In C++, the primary difference between a structure (`struct`) and a class (`class`) is that members of a structure default to public access, while members of a class default to private access.
Here’s a code snippet demonstrating this difference:
#include <iostream>
struct MyStruct {
int a; // default public
};
class MyClass {
int a; // default private
public:
MyClass(int val) : a(val) {}
void show() { std::cout << "Class value: " << a << std::endl; }
};
int main() {
MyStruct s;
s.a = 10; // Accessible directly
std::cout << "Struct value: " << s.a << std::endl;
MyClass c(20);
c.show(); // Access through public method
return 0;
}
What is a Class?
Definition of a Class
A class in C++ is a foundational concept in Object-Oriented Programming (OOP) that acts as a blueprint for creating objects. It encapsulates data (attributes) and functions (methods or behaviors) into a single entity, allowing for data abstraction and organization. Classes enable you to model real-world entities, making it easier to manage complex software systems.
Syntax of a Class
Defining a class involves using the `class` keyword followed by the class name and a pair of braces that enclose its members (attributes and methods). Here's a basic example:
class Example {
public:
int value;
void display() {
std::cout << "Value: " << value << std::endl;
}
};
In this example, the class `Example` contains a public integer member `value` and a public method `display()` that outputs the value.
Access Modifiers in Classes
Access modifiers control the visibility and accessibility of class members. C++ supports three types of access specifiers:
- Public: Members are accessible from anywhere in the program.
- Private: Members are accessible only within the class.
- Protected: Members are accessible within the class and by derived classes.
Here’s an example demonstrating access modifiers:
class Example {
private:
int secretValue; // Accessible only within Example
public:
void setSecret(int val) {
secretValue = val; // Public method can access private member
}
int getSecret() {
return secretValue; // Public access for retrieval
}
};
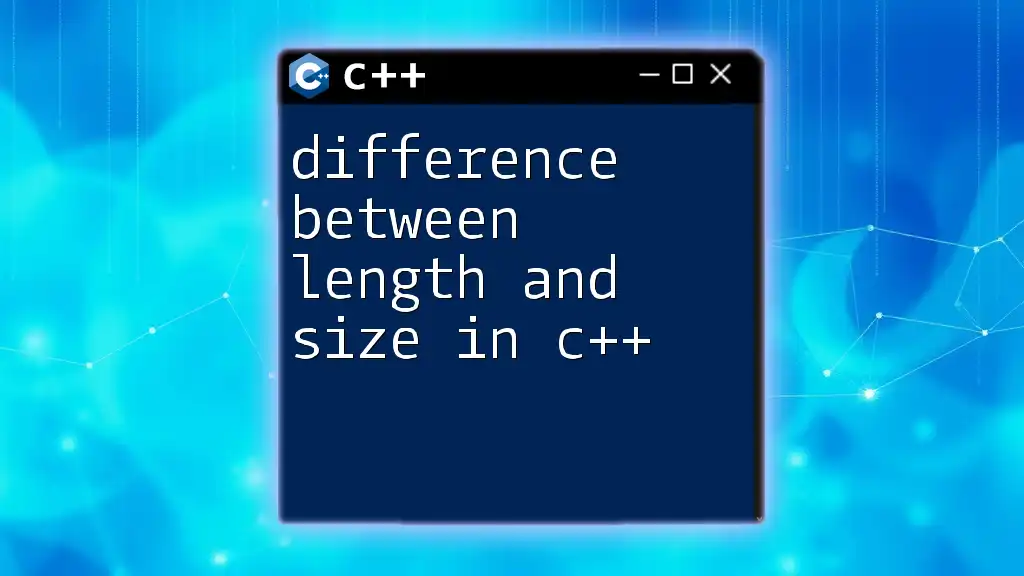
What is a Structure?
Definition of a Struct
A struct in C++ is similar to a class but tends to be simpler and traditionally used for grouping related data. Structs originated in the C language and have become more powerful in C++, allowing member functions and even inheritance.
Syntax of a Structure
Defining a struct follows a syntax similar to a class, using the `struct` keyword:
struct Example {
int value;
void display() {
std::cout << "Value: " << value << std::endl;
}
};
In this case, the struct `Example` contains an integer member `value` and a method `display()` that behaves just like the method found in our class example.
Default Access Modifiers in Structs
Unlike classes, members of a struct are public by default. This means that if no specific access modifier is declared, members of the struct are accessible from outside of it:
struct Example {
int value; // public by default
};
This highlights a key difference when using structs; simplicity in access control.
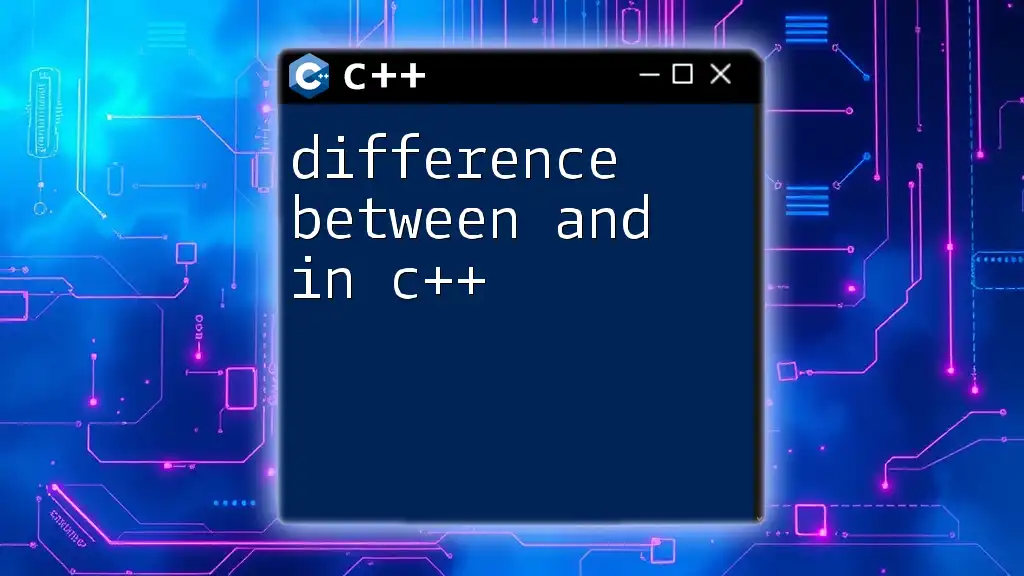
Class vs Struct in C++
Key Differences Between Class and Struct
Default Access Specifiers
The most significant distinction between classes and structs lies in their default access levels. In a class, members default to private access, while in a struct, they default to public. This difference influences how encapsulation is handled in your code.
Inheritance
Inheritance in C++ also differs between classes and structs. By default, inheritance from a class is private, while inheritance from a struct is public. This can impact how derived classes access base class members.
Here’s an illustrative example:
class Base {
};
class Derived : protected Base {
// Derived is protected from Base
};
struct BaseStruct {
};
struct DerivedStruct : public BaseStruct {
// DerivedStruct is public from BaseStruct
};
This showcases how struct inheritance can promote accessibility, which is essential to maintain in certain designs.
Member Functions
Both classes and structs can have member functions, allowing them to not just store data but also define behaviors. This feature enhances the capabilities of both constructs and allows for polymorphism within OOP.
class ClassExample {
public:
void classFunc() {
std::cout << "Method in a class." << std::endl;
}
};
struct StructExample {
void structFunc() {
std::cout << "Method in a struct." << std::endl;
}
};
This flexibility brings functionality to C++ structures, making them more akin to classes than they were in C.
When to Use Class or Struct
Choosing between a class and a struct depends on context:
- Use a class when you need to encapsulate data and behavior tightly, enforce access control, and benefit from OOP principles.
- Use a struct when you want a simple data aggregation or management and do not require the extensive features of a class, or when you prefer the default public access.
Structs are often utilized for representing simple data constructs like Point, Color, or a record, while classes are more suited for objects that require more comprehensive behavior management.
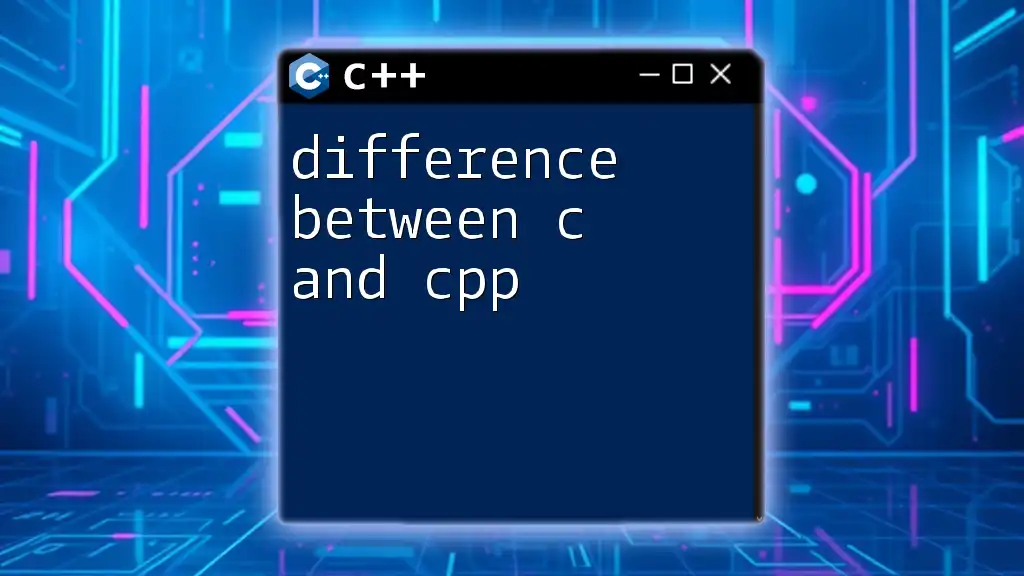
Conclusion
In summary, understanding the difference between structure and class in C++ is essential for anyone looking to grasp the nuances of C++ programming. While structs are primarily designed for simple data storage, classes provide a mechanism for more complex encapsulation of data and functionality. Recognizing these differences and knowing when to apply each will greatly enhance your programming skills and the design of your C++ applications.
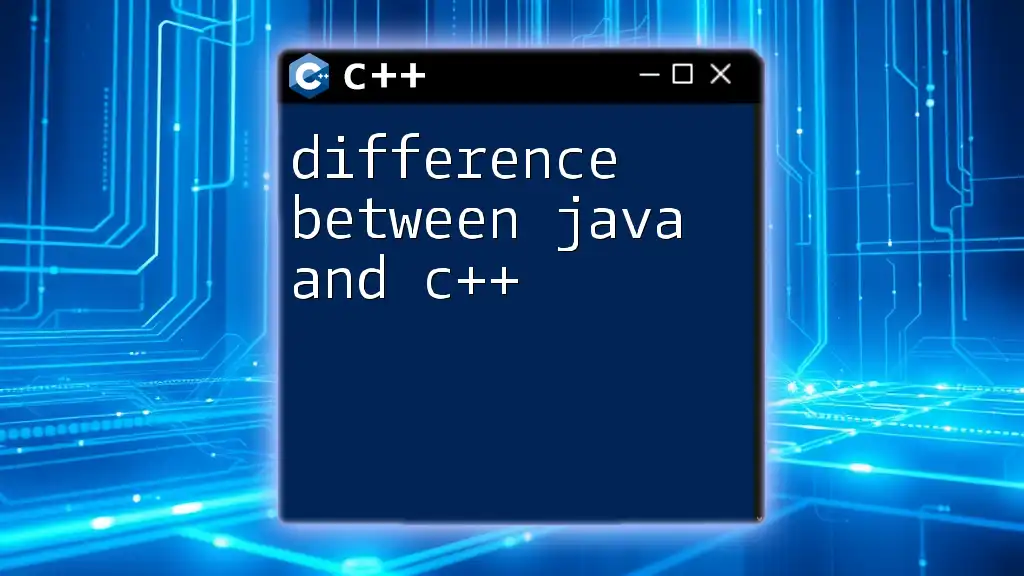
Additional Resources
For further reading, consult C++ documentation on OOP principles and explore recommended tutorials and books. Delving deeper into these topics will solidify your understanding and use of classes and structs in C++.
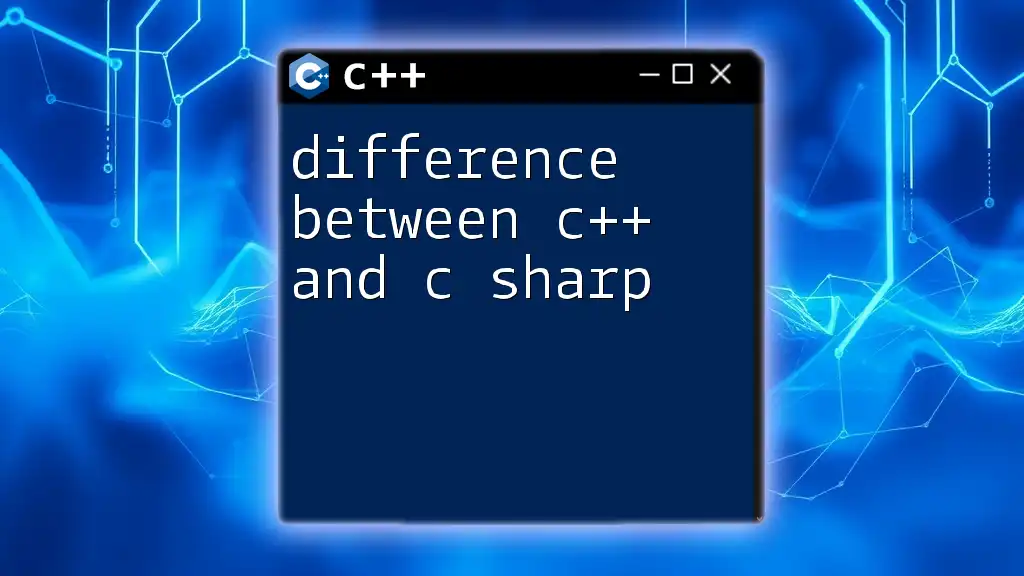
FAQs
-
Can a struct have private members? Yes, structs can have private members if you specify the access modifier.
-
Is it possible to inherit from a struct? Absolutely, structs can be inherited just like classes.
-
When should I use a struct over a class? Prefer structs for simple data collections, and utilize classes for more complex data and behavior encapsulation.