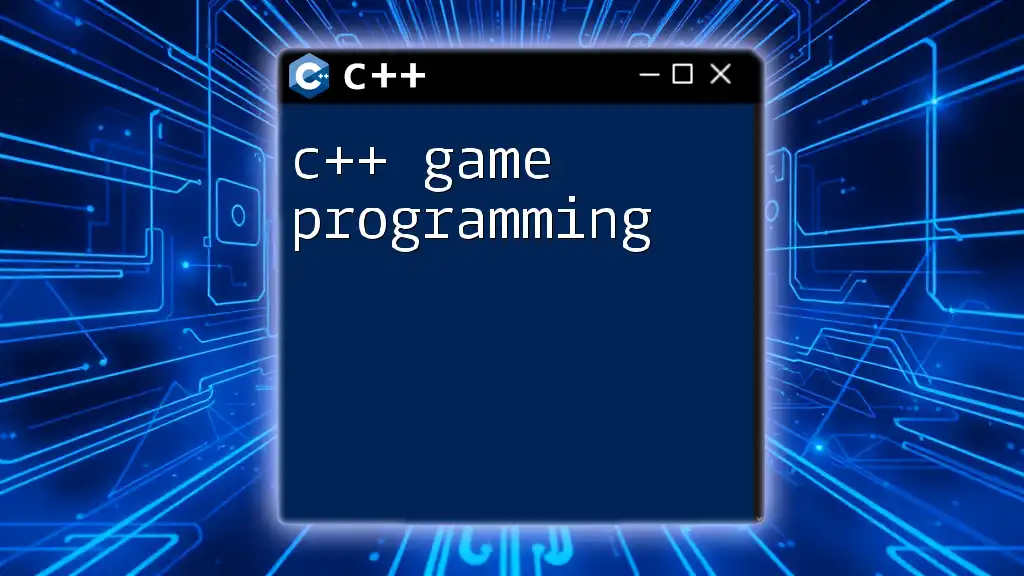
Basic Image Processing Techniques
Image Resizing and Scaling
Resizing an image allows you to alter its dimensions, which can be useful for various applications, including web optimization and real-time tasks.
Here’s a code example demonstrating how to resize an image:
Mat resizedImage;
resize(image, resizedImage, Size(newWidth, newHeight));
In this example, `newWidth` and `newHeight` specify the desired dimensions. The `resize` function modifies the image to fit those dimensions, effectively changing its size.
Image Filtering and Smoothing
Image filtering is crucial for removing noise and enhancing image quality. Common filters include Gaussian filters and Median filters, which are essential for tasks like blurring or sharpening images.
Here’s how to apply a Gaussian filter using OpenCV:
Mat filteredImage;
GaussianBlur(image, filteredImage, Size(15, 15), 0);
In this case, `Size(15, 15)` defines the filter size, while a sigma value of 0 lets OpenCV automatically calculate it. This operation smooths the image, reducing high-frequency noise.
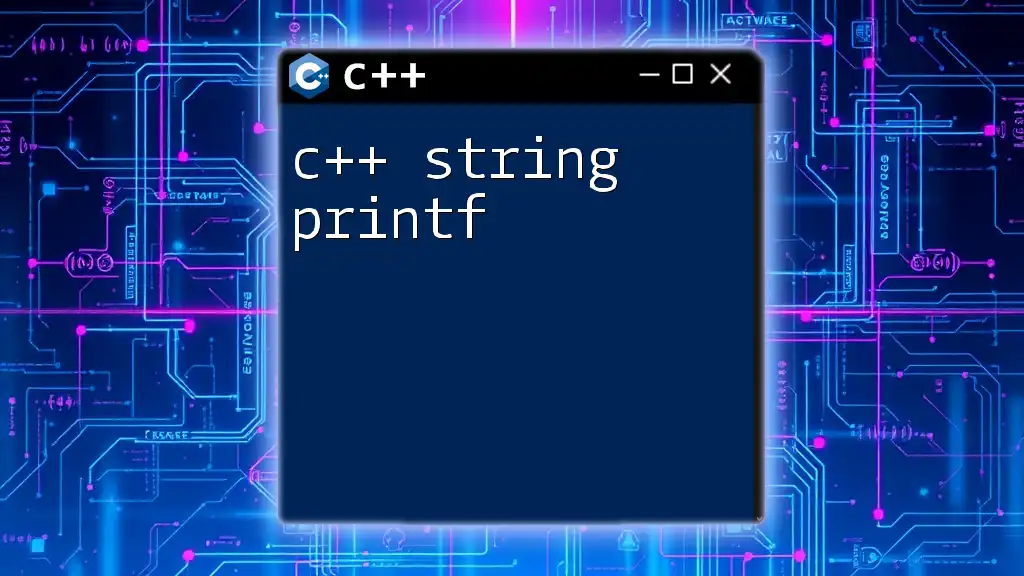
Advanced Image Processing Techniques
Edge Detection
Edge detection plays a pivotal role in image analysis, allowing us to identify object boundaries. The Canny edge detection algorithm is one of the most popular methods used.
Here’s a code example for implementing Canny edge detection:
Mat edges;
Canny(image, edges, 100, 200);
In this code, the values `100` and `200` are thresholds for detecting edges. Adjusting these values changes the sensitivity of the edge detection process.
Image Transformation Techniques
Geometric transformations, such as rotation, scaling, and translation, allow for the manipulation of image perspective.
To rotate an image, you can use the following code:
Mat rotatedImage;
Point2f center(resizedImage.cols/2.0, resizedImage.rows/2.0); // Define the center of rotation
Mat rotationMatrix = getRotationMatrix2D(center, angle, 1.0); // Create a rotation matrix
warpAffine(resizedImage, rotatedImage, rotationMatrix, resizedImage.size()); // Apply the rotation
Here, `getRotationMatrix2D` generates a transformation matrix, which is then applied to the image with `warpAffine`. The `angle` defines how much the image should be rotated.
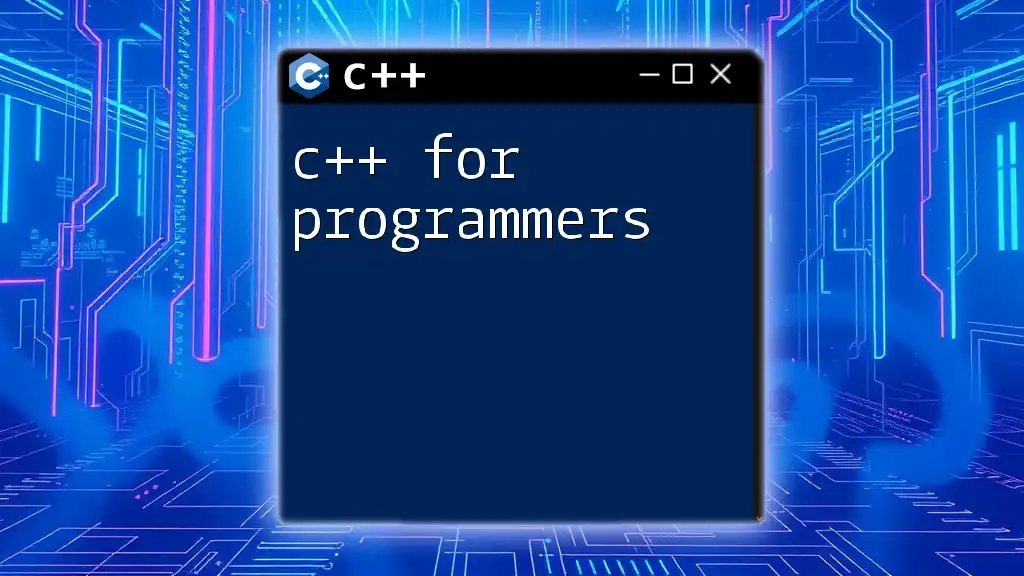
Working with Video Streams
Capturing Video from a Camera
C++ also enables video capture from cameras, making it possible to apply image processing techniques on video streams.
Here’s an example of how to capture video using OpenCV:
VideoCapture cap(0); // 0 for default camera
Mat frame;
while(true) {
cap >> frame; // Capture a new frame
imshow("Video Frame", frame); // Display the frame
if(waitKey(30) >= 0) break; // Exit loop on key press
}
In this code, `VideoCapture` initializes video capture from the default camera. The program captures frames in a loop and displays them until a key is pressed.
Applying Image Processing Techniques on Video
Real-time image processing opens up possibilities for various applications, including motion detection and real-time object tracking. By layering the techniques you’ve learned, you can enhance video streams dynamically.
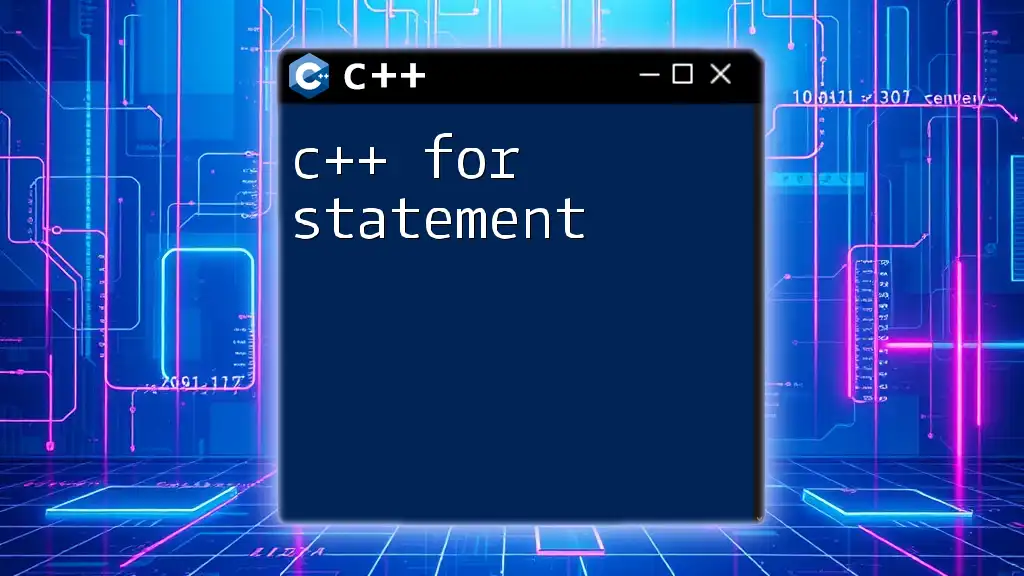
Conclusion
By mastering C++ for image processing, you unlock the potential to develop sophisticated applications that manipulate and analyze visual data effectively. The speed and control offered by C++ combined with the powerful capabilities of libraries like OpenCV create an ideal environment for image processing innovations. As you explore further, experiment with different techniques and tools to expand your knowledge and capabilities in this exciting field.