Data structures and algorithm analysis in C++ focus on efficiently organizing data and understanding the performance of algorithms, enabling developers to write optimized and maintainable code.
Here’s a simple example demonstrating the use of a vector (a dynamic array) in C++ to store and display integer values:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Data Structures
Definition of Data Structures
A data structure is a specific way of organizing and storing data in a computer so that it can be accessed and modified efficiently. Data structures are crucial in programming because they allow for the effective use of memory and improve the performance of algorithms, making them foundational elements in software development.
Types of Data Structures
Primitive Data Structures
Primitive data structures are the basic types of data that are directly supported by programming languages. In C++, examples of primitive data structures include:
- Integers
- Floats
- Characters
For instance, a simple C++ declaration for these types looks as follows:
int number = 42;
float price = 19.99;
char grade = 'A';
Non-Primitive Data Structures
Non-primitive data structures are more complex and can be classified into two main categories: linear and non-linear data structures.
Linear Data Structures
Linear data structures have elements arranged sequentially. Examples include:
- Arrays: A collection of elements stored in contiguous memory locations.
- Linked Lists: A collection of nodes where each node points to the next.
The implementation of an array in C++ is straightforward:
int arr[5] = {1, 2, 3, 4, 5};
For linked lists, here's a simple C++ structure for a node:
struct Node {
int data;
Node* next;
};
Non-Linear Data Structures
Non-linear data structures do not store data in a sequential manner. Instead, they allow hierarchical relationships between elements.
Trees
Trees are a type of non-linear data structure that consist of nodes connected in a parent-child relationship. A binary tree has a maximum of two children for each parent. The structure can be represented in C++ as:
struct TreeNode {
int value;
TreeNode* left;
TreeNode* right;
};
Graphs
Graphs are collections of nodes (vertices) connected by edges. They can be represented in two primary ways in C++:
- Adjacency Matrix: A 2D array to represent connections.
- Adjacency List: A more space-efficient method using lists for each vertex.
Choosing the Right Data Structure
Choosing the right data structure depends on various factors such as time complexity and space complexity. Understanding the characteristics of the data you are working with is essential.
For instance, if you frequently insert and delete data, a linked list may be more suitable than an array due to its dynamic size and efficient operations.

Core Data Structures in C++
Arrays
Arrays are one of the most basic data structures. They come with several characteristics: fixed size, indexed access, and storing elements of the same type.
In C++, you can create an array like this:
int myArray[5];
myArray[0] = 10; // Assigning values to the array
Linked Lists
Linked lists are versatile data structures. They consist of nodes with pointers to the next node, allowing dynamic memory allocation.
A simple insertion operation for a linked list can look like this:
void insert(Node*& head, int newData) {
Node* newNode = new Node();
newNode->data = newData;
newNode->next = head;
head = newNode;
}
Stacks
A stack is a linear data structure that follows the Last In, First Out (LIFO) principle. It is used in scenarios like backtracking and parsing expressions.
In C++, a stack can be implemented as:
class Stack {
int top;
int arr[100];
public:
Stack() { top = -1; }
void push(int x) {
if (top >= 99) return; // Check for overflow
arr[++top] = x;
}
int pop() {
if (top < 0) return -1; // Check for underflow
return arr[top--];
}
};
Queues
Queues operate on the First In, First Out (FIFO) principle. They are useful in scenarios like breadth-first search and scheduling tasks.
A basic queue implementation in C++ may look like this:
class Queue {
int front, rear;
int arr[100];
public:
Queue() : front(0), rear(0) {}
void enqueue(int x) {
if (rear >= 100) return; // Check for overflow
arr[rear++] = x;
}
int dequeue() {
if (front == rear) return -1; // Check for underflow
return arr[front++];
}
};
Trees
The binary search tree (BST) is a refined version of the binary tree that maintains sorted order. Inserting a value in the BST can be done as follows:
TreeNode* insert(TreeNode* root, int value) {
if (root == nullptr) {
return new TreeNode{value, nullptr, nullptr};
}
if (value < root->value) {
root->left = insert(root->left, value);
} else {
root->right = insert(root->right, value);
}
return root;
}
Graphs
Graphs, with their versatile structures, are essential for representing networks. Using an adjacency list for representation lets you efficiently store connections:
#include <vector>
class Graph {
int V; // Number of vertices
std::vector<std::vector<int>> adj;
public:
Graph(int v) : V(v) {
adj.resize(V);
}
void addEdge(int u, int v) {
adj[u].push_back(v);
adj[v].push_back(u); // for undirected graph
}
};

Algorithm Analysis
Importance of Algorithm Analysis
Analyzing algorithms is critical because it allows developers to make informed choices about the efficiency and suitability of their code. Performance optimization can lead to faster applications and better resource utilization.
Time Complexity
Big O notation is a mathematical representation that specifies the upper limit of an algorithm's runtime in relation to input size. Common time complexities include:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n^2): Quadratic time
Understanding these complexities is important for evaluating how an algorithm scales with increasing input sizes.
Space Complexity
While time complexity refers to the time an algorithm takes, space complexity measures the amount of memory required. This can drastically impact performance, especially in environments with limited resources.
For example, a recursive algorithm may use more space due to the function call stack, impacting its overall memory footprint.
Analyzing Algorithms in C++
Efficient analysis starts with measuring your algorithm's performance. Tools like Valgrind and gprof can help identify memory usage and bottlenecks. These analyses lead to better-optimized code.
Case Studies
To understand algorithm efficiency better, consider reviewing various C++ algorithms—such as sorting or searching algorithms—and their analyses based on time and space complexity. These case studies highlight practical implications and help you become adept in making the right choices when it comes to data structures and algorithm deployment.
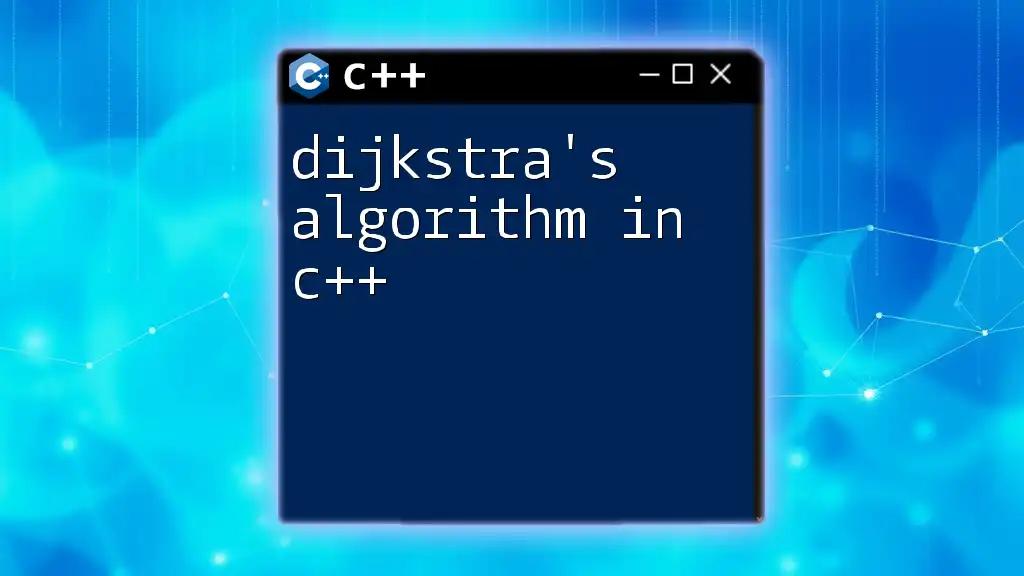
Conclusion
Summary of Key Points
Through this comprehensive guide on data structures and algorithm analysis in C++, we covered the fundamental concepts, types of data structures, and the importance of algorithm analysis in improving application performance. Understanding these principles is essential for every C++ programmer.
Practical Steps for Learning
As you start learning, focus on the practical application of these concepts. Implement different data structures and analyze their performance in real-world scenarios. Online resources, communities, and courses provide excellent platforms for exploration and growth.
Final Thoughts
Mastering data structures and algorithms is an ongoing journey. Consistent practice, problem-solving, and analysis will equip you to handle more complex tasks efficiently in C++.

Further Reading and Resources
For further exploration, consider delving into recommended books, online courses, and programming communities that focus on data structures and algorithm analysis in C++.