The `abs` function in C++ is used to compute the absolute value of a number, ensuring that the result is non-negative regardless of the input's sign.
Here's an example code snippet demonstrating its use:
#include <iostream>
#include <cstdlib> // Required for abs function
int main() {
int number = -5;
std::cout << "The absolute value of " << number << " is " << abs(number) << std::endl;
return 0;
}
What is Absolute Value?
The absolute value of a number represents its distance from zero on the number line, regardless of direction. This means that both positive and negative values share the same absolute value. For example, the absolute value of -5 is the same as the absolute value of 5, which is 5 itself.
In programming, the absolute value is crucial in various computations, like finding distances, ensuring values are non-negative, and handling mathematical functions that require non-negative inputs.
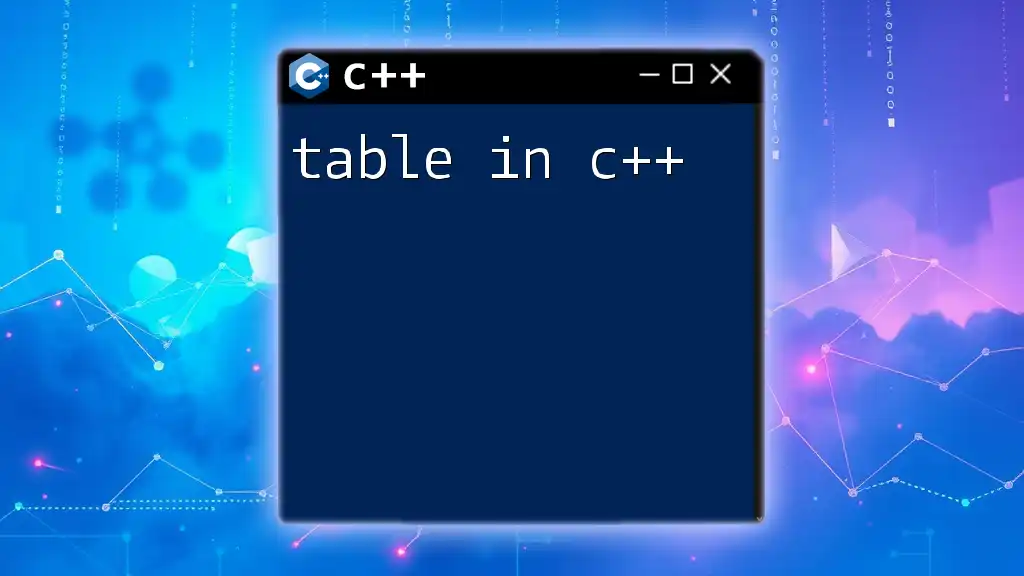
Overview of the C++ Absolute Value Function
In C++, the function used to compute the absolute value is called `abs`. It is included in the C++ Standard Library, which provides a wide range of built-in functions for efficient programming.
The `abs` Function
The `abs` function can take different data types as arguments:
- int: For integer values.
- float: For floating-point values.
- double: For double-precision floating-point values.
Example of Using `abs` in C++
Here is a simple code snippet demonstrating how to use the `abs` function to find the absolute value of an integer:
#include <iostream>
#include <cstdlib> // Required for abs function
int main() {
int num = -42;
int absoluteValue = abs(num);
std::cout << "Absolute value of " << num << " is " << absoluteValue << std::endl;
return 0;
}
In this example, we include the `cstdlib` header, which provides access to the `abs` function. The program initializes a variable `num` with a negative integer, and then it computes the absolute value using the `abs` function, storing the result in `absoluteValue`. Finally, the result is printed to the console.
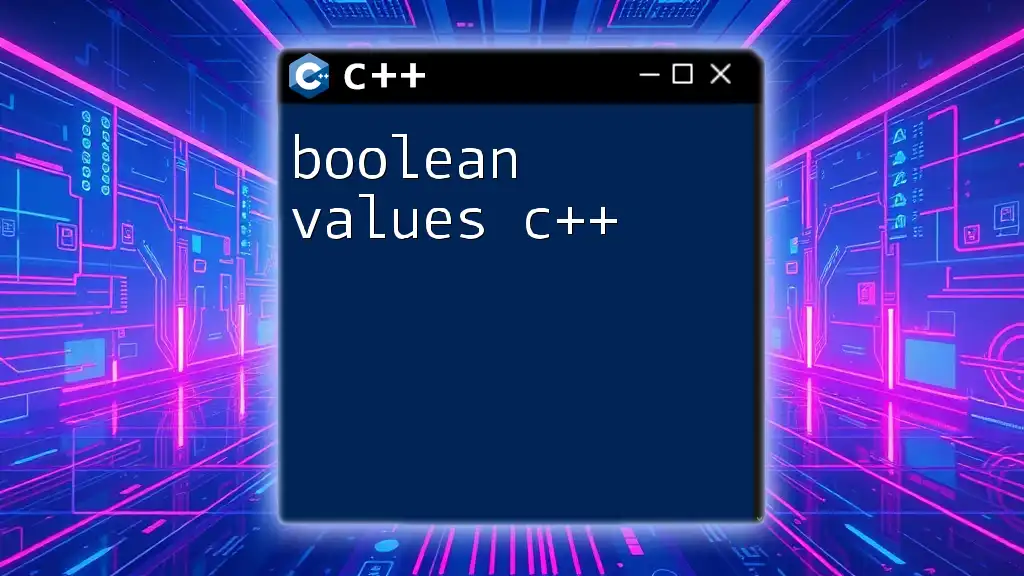
Using Absolute Value in Different Contexts
Absolute Value for Integers
For integer values, the `abs` function can be used directly. The behavior is straightforward: it returns the non-negative form of the integer.
Absolute Value for Floating Points
For floating-point numbers, C++ uses the `fabs` function, which stands for floating-point absolute value. Here's an example:
#include <iostream>
#include <cmath> // Required for fabs function
int main() {
double num = -3.14;
double absoluteValue = fabs(num);
std::cout << "Absolute value of " << num << " is " << absoluteValue << std::endl;
return 0;
}
In this code, we include the `<cmath>` library, which provides access to mathematical functions. The variable `num` is initialized with a negative floating-point value, and we calculate the absolute value using the `fabs` function. The result is printed to the console, demonstrating that `fabs` effectively handles floating-point types.
Absolute Value with User Input
You can also compute the absolute value of a number that the user inputs. Here’s how:
#include <iostream>
#include <cstdlib> // Required for abs function
int main() {
int num;
std::cout << "Enter an integer: ";
std::cin >> num;
std::cout << "Absolute value is " << abs(num) << std::endl;
return 0;
}
In this example, we prompt the user to enter an integer. The program then reads the input and calculates the absolute value by directly using the `abs` function. It’s an excellent way to encourage user interaction while showcasing the functionality of the `abs` function.
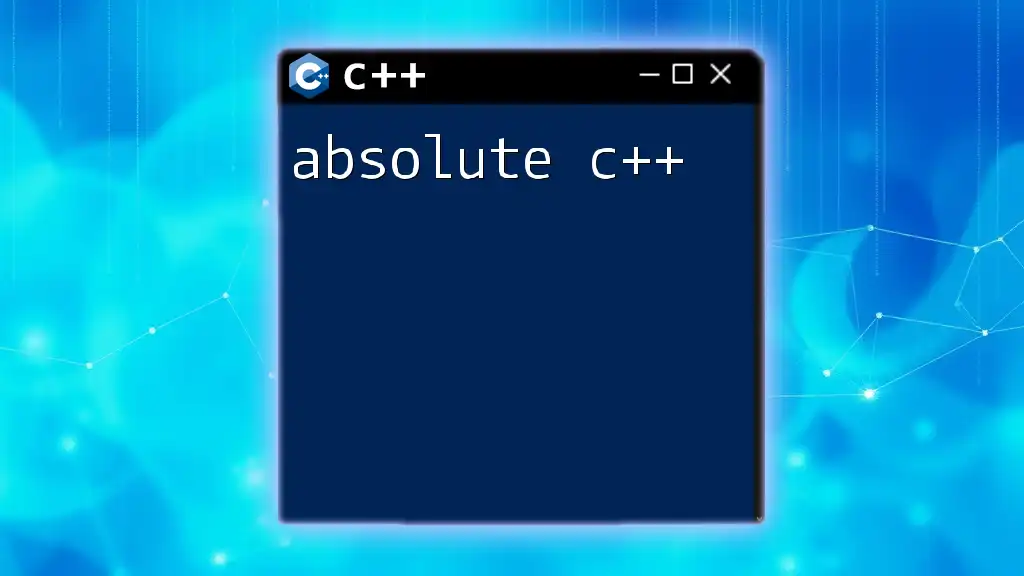
Custom Absolute Value Function in C++
When to Create Your Own Absolute Function
While C++ provides the `abs` and `fabs` functions, there may be cases—such as educational purposes or when implementing customized logic—where you may want or need to create your own absolute value function.
Code Example for Custom Absolute Function
Here’s how you can implement your own custom absolute value function:
#include <iostream>
int customAbs(int num) {
return (num < 0) ? -num : num;
}
int main() {
int num = -10;
std::cout << "Custom absolute value of " << num << " is " << customAbs(num) << std::endl;
return 0;
}
In the above snippet, we create a function named `customAbs` that takes an integer as an argument. Using a simple ternary operator, it returns the negation of the number if it is less than zero; otherwise, it simply returns the number. This showcases how you can implement logical checks to replicate existing functionality or cater to specific needs.
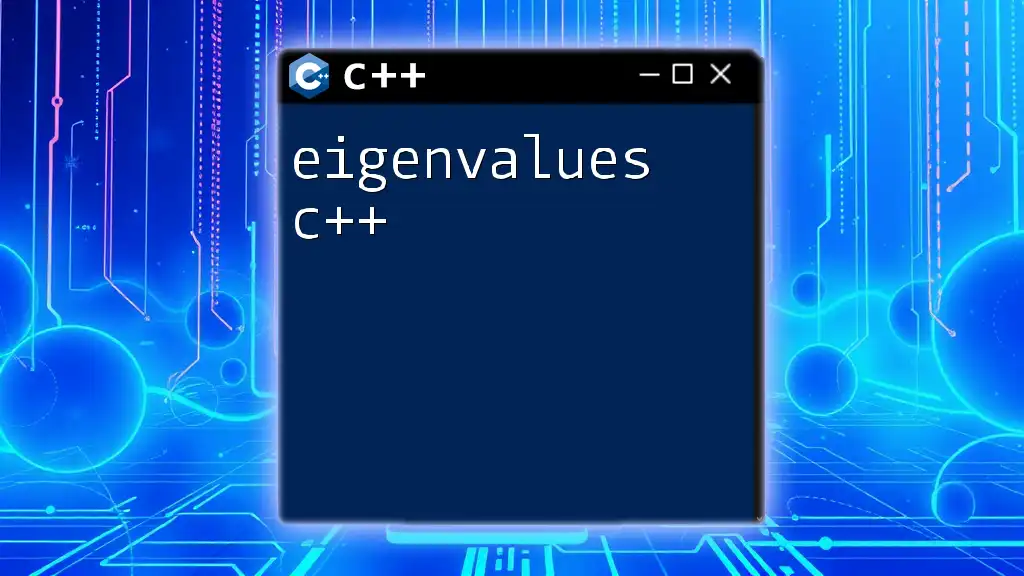
Common Errors and Troubleshooting
Ensuring Correct Header Files
When using the `abs` function, make sure to include the correct header files. The `<cstdlib>` header is essential for integer operations with `abs`, while the `<cmath>` header is required for floating-point operations with `fabs`.
Data Type Mismatches
A common issue when using absolute functions is data type mismatches. If you try to use `abs` on types other than integers, it may return unexpected results or even compile errors. Always ensure you are passing the correct data type to the function.
Precision Errors with Float and Double
Floating-point calculations involve precision and representation limitations. When using absolute values with floats or doubles, be aware of these potential issues, especially in high-precision environments. Utilize `fabs` for floating-point absolute value calculations to maintain accuracy.
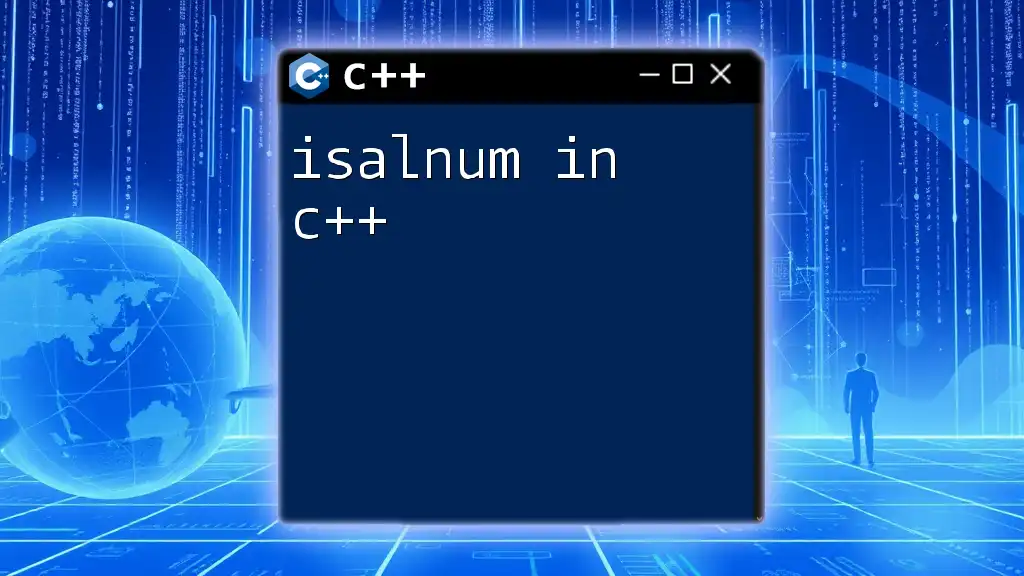
Practical Applications of Absolute Value in C++
Understanding how to use the absolute value function can be essential in many programming scenarios. Here are a few practical applications:
-
Distance Calculation: Finding the distance between two points on a number line requires absolute values to account for direction.
-
Game Development: In game programming, absolute values are used to compute rendering distances, health points, and other metrics where magnitude matters rather than direction.
-
Finance: In financial applications, calculations comparing gains and losses often require the absolute value to interpret them in terms of magnitude.
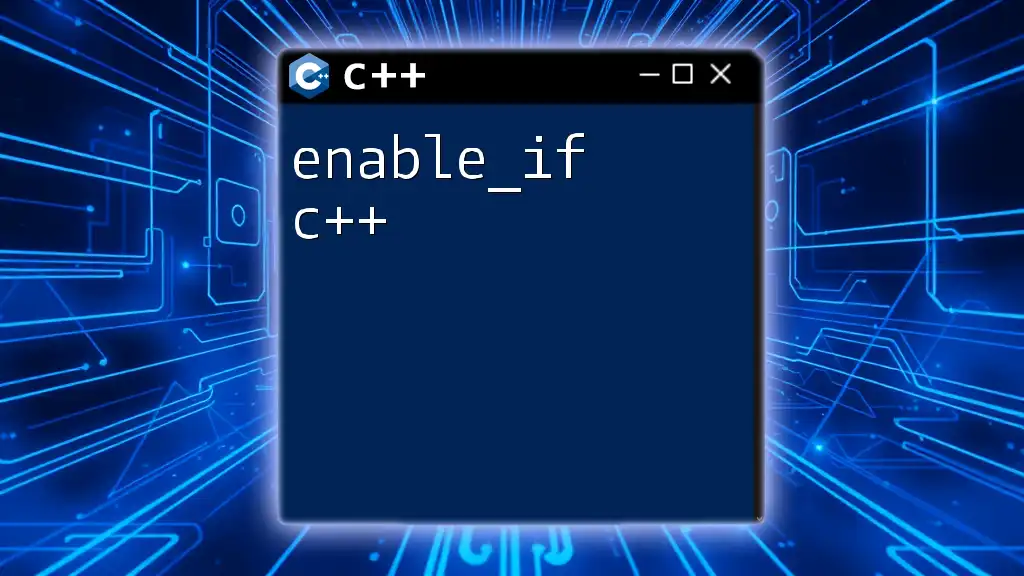
Conclusion
Understanding how to compute the abs value in C++ is vital for programming tasks involving mathematics, user interactions, and algorithms. Through the `abs` function and its floating-point counterpart, `fabs`, programmers can effectively employ absolute values for diverse applications.
Experimenting with both built-in and custom implementations of these functions can enhance your programming skills and deepen your understanding of C++. Embrace the challenge and explore the various ways to incorporate absolute values into your C++ projects!
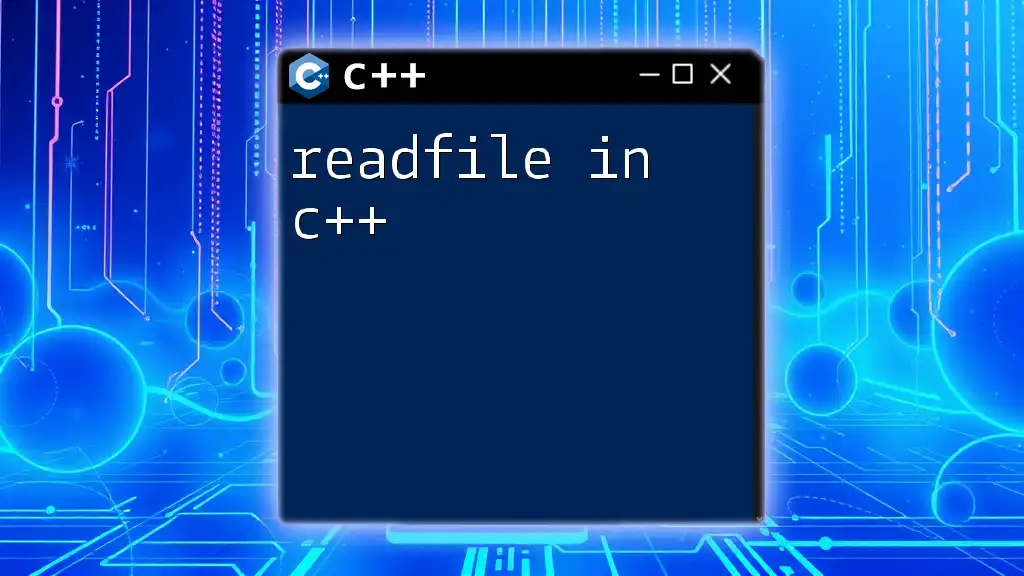
Further Reading and Resources
For those interested in expanding their knowledge further, consider delving into C++ programming books, online courses, and official documentation. Forums and communities are also excellent places to seek additional help and share experiences related to C++.
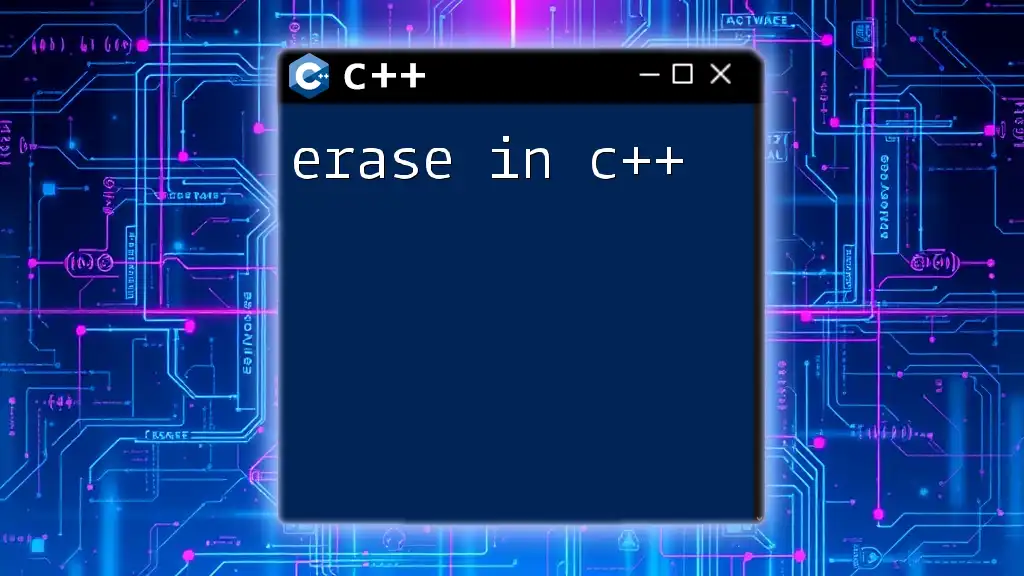
Call to Action
We encourage you to share your experiences or questions about using absolute values in C++. How have you integrated them into your projects? Let us know in the comments below or share your thoughts on social media!