C++ folding expressions provide a convenient way to apply a binary operator to a parameter pack in templates, simplifying operations like summation or logical conjunction over multiple arguments.
Here's an example of a folding expression using the binary `+` operator:
#include <iostream>
template<typename... Args>
auto sum(Args... args) {
return (args + ...); // Fold expression
}
int main() {
std::cout << sum(1, 2, 3, 4, 5) << std::endl; // Outputs: 15
return 0;
}
Understanding Folding Expressions in C++
What are Folding Expressions?
C++ folding expressions are a powerful feature introduced in C++17 that allow for the easy and efficient handling of parameter packs in variadic templates. They provide a syntactically concise way to apply binary operators across arguments, enabling more readable code and eliminating the need for manual construction of operations. By leveraging folding expressions, developers can reduce boilerplate code and minimize the risk of errors that come from repetitive patterns.
The Evolution of Folding Expressions in C++
Prior to the introduction of folding expressions, developers often resorted to alternative methods such as recursive template instantiations or manually expanding parameter packs using helper functions. These methods, while functional, were often cumbersome and resulted in verbose code that was difficult to read and maintain.
With the advent of C++17, folding expressions have simplified these operations. They not only promote code clarity but also optimize performance by reducing the level of indirect function calls. As a result, C++ programmers now have an elegant and efficient tool for working with variadic templates.
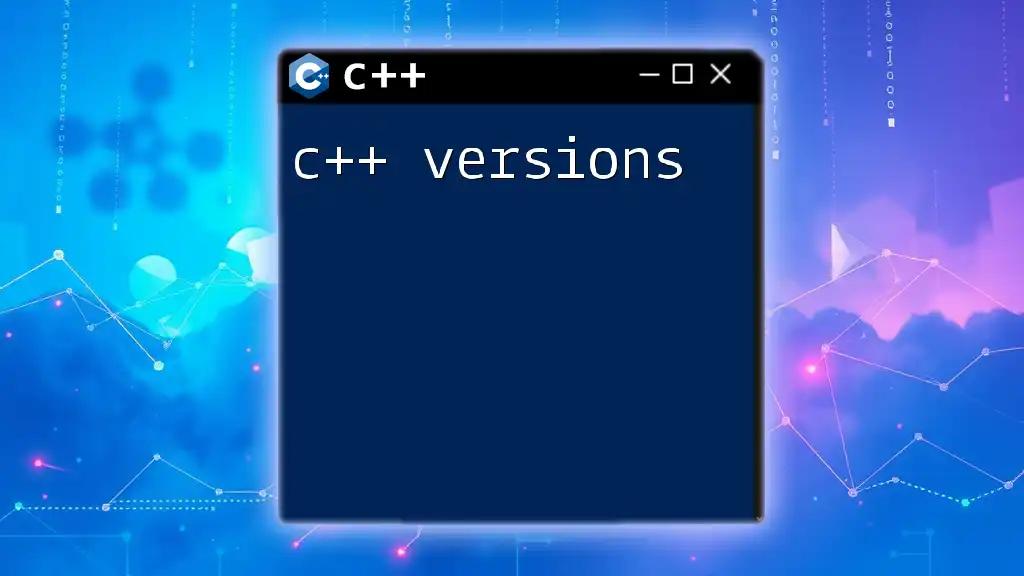
Types of Folding Expressions
Overview of Binary Fold Expressions
Binary fold expressions apply a binary operator to a parameter pack, allowing developers to specify an operation to combine all elements of the pack. There are two principal types: left-fold and right-fold.
In left-folding, the operation is applied from the left, while in right-folding, it operates from the right. The general syntax for a binary fold expression is as follows:
(... op args) // Fold Left
(args op ...) // Fold Right
Examples of Binary Fold Expressions
Imagine you want to create a function that computes the sum of multiple numbers. Here’s how you can implement that using a left fold:
template<typename... Args>
auto sum(Args... args) {
return (... + args); // Left fold
}
The above function effectively evaluates as `((arg1 + arg2) + arg3) + ...`. On the other hand, for a right fold, you could have:
template<typename... Args>
auto product(Args... args) {
return (args * ...); // Right fold
}
Overview of Unary Fold Expressions
Unary fold expressions simplify operations that involve a single parameter pack. They allow you to apply a unary operator to each element of the pack. The syntax for a unary fold is:
(... op args) // Unary Fold
Practical Usage Scenarios
For instance, if you wish to determine if all items in the pack are non-zero, you can efficiently write:
template<typename... Args>
bool allTrue(Args... args) {
return (... && args); // Unary fold
}
Here, `allTrue` will evaluate as `arg1 && arg2 && arg3 && ...`, which is a straightforward way to check conditions across multiple arguments.
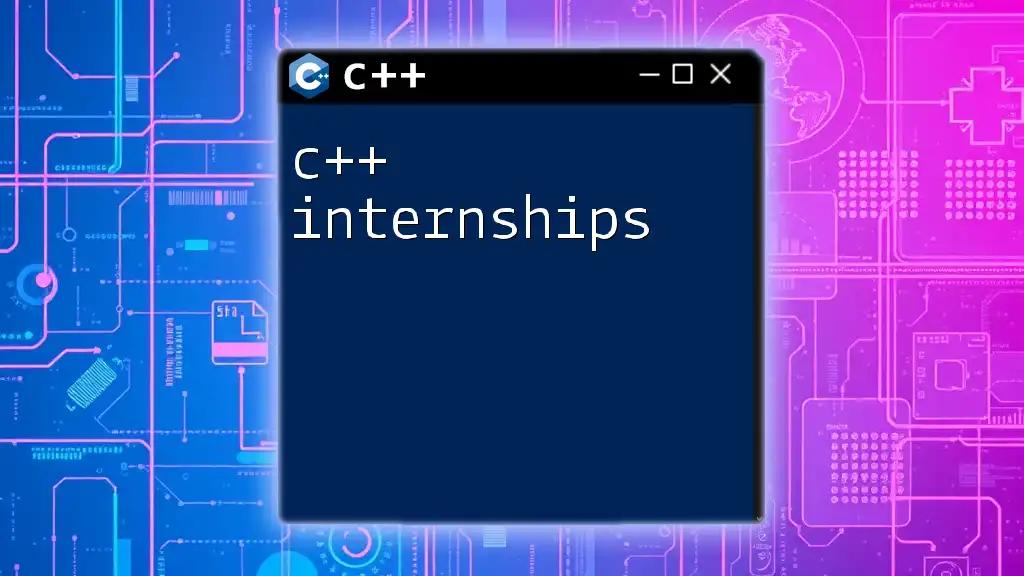
Syntax and Usage of Folding Expressions
Basic Syntax Explanation
The syntax for folding expressions can initially seem confusing, but breaking it down reveals its elegance. The folded operation relies on a binary operator, which must be one of the following: `+`, `-`, `*`, `&&`, `||`, etc. The nature of the operator defines how the parameters are combined, and parentheses are crucial for ensuring the intended order of operations.
Practical Examples of Folding Expressions
Fold expressions expressively simplify the implementation of operations on variadic templates. For instance, if you want to compute the product of a series of numbers, the left fold will allow you to achieve that succinctly:
template<typename... Args>
auto multiply(Args... args) {
return (... * args); // Left fold for multiplication
}
When calling `multiply(2, 3, 4)`, it evaluates as `((2 * 3) * 4)`, yielding `24`.
In another real-world scenario, you might want to log multiple values at once. Utilizing a unary fold makes this efficient:
template<typename... Args>
void printAll(Args... args) {
(std::cout << ... << args) << std::endl; // Print all arguments
}
This function concisely prints all the provided arguments to the console in a single line, demonstrating the efficiency of C++ folding expressions.
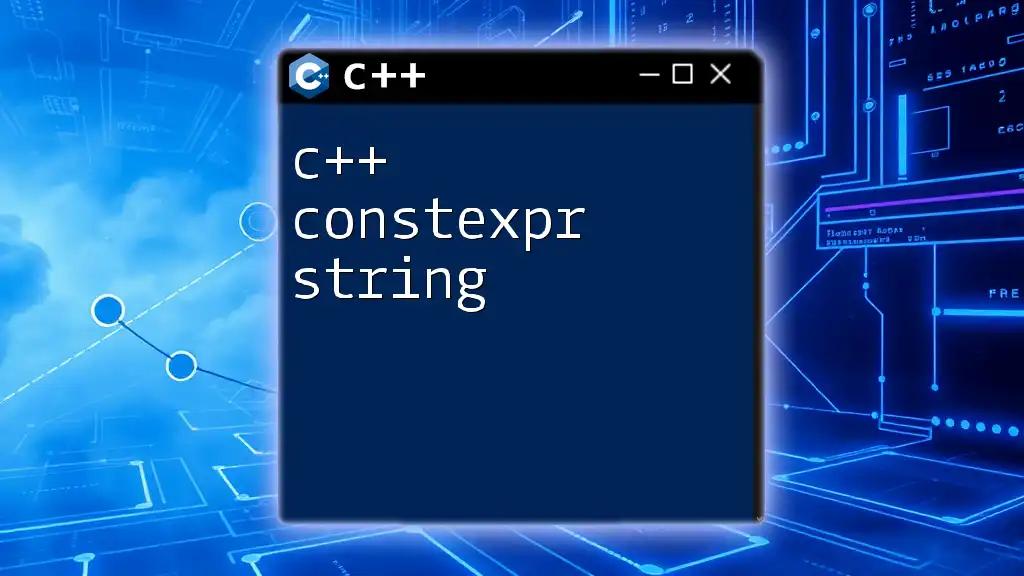
Use Cases for Folding Expressions
Reducing Code Complexity
Folding expressions lower the complexity of your codebase by promoting compact coding practices. By consolidating several lines of code into a single statement, developers can achieve clearer code that’s easier to read, manage, and refactor as needed. This not only makes the code more maintainable but also enhances collaboration among team members.
Enhancing Performance
The efficiency of folding expressions can lead to significant performance improvements. Because they minimize the number of function calls at compile-time, the resulting binary can be lighter and faster. For performance-critical applications, this can lead to tangible advancements in speed, especially when dealing with large numbers of parameters.
Applicability in Different Scenarios
Folding expressions shine in various scenarios, from mathematical computations like sums and products to checks and validations with Boolean operators. Their adaptability makes them a versatile tool in a C++ developer's toolkit. When comparing traditional recursive or iterative methods, folding expressions often result in less code and faster execution times.
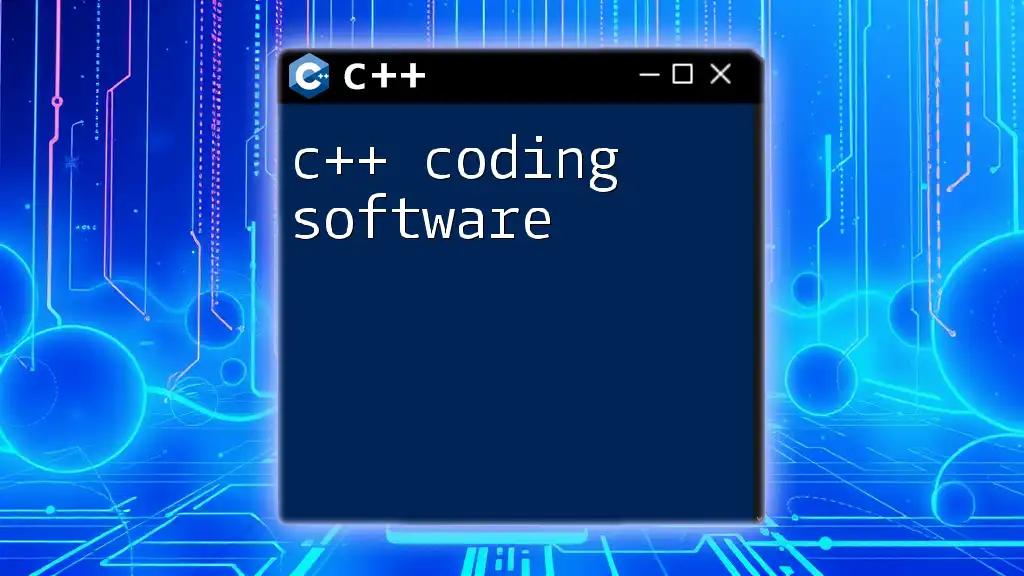
Common Pitfalls and Best Practices
Avoiding Logical Errors
While folding expressions are powerful, they come with their own set of challenges. One common pitfall occurs when developers incorrectly assume the order of evaluation. A wrong assumption about the operator's associativity can lead to logic errors in the program. Always clearly understand the implications of the operators you are using.
Best Practices for Writing Clear Folding Expressions
To ensure clarity, take care to document your folding operations and follow consistent naming conventions. Test thoroughly and consider edge cases—in particular, zero-length parameter packs can lead to compiler errors if not handled explicitly. Enforce readability by avoiding overly complex expressions, as they might obfuscate your intent.
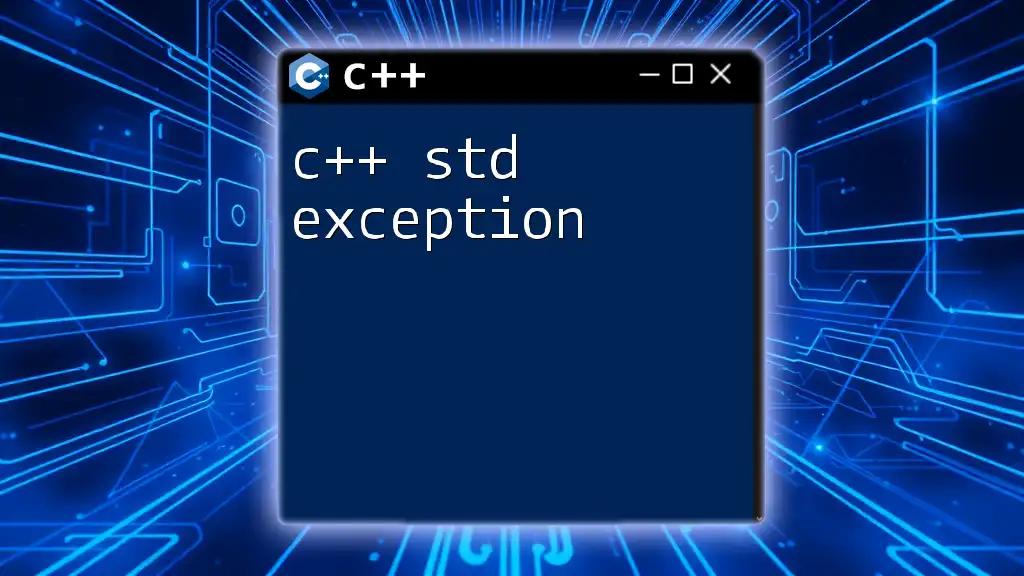
Conclusion
Summary of Key Takeaways
In summary, C++ folding expressions significantly enhance the way developers interact with variadic templates. They simplify operations by offering a concise syntax for combining elements, reduce code complexity, and offer performance benefits that are hard to overlook. Embracing folding expressions can lead to cleaner, efficient, and more maintainable code.
Further Resources
For those looking to dive deeper, explore the official C++ documentation on C++17 features and other resources such as recommended books and online courses focused on C++ programming.