Creating a simple game in C++ can be achieved by utilizing basic programming constructs to set up a game loop and handle user input, as demonstrated in the following example that implements a basic text-based guess-the-number game:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0));
int number = std::rand() % 100 + 1;
int guess = 0;
std::cout << "Guess the number (1-100): ";
while (guess != number) {
std::cin >> guess;
if (guess < number) std::cout << "Too low! Try again: ";
else if (guess > number) std::cout << "Too high! Try again: ";
else std::cout << "Congratulations! You guessed it!" << std::endl;
}
return 0;
}
Getting Started with C++
Setting Up Your Development Environment
Before diving into the world of game development, setting up the appropriate development environment is crucial. Here are the steps to get started:
- Choose an Integrated Development Environment (IDE): Popular choices include Visual Studio, CLion, and Code::Blocks. Each of these IDEs provides tools that simplify coding, compiling, and debugging.
- Install a C++ Compiler: Compilers like g++ or those bundled with your chosen IDE will allow you to run and test your code. Make sure that your installation paths are configured correctly.
- Install Recommended Libraries: Libraries like SDL (Simple DirectMedia Layer) or SFML (Simple and Fast Multimedia Library) streamline tasks such as graphics rendering, sound output, and user input handling. For advanced projects, consider using Unreal Engine, which is built on C++.
Basic C++ Concepts for Game Development
To effectively create a game with C++, you must grasp fundamental C++ concepts. Familiarity with the following aspects is imperative:
- Syntax and Structure: C++ codes begin with `#include` directives followed by the `main()` function, which serves as the entry point of the program.
- Variables, Loops, Functions, and Classes: Understanding these concepts helps in organizing the game logic and state.
For instance, consider this simple code snippet that prints a welcoming message:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, Game Development!" << endl;
return 0;
}
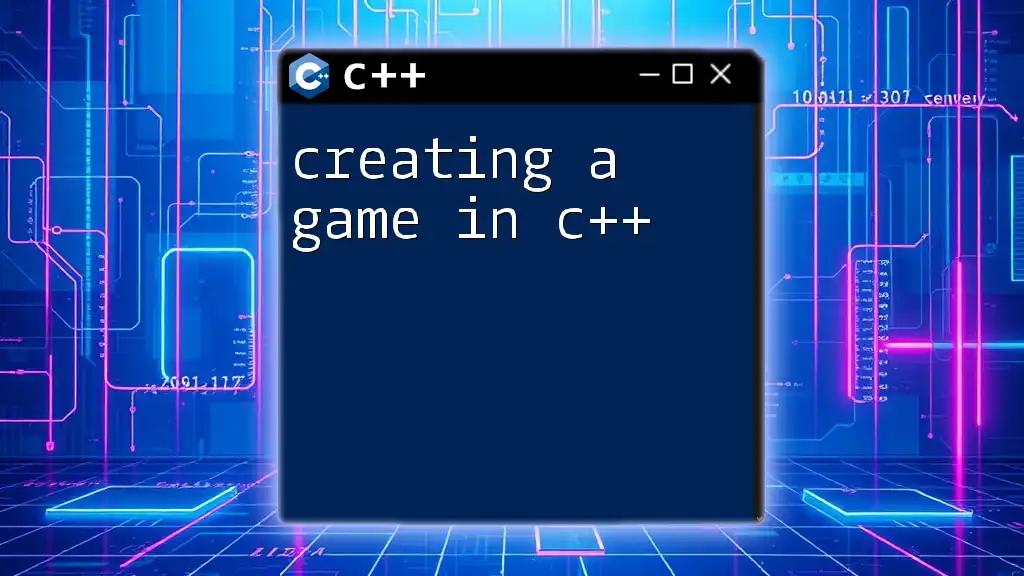
Designing Your Game
Game Concept and Storyboarding
Every successful game begins with a strong concept. Think about your target audience and desired gameplay experience. Develop a storyboard to outline the game's narrative flow and main events. This step ensures clarity in your design process.
Basic Design Principles
When crafting your game, focus on the following design principles:
- Game Mechanics: Define the rules that govern gameplay. This includes game objectives and player actions.
- Level Design: Create engaging levels that incrementally challenge the player.
- Character and Environment Design: Consider aesthetics, character abilities, and interactive elements that enhance the player's immersion.
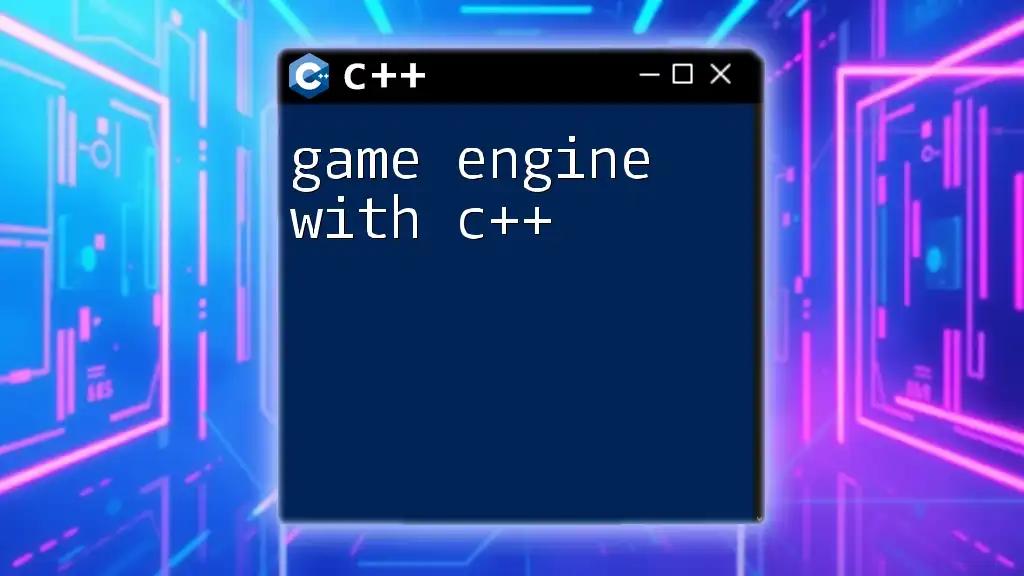
Developing Your Game
Setting Up a Simple Game Project
Establish a clear project structure by organizing files and resources. This includes directories for assets (images, sounds, etc.), source code, and libraries.
Implementing Game Mechanics
The game loop is central to any game. It continuously runs the game logic, processes user input, updates game state, and renders graphics. Here’s how it generally looks:
while (gameIsRunning) {
processInput();
updateGame();
renderGame();
}
Creating Game Objects
Utilizing classes and objects is essential for structured game development. Define your game entities as classes that encapsulate their properties and behaviors.
For example, a basic player class could look like this:
class Player {
public:
void move(int x, int y);
private:
int posX, posY;
};
Handling User Input
Capturing user input is key for interactive gameplay. Implement keyboard and mouse controls effectively to allow players to maneuver through your game.
Here’s a simple keyboard input example:
if (keyPressed("W")) {
player.move(0, -1);
}
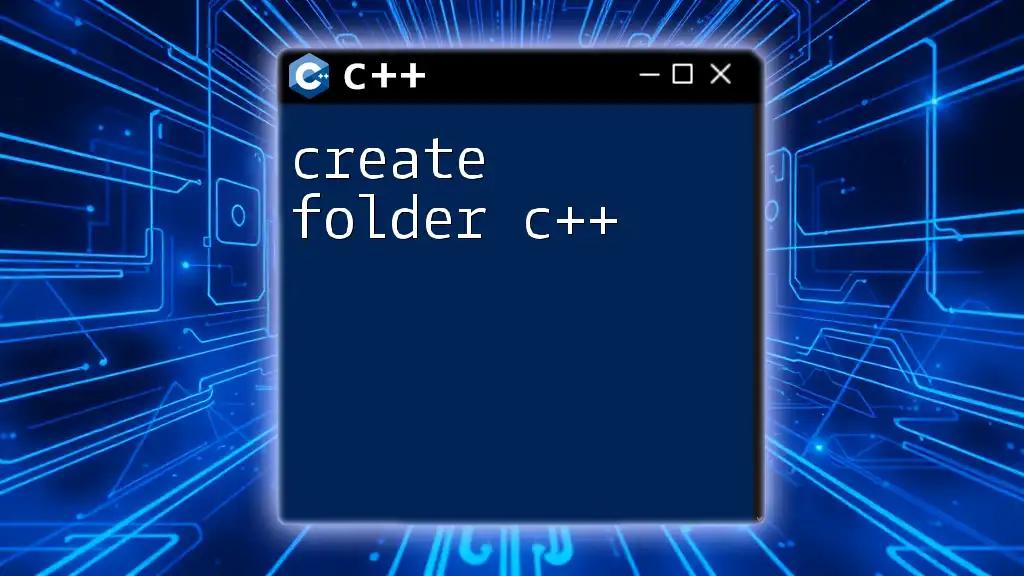
Adding Graphics and Sound
Integrating Graphics with a Library
Games rely heavily on graphics for visualization. Libraries like SDL or SFML provide easy-to-use functions for loading images and rendering graphics. Load an image into your game as follows:
SDL_RenderCopy(renderer, texture, NULL, &destRect);
Implementing Sound Effects and Music
To enhance the gaming experience, incorporate sound effects and background music. Libraries such as FMOD or OpenAL make audio integration straightforward. Here’s an example of how to play a sound effect:
Mix_PlayChannel(-1, soundEffect, 0);
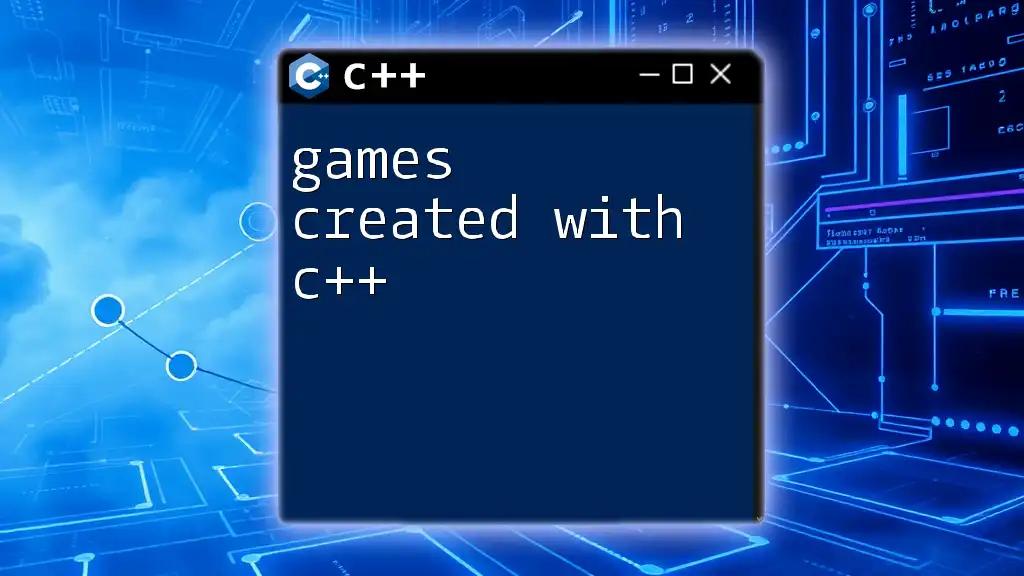
Testing and Debugging
Strategies for Effective Testing
Testing is essential in ensuring your game functions as intended. Adopt a systematic approach to bug tracking and incorporate testing at every stage of development.
Common Pitfalls in Game Development
Beware of potential pitfalls, including performance issues and memory leaks. Tools like Valgrind help you monitor your application's memory usage, tracking down leaks and improving efficiency.
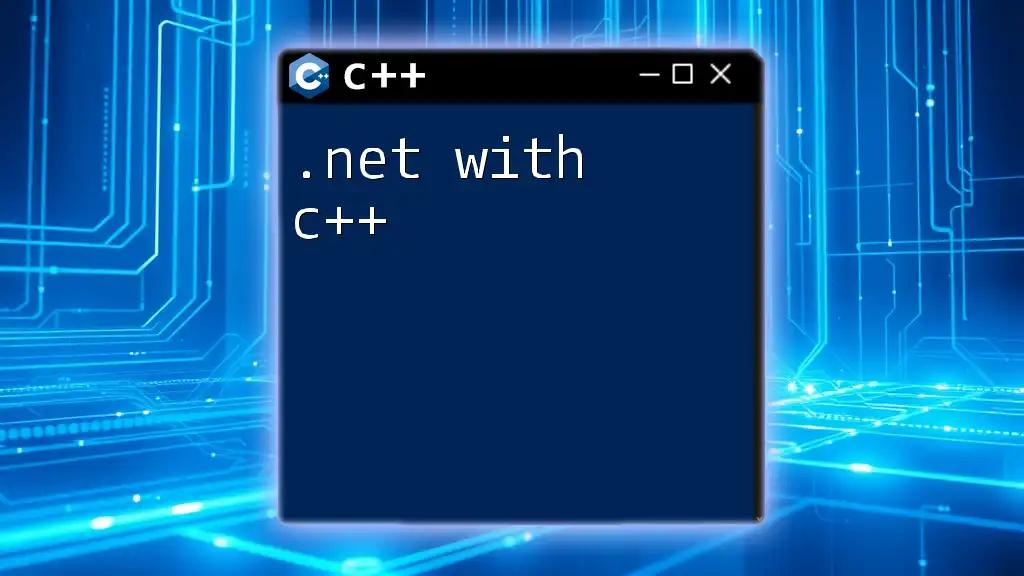
Packaging and Distribution
Compiling Your Game
Before distributing your game, compile it into executable files. Configure your build process to ensure your game runs smoothly across different systems.
Distributing Your Game
Platform choice plays a significant role in getting your game to the audience. Popular options include Steam and itch.io. Ensure you follow platform-specific guidelines for submitting and sharing your game effectively.
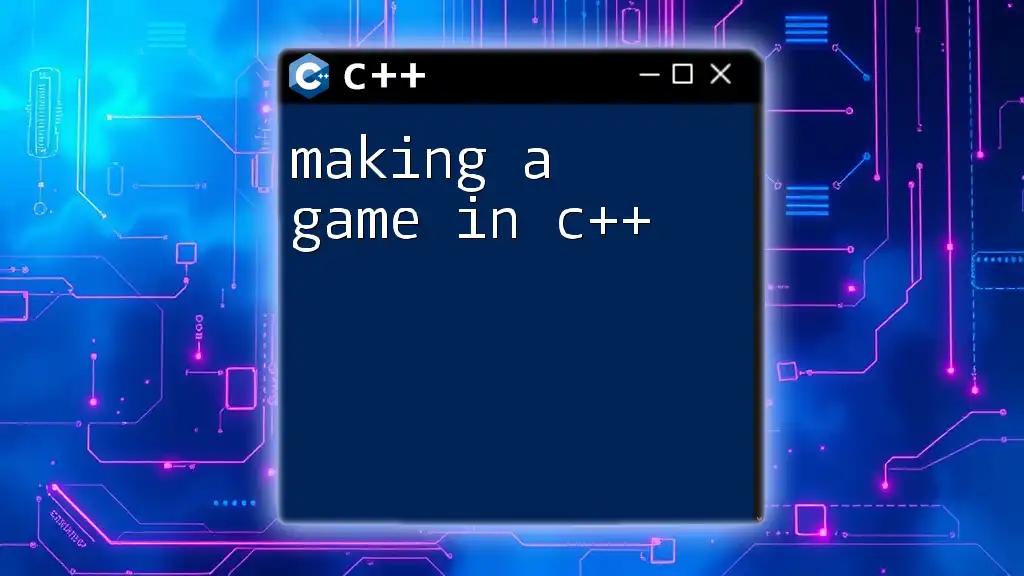
Conclusion
Creating a game with C++ can be both an exciting and challenging journey. From setting up your environment to implementing game mechanics, each step contributes to your growth as a developer. Embrace the learning process, and don’t hesitate to explore additional resources to further your skills.

Additional Resources
For continued learning, look into these resources:
- Books on C++ Game Development: Insightful for deepening your understanding.
- Online Courses: Platforms like Udemy or Coursera offer structured learning paths.
- Game Development Communities: Engage with groups on forums or social media to share insights, seek feedback, and network with other developers.
By following these guidelines and keeping a curious mindset, you're well on your way to successfully creating your first game with C++. Happy coding!