C++ is a powerful programming language that has been used to develop many high-performance games, offering developers enhanced control over system resources and performance.
#include <iostream>
int main() {
std::cout << "Welcome to the C++ Game Development!" << std::endl;
return 0;
}
Understanding C++ and Its Advantages in Game Development
What is C++?
C++ is a powerful, object-oriented programming language that evolved from C, created by Bjarne Stroustrup in the early 1980s. Renowned for its performance and flexibility, C++ has become a staple in various software development fields, especially in game development. Unlike some higher-level languages, C++ allows developers to finely control system resources, which can lead to efficient and responsive games.
Comparative advantages of C++ include stricter performance metrics compared to languages like C# or Java. This makes it particularly useful for developing complex, resource-intensive games where optimizing performance is vital.
Key Advantages of Using C++ in Games
Performance and Efficiency
C++ excels in speed and memory management, which is crucial for games that require real-time responsiveness. The language compiles directly to machine code, ensuring that games run quickly and smoothly. For instance, a basic C++ loop to process game logic might look like this:
for (int i = 0; i < MAX_FRAMES; ++i) {
updateGameState();
renderFrame();
}
This simple loop showcases how efficiently C++ can handle repetitive processes inherent in game mechanics.
Object-Oriented Programming
C++ is designed around object-oriented programming (OOP) principles, which allow for better organization and reusability of code. OOP concepts such as inheritance, encapsulation, and polymorphism enable developers to create complex game systems more manageably. A basic class structure for a game character would appear as follows:
class Character {
public:
Character(std::string name) : name(name) {}
void move(int x, int y) {
position.x += x;
position.y += y;
}
private:
std::string name;
struct { int x, y; } position;
};
Here, encapsulation protects the character’s data while providing methods to manipulate it, which is essential for maintaining the integrity of game data.
Cross-Platform Development
C++ supports cross-platform development, allowing developers to create games that run on multiple platforms, including Windows, macOS, and various consoles. This is largely due to the extensive libraries that interact with platform-specific features. For example, libraries like SDL (Simple DirectMedia Layer) help streamline the process of creating applications across diverse environments.

Iconic Games Developed in C++
AAA Titles Using C++
Several iconic games have been developed using C++, demonstrating the language's capabilities:
-
The Witcher 3: Wild Hunt: This critically acclaimed RPG showcases intricate world-building and complex character interactions. C++ is pivotal in managing the game's vast open-world environment and the myriad AI behaviors that populate it.
-
Doom (2016): By leveraging C++ for its engine architecture, Doom achieved exceptional performance and realism. The game's fast-paced mechanics benefit greatly from the language's efficiency.
-
Counter-Strike: This staple of the multiplayer landscape is largely built on C++. The language's strength in handling network communications and real-time decision-making has been crucial for the game's development.
Indie Games That Made Their Mark
Indie developers have also embraced C++ for its power and flexibility, resulting in successful titles such as:
-
Fez: This puzzle-platformer employs C++ to create its visually distinctive, non-linear gameplay experience, optimizing rendering capabilities for its unique graphic design.
-
Braid: Well-known for its innovative gameplay mechanics, Braid utilizes C++ to implement time manipulation features. The game showcases how C++ can efficiently manage complex state changes in response to player actions.
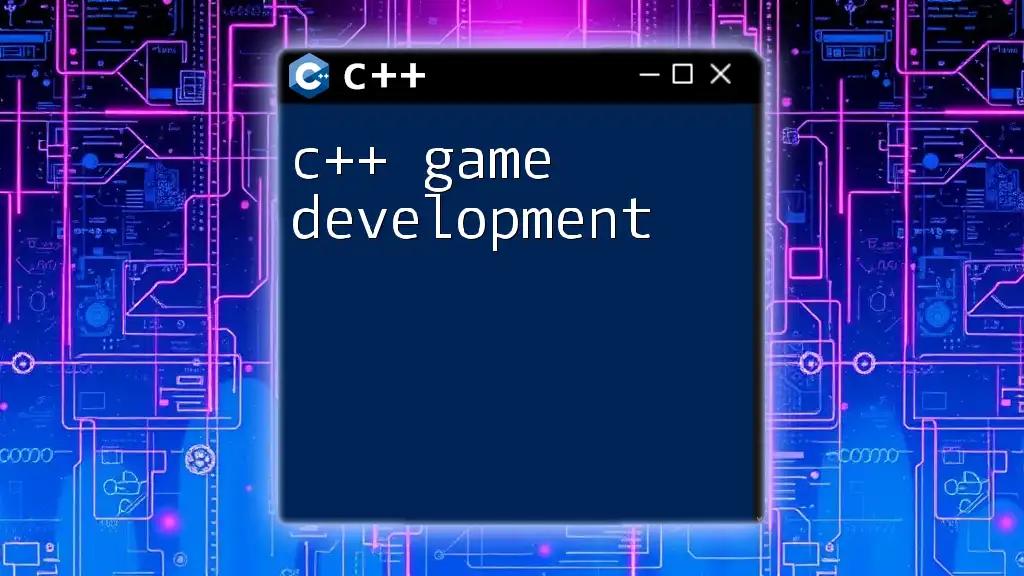
Popular Game Engines That Use C++
Unreal Engine
The Unreal Engine, developed by Epic Games, exemplifies the power of C++ in game development. Providing robust features for both 2D and 3D games, it allows developers to harness high-fidelity graphics and immersive gameplay environments. A simple snippet of player movement coded in Unreal Engine could appear as:
void AMyPlayer::MoveForward(float Value) {
if (Value != 0.0f) {
AddMovementInput(GetActorForwardVector(), Value);
}
}
This example demonstrates how C++ can facilitate complex interactions within a game environment effortlessly.
Unity (C++ Libraries)
While Unity is primarily scripted in C#, it allows developers to use C++ libraries for performance-critical tasks. Integrating C++ involves creating native plugins that can optimize rendering or physics computations, utilizing the strengths of both languages.
CryEngine
CryEngine is another robust game engine built primarily on C++. Known for its stunning graphics and realistic physics, CryEngine utilizes C++ to manage graphics rendering and game logic execution seamlessly, making it a preferred choice for high-end game development.
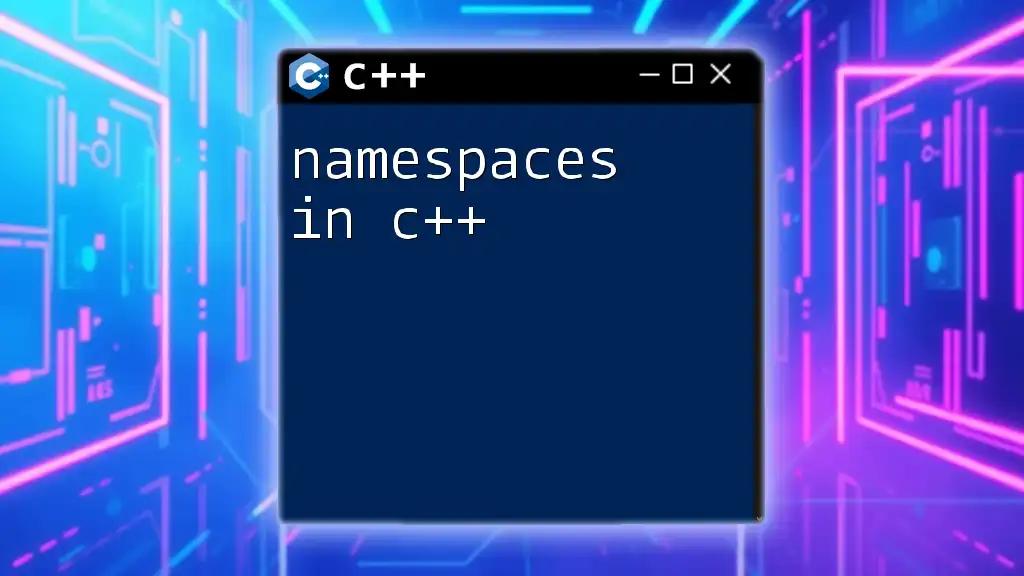
Case Studies: C++ in Game Development
Analyzing Game Architecture
Many successful games attribute their performance improvements to efficient source code architecture achieved through C++. By analyzing case studies, developers can pinpoint how optimizing code in C++ leads to smoother gameplay and lower latency.
Success Stories from Development Teams
Insights from development teams often reveal challenges and breakthroughs in C++. Developers emphasize that the language enabled them to solve complex problems related to memory management and performance tuning, vital for delivering quality gaming experiences under competitive conditions.

Key Concepts in C++ Game Programming
Memory Management
C++ gives developers intense control over memory allocation and management, which is crucial in optimizing game performance. Manual memory management allows for the allocation and deallocation of resources on-demand. A simple representation of dynamic memory allocation would look like:
Object* obj = new Object();
// Utilize obj for gameplay functionality
delete obj; // Important to free memory
Game Loops
Understanding the game loop is fundamental in C++ game development. It allows for continuous updating of game status and rendering of visuals. A straightforward game loop might resemble:
while (gameIsRunning) {
processInputs();
updateGameState();
renderFrame();
}
This loop illustrates how C++ maintains a steady frame rate and response time during gameplay.
Graphics Programming
C++ is indispensable in graphics programming through libraries like OpenGL and DirectX. For rendering graphics, C++ code might be structured as follows:
// Initializing OpenGL
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_TRIANGLES);
// Define vertices here
glEnd();
This snippet signifies how C++ can efficiently control and render complex graphical scenes in real-time.
Artificial Intelligence in Games
C++ is widely employed to create advanced AI systems in games. Developers leverage finite state machines or behavior trees for dynamic character behaviors. A simplistic state machine implementation might look like this:
enum State { IDLE, RUNNING, ATTACKING };
class AICharacter {
State currentState;
void update() {
switch (currentState) {
case IDLE: // Logic for idle state
break;
case RUNNING: // Logic for running state
break;
case ATTACKING: // Logic for attacking behavior
break;
}
}
};
Through this use of C++, developers can create rich, engaging AI behaviors, enhancing the player's gaming experience.

Resources for Learning C++ for Game Development
Online Courses and Tutorials
Numerous online platforms offer dedicated courses on C++ for game development. Websites like Udemy, Coursera, and even YouTube channels provide valuable resources and tutorials tailored specifically to C++ gaming.
Books and Documentation
Books such as "Programming: Principles and Practice Using C++" by Bjarne Stroustrup offer foundational knowledge, alongside official C++ documentation which is crucial for understanding the syntax and features of the language.
Community and Forums for C++ Developers
Networking with fellow developers is crucial in fostering growth. Engaging with online forums such as Stack Overflow or dedicated C++ game development communities can provide insights, troubleshooting help, and mentorship opportunities.
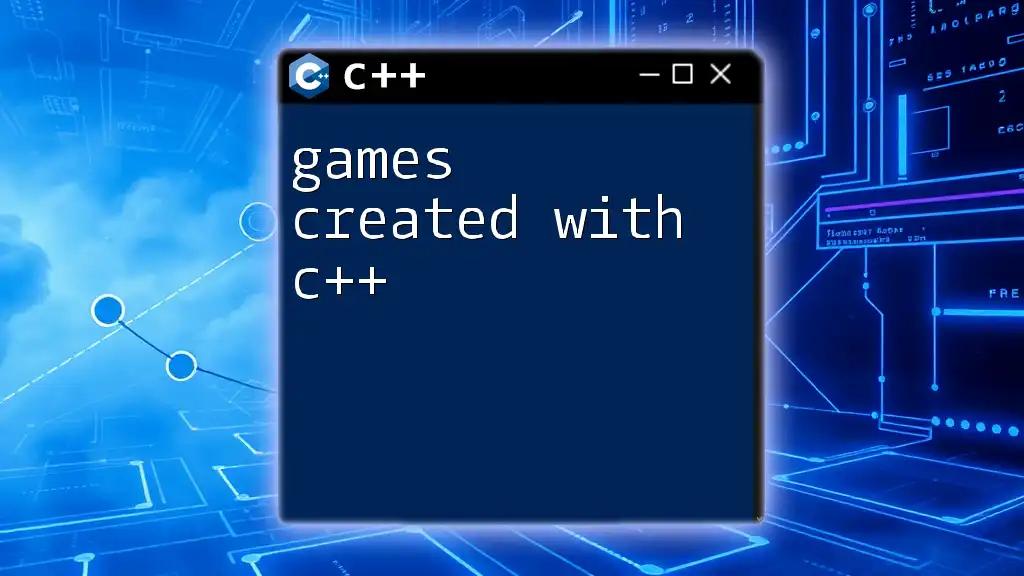
Future of C++ in Game Development
The landscape of game development is constantly evolving, and C++ is at the forefront of emerging trends such as virtual reality (VR) and augmented reality (AR). With increasing demands for realistic graphics and intricate game mechanics, C++ continues to evolve, adapting to the growing needs of developers and gamers alike.
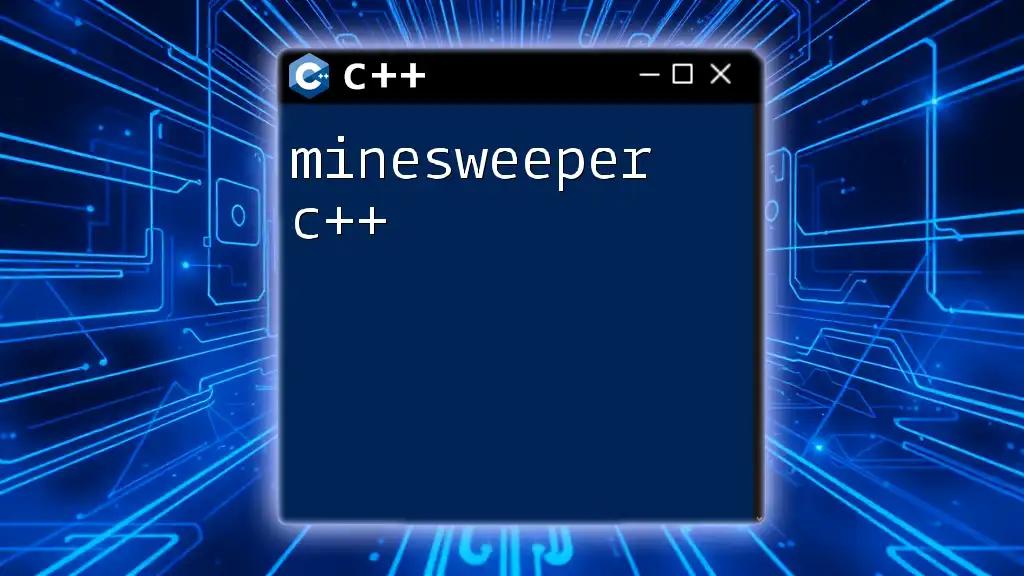
Conclusion
C++ remains an indispensable tool in the development of games, from high-budget AAA titles to innovative indie projects. Its performance and flexibility ensure that developers can maintain control over every aspect of game design and execution. As you explore your own journey into game development with C++, the various resources and techniques discussed will equip you with the knowledge needed to succeed in this exciting field.
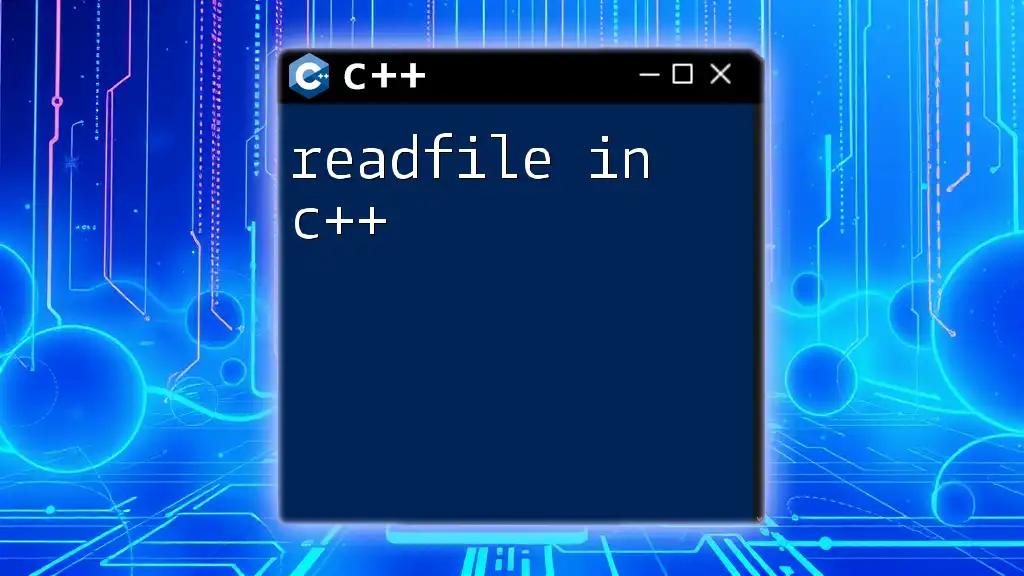
Call to Action
Embark on your game development journey today using C++. Explore additional resources, engage with other developers, and start creating captivating gaming experiences!