Many popular games have been developed using C++, showcasing its efficiency and performance in rendering graphics and managing resources. Here's a simple code snippet demonstrating a basic C++ structure for a game loop:
#include <iostream>
int main() {
bool isRunning = true;
while (isRunning) {
// Game logic goes here
std::cout << "Game is running..." << std::endl;
// Exit condition (for demonstration purposes)
isRunning = false; // Set to 'false' to exit loop after one iteration
}
return 0;
}
Understanding C++ as a Game Development Language
Advantages of Using C++
Performance and Speed
C++ is renowned for its performance capabilities, allowing developers to create games that run efficiently and smoothly. This performance is largely due to its close-to-hardware nature, granting finer control over system resources than many higher-level languages. For example, in a game where framerate is critical, C++ can optimize calculations to ensure seamless gameplay. Here’s a simple illustration of why C++ is faster compared to an interpreted language:
// Simple performance comparison
#include <iostream>
#include <chrono>
void runHeavyCalculation() {
for (int i = 0; i < 1000000; ++i) {
// Simulated heavy calculation
}
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
runHeavyCalculation();
auto stop = std::chrono::high_resolution_clock::now();
std::cout << "Time taken: " << std::chrono::duration_cast<std::chrono::milliseconds>(stop - start).count() << " ms" << std::endl;
return 0;
}
Control Over System Resources
One crucial aspect of game development is memory management. C++ provides extensive capabilities to allocate and deallocate memory efficiently, which is pivotal when creating expansive game worlds. For example, pointers in C++ allow developers to manipulate memory directly, enabling them to manage resources dynamically, which can enhance the gameplay experience.
Here’s a quick demonstration of using pointers:
// Using pointers in C++
#include <iostream>
void createGameObject(int* object) {
*object = 42; // Assigning value to the allocated memory
}
int main() {
int* gameObject = new int;
createGameObject(gameObject);
std::cout << "Game object value: " << *gameObject << std::endl;
delete gameObject; // Don't forget to free memory!
return 0;
}
Object-Oriented Programming
C++ is a powerful object-oriented programming language, allowing developers to use classes and objects to model real-world entities in games. This helps in creating maintainable and scalable code. By utilizing OOP concepts, developers can encapsulate data and behavior, promoting code reusability. Below is a simple class implementation representing a game character:
// Simple game character class
#include <iostream>
class GameCharacter {
public:
std::string name;
int health;
GameCharacter(std::string characterName, int characterHealth) {
name = characterName;
health = characterHealth;
}
void attack() {
std::cout << name << " attacks!" << std::endl;
}
};
int main() {
GameCharacter hero("Knight", 100);
hero.attack();
return 0;
}
Popular Game Engines Using C++
Unreal Engine
Unreal Engine, one of the most popular game engines, is heavily reliant on C++. Its robust architecture and extensive features are facilitated by the efficiency of C++. Genres from FPS to RPG have been built on this engine, showcasing its versatility. Notable games include Fortnite, Final Fantasy VII Remake, and Gears of War.
CryEngine
CryEngine is another powerful C++ based engine, known for its impressive graphics capabilities and real-time rendering. Games like Crysis and Far Cry leverage the power of C++ to maintain high graphical fidelity and complex gameplay mechanics.
Custom C++ Game Engines
While there are numerous popular engines available, many developers opt to create custom engines tailored to specific game requirements. This approach, although time-consuming, allows complete control over every aspect of the game. A simple structure might include a game loop, rendering engine, and resource management modules.
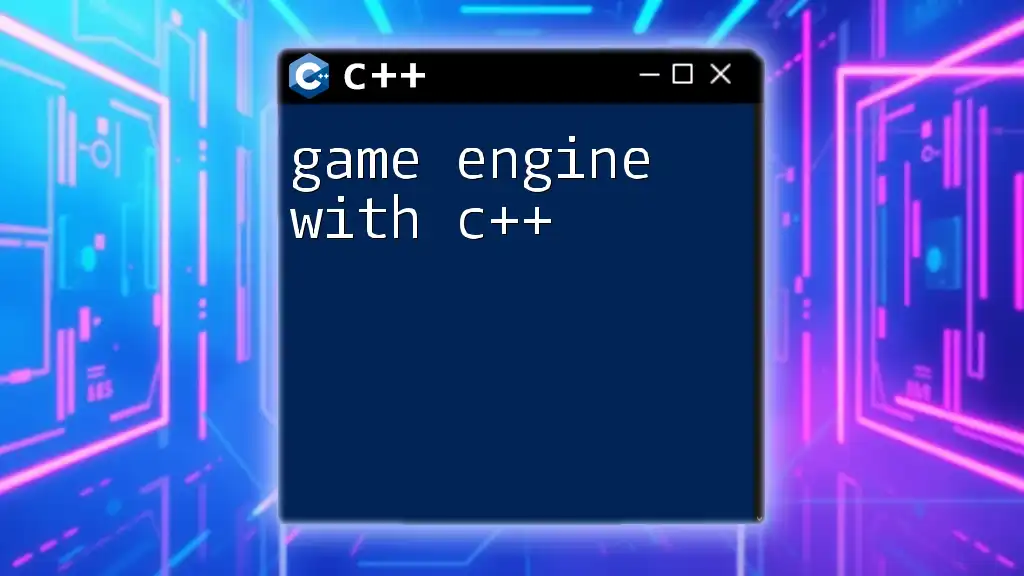
Iconic Games Developed with C++
AAA Titles
The Witcher 3: Wild Hunt
Developed by CD Projekt Red, The Witcher 3 is celebrated for its storytelling and vast open world. The game extensively utilizes C++ for its performance and ability to manage complex systems such as AI characters and real-time graphics. The seamless immersion and fluid gameplay are attributed to C++ optimizing computations and memory usage.
Call of Duty Series
With a legacy spanning over a decade, the Call of Duty series employs C++ to deliver frenetic, action-packed gameplay. The engine’s optimization is crucial in delivering high-intensity first-person shooting experiences that demand low latency and high responsiveness.
Indie Games
Fez
Fez combines retro graphics with innovative gameplay mechanics. The unique perspective-shifting mechanics are coded in C++, demonstrating the language's flexibility for both indie and larger scale projects. The efficient use of resources contributes to the artistic experience and gameplay fluidity.
Stardew Valley
This beloved indie farming simulator showcases C++ for smooth and engaging gameplay. With its pixel art style and complex game logic (like schedules for characters), C++ plays a vital role in managing various game elements efficiently, ensuring players enjoy a seamless gaming experience.
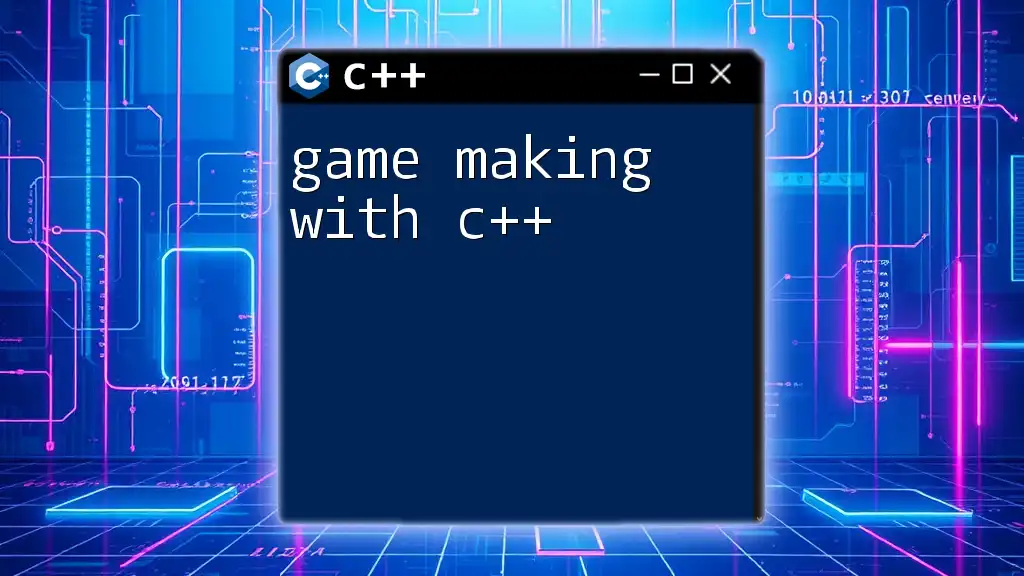
How C++ Addresses Game Development Challenges
Memory Management
Effective memory management is critical in game development. C++ allows developers to manage memory dynamically and provide the required optimizations for high-performance applications. Using smart pointers, such as `std::unique_ptr` or `std::shared_ptr`, helps automate memory management and reduce memory leaks.
Here’s a code snippet illustrating smart pointers:
#include <iostream>
#include <memory>
class GameObject {
public:
GameObject() { std::cout << "GameObject created." << std::endl; }
~GameObject() { std::cout << "GameObject destroyed." << std::endl; }
};
int main() {
std::unique_ptr<GameObject> obj = std::make_unique<GameObject>();
// Automatic memory management - no need for delete
return 0;
}
Graphics and Rendering
C++ plays a central role in graphics programming. Large-scale game studios often leverage graphics APIs like OpenGL or DirectX, both of which provide powerful tools for rendering stunning visuals. Rendering loops are fundamental, and here’s a basic example of a rendering loop in C++:
// Basic rendering loop
#include <iostream>
#include <chrono>
void renderFrame() {
// Simulated rendering logic
std::cout << "Rendering frame..." << std::endl;
}
int main() {
while (true) {
auto start = std::chrono::high_resolution_clock::now();
renderFrame();
auto stop = std::chrono::high_resolution_clock::now();
// Simulated frame timing (60 FPS)
std::this_thread::sleep_for(std::chrono::milliseconds(16));
}
return 0;
}
Physics and Simulation
Realistic physics simulation enhances gaming experience and immersion. C++ is often used in conjunction with physics libraries such as Bullet Physics or Unity’s built-in physics engine. Below is a simplified example showing how physics interactions can be simulated:
// Simulated simple physics interaction
#include <iostream>
class PhysicsObject {
public:
float mass;
float velocity;
PhysicsObject(float m, float v) : mass(m), velocity(v) {}
void applyForce(float force) {
velocity += force / mass; // Basic F=ma
}
void update() {
std::cout << "Current velocity: " << velocity << std::endl;
}
};
int main() {
PhysicsObject ball(2.0f, 0.0f);
ball.applyForce(10.0f); // Apply a force
ball.update(); // Output updated velocity
return 0;
}
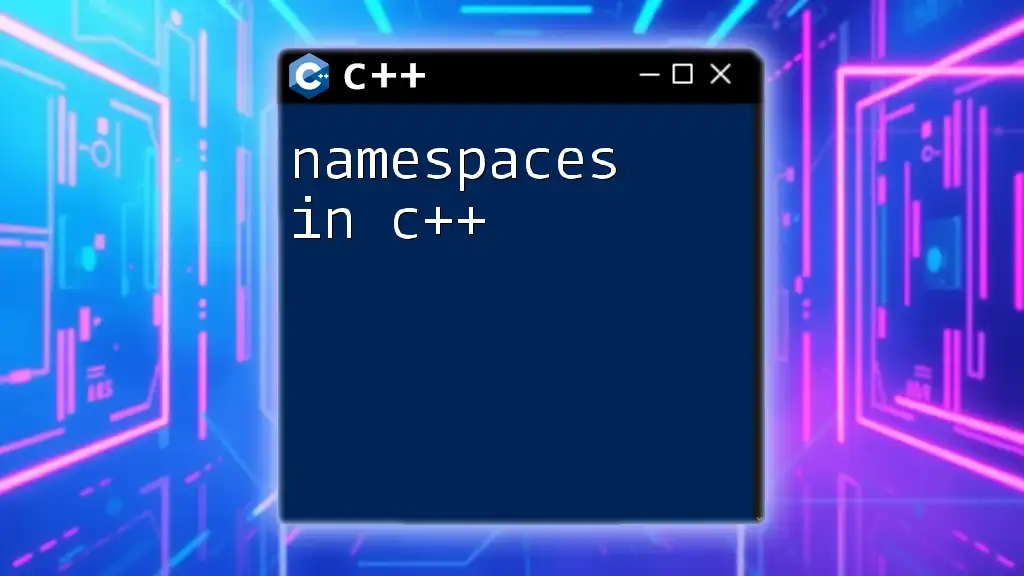
Learning C++ for Game Development
Resources for C++ Game Developers
To dive deeper into C++ and game development, consider these resources:
- Books: "Beginning C++ Through Game Programming" by Michael Dawson is a great start.
- Online Courses: Websites like Coursera or Udemy provide excellent courses focused on C++ for game development.
- Communities: Join forums such as Stack Overflow or game development discords. GitHub is also a treasure trove of open-source C++ projects you can explore and learn from.
Essential Skills and Concepts
Understanding Game Loops
The game loop is a fundamental element of any game. It executes continuously, allowing the game to process input, update game state, and render graphics. Here’s a basic structure for a game loop:
// Basic game loop structure
#include <iostream>
bool isRunning = true;
void processInput() {
// Handle player input
}
void updateGame() {
// Update game state
}
void render() {
// Render graphics
}
int main() {
while (isRunning) {
processInput();
updateGame();
render();
}
return 0;
}
Event Handling
Creating responsive input management is vital. C++ can handle user inputs effectively, enabling game characters to react immediately. Here’s an example of event handling:
// Simple event handling example
#include <iostream>
void handleKeyPress(char key) {
if (key == 'w') {
std::cout << "Move forward" << std::endl;
}
else if (key == 's') {
std::cout << "Move backward" << std::endl;
}
}
int main() {
char keyPressed;
std::cout << "Press 'w' or 's': ";
std::cin >> keyPressed;
handleKeyPress(keyPressed);
return 0;
}
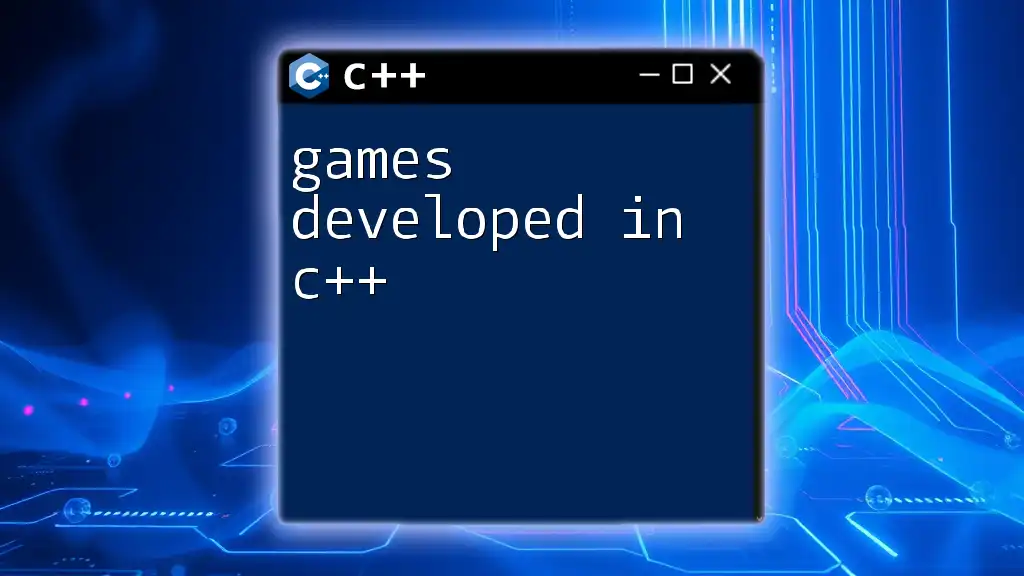
Conclusion
C++ plays an indispensable role in game development, thanks to its performance, efficiency, and adaptability. Understanding the role of C++ in games created with C++ can enhance your game development journey. Whether you are developing a high-octane AAA title or an intimate indie game, leveraging C++ can elevate your project to new heights. If you're eager to dive deeper into game programming, consider joining our company’s learning programs to master C++ effectively and unlock your potential in game development.