.NET provides a framework to develop applications that can interact with C++ code through interoperability, allowing developers to leverage the performance and capabilities of C++ within .NET applications.
Here's a simple code snippet demonstrating how to use C++/CLI to create a .NET application:
using namespace System;
public ref class HelloWorld
{
public:
void Greet()
{
Console::WriteLine("Hello from C++/CLI in .NET!");
}
};
Understanding the Relationship Between C++ and .NET
C++ and .NET are not only two powerful programming tools but also complement each other remarkably well. Understanding their relationship begins with recognizing how C++ fits into the larger .NET ecosystem. When C++ is integrated with .NET, it can leverage the Common Language Runtime (CLR), which allows developers to write code that can run on multiple platforms without being tightly coupled to any specific architecture. This ability to harness the benefits of both worlds is a significant reason for using .NET with C++.
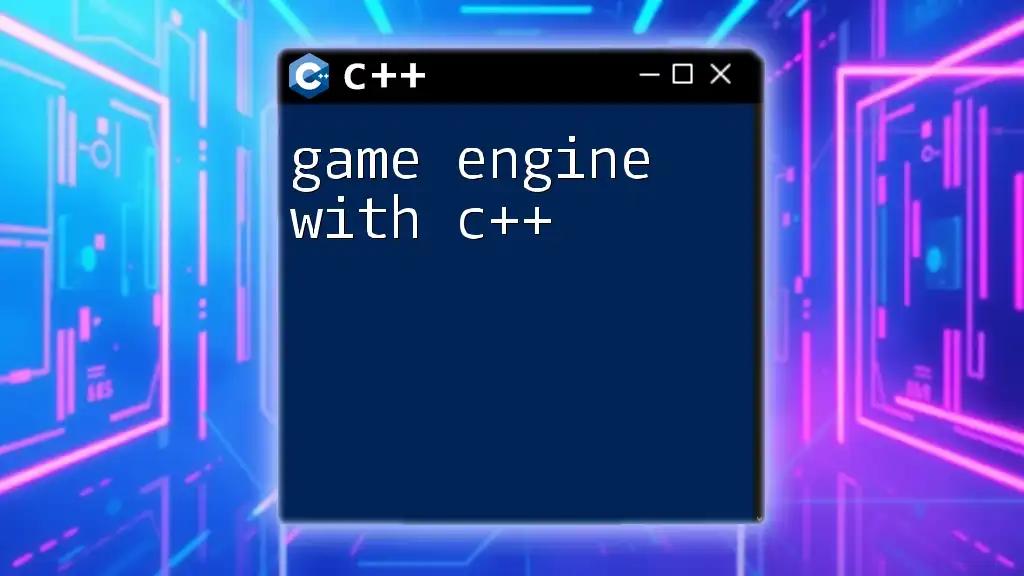
Why Choose C++ for .NET Development?
Choosing C++ for .NET development comes with several advantages.
-
Performance: C++ is renowned for its high performance and efficient resource management. When you're working on performance-critical applications, C++ can provide the speed and efficiency required.
-
Rich Set of Libraries: C++ developers can utilize vast libraries including those for GUI, networking, and database operations while still accessing .NET features, providing flexibility.
-
Interoperability: With C++/CLI, you can easily bridge native C++ code and .NET languages, facilitating a smooth interaction between the two.
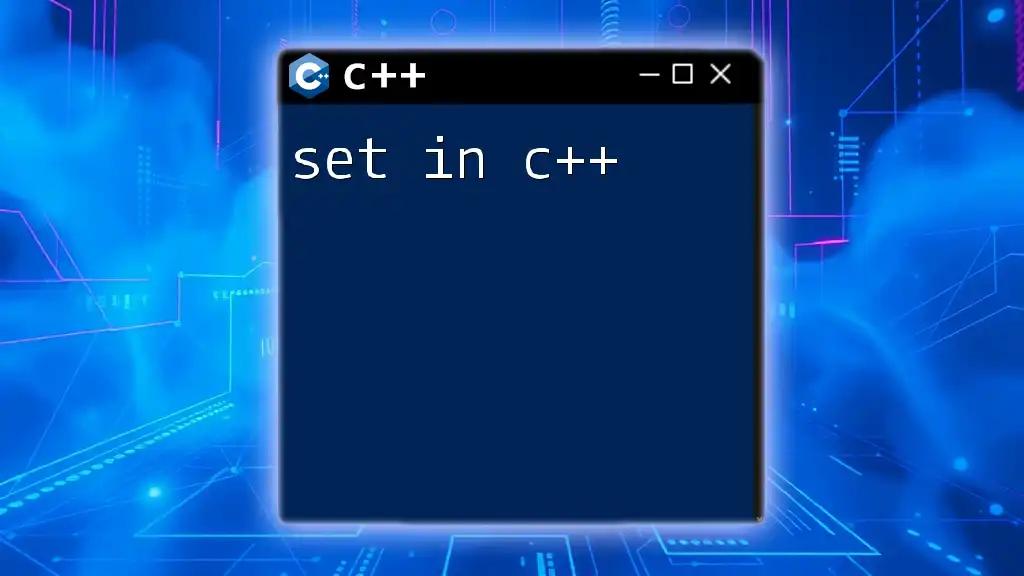
Setting Up Your Development Environment
Selecting the Right Tools
To begin your journey of .NET with C++, you first need to establish a suitable development environment. Visual Studio is the recommended IDE as it offers robust support for C++ development in a .NET context.
-
Installation and Setup: Download Visual Studio from the official website. During the installation, select the workload for "Desktop development with C++". This sets up the necessary tools for C++ projects.
-
Configuration for C++ Development with .NET: Launch Visual Studio, and ensure you have the .NET framework installed. You can alter project settings to target either the managed (.NET) or unmanaged (native) C++ code.
Creating Your First C++ Application in .NET
Creating your foundational C++ application in the .NET ecosystem is straightforward.
- Open Visual Studio and select New Project.
- Choose the CLR Empty Project template under Visual C++ and provide a project name.
Here's a simple "Hello, World!" example to get you started:
#include <iostream>
using namespace System;
int main()
{
Console::WriteLine("Hello, World! Welcome to .NET with C++");
return 0;
}
This code uses the System namespace from .NET to write to the console, showcasing the integration of both languages.
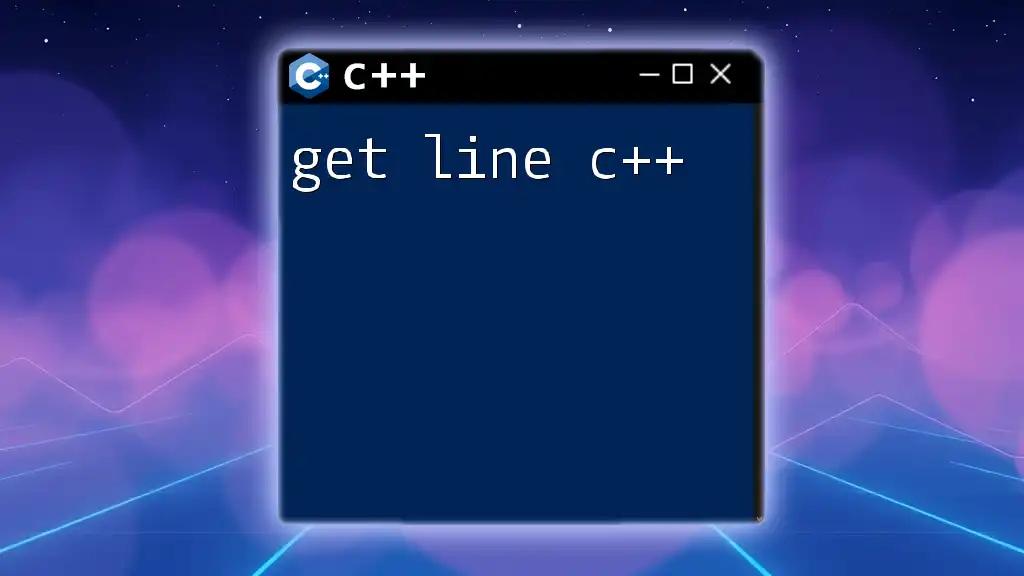
C++ as a Managed Language in .NET
Understanding Managed vs. Unmanaged Code
In the world of programming, it’s essential to differentiate between managed and unmanaged code. Managed code runs under the management of CLR, enabling features like garbage collection, while unmanaged code operates directly with the system hardware.
C++/CLI allows C++ developers to create managed code, allowing easier access to .NET features. This gives C++ programmers an augmented ability to build Windows applications that can leverage .NET libraries seamlessly.
Essential C++ Commands for .NET
When developing applications using .NET with C++, it's crucial to be fluent in key C++ commands that work harmoniously within the .NET framework. Here’s a brief overview:
- System::String: Represents strings in the C++/CLI context, allowing integration with .NET libraries.
- gcnew: A keyword used to allocate managed objects.
For example, using these commands, you can instantiate a string:
String^ myString = gcnew String("This is a managed string in C++/CLI");

C++/CLI: Bridging the Gap
Understanding C++/CLI
C++/CLI (Common Language Infrastructure) bridges native C++ code with the .NET framework. It introduces specific syntax and constructs that facilitate interoperability between managed and unmanaged code.
C++/CLI Syntax and Features
C++/CLI modifies some C++ syntax to enable the creation and management of .NET objects. Below are key features:
- gcnew: Use this syntax to create managed objects.
- ^: This symbol indicates a handle to a managed object.
Here is a code snippet showcasing the creation of managed objects in C++:
ref class MyClass
{
public:
void DisplayMessage()
{
Console::WriteLine("Hello from C++/CLI!");
}
};
int main()
{
MyClass^ obj = gcnew MyClass();
obj->DisplayMessage();
return 0;
}
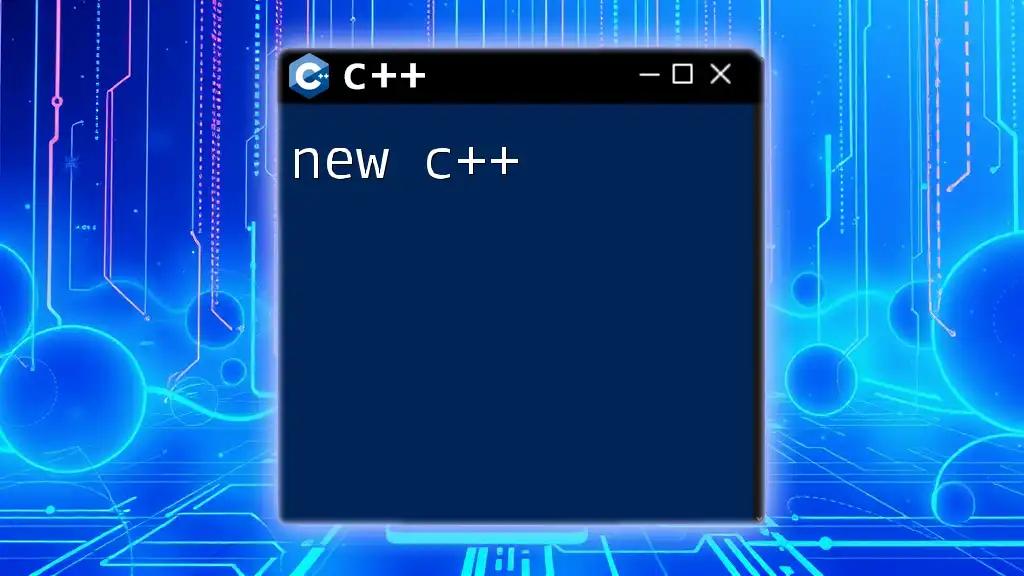
Building Windows Forms Applications with C++
Creating GUI Applications Using C++ in .NET
With .NET with C++, you can build graphical user interfaces (GUIs) using Windows Forms. This framework allows you to create rich client applications with event-driven programming.
Start by creating a new Windows Forms App project in Visual Studio, selecting C++/CLI as the language. Here’s a simple example of a form with a button:
#include <Windows.h>
#include <System.Windows.Forms.h>
using namespace System;
using namespace System::Windows::Forms;
void Main()
{
Application::EnableVisualStyles();
Application::Run(gcnew Form());
}
Handling Events
Event handling is a crucial aspect of GUI programming. Here’s how you can handle a button click event in a Windows Forms application:
private: System::Void button1_Click(System::Object^ sender, System::EventArgs^ e)
{
MessageBox::Show("Button clicked!");
}
Make sure to connect the event with the button by assigning the `button1_Click` function to the button’s Click event in the form’s constructor.
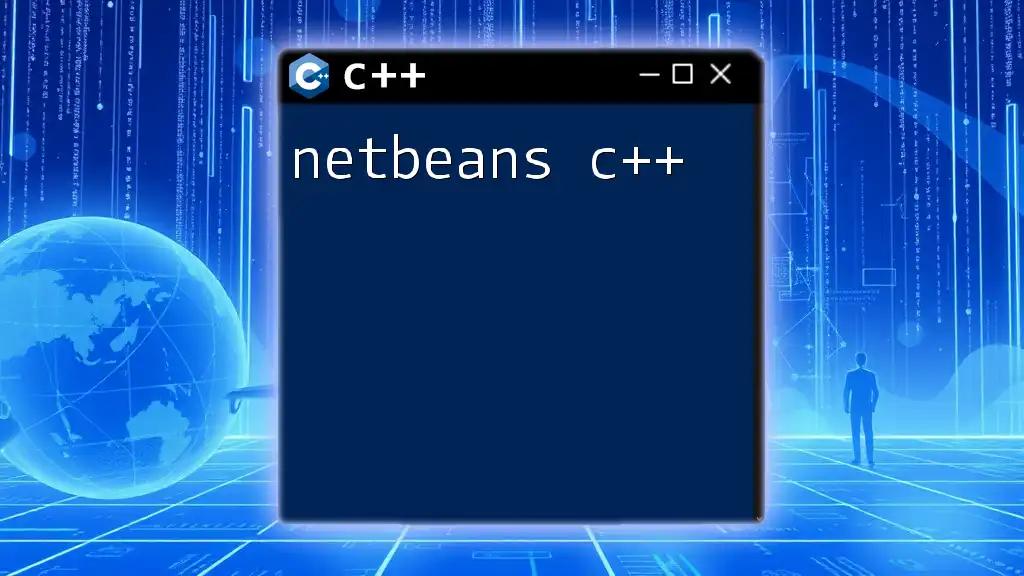
Using C++ Libraries in .NET Applications
Integrating Existing C++ Libraries
When working with .NET with C++, you can incorporate existing unmanaged C++ libraries into your .NET application. This can be particularly beneficial if you have a robust library that performs specific tasks.
Here’s how you can use a C++ library within your project:
- Add a reference to the library in your project settings.
- Create a wrapper in C++/CLI to call functions from the unmanaged library.
Utilizing .NET Libraries in C++
C++ applications can also leverage .NET libraries, offering a rich set of tools and functionalities. From accessing .NET types to utilizing LINQ, the interoperability can significantly enhance your application:
using namespace System;
using namespace System::Collections::Generic;
List<int>^ numbers = gcnew List<int>();
for (int i = 0; i < 10; i++)
{
numbers->Add(i);
}
In this code snippet, we create a List from the System.Collections namespace, showcasing how C++ can access and manipulate .NET library classes.
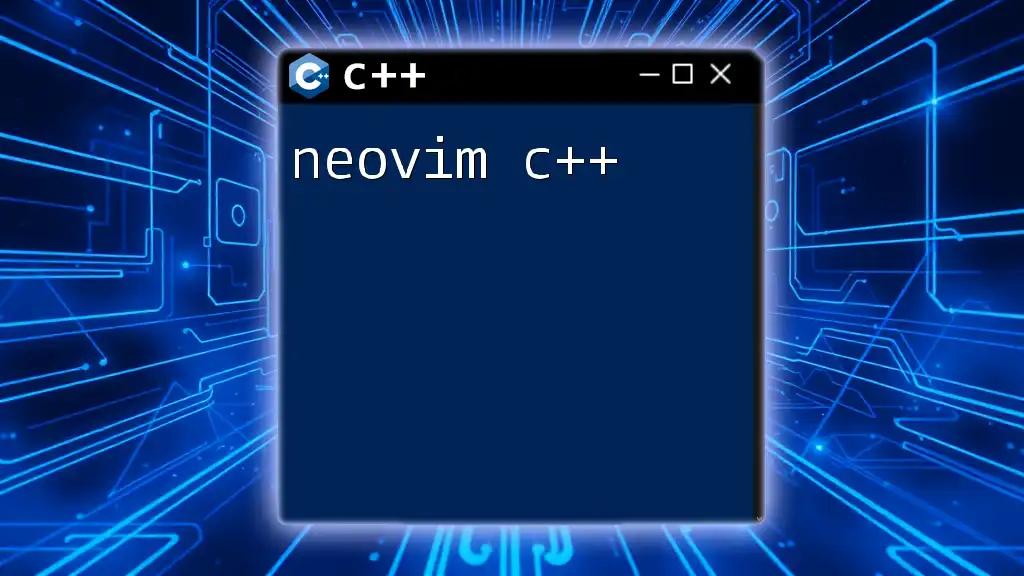
Performance Optimization Techniques
Best Practices for C++ in .NET
Optimizing performance is essential when developing applications with .NET and C++. Here are several best practices:
- Memory Management: Utilize managed and unmanaged resources judiciously to minimize garbage collection overhead.
- Use of automated tools: Leverage the profiling tools available in Visual Studio to identify bottlenecks.
Profiling and Performance Tuning
Profiling your applications can provide insights into performance issues. Use tools like the Visual Studio Profiler to monitor resource usage, identify slow-running functions, and optimize them accordingly.

The Future of C++ in the .NET Ecosystem
As technology continues to evolve, so does the landscape for C++ in the .NET ecosystem. The growing trend towards cross-platform development with .NET 5 and .NET 6 brings new opportunities for C++ developers.
Encouragement to Experiment
The integration of .NET with C++ opens an array of possibilities for developers. As you work on your projects, don't hesitate to experiment with various features, libraries, and frameworks. The more you explore, the more proficient you'll become in utilizing C++ within the expansive .NET framework.

Additional Resources
For anyone looking to delve deeper into .NET with C++, the official Microsoft documentation is an invaluable resource. Engaging with community forums and online support can also provide you with invaluable advice and techniques as you expand your knowledge and skills in this area.