C and C++ are distinct programming languages, where C is a procedural language, while C++ is an extension of C that incorporates object-oriented programming features.
Here’s a simple code snippet demonstrating a basic concept in both languages:
// C code example
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
// C++ code example
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Basics
What is C?
C is a general-purpose programming language that was developed in the early 1970s by Dennis Ritchie at Bell Labs. It’s known for its efficiency and control over system resources, which has made it a staple in systems programming, operating systems, and other resource-constrained applications.
Core Features of C:
- C is primarily a procedural programming language, focusing on functions and the sequence of instructions executed.
- It has a rich set of data types, including primitive types like integers and characters, and allows the creation of structures for complex data handling.
- C relies heavily on control structures like loops and conditionals to dictate the flow of execution.
What is C++?
C++ was developed in the late 1970s as an extension of C. Bjarne Stroustrup aimed to incorporate object-oriented features into C, making it more suitable for larger applications.
Core Features of C++:
- C++ supports object-oriented programming (OOP), introducing concepts such as classes, objects, inheritance, and polymorphism.
- C++ allows for template programming, enabling functions and classes to operate with any data type.
- It includes improved mechanisms for error handling through exceptions, enhancing robustness in complex applications.
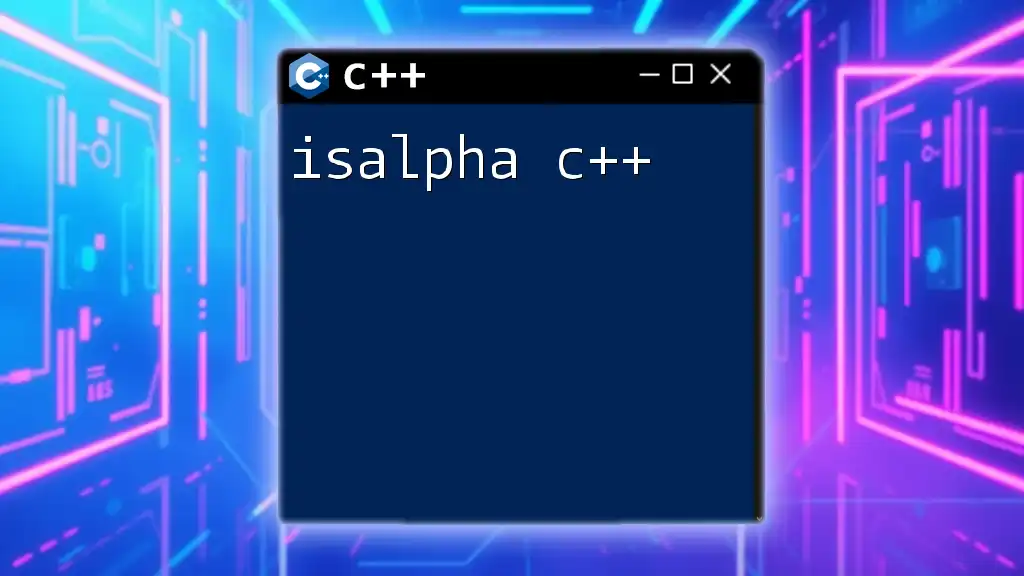
Key Differences Between C and C++
Programming Paradigms
One of the foundational differences between C and C++ lies in their programming paradigms.
Procedural vs. Object-Oriented:
- C is inherently procedural, designed around the idea of using functions to manipulate data. This involves a linear flow of execution, which can be limiting for larger or more complex programs.
- In contrast, C++ facilitates object-oriented programming, which encapsulates data with methods, allowing for clearer and more modularized code. By utilizing classes, developers can create blueprints that can be reused with variations.
Syntax Variations
While both languages share a similar syntax, there are notable differences.
Function Definition Differences: For instance, defining a function in both languages shares similarities, but C++ allows for function overloading, enabling multiple functions with the same name but different parameters.
// C Function
int sum(int a, int b) {
return a + b;
}
// C++ Function with Overloading
int sum(int a, int b) {
return a + b;
}
double sum(double a, double b) {
return a + b;
}
Memory Management
Memory management is another area where C and C++ diverge significantly.
Manual vs. Automatic:
- In C, memory allocations are handled through `malloc` and deallocations with `free`, placing the onus on the developer to manage memory efficiently and safely.
int* arr = (int*)malloc(10 * sizeof(int));
// Use arr...
free(arr);
- C++, however, introduces `new` and `delete`, which streamlines memory management by providing a clearer perspective on resource allocation and deallocation.
int* arr = new int[10];
// Use arr...
delete[] arr;
Standard Libraries
Both languages provide a robust set of standard libraries, but they differ in composition and functionality.
Library Availability and Use:
-
C features the Standard C Library, crucial for file operations, string handling, and mathematical functions.
-
C++ builds upon this foundation with the Standard Template Library (STL), which introduces data structures like vectors, lists, and algorithms for efficient data manipulation.
#include <vector>
#include <iostream>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
for (int i : nums) {
std::cout << i << " ";
}
return 0;
}
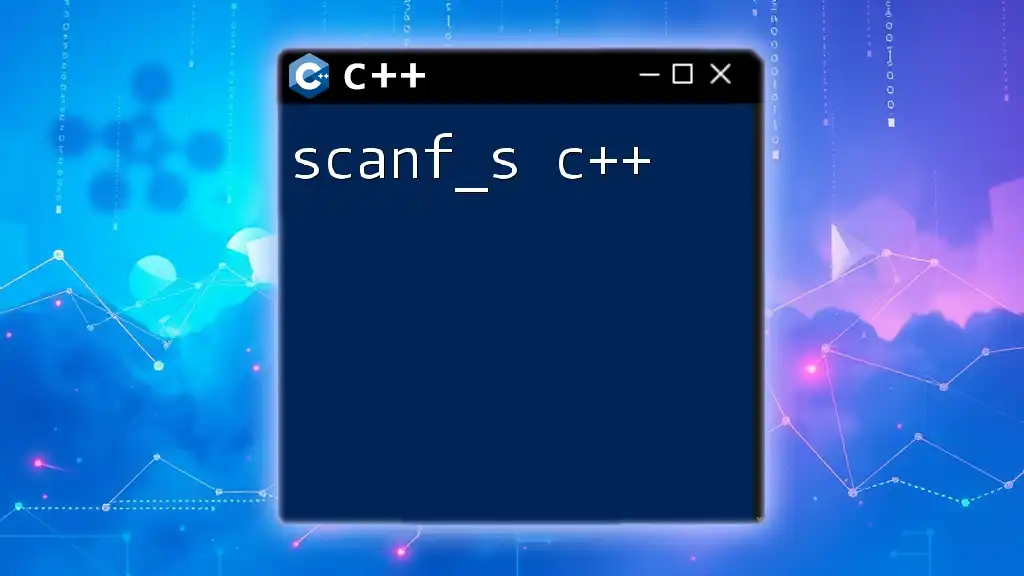
Similarities Between C and C++
Shared Foundation
Despite their differences, C and C++ share many foundational aspects that make them relatable.
Common Syntax and Structure: Both languages utilize similar keywords, operators, and basic control structures, such as loops and conditionals, making it easier for developers to transition between them.
Performance and Efficiency
Both C and C++ are high-performance, compiled languages, which makes them ideal for applications where speed and memory efficiency are paramount. This shared characteristic allows them to excel in system-level programming and resource-constrained environments.
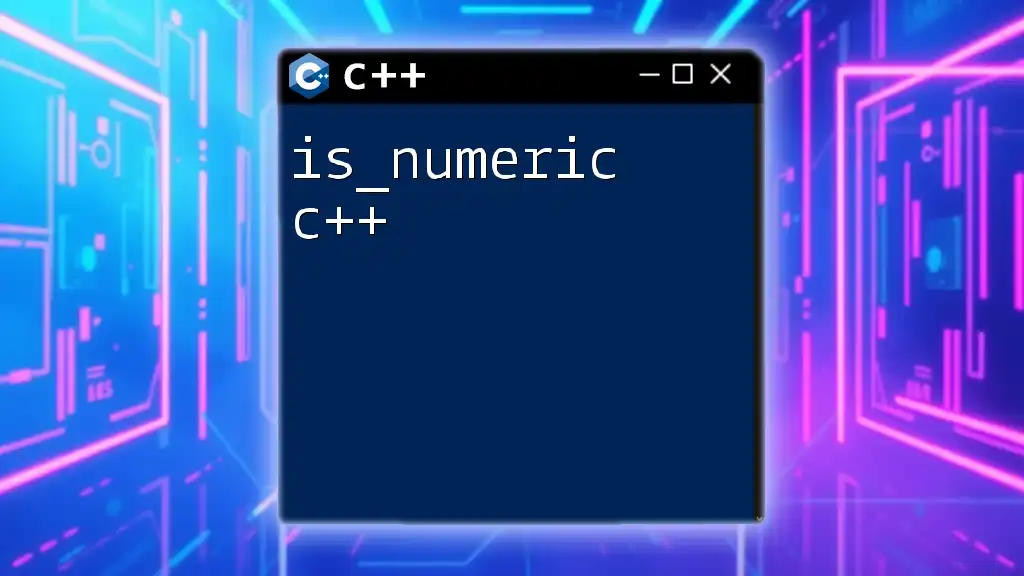
Practical Applications of C vs. C++
When to Use C
C shines in scenarios such as system programming and embedded systems due to its close-to-hardware capabilities and minimal runtime overhead. For instance, operating systems, firmware, and device drivers are commonly written in C.
When to Use C++
On the other hand, C++ is typically favored in environments requiring complex data handling and abstraction, such as game development, GUI application development, and large-scale enterprise applications. Its object-oriented features facilitate the development of scalable and manageable codebases.
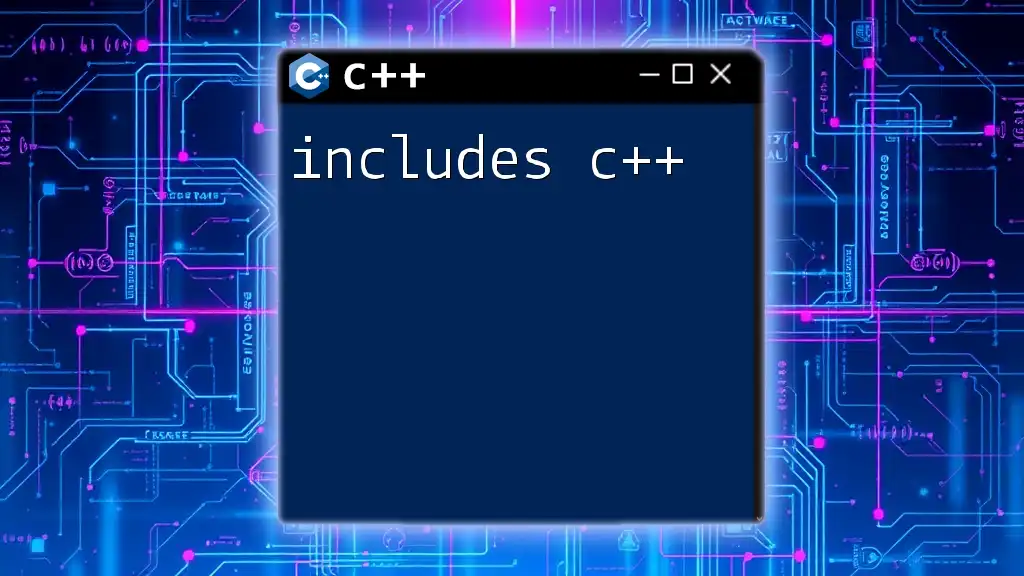
Transitioning from C to C++
Learning Curve
Transitioning from C to C++ can present challenges, particularly as programmers must become comfortable with OOP concepts and structures.
Understanding OOP Concepts:
- C++ introduces the idea of encapsulation, making it crucial for developers to embrace concepts like classes and inheritance, which can differ significantly from procedural paradigms.
Tips for C Programmers
To ease the transition, C programmers should:
- Practice with small C++ projects that leverage OOP principles.
- Explore C++ tutorials focusing on object-oriented concepts and standard libraries.
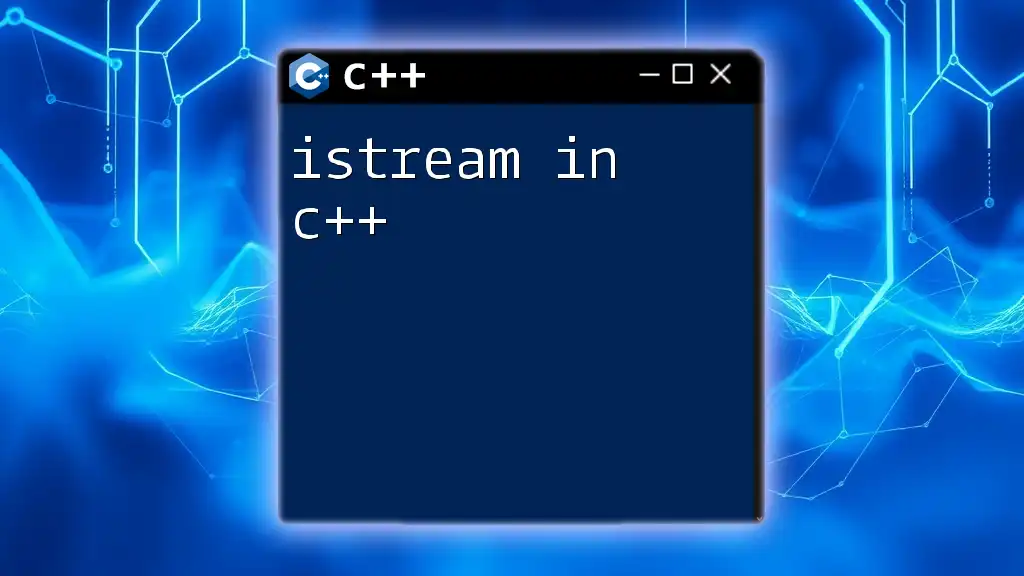
Conclusion
In summary, while C and C++ share a common ancestry and some syntactical similarities, they are fundamentally different in their programming paradigms, feature sets, and typical applications. C is primarily procedural, making it simple and efficient for low-level programming, while C++ expands on this foundation to incorporate powerful object-oriented features, making it a versatile language for building complex applications.
Understanding these distinctions can help programmers choose the right tool for the job, whether they are optimizing resource-intensive applications or designing modular and scalable software solutions.
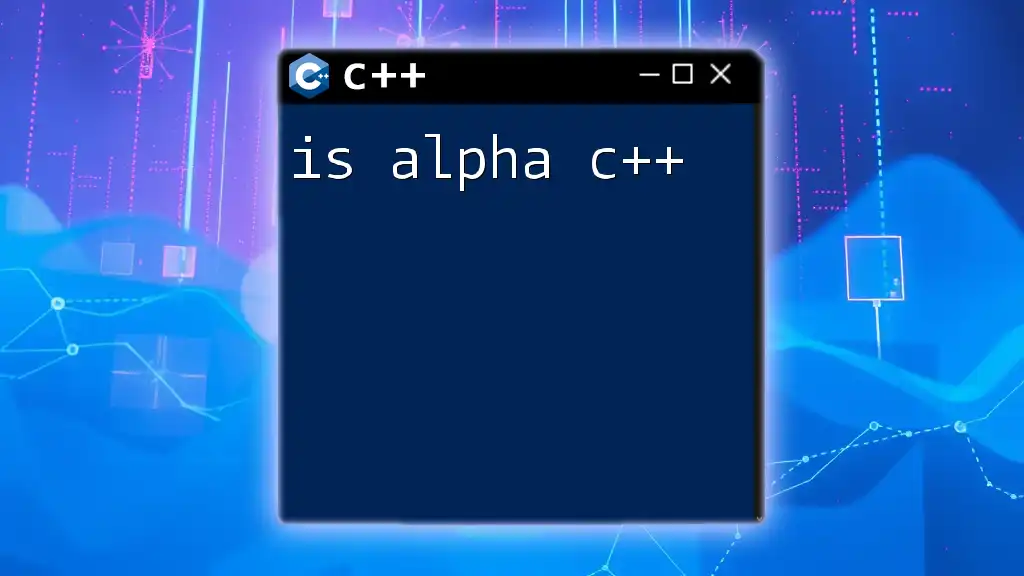
Additional Resources
For further exploration, consider delving into recommended books and online courses dedicated to both C and C++. Engaging with community forums and programming groups can also provide ongoing support as you navigate the nuances of these languages.