In C++, the `new` operator allocates memory for an integer and returns a pointer to that memory, allowing for dynamic memory management.
Here's a simple code snippet illustrating how to use `new` to create an integer:
int* myInt = new int(42);
Understanding Dynamic Memory Allocation
What is Dynamic Memory Allocation?
Dynamic memory allocation refers to the process of allocating memory at runtime rather than at compile time. Unlike static memory allocation, where the size of memory is fixed, dynamic memory allocation allows the program to request memory as needed, providing flexibility and efficient management of resources.
Why Use Dynamic Memory Allocation?
Dynamic memory allocation offers several advantages:
- Flexibility: You can allocate only as much memory as you need and can adjust the size based on the program's requirements.
- Efficient Use of Memory: It prevents the allocation of more memory than necessary, which can conserve memory resources and enhance performance.
In many cases, dynamic memory allocation is crucial, such as when dealing with data structures like linked lists, trees, or when the size of the data isn't known beforehand.
![Understanding Unsigned Int in C++ [Quick Guide]](/images/posts/u/unsigned-int-cpp.webp)
The `new` Keyword in C++
Introduction to the `new` Keyword
In C++, the `new` keyword is used to allocate memory dynamically. This keyword allows you to create variables or arrays on the heap, which persists until you explicitly deallocate that memory, unlike stack memory that has automatic storage duration.
Syntax of the `new` Command
The basic syntax for using `new` is straightforward:
int *ptr = new int;
In this snippet, we declare a pointer `ptr` and allocate space for a single integer on the heap. When you use `new`, it returns the address of the allocated memory.
For allocating an array, the syntax slightly changes:
int *arr = new int[10];
Here, we allocate memory for an array of 10 integers. The memory remains allocated until it is explicitly freed using `delete`.
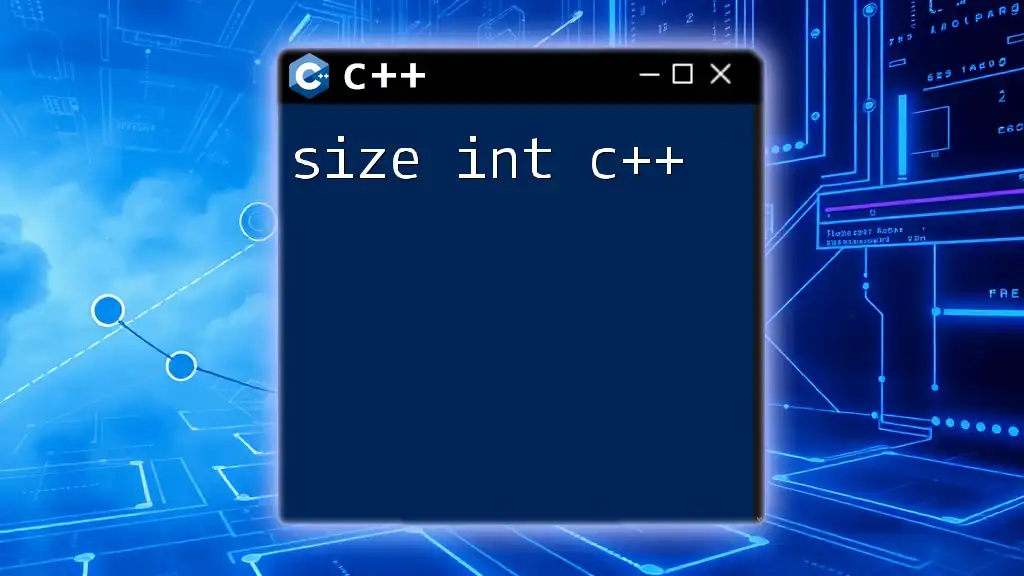
Using the `new` Command Effectively
Allocating Single Variables
Allocating single variables using `new` is efficient when you need a variable that should outlive the function scope or when you need to manage the memory manually. For instance:
int *singleInt = new int(5);
This code snippet not only allocates memory for an integer but also initializes it with the value 5. This demonstrates how `new` can be used both for allocation and initialization at the same time.
Allocating Arrays
Dynamic arrays are often used when the size of the array cannot be determined at compile time. Consider the following code:
int *array = new int[5]{1, 2, 3, 4, 5};
Here, we allocate an array of five integers with initial values provided in braces. This offers great flexibility, especially in scenarios with varying sizes of input.
Best Practices
While using `new`, it's essential to do it responsibly:
- Avoid Memory Leaks: Ensure that every allocation with `new` is paired with a corresponding `delete` operation to free the memory.
- Performance Considerations: Frequent dynamic allocations can lead to fragmentation and decreased performance. It's advisable to consider alternatives like using Standard Template Library (STL) containers which manage memory more efficiently.
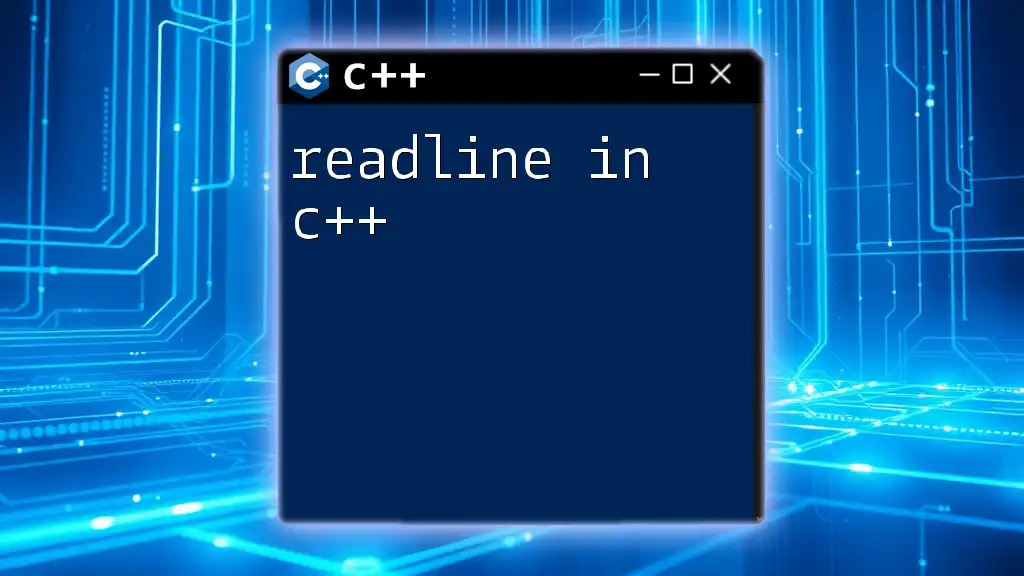
Memory Management with `delete`
Introduction to `delete`
Once memory has been allocated using `new`, you must deallocate it to prevent memory leaks. The `delete` keyword serves this purpose. The syntax for using `delete` for a single variable is:
delete singleInt;
In this case, `singleInt` points to the memory we previously allocated. If we forget to execute this command, that memory will remain allocated until the program terminates.
For arrays allocated using `new`, you must use the following syntax:
delete[] array;
This is crucial because it tells C++ to free the entire block of memory allocated for the array, preventing leaks.
Common Pitfalls
- Memory Leaks: Always audit your code to ensure each `new` has a corresponding `delete` to prevent memory leaks that consume resources.
- Deleting Unallocated Memory: Attempting to delete memory not allocated with `new` results in undefined behavior. Make sure you only call `delete` on pointers that were obtained via `new` or `new[]`.
- Double Deletion: Avoid deleting memory that has already been freed; this can lead to critical errors in your program.
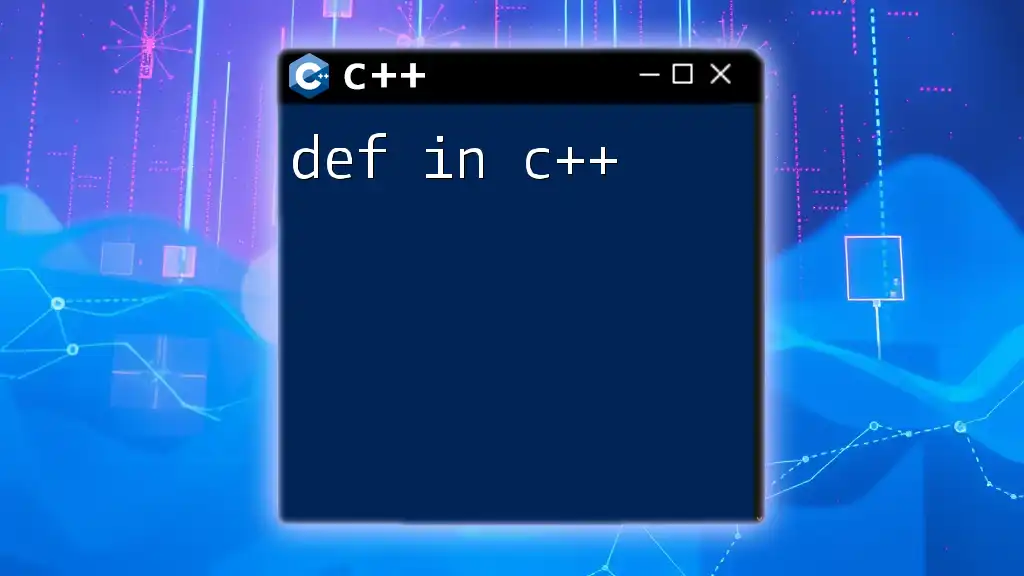
Practical Examples
Real-World Example: Dynamic Array Resizing
In scenarios where you need to resize an array dynamically, here’s an effective way:
#include <iostream>
int main() {
int *arr = new int[5]; // Initial allocation
// Populate and resize
int *temp = new int[10]; // New larger array
for (int i = 0; i < 5; i++) {
temp[i] = arr[i]; // Copy elements
}
delete[] arr; // Free old array
arr = temp; // Point to the new array
// Cleanup
delete[] arr; // Finally deallocate
return 0;
}
In this example, we first allocate an array of 5 integers. As we encounter the need to resize it to 10 integers, we create a new array, copy the existing elements, and delete the old array. This reflects typical use in dynamic memory management.
Real-World Example: Using `new` in Classes
When managing memory within class objects, using `new` is vastly beneficial:
class Sample {
public:
int *data;
Sample(int value) {
data = new int(value); // Allocate memory during construction
}
~Sample() {
delete data; // Deallocate in the destructor
}
};
This example shows a class called `Sample` that dynamically allocates memory for an integer in its constructor. It also implements a destructor to ensure that the allocated memory is released when an object of the class goes out of scope. This encapsulation is vital for building robust software.
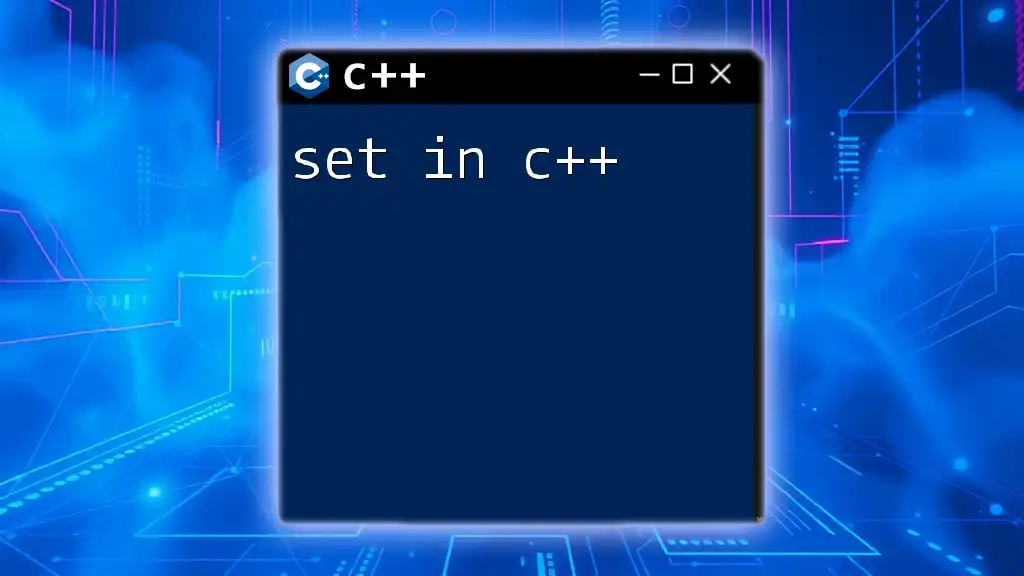
Conclusion
Understanding how to use `new` for dynamic memory allocation in C++ is crucial for effective memory management. By mastering the use of this keyword, alongside robust practices for deallocation and memory handling, you can enhance your C++ programming skills significantly. Memory management is a fundamental aspect of writing efficient and predictable code in C++.
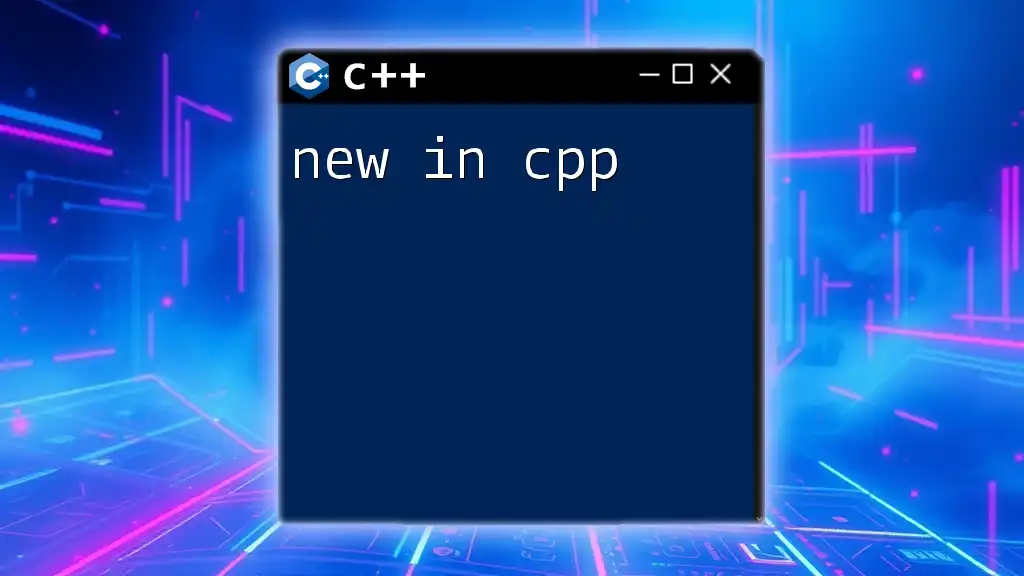
Additional Resources
For further reading, consider exploring more about C++ memory management through tutorials, books, or online courses that dive deeper into best practices for using `new` and managing dynamic memory effectively.
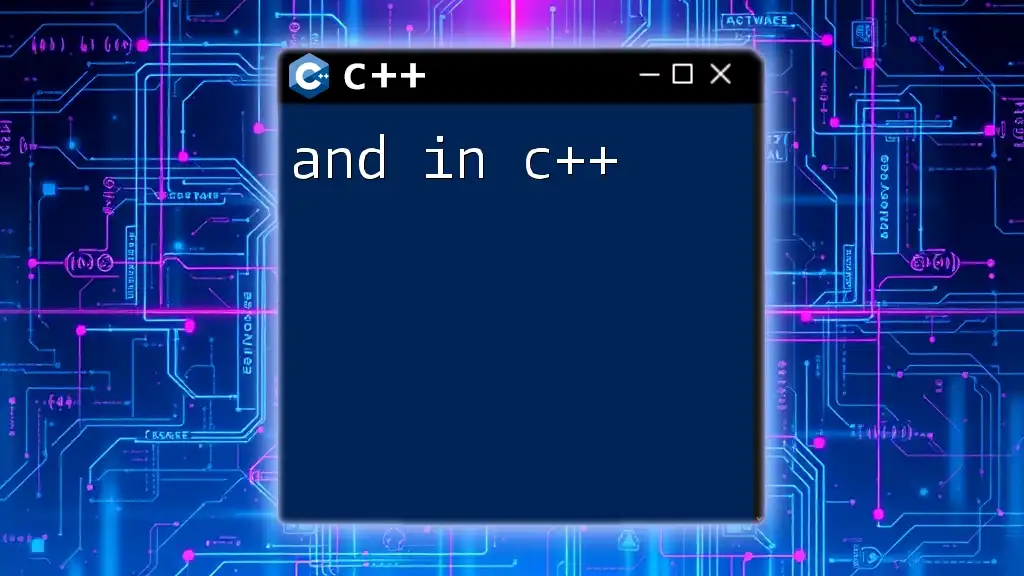
Call to Action
Try implementing dynamic memory allocation in your next C++ project and see how it can optimize your code. Feel free to share your experiences or questions in the comments section; sharing knowledge is key to mastering programming!