Game making with C++ involves leveraging the language's powerful performance and flexibility to create dynamic game environments and interactive experiences.
Here’s a simple example of how to create a basic game loop in C++:
#include <iostream>
int main() {
bool isRunning = true;
while (isRunning) {
std::cout << "Game is running..." << std::endl;
// Add game logic here
// For demonstration purposes, let's stop the loop after one iteration
isRunning = false;
}
return 0;
}
What is Game Development?
Game development is the intricate process of creating video games that span various entertainment mediums, encompassing everything from mobile applications to extensive gaming consoles. It integrates multiple disciplines, including programming, game design, art, and sound design, making it a multifaceted field. The importance of game development is reflected in the booming industry that generates billions in revenue each year, captivating audiences worldwide.
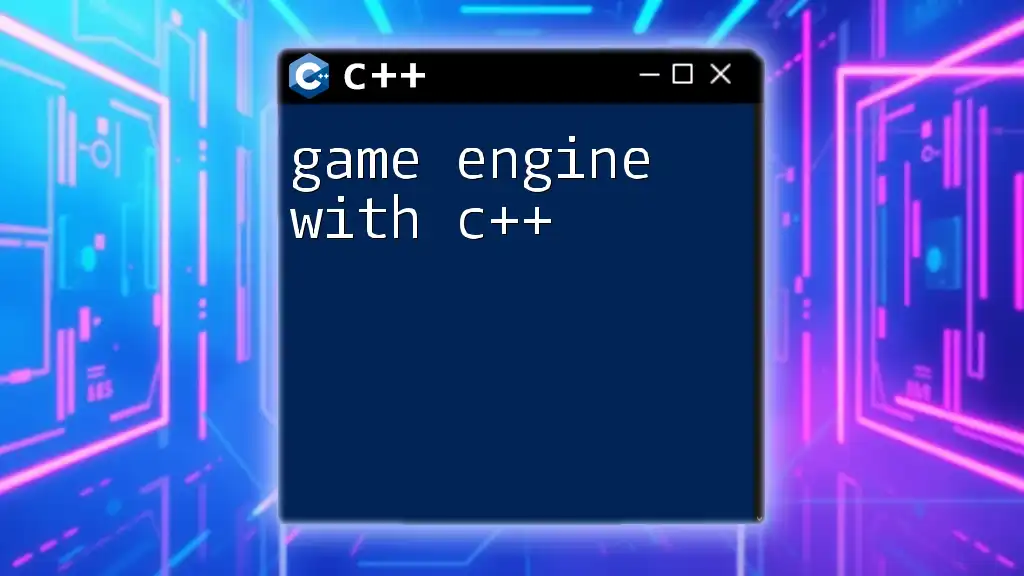
Why Choose C++ for Game Development?
C++ has earned its reputation as a powerhouse in the game development realm. Its performance advantages stem from its lower-level capabilities, allowing for fine-tuning and optimization of complex systems, which is essential for resource-intensive gaming applications. Many high-performance game engines, such as Unreal Engine, leverage C++ for these reasons, solidifying its status in the industry.
Unlike higher-level languages that may abstract away crucial system interactions, C++ gives developers direct access to memory management, enabling faster execution times—an indispensable feature for real-time applications like games. Furthermore, being familiar with C++ opens pathways to understanding game development in other environments, thereby enhancing overall versatility.
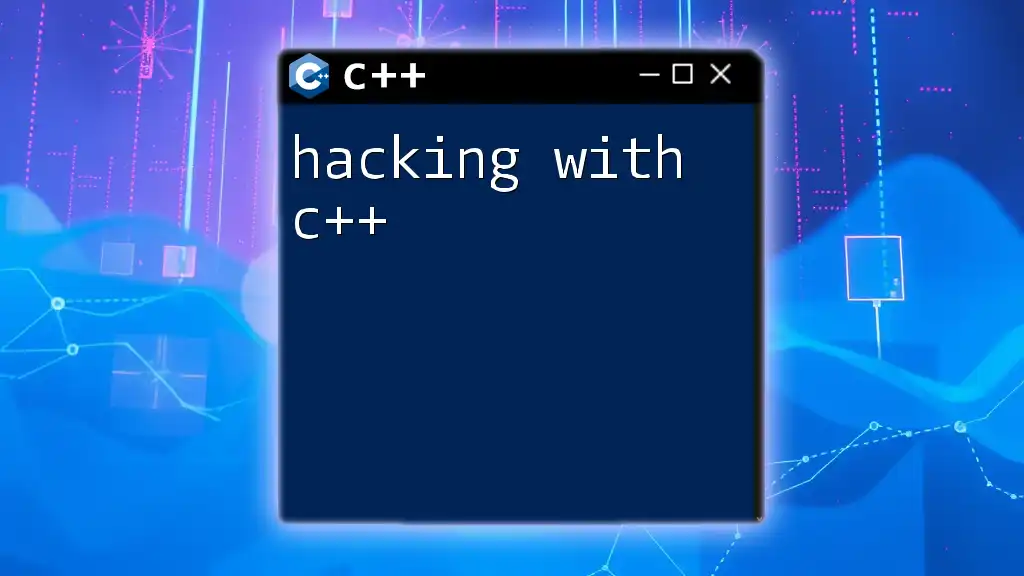
Getting Started with C++
Setting Up Your Development Environment
Before diving into the world of game making with C++, you need a robust development environment. Some widely recommended Integrated Development Environments (IDEs) include:
- Visual Studio: A powerful IDE that is often favored for its debugging tools and support.
- Code::Blocks: An open-source IDE that is lightweight and easy to use.
Once you have your chosen IDE installed, you might have to set up necessary libraries. For instance, installing SDL (Simple DirectMedia Layer) or SFML (Simple and Fast Multimedia Library) will help in handling graphics, sound, and input.
Basic C++ Syntax for Game Development
Understanding C++ syntax is foundational as you embark on your game development journey. C++ employs a rich set of built-in data types, such as `int`, `float`, and `char`. The following example highlights variable declaration, a crucial component for managing game states and properties:
int score = 0;
float playerSpeed = 5.0f;
char playerDirection = 'N'; // North
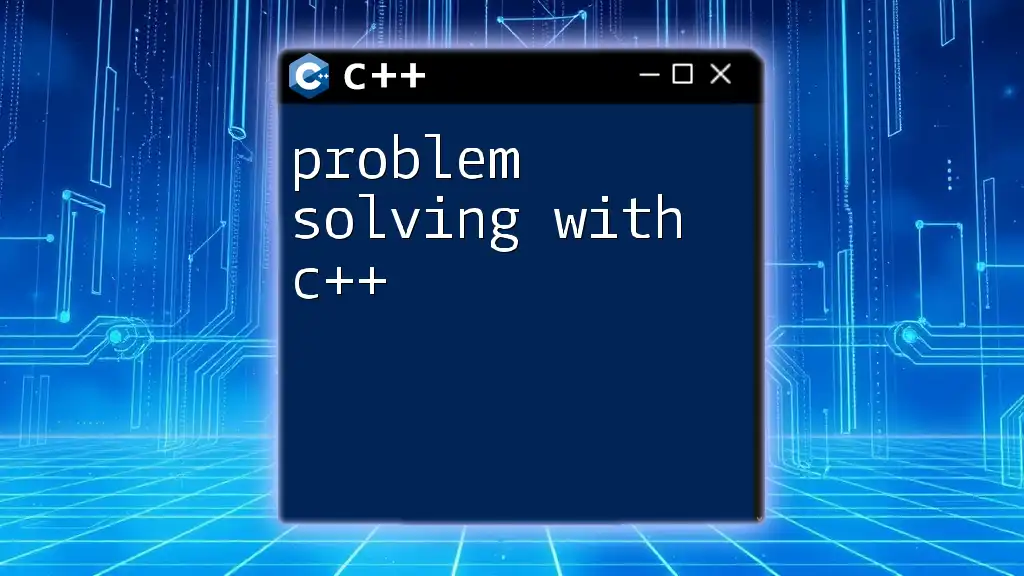
Game Development Concepts
Understanding Game Loops
At the heart of every game lies the game loop. This is a continual cycle responsible for processing input, updating game states, and rendering graphics. Without a well-functioning game loop, games would fail to respond to player actions or render properly.
A basic game loop can be structured like this:
while (gameRunning) {
processInput();
updateGameState();
render();
}
In this structure:
- processInput() handles player input.
- updateGameState() alters the game state based on inputs or events.
- render() is responsible for drawing the game visuals.
Introduction to Game Physics
In the realm of game development, physics plays a vital role in crafting realistic gameplay experiences. Physics in games mimics real-world mechanics like gravity, movement, and collisions. For a simple implementation, you can apply basic principles such as:
- Gravity: Implementing constant downward acceleration to simulate falling objects.
- Friction: Implementing resistance when objects move across surfaces.
Managing Game Assets
Every game is enriched with assets like textures, sounds, and fonts. Managing these assets effectively is key to an immersive gaming experience. To load a texture using SFML, the following code can be used:
sf::Texture texture;
if (!texture.loadFromFile("image.png")) {
// Handle error
}
This snippet demonstrates how to load a texture asset while also handling potential errors—an essential practice in ensuring robustness in your game.
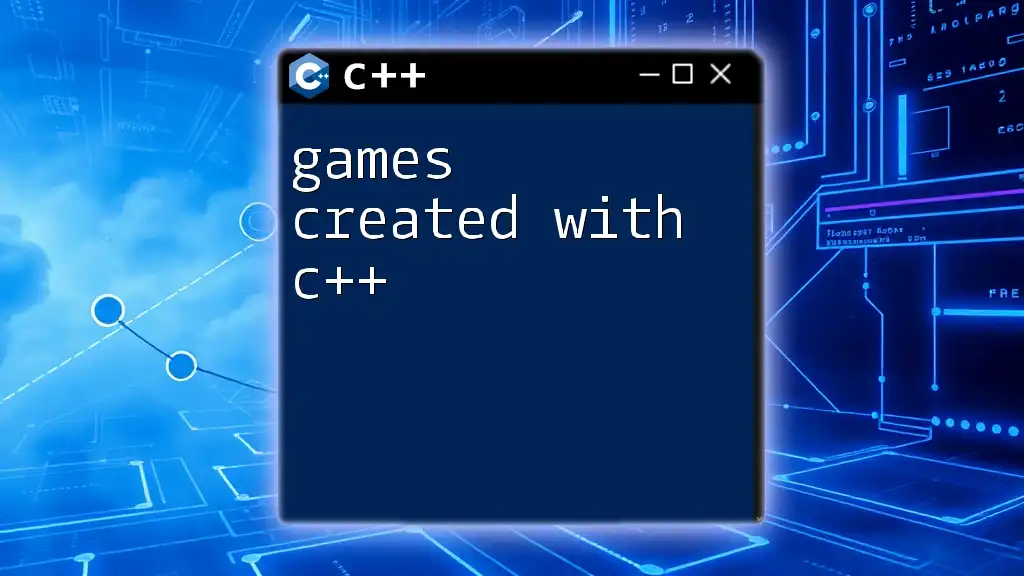
Game Development Frameworks
Overview of Popular Game Engines Using C++
Several game engines are based on C++, notably Unreal Engine and Godot. These engines provide robust tools and functionalities that streamline the game development process, allowing developers to create immersive experiences without reinventing the wheel.
Getting Started with SFML (Simple and Fast Multimedia Library)
SFML is an excellent choice for beginners wanting to learn game making with C++. It provides a straightforward API for handling graphics, audio, and input. Setting up your first SFML project requires installing the library and linking it within your IDE.
Example: Creating a Window with SFML
The initial step in any game is to create a window for rendering graphics. Here’s a simple code snippet to create a window using SFML:
sf::RenderWindow window(sf::VideoMode(800, 600), "My Game");
// Main loop
while (window.isOpen()) {
// Event processing and rendering
}
This code initializes a window of size 800 by 600 pixels, essential for rendering your game world.
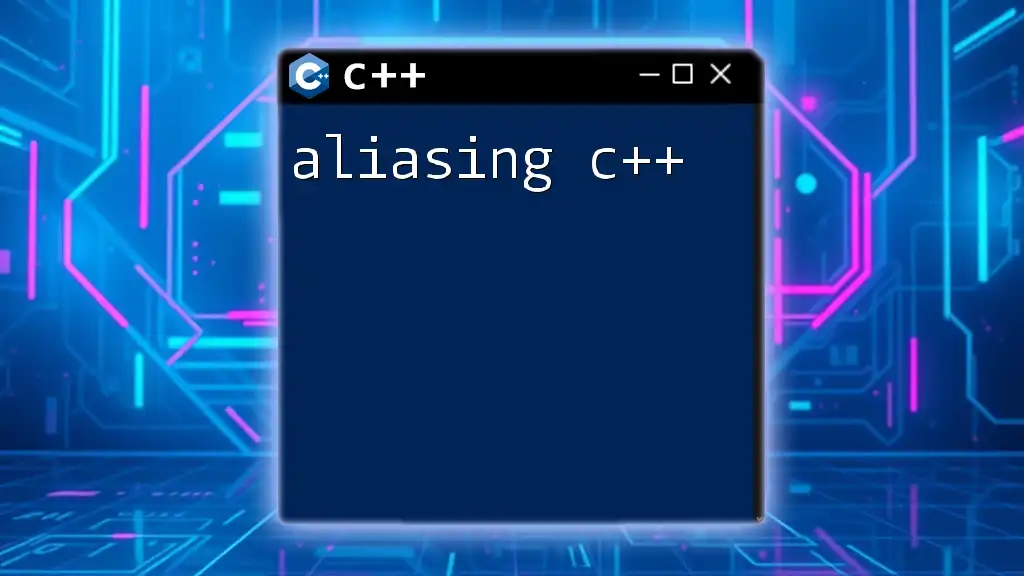
Implementing Game Mechanics
Character Movement
Character movement is a cornerstone mechanic in nearly all games. Implementing this can be achieved with keyboard input, as shown in the following example:
if (sf::Keyboard::isKeyPressed(sf::Keyboard::Left)) {
character.move(-playerSpeed, 0);
}
This code moves the character left when the left key is pressed, allowing players to navigate the game space intuitively.
Collision Detection
Collision detection is crucial for determining when two game entities interact. Simple bounding box collision detection can be easily implemented. Here’s how:
if (player.getGlobalBounds().intersects(enemy.getGlobalBounds())) {
// Handle collision
}
This snippet checks if the player's bounding box intersects with that of an enemy, a fundamental aspect of game strategies.
Game States
Games often feature several states—such as a main menu, gameplay, and pause screens. Managing these states requires a structure that allows switching among them smoothly. Using enums can simplify state management by providing clear labels for each state.
enum class GameState {
Menu,
Playing,
Paused
};
GameState currentState = GameState::Menu;
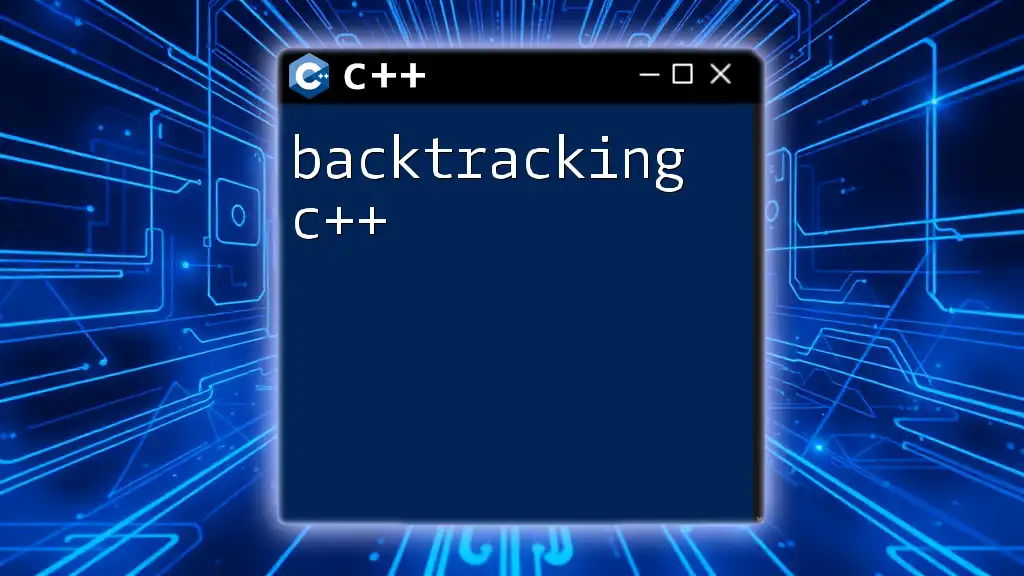
Enhancing Your Game
Adding Sound and Music
To elevate your game's atmosphere, adding sound effects and music is a vital component. Utilizing libraries such as FMOD or OpenAL can significantly enhance the audio experience in your game. Here’s a simple example of how to play sound using SFML:
sf::SoundBuffer buffer;
buffer.loadFromFile("sound.wav");
sf::Sound sound;
sound.setBuffer(buffer);
sound.play();
This demonstrates how you can load and play a sound file, enhancing the player's experience through audio feedback.
Creating Levels and Environments
Level design is an artistically driven process that involves creating compelling, immersive worlds for players to explore. Tips for effective level design include:
- Start small: Focus on creating a single level and expand from there.
- Use intuitive layouts: Ensure that the environment guides players through exploration naturally.
Optimizing Game Performance
Performance optimization is crucial in game development, especially as complexity increases. Common bottlenecks include excessive memory use and inefficient algorithms. Employing techniques such as memory management and optimizing data structures can lead to smoother gameplay.
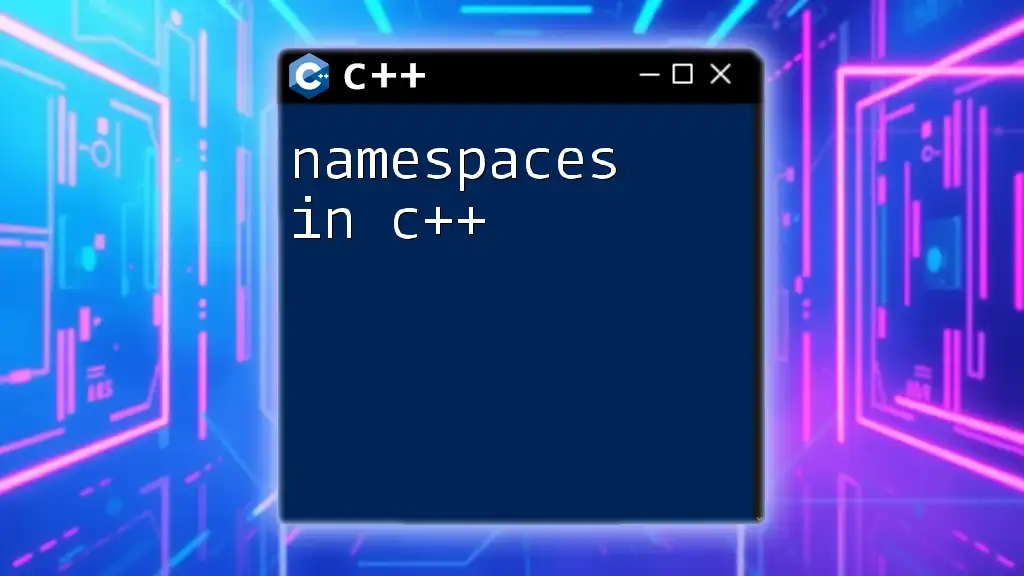
Final Steps in Game Development
Testing Your Game
Testing is a critical phase in game development, ensuring quality and functionality. Implement a systematic approach to testing, covering aspects such as:
- Unit tests for individual components,
- Playtesting for user experience feedback.
Publishing Your Game
Once your game is polished and tested, publishing becomes the next step. You can consider platforms like Steam, Itch.io, or even mobile application stores, each with its own submission guidelines and audiences.
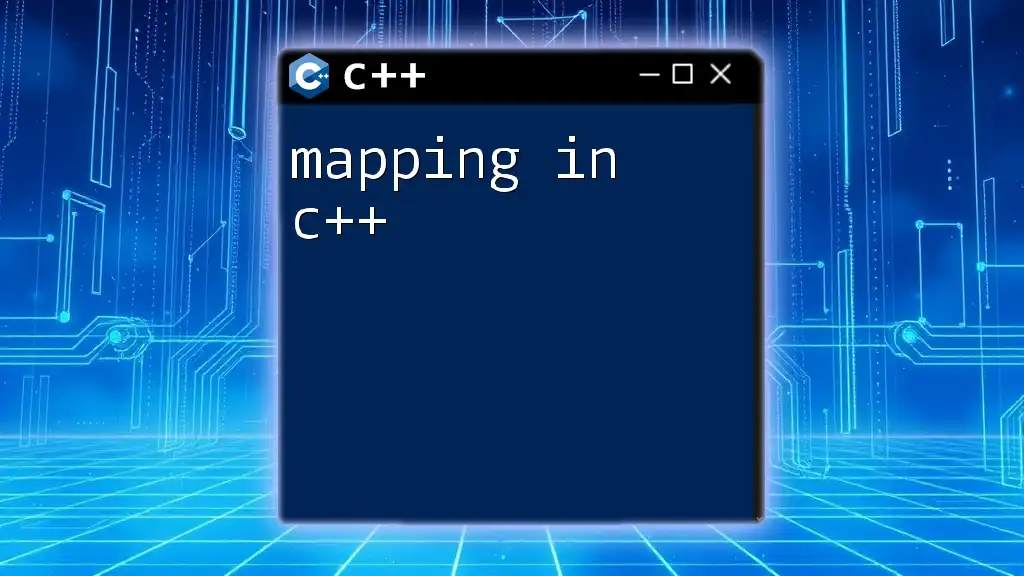
Conclusion
The Future of Game Development with C++
As technology continues to advance, so does the landscape of game development. Trends such as virtual reality (VR), augmented reality (AR), and AI-driven game mechanics are shaping future opportunities. Staying updated with these movements is crucial for any aspiring game developer.
Encouragement to Create Your Own Game
The best way to learn game development is by doing. Use C++ to harness your creativity, trial error, and bring your ideas to life. Start small, expand your skills, and don't be afraid to experiment. Your journey into game making with C++ begins now!
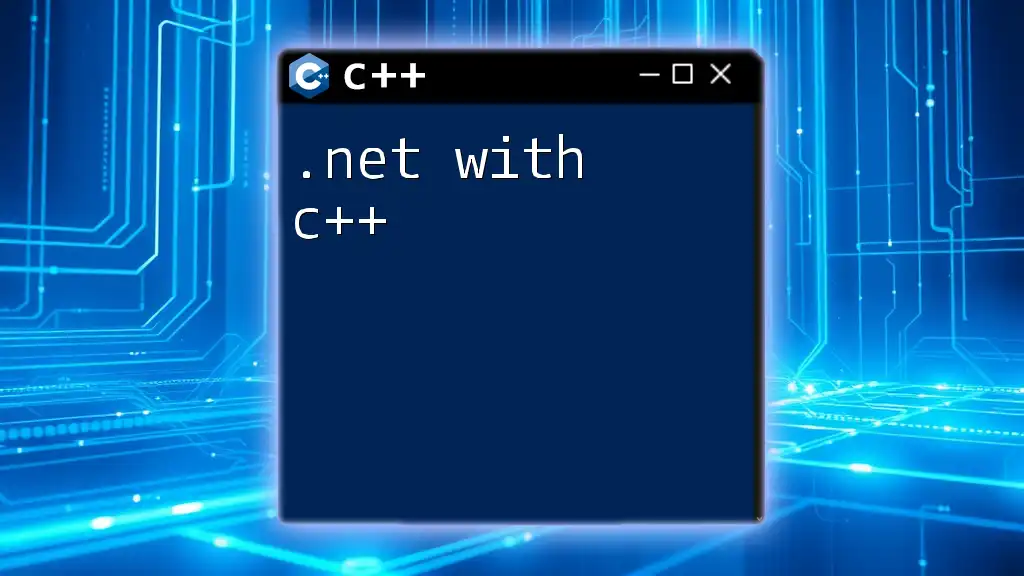
Additional Resources
Recommended Books and Online Courses
There are numerous educational resources available to enhance your knowledge in game development. Explore online courses in platforms like Coursera or Udemy, and consider reading specialized books that delve deeper into specific topics.
Community and Forums
Engaging with the game development community can be invaluable, providing support, feedback, and collaboration opportunities. Participate in forums like GameDev.net or Stack Overflow, and harness the shared knowledge of fellow developers.