Java is a high-level, object-oriented programming language designed for portability across platforms, while C++ is a middle-level language that combines high-level features with low-level programming capabilities, allowing for direct memory management.
Here’s a simple example demonstrating the syntax for defining a class in both languages:
Java Example
class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
C++ Example
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Historical Background
Origin of Java
Java was created by Sun Microsystems in the mid-1990s with the goal of providing a programming language that was both platform-independent and easy to use. The core philosophy behind Java was to enable developers to write code once and run it anywhere, thanks to its use of the Java Virtual Machine (JVM). This revolutionary idea transformed the way software applications were developed, subsequently leading to its widespread adoption in various domains, particularly in web and mobile applications.
Origin of C++
On the other hand, C++ was developed by Bjarne Stroustrup in the late 1970s, with its roots deeply embedded in the C programming language. Stroustrup aimed to enhance C by adding object-oriented features while maintaining the efficiency and flexibility of procedural programming. As a result, C++ became a hybrid programming language that accommodates both procedural and object-oriented programming paradigms.
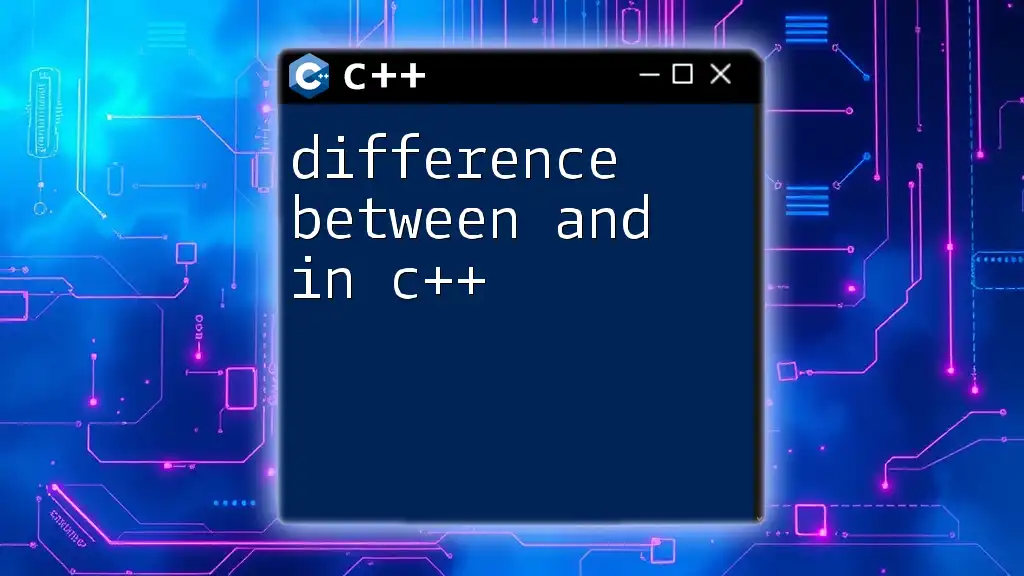
Basic Philosophy
Object-Oriented vs. Hybrid Model
Java is considered a purely object-oriented programming language, which means everything in Java revolves around the concept of classes and objects. This design philosophy is aimed at enabling encapsulation, inheritance, and polymorphism.
Example: A simple class definition in Java.
class Car {
String color;
void drive() {
System.out.println("Car is driving");
}
}
In contrast, C++ implements a hybrid model, allowing developers to utilize both procedural and object-oriented programming. This flexibility enables more control over system resources, making C++ suitable for applications where performance and resource management are critical.
Example: A simple class definition in C++.
class Car {
public:
string color;
void drive() {
cout << "Car is driving" << endl;
}
};
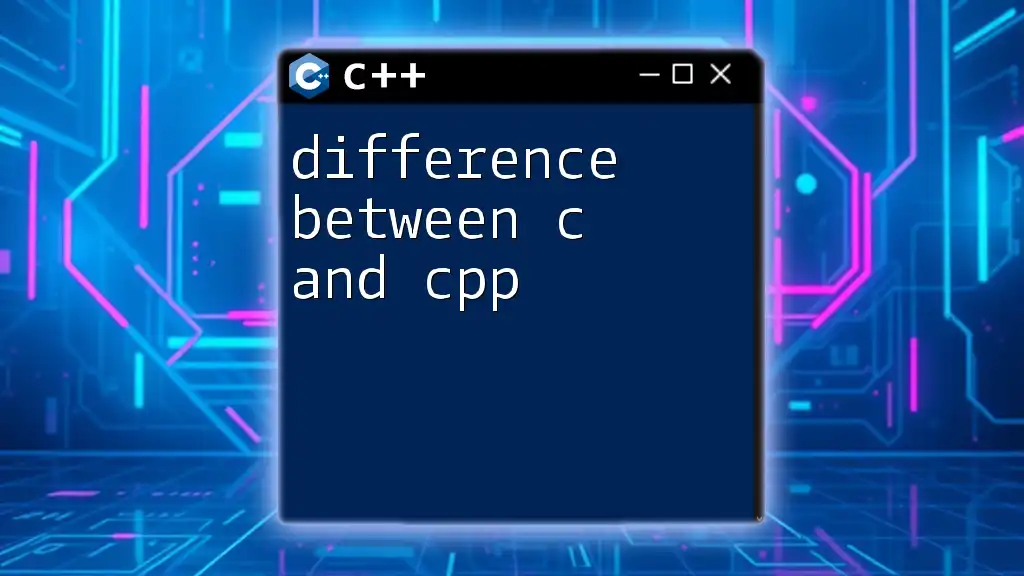
Syntax and Structure
Code Structure Differences
When it comes to code structure, Java enforces a strict object-oriented approach. This leads to all Java code being encapsulated within classes and characterized by the use of interfaces. C++, however, allows for both class-based and procedural styles, giving developers more leeway to decide how to structure their code.
Control Structures
Both Java and C++ share various control structures like if-else statements, loops (for, while), and switch cases, but their syntax can differ slightly.
For instance, in Java:
for (int i = 0; i < 10; i++) {
System.out.println(i);
}
In C++:
for (int i = 0; i < 10; i++) {
cout << i << endl;
}
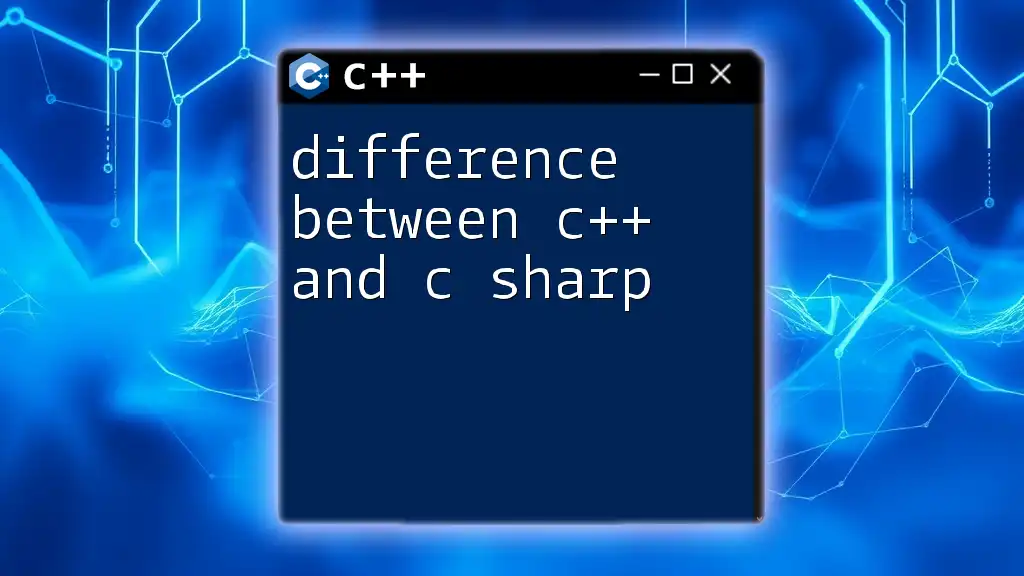
Memory Management
Garbage Collection vs Manual Management
One of the most significant differences between Java and C++ is their approach to memory management. Java incorporates automatic garbage collection, which means that the Java Virtual Machine (JVM) handles memory allocation and deallocation. This simplifies the development process by reducing the risk of memory leaks.
In contrast, C++ requires developers to manage memory manually using new and delete operators. While this grants developers more control, it also increases the complexity and potential for errors.
Example: Memory Allocation in Java vs. C++.
// Java
Car myCar = new Car();
// C++
Car* myCar = new Car();
delete myCar;
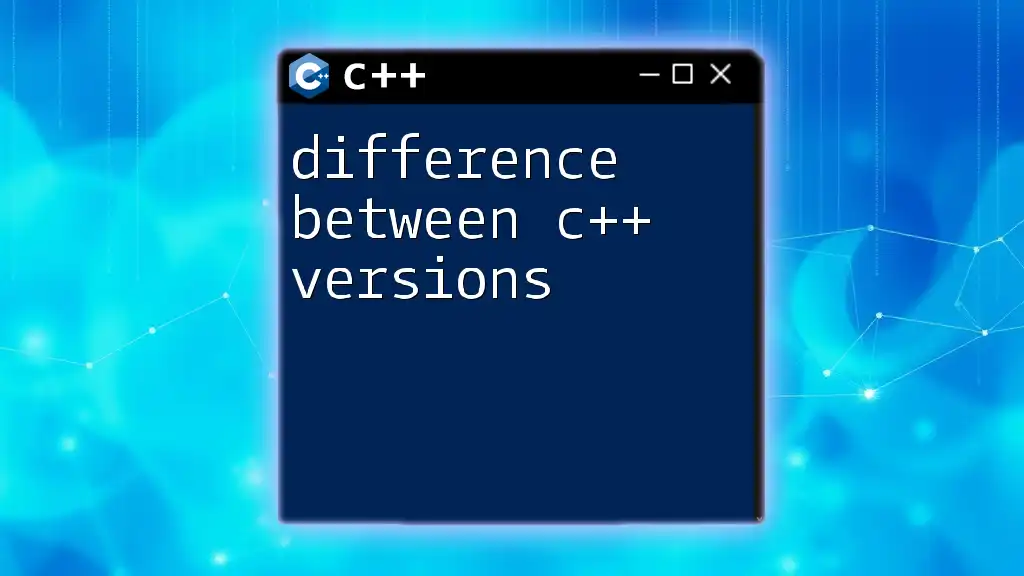
Performance
Runtime Performance
Performance-wise, Java and C++ exhibit significant differences. Java uses Just-In-Time (JIT) compilation, which compiles bytecode into native machine code at runtime. This process can result in slower performance compared to C++, which is compiled directly into native code before execution. Consequently, applications built in C++ are generally more efficient.
Example: Performance Comparison
While specific benchmarks may vary with updates and optimizations, numerous studies indicate that C++ applications possess lower memory overhead and execute faster than comparable Java applications. It's vital for developers to assess the performance requirements of their projects when deciding between these two languages.
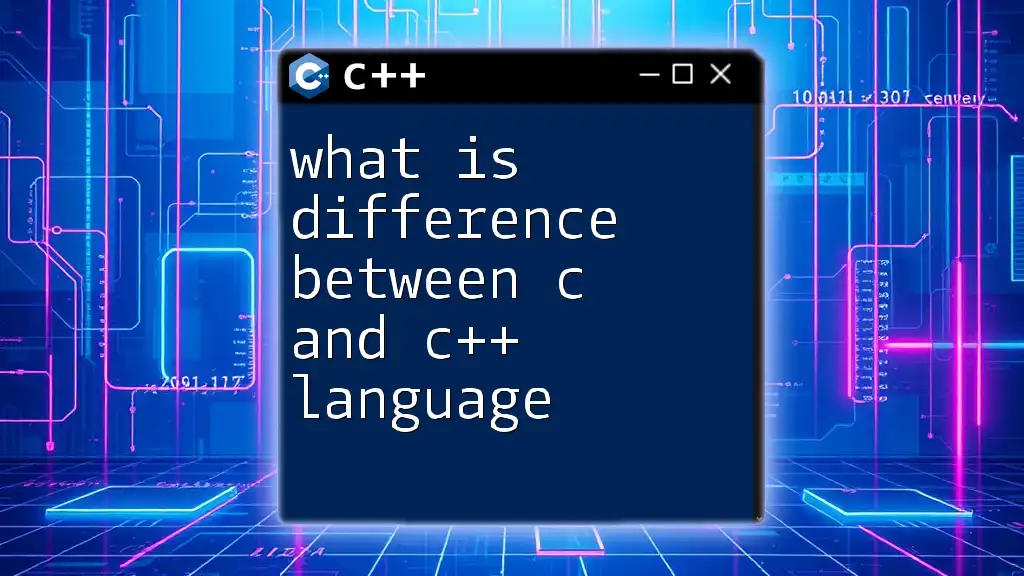
Platform Independence
Write Once, Run Anywhere (WORA)
Java adheres to the "Write Once, Run Anywhere" (WORA) philosophy. This is achievable through the JVM, which creates a layer of abstraction between the program and the underlying operating system, allowing Java applications to run on any device that has a JVM installed.
C++: Platform Specificity
In contrast, C++ is inherently platform-dependent, necessitating recompilation on different systems. While this means that C++ can exploit specific hardware benefits, it also introduces challenges concerning code portability.
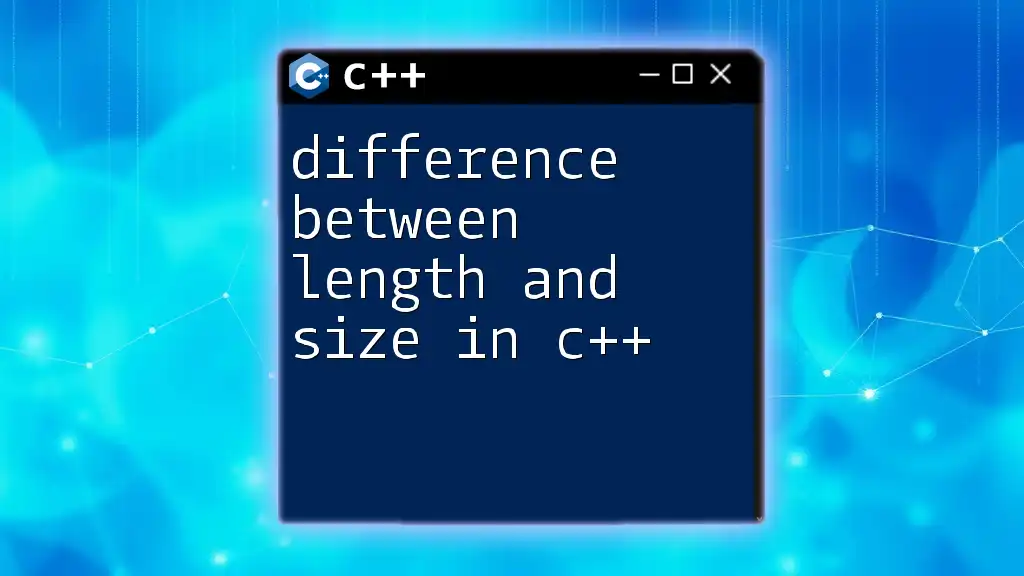
Standard Libraries
Libraries and Frameworks
Both languages boast extensive standard libraries, yet their focuses differ. Java features a rich ecosystem with libraries like the Java Standard Library and frameworks such as Spring and Hibernate, aimed primarily at enterprise-level application development and web services.
C++, on the other hand, leverages powerful libraries such as the Standard Template Library (STL) and Boost, which provide data structures and algorithms that enhance the language’s performance capabilities.
Comparison of Features
When comparing their features, Java's libraries focus on ease-of-use and a broad array of functionalities, while C++ libraries often emphasize performance and lower-level control.
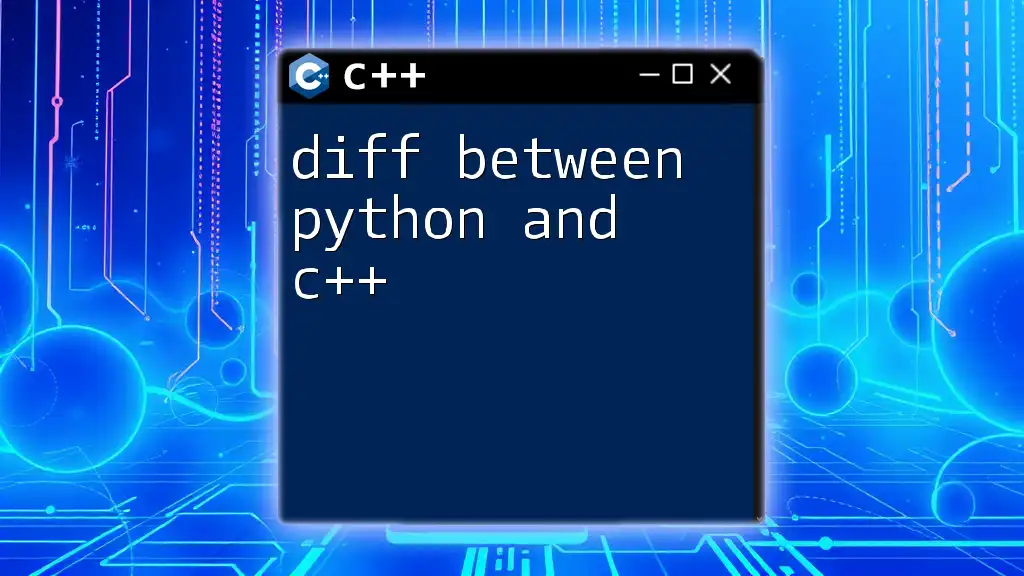
Exception Handling
Approach to Handling Exceptions
Java employs a distinct methodology in its exception handling mechanism by categorizing exceptions into checked and unchecked exceptions. This enforces stricter compile-time checks, compelling developers to manage potential exceptions proactively.
Example: Exception handling in Java.
try {
// code that might throw an exception
} catch (ExceptionType e) {
// handle exception
}
In C++, the exception-handling mechanism is less structured, relying on simple try-catch blocks without the distinction between checked and unchecked exceptions.
Example: Exception handling in C++.
try {
// code that might throw an exception
} catch (ExceptionType& e) {
// handle exception
}
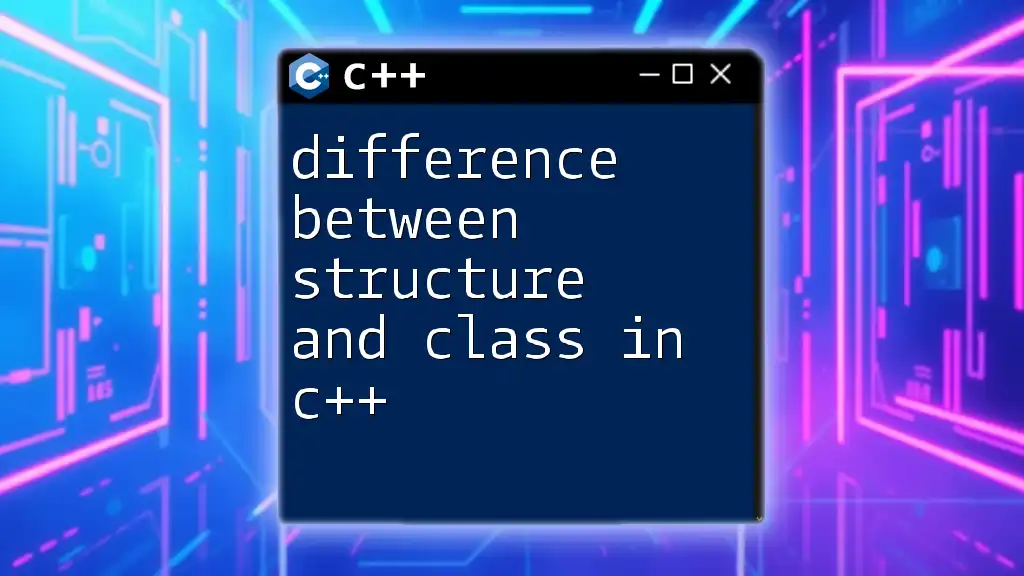
Use Cases and Applications
Typical Use Cases for C++
C++ has become the language of choice for specific domains where performance is paramount. It's widely used in:
- Game Development: C++ provides high efficiency required for real-time graphics and resource management.
- Systems Programming: Operating systems, drivers, and applications that require low-level memory management are typically written in C++.
Typical Use Cases for Java
Java is commonly found in:
- Web Development: With widespread frameworks, Java excels in building robust web applications.
- Mobile Applications: Java is the primary language for Android development, which prevails in the mobile sector.
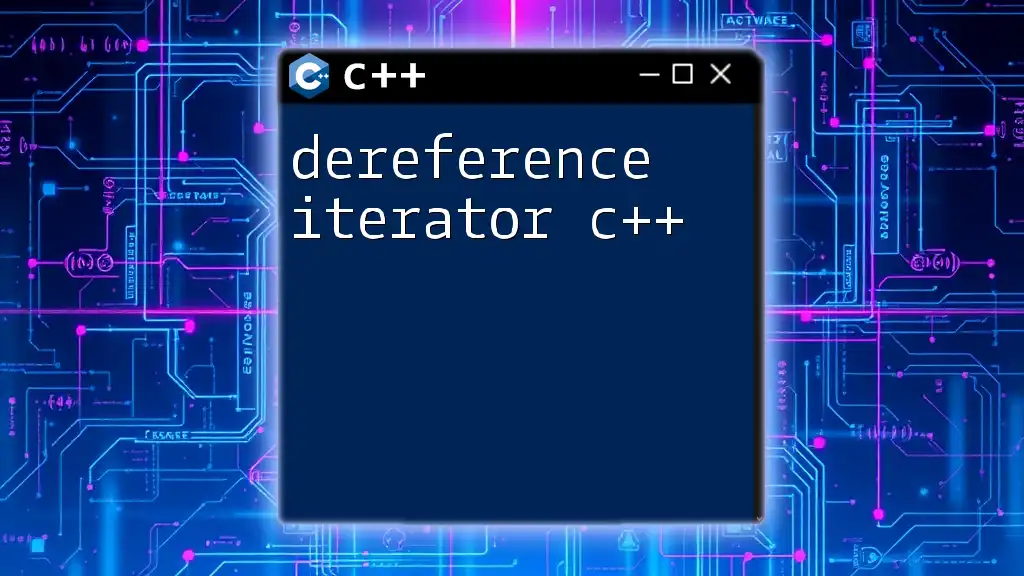
Conclusion
In summary, understanding the key difference between Java and C++ is crucial for developers when selecting the most appropriate language for their projects. While Java offers platform independence and automatic memory management, C++ provides superior performance and flexibility in resource management. Each language has its strengths and weaknesses, and the choice ultimately depends on the project requirements, performance considerations, and development preferences. As you delve into the world of programming, consider leveraging your knowledge of both languages for a holistic approach to application development.