To generate a random number between 0 and 1 in C++, you can use the `<random>` library to create a uniform distribution.
#include <iostream>
#include <random>
int main() {
std::random_device rd; // obtain a random number from hardware
std::mt19937 eng(rd()); // seed the generator
std::uniform_real_distribution<> distr(0.0, 1.0); // define the range
double random_number = distr(eng); // generate the random number
std::cout << random_number << std::endl; // output the random number
return 0;
}
Understanding Random Number Generation
What is a Random Number?
A random number is a value generated in such a way that it cannot be predicted. In computing, we typically deal with pseudo-random numbers, which are generated using algorithms to generate sequences that appear random. True randomness can be difficult (if not impossible) to achieve, especially in a digital environment where computations follow deterministic rules.
Importance of Random Numbers in Programming
Random numbers are crucial in various fields such as gaming, simulations, cryptography, and statistical sampling. In programming, randomness is often used to create unpredictability. For example, in a game, random number generation can influence the outcome of a player's win or loss, or it can determine the layout of levels. Hence, proper understanding and implementation of a C++ random number generator between 0 and 1 can greatly enhance the functionality and user experience of your applications.

Exploring C++ Random Number Generation
The Basics of C++ Random Number Generation
C++ provides a robust random number generation framework via the `<random>` header. This header includes random engines and distributions that allow developers to create pseudo-random numbers efficiently.
Key Components of the Random Number Generator
Random Engine
In C++, a random engine generates the sequence of numbers. The most commonly used engine is `std::default_random_engine`, which provides a well-rounded option for generating random values.
Random Distribution
The distribution determines how random values are spread across the specified range. For generating random numbers between 0 and 1, uniform distribution is the most suitable option. This distribution ensures that each number within the specified range has an equal chance of being generated.

Generating Random Numbers Between 0 and 1
Setting Up the Environment
To begin, ensure you include the necessary header for random number generation:
#include <iostream>
#include <random>
Creating the Random Number Generator
Step-by-Step Guide
-
Create a random engine: This is the foundation for generating numbers.
std::default_random_engine generator;
-
Define the distribution: Specify that you want random numbers between 0 and 1 using uniform real distribution.
std::uniform_real_distribution<double> distribution(0.0, 1.0);
Complete Code Example
Here’s a complete example that illustrates generating a random number between 0 and 1:
#include <iostream>
#include <random>
int main() {
std::default_random_engine generator; // Step 1: Create random engine
std::uniform_real_distribution<double> distribution(0.0, 1.0); // Step 2: Define distribution
// Generating a random number
double random_number = distribution(generator);
std::cout << "Random Number between 0 and 1: " << random_number << std::endl;
return 0;
}
Explanation of the Code
- Line 1-2: These lines include the necessary libraries. `<iostream>` is for input and output, while `<random>` contains the tools for random number generation.
- Line 5: We create a random engine named `generator`. It initializes the engine to start producing numbers.
- Line 6: The distribution `uniform_real_distribution<double>` is defined to create random numbers in the range from 0.0 to 1.0.
- Line 9: The actual random number is generated and stored in `random_number`.
- Line 10: Finally, we print the generated random number.
It's important to recognize that if you run the program multiple times without seeding, it will produce the same sequence of numbers each time since the default engine initializes the same way.
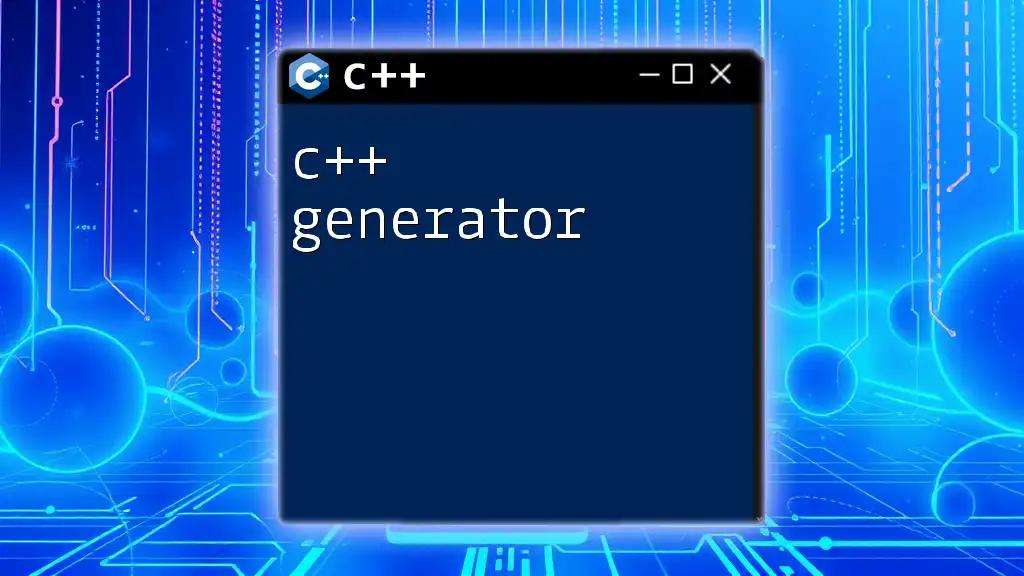
Common Pitfalls and How to Avoid Them
Misunderstanding Seed Values
To produce varied outputs with each execution, seeding is crucial. You can use `std::random_device`, which provides a non-deterministic seed:
std::random_device rd; // Use random device as seed
std::default_random_engine generator(rd()); // Seed the generator
Not Considering Performance
Different random engines have various performance characteristics. In scenarios requiring high-speed random number generation, consider engines like `std::mt19937`, which is often faster than `std::default_random_engine`.
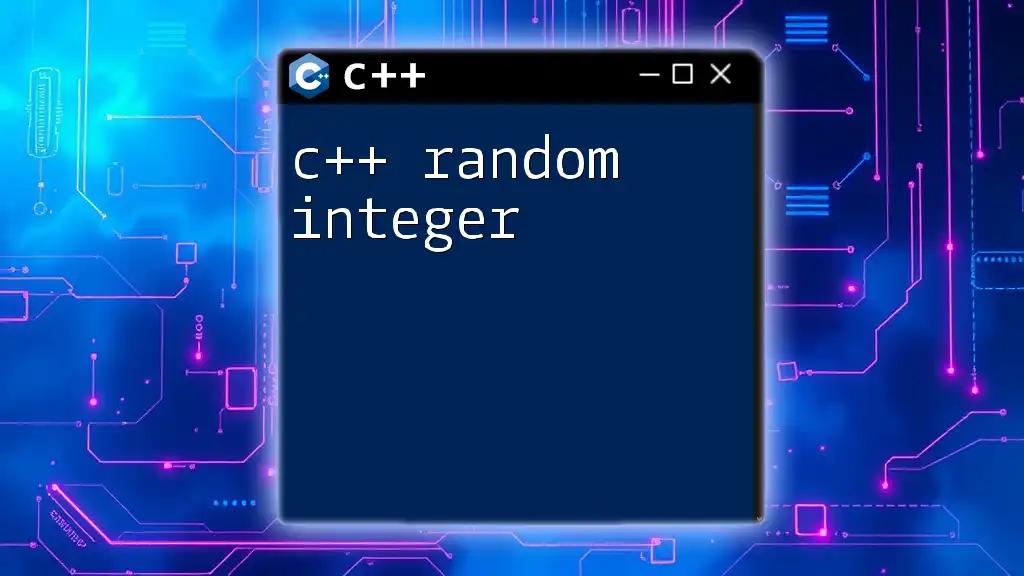
Advanced Techniques
Generating Multiple Random Numbers
To generate several random numbers in a single execution, you can utilize a loop. Here’s how to do that:
for (int i = 0; i < 10; ++i) {
std::cout << distribution(generator) << std::endl; // Generate and print ten random numbers
}
Using Different Distributions
C++ provides a variety of distributions. For instance, if you wanted to create random numbers that follow a normal distribution, you could use:
std::normal_distribution<double> normal_dist(0.5, 0.1); // mean 0.5, stddev 0.1
This will give you random numbers centered around 0.5 with a standard deviation of 0.1, meaning most numbers will fall close to this mean.
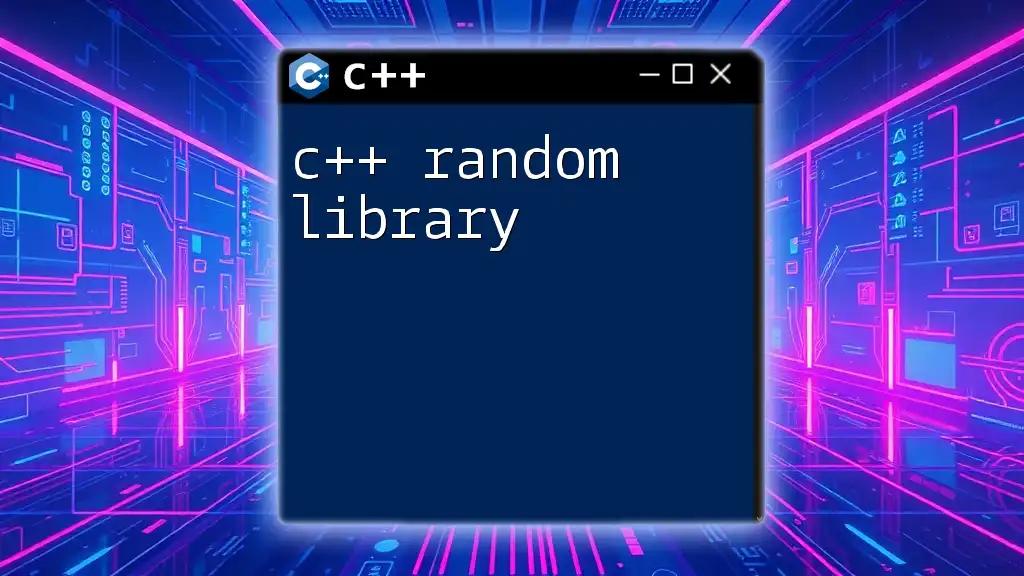
Practical Applications
Use in Game Development
Random number generation is widely used in game development. For instance, it can be used to determine the position of random enemies or loot drops. By controlling randomness, developers can maintain game balance and enhance user engagement.
Statistical Sampling
Random numbers also play a crucial role in statistical sampling. In simulations or surveys, random selections ensure unbiased results.
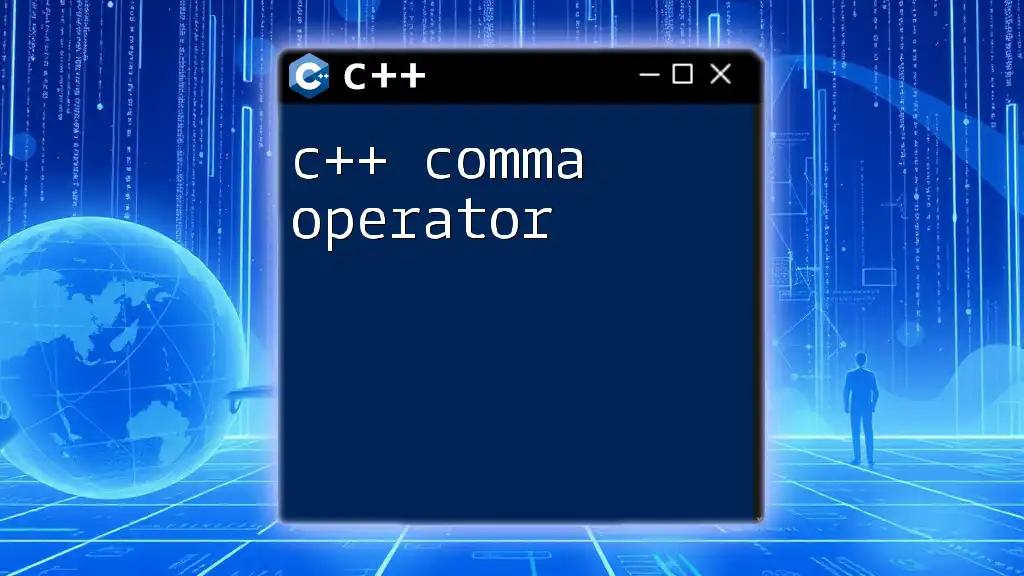
Conclusion
In conclusion, understanding the C++ random number generator between 0 and 1 is essential for creating dynamic and adaptable programs. With the ability to generate random numbers, you can enhance interactivity in games, improve simulations, and even conduct statistical analyses efficiently. Don’t hesitate to experiment with various random engines and distributions to discover the best solutions for your projects.
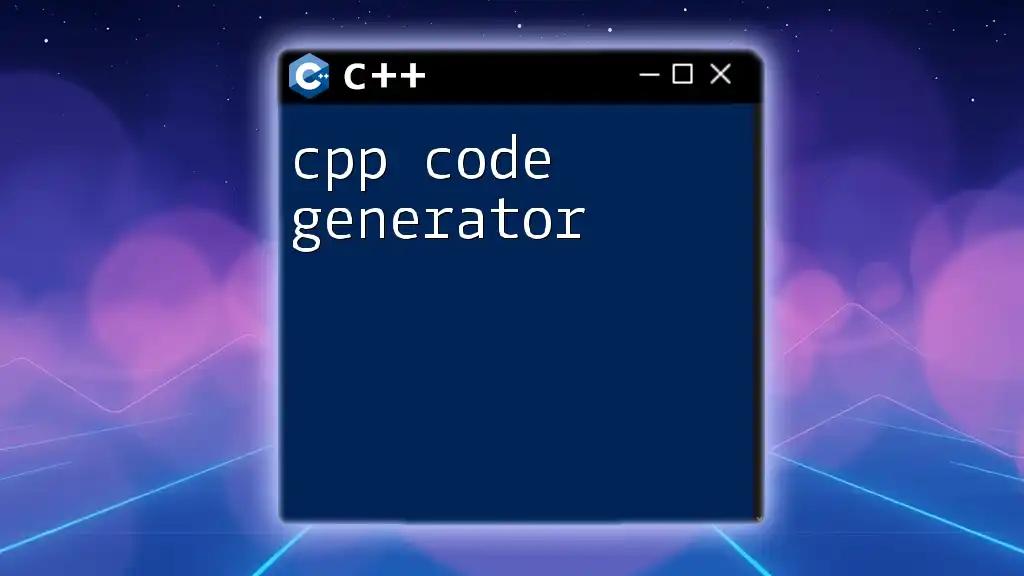
FAQs
How do I generate a truly random number in C++?
For truly random numbers, consider using external libraries that access hardware randomness, or utilize `std::random_device`, which may provide a better seed.
Are there any libraries for enhanced randomness in C++?
Yes, the Boost Random library offers enhanced capabilities and a variety of random number distributions.
What is the difference between `std::uniform_real_distribution` and `std::uniform_int_distribution`?
`std::uniform_real_distribution` generates floating-point numbers within a specified range, while `std::uniform_int_distribution` generates integer values within a defined interval. For instance, the latter could be used for scenarios like rolling a dice.