C++ was developed by Bjarne Stroustrup at Bell Labs in the early 1980s as an extension of the C programming language to include object-oriented features.
Here’s a simple C++ code snippet demonstrating a basic class:
#include <iostream>
class HelloWorld {
public:
void greet() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
HelloWorld hw;
hw.greet();
return 0;
}
The Birth of C++
The development of C++ can be traced back to the late 1970s and early 1980s, sparked by the limitations of the existing programming languages. Bjarne Stroustrup, a Danish computer scientist, was working at Bell Labs during this time. His key inspiration for creating C++ came from earlier programming languages such as C and Simula, the latter of which introduced robust object-oriented programming concepts.
Stroustrup's vision was to combine the low-level efficiency of C with high-level features found in Simula, allowing programmers to manage system resources effectively while also facilitating the development of complex software through abstraction and modularity.
Bjarne Stroustrup: The Creator
Bjarne Stroustrup's impact on the programming world cannot be understated. Having studied at the University of Aarhus in Denmark and obtaining a Ph.D. at Princeton University, Stroustrup focused on designing languages that combine the efficiency of C with features that could simplify programming challenges.
His journey began in 1979 when he started customizing a C compiler to create a language he eventually named C++. Stroustrup aimed to enable developers to write more complex programs without sacrificing performance. This vision ultimately led to the inception of a language that has become a cornerstone of software development.
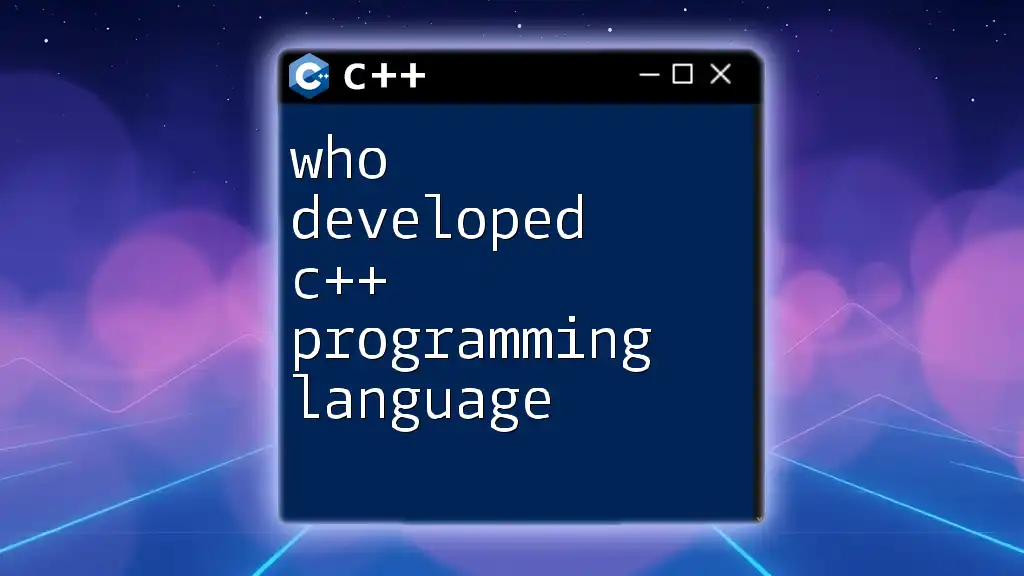
The Evolution of C++
From C to C++
C++ officially emerged in 1985, but its development process began years earlier with various iterations that incorporated features like classes and basic object-oriented programming. The key breakthrough came with the addition of features that were proactive rather than reactive, allowing developers to shape their programming practices around C++'s structure.
The first official version of C++ introduced essential concepts that would later be expanded upon, laying the groundwork for modern programming. For example, here’s a simple C++ code snippet that showcases basic syntax:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, you can see how C++ syntax allows for clear expression and output of information.
The Standardization of C++
The standardization process of C++ marked a significant milestone in its history. By the early 1990s, the language gained widespread popularity, prompting the need for a unified standard. The ANSI (American National Standards Institute) and later the ISO (International Organization for Standardization) committees stepped in to formalize the language.
Some key standards include:
- C++98: The first standardized version of C++.
- C++03: A bug-fix release of C++98.
- C++11: Introduced many modern features like auto, ranged for loops, and more.
- C++14, C++17, and C++20: Continued the trend of enhancing the language with additional features and improvements.
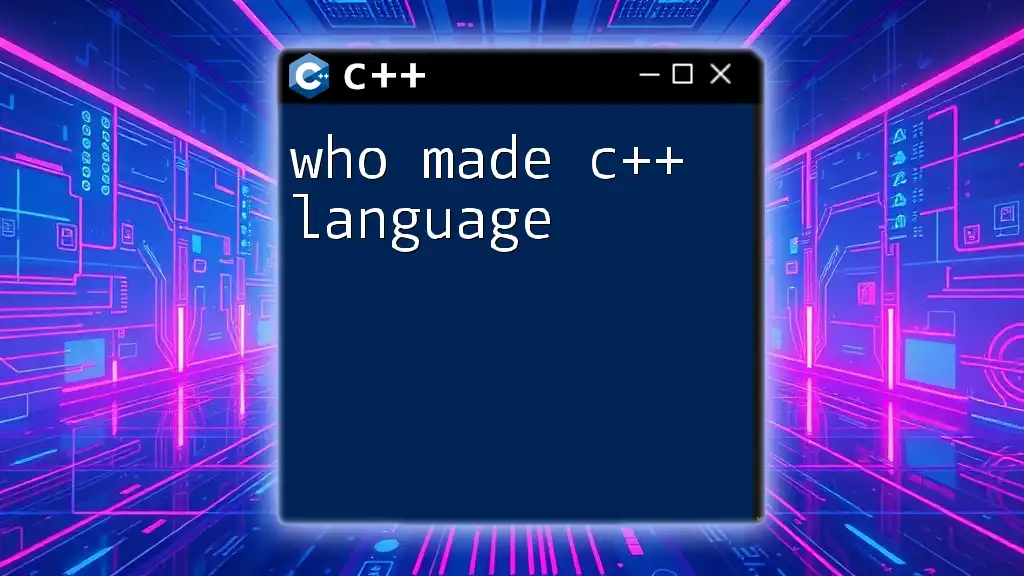
Key Contributions to C++
Major Enhancements and Features Over Time
One of the hallmark features of C++ is its Object-Oriented Programming (OOP) capabilities. Concepts such as classes, objects, inheritance, and polymorphism enable developers to create modular and reusable code. Here’s an example demonstrating OOP principles in C++:
class Animal {
public:
virtual void speak() { cout << "Animal speaks"; }
};
class Dog : public Animal {
public:
void speak() override { cout << "Bark!"; }
};
int main() {
Dog dog;
dog.speak(); // Outputs: Bark!
return 0;
}
In this example, we see how inheritance allows `Dog` to extend `Animal` while overriding the `speak` method for its specific behavior.
The Opening Up of C++
Another crucial milestone came with the introduction of templates and the Standard Template Library (STL). These features revolutionized C++ programming by enabling developers to write generic and highly reusable components. Templates allow for functions and classes to operate with any data type. Here’s a simple demonstration:
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
cout << add<int>(5, 3) << endl; // Outputs: 8
return 0;
}
This code defines a template function called `add`, showcasing how flexibility in data handling can simplify complex coding tasks.
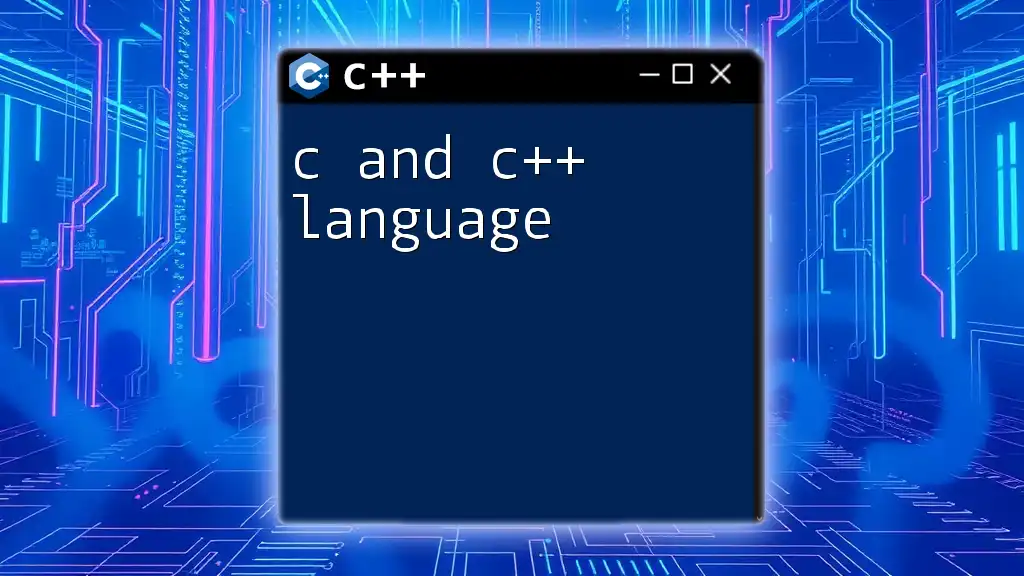
Impact of C++ on Software Development
Advancements in the Software Industry
C++ has had a profound impact on various domains, particularly in systems programming, game development, real-time simulations, and high-performance applications. Its capability to blend object-oriented and low-level procedural programming makes it ideal for performance-sensitive applications.
Comparison With Other Programming Languages
When comparing C++ with other programming languages like Java and Python, each language has its strengths and weaknesses. C++ is often noted for its performance and control over system resources, while Java excels in cross-platform portability, and Python is favored for ease of learning and readability.
However, the complexity of C++ can present a steep learning curve for beginners, yet its power and flexibility remain unmatched in many development scenarios.
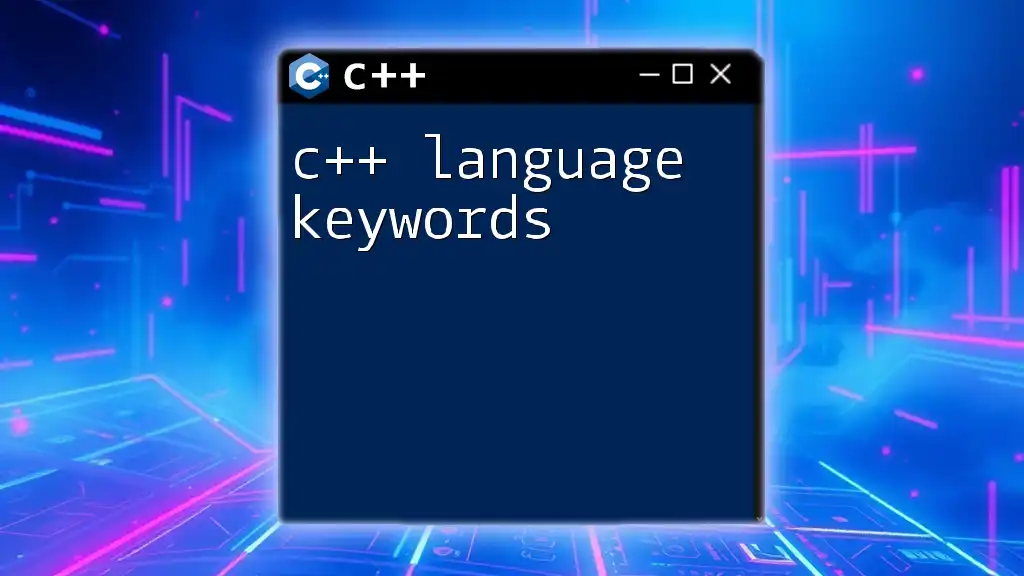
Conclusion
The journey of C++ from its inception to its role as a dominant programming language demonstrates the vision and ingenuity of Bjarne Stroustrup. His ingenuity turned an idea into a language that empowers programmers to create efficient and scalable software. The future of C++ seems promising, continuing to evolve and adapt to industry needs while preserving the principles that have made it a staple in software development. C++ remains a vital tool for developers aiming to harness the full potential of modern computing.
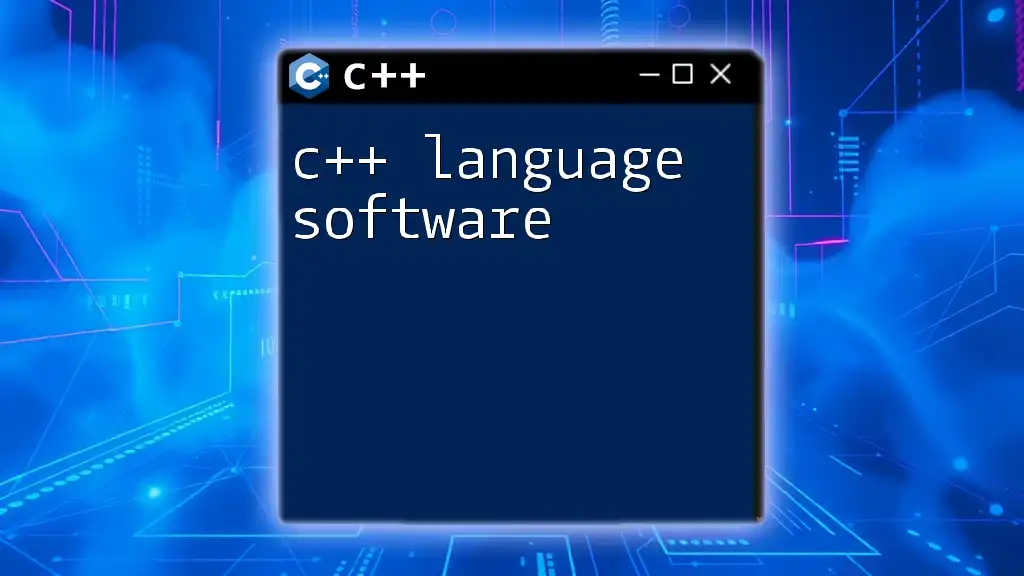
References and Further Reading
For those interested in exploring C++ deeper, consider diving into the numerous resources available including classic texts like "C++ Primer" by Stanley B. Lippman, Lajoie, and Moo, or "Effective Modern C++" by Scott Meyers, which can offer insights into advanced techniques and best practices. Online courses on platforms like Udemy or Coursera can also provide structured learning paths for mastering C++.