The primary difference between C and C++ is that C is a procedural programming language focused on functions and structured programming, while C++ is an object-oriented programming language that supports classes and objects, enabling more complex data management and code reusability.
Here's a simple code snippet demonstrating the difference in approach:
// C code: Procedure-oriented
#include <stdio.h>
void greet() {
printf("Hello from C!\n");
}
int main() {
greet();
return 0;
}
// C++ code: Object-oriented
#include <iostream>
using namespace std;
class Greeter {
public:
void greet() {
cout << "Hello from C++!" << endl;
}
};
int main() {
Greeter greeter;
greeter.greet();
return 0;
}
Overview of C Language
What is C?
C is a high-level programming language developed in the early 1970s by Dennis Ritchie at Bell Labs. It is known for its efficiency, close-to-hardware capabilities, and foundational role in many programming languages. C is characterized by its low-level access to memory and straightforward procedural programming approach. These features make it a preferred choice for system programming, embedded systems, and creating operating systems.
General Characteristics of C
- Procedural Language: C follows a top-down approach and emphasizes functions and procedure calls.
- Static Typing: The data types in C must be defined at compile time, which helps optimize memory usage.
- Low-Level Memory Control: Developers have direct access to memory through pointers, allowing for fine control over system resources.
Key Features of C
- Lightweight and efficient, making it suitable for resource-constrained environments.
- A rich set of built-in functions and operators.
- A modular approach to code organization through functions.
- Ability to interact with assembly language for even lower-level programming tasks.
Code Example in C
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
This simple code snippet demonstrates the basic structure of a C program. It includes the standard input-output library and displays "Hello, World!" in the console.
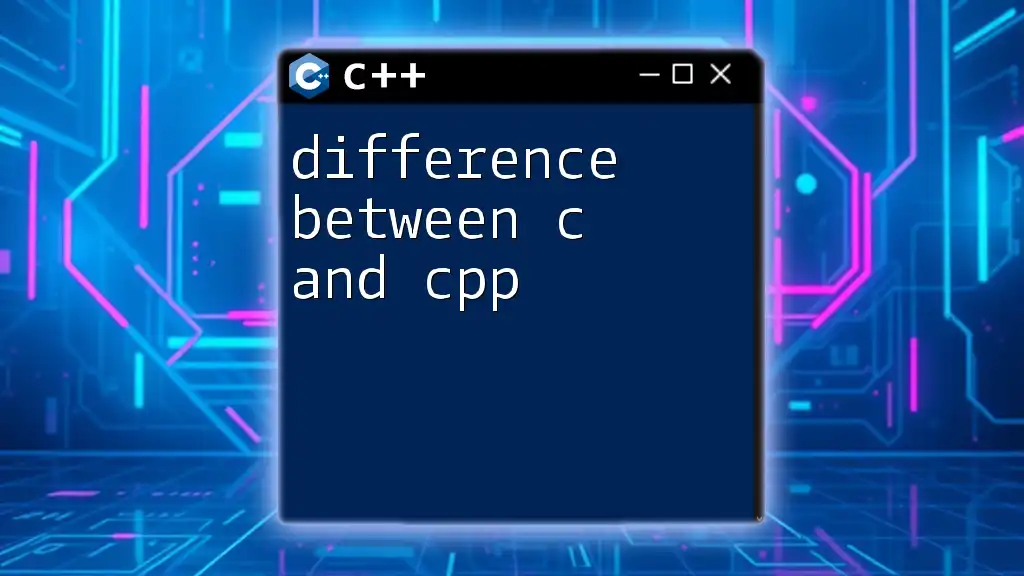
Overview of C++ Language
What is C++?
C++ is an extension of the C programming language, developed by Bjarne Stroustrup in the early 1980s. It incorporates object-oriented programming (OOP) principles, enabling more sophisticated software development. C++ maintains the efficiency of C while adding features that support encapsulation, inheritance, and polymorphism.
General Characteristics of C++
- Object-Oriented Language: C++ thrives on concepts like classes and objects, making it easier to design complex systems and manage data.
- Strongly Typed: Like C, C++ requires type definitions at compile time, ensuring that errors can be identified early.
- Standard Template Library (STL): C++ offers extensive libraries that provide ready-to-use data structures and algorithms, enhancing productivity.
Key Features of C++
- Supports both procedural and object-oriented programming paradigms.
- Provides features like operator overloading and multiple inheritance.
- Enhanced memory management with constructors and destructors, streamline resource management.
Code Example in C++
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This snippet illustrates how to display a message in C++. It utilizes `iostream` for input and output operations and demonstrates the syntax differences compared to C.
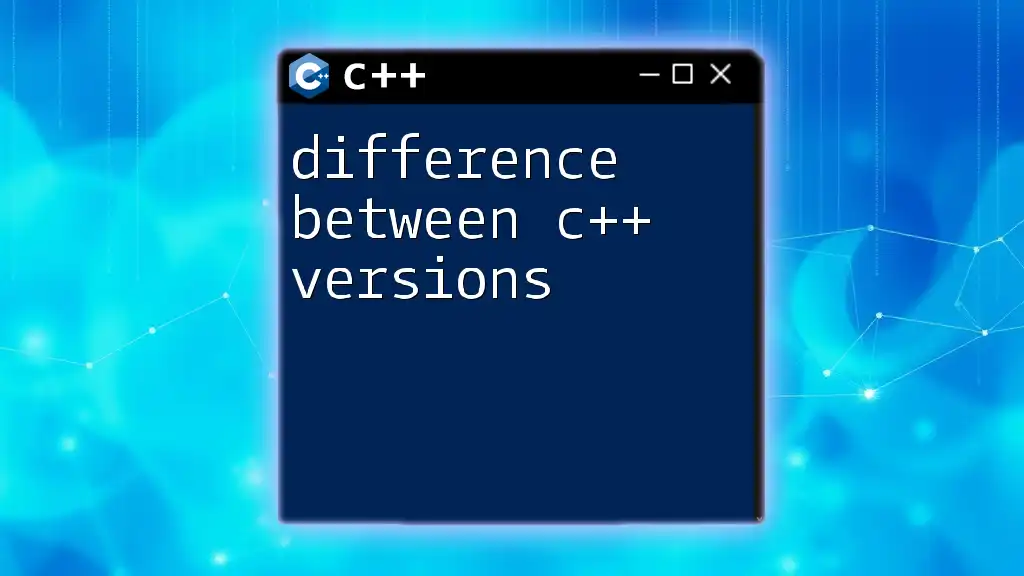
Fundamental Differences Between C and C++
Programming Paradigms
One of the most significant differences lies in their programming paradigms. C is primarily a procedural language where the focus is on functions and procedure calls. In contrast, C++ is recognized for its object-oriented programming (OOP) capabilities, introducing concepts such as classes, objects, encapsulation, inheritance, and polymorphism, which facilitate code reuse and organization.
Syntax and Structure
Syntax plays a crucial role in distinguishing between the two languages. While both share a similar syntax base, C++ expands on this:
-
C Syntax:
int add(int a, int b) { return a + b; }
-
C++ Syntax:
class Calculator { public: int add(int a, int b) { return a + b; } };
The above examples illustrate how C utilizes a procedural approach with standalone functions, while C++ employs classes that encapsulate data and functions, promoting modularity and data abstraction.
Memory Management
Memory management in C and C++ also showcases differences:
-
C relies heavily on manual memory management through the use of functions like `malloc` and `free`. Developers must keep a keen eye on memory allocation and deallocation to avoid memory leaks.
-
C++ introduces constructors and destructors, automating memory management to a degree. Constructors are called when an object is created, and destructors are executed when the object goes out of scope, streamlining resource cleanup.
Standard Libraries and Functionality
The libraries available in both languages also differ markedly:
-
C provides standard libraries primarily for I/O operations and string manipulation, while the functionality is often more limited.
-
C++ offers the Standard Template Library (STL), which includes pre-defined templates for data structures (like vectors, lists, and queues) and algorithms (like sorting and searching), significantly enhancing productivity and efficiency in software development.
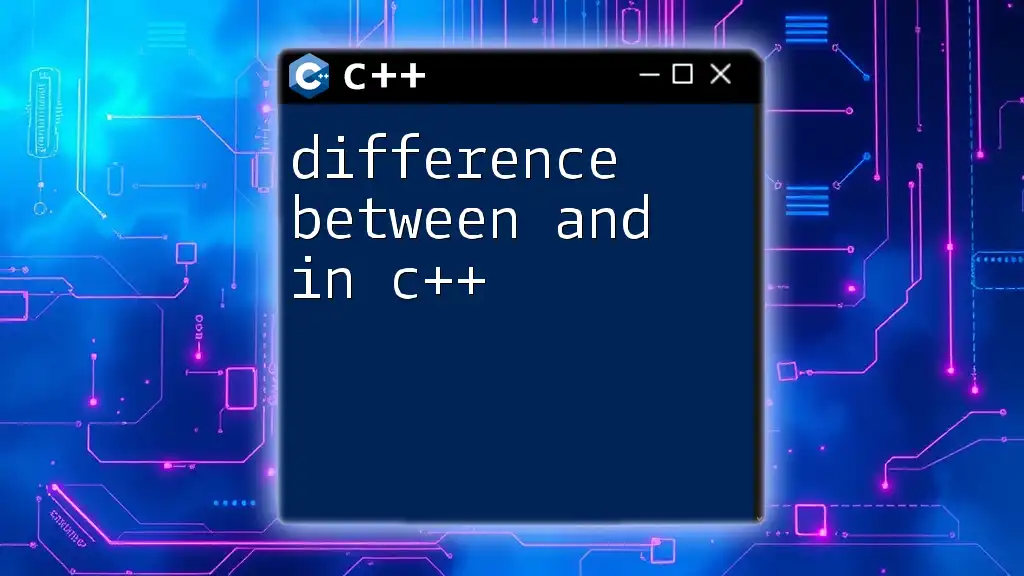
Performance Considerations
Execution Speed
In many cases, C offers faster execution speed due to its simpler semantics and lower-level nature. Programs written in C may have less overhead because they lack some of the complexities found in C++. However, with careful optimization, C++ can achieve comparable performance.
Resource Management
When it comes to resource management, the encapsulation capabilities of C++ can lead to cleaner and more efficient code. By using objects to manage resources, developers can reduce the risk of logical errors that might arise from manual memory management in C.
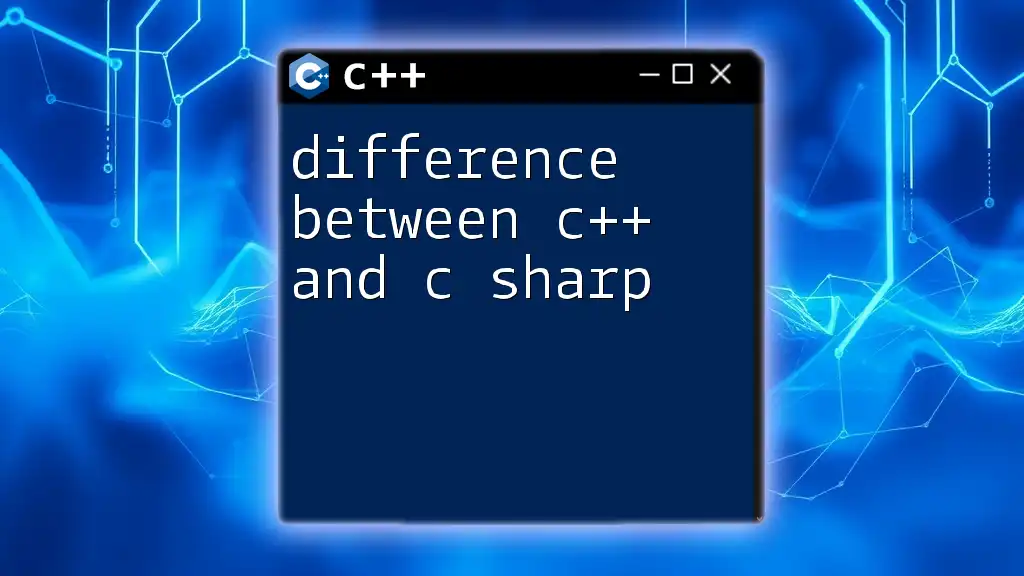
Application Areas
Where is C Used?
C is widely employed in sectors requiring high-performance computing, including:
- Embedded Systems: Used in microcontrollers and hardware devices.
- Operating System Development: Fundamental in building core parts of operating systems (e.g., Unix, Linux).
Where is C++ Used?
C++ is preferred in application domains that benefit from its OOP features such as:
- Game Development: Utilized in game engines for better resource management and graphics manipulation.
- GUI Applications: Due to its ability to manage complex user interfaces efficiently.
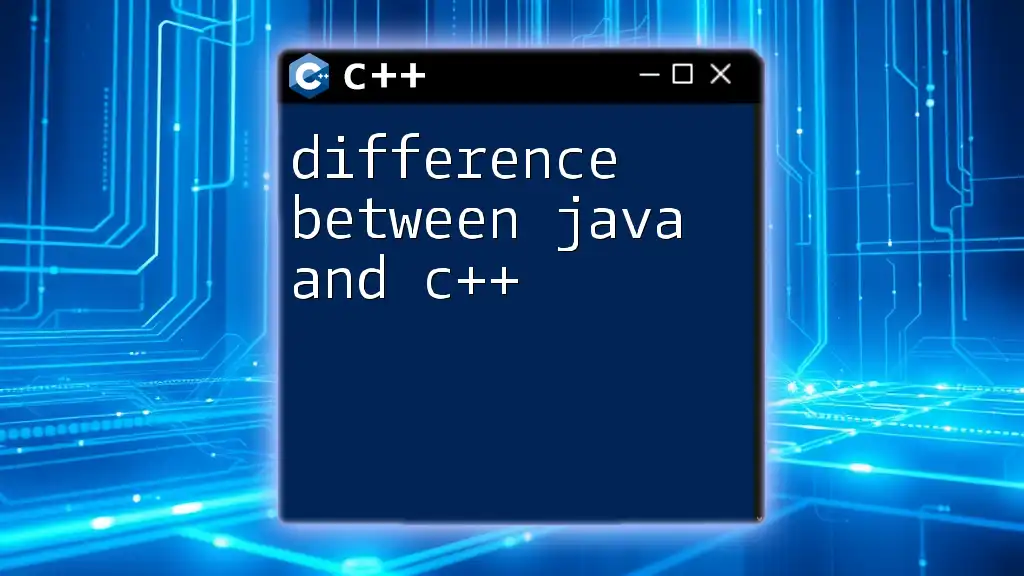
Conclusion
Understanding the difference between C and C++ language is crucial for aspiring programmers and developers. While C excels in low-level programming and system access, C++ builds upon these strengths by introducing powerful abstractions and object-oriented features. With applications in diverse areas, both languages are essential tools in a programmer’s repertoire.
As you explore the world of programming, consider diving deeper into these languages to discover their unique capabilities and features. Keep practicing and expanding your knowledge, and don’t hesitate to seek resources that can aid you in mastering both C and C++.
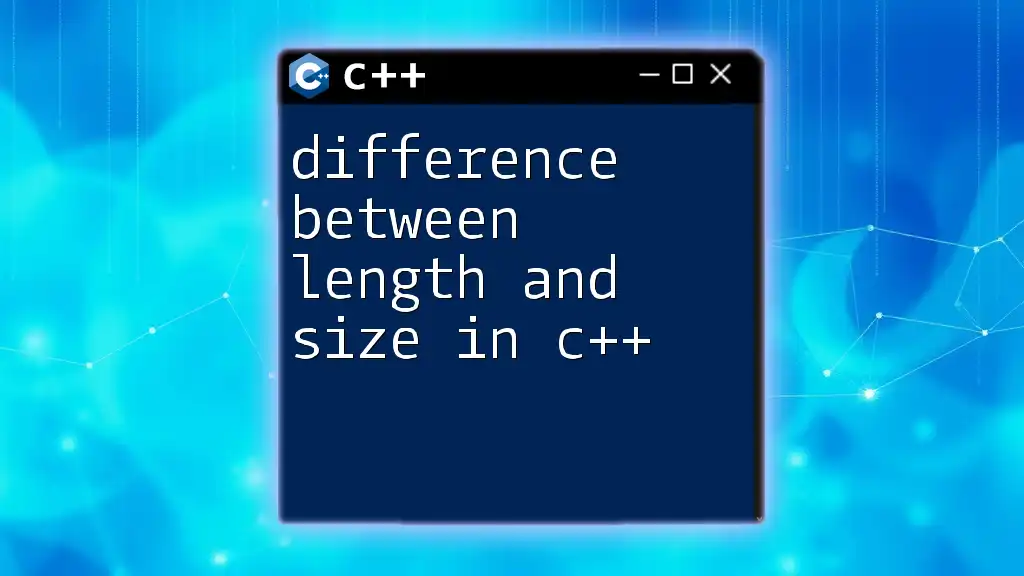
Call to Action
Join our C++ learning courses to enhance your programming skills further! For more information and resources, feel free to reach out to us. Together, let's unlock the full potential of programming!
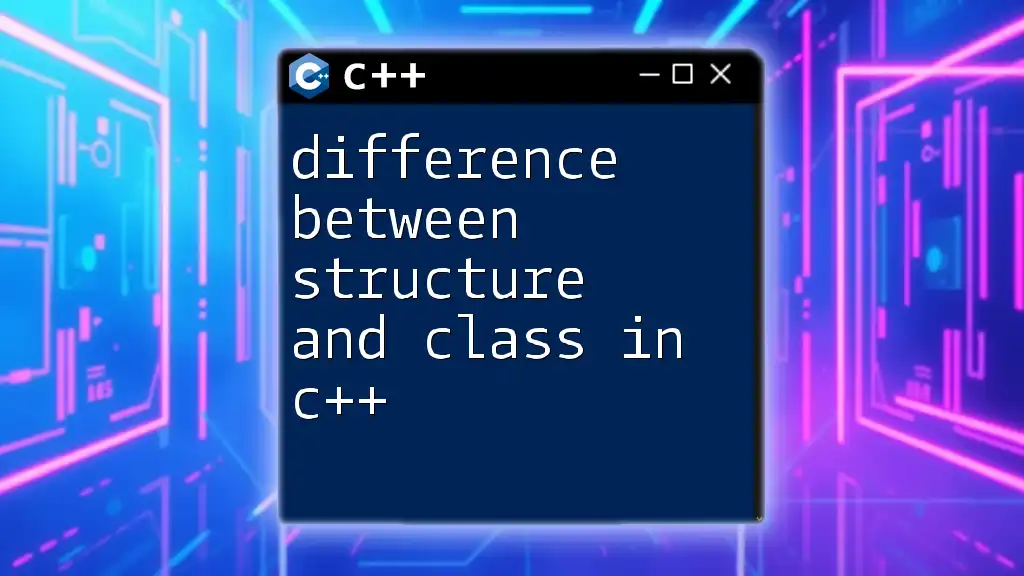
References
- List of relevant books and online resources for deeper investigation on C and C++.