Creating games in C++ involves leveraging its powerful performance and flexibility to develop engaging applications, as illustrated by this simple code snippet that sets up a basic game loop:
#include <iostream>
#include <cstdlib>
int main() {
bool running = true;
while (running) {
std::cout << "Game is running...\n";
// Game logic here
// For demonstration, we exit the loop after one iteration
running = false;
}
return 0;
}
Getting Started with C++
C++ Basics
C++ is a powerful programming language renowned for its performance, flexibility, and control over system resources. It combines high-level language features with low-level programming capabilities, making it an ideal choice for game development. Understanding concepts like object-oriented programming, memory management, and polymorphism is crucial for anyone interested in creating games in C++.
Setting Up Your Environment
Before diving into game development, setting up the right environment is crucial. Choose an Integrated Development Environment (IDE) that suits your style and preferences. Popular options include:
- Visual Studio: A robust IDE with comprehensive support for C++ projects.
- Code::Blocks: A lightweight, cross-platform IDE known for its simplicity.
After selecting your IDE, you’ll need to install necessary libraries. Two popular graphics libraries for game development are SFML (Simple and Fast Multimedia Library) and SDL (Simple DirectMedia Layer). These libraries provide essential functionalities, such as handling graphics, sound, and input.
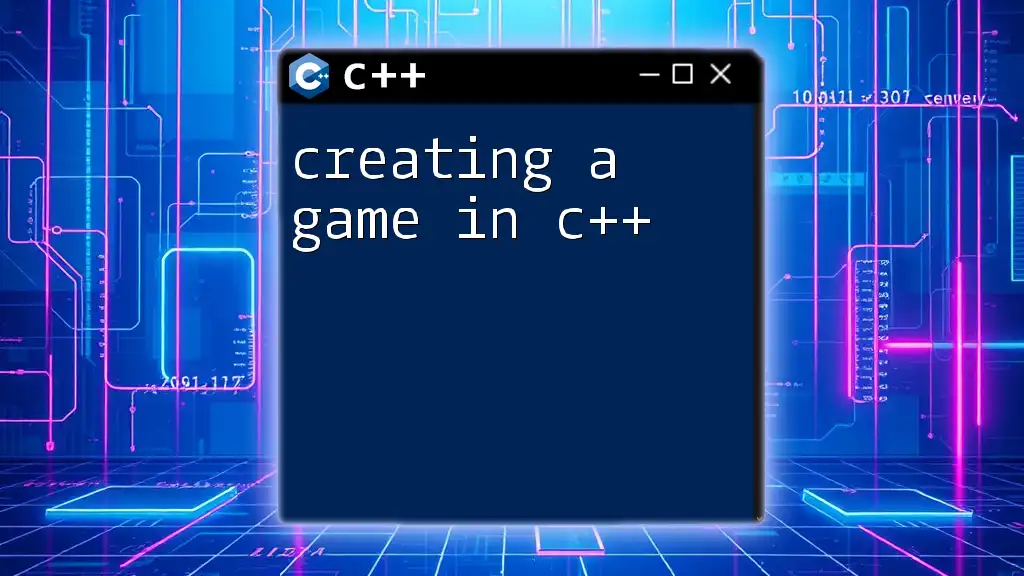
Understanding Game Development Fundamentals
Game Development Frameworks and Engines
While it’s possible to start coding a game from scratch in C++, many developers choose to leverage game engines and frameworks. Unreal Engine is a widely used game engine that supports C++ coding, providing features like physics, rendering, and animation out of the box. Alternatively, if you prefer more hands-on control, frameworks like SFML or SDL give you the tools to create your own engine or game structure from the ground up.
Game Loop Concept
One of the most integral components of game development is the game loop. This loop continuously updates the game state and renders graphics on the screen. A basic game loop consists of three primary functions: processInput, updateGame, and renderGame.
Here’s a simple C++ code snippet demonstrating a game loop:
while (gameRunning) {
processInput();
updateGame();
renderGame();
}
- processInput handles user interactions.
- updateGame refreshes the game state based on input and game rules.
- renderGame draws everything on the screen, reflecting the updated game state.
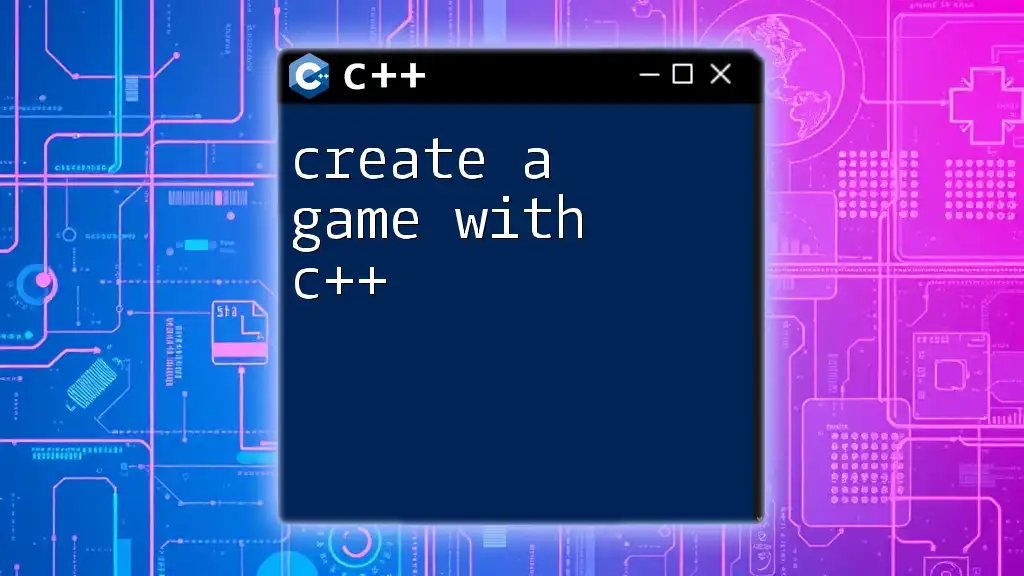
Step-by-Step Guide to Creating a Simple Game in C++
Idea Conception
Before jumping into coding, you should take some time to brainstorm potential game ideas. The simpler the game, the easier it will be to manage within a development cycle. A classic example is creating Pong or Tic-Tac-Toe, which teaches essential programming constructs while allowing for creativity in design.
Designing Your Game
Once you have a game idea, outline the key mechanics and flow of the game. Create flowcharts or diagrams to visualize the game's logic and how different components interact with one another.
Coding the Game
Setting Up the Project
Project organization plays a vital role in maintaining project structure and readability. Create a directory structure with separate folders for assets (graphics, sounds), source code, and documentation.
Basic Game Structure in C++
The main entry point of your game can be structured very simply. Here’s an example of a basic structure in `main.cpp`:
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to My C++ Game!" << endl;
// Game initialization code here
return 0;
}
This code initializes your terminal output, welcoming players to the game. From here, you can layer in more functionality as your game structure develops.
Creating Game Entities
Game entities are the backbone of your game, representing players, enemies, obstacles, and other interactive elements. You can define a simple `Player` class as shown below:
class Player {
public:
int x, y; // Player's position
void move(int dx, int dy) {
x += dx;
y += dy;
}
};
This class encapsulates player behavior and attributes, making it easier to manage multiple instances and interactions.
Implementing Game Logic
Handling Input
User input is crucial for interactive gameplay. You need to capture keyboard and mouse events efficiently. Here's an example of how to handle keyboard input:
if (keyPressed(KEY_UP)) {
player.move(0, -1);
}
By checking which keys are pressed, you can modify the state of your game accordingly.
Updating Game State
Proper state management is vital in maintaining a smooth user experience. The update function will implement rules, such as boundary checks and score updates, which keep the gameplay engaging and functional.
Rendering Graphics
Incorporating graphics is essential for any modern game. Using SFML or SDL, you can easily draw on the screen. Here's how to use SFML to render a player object:
window.draw(player);
The rendering system updates the game’s visual output frame by frame, allowing players to perceive movement and changes effectively.
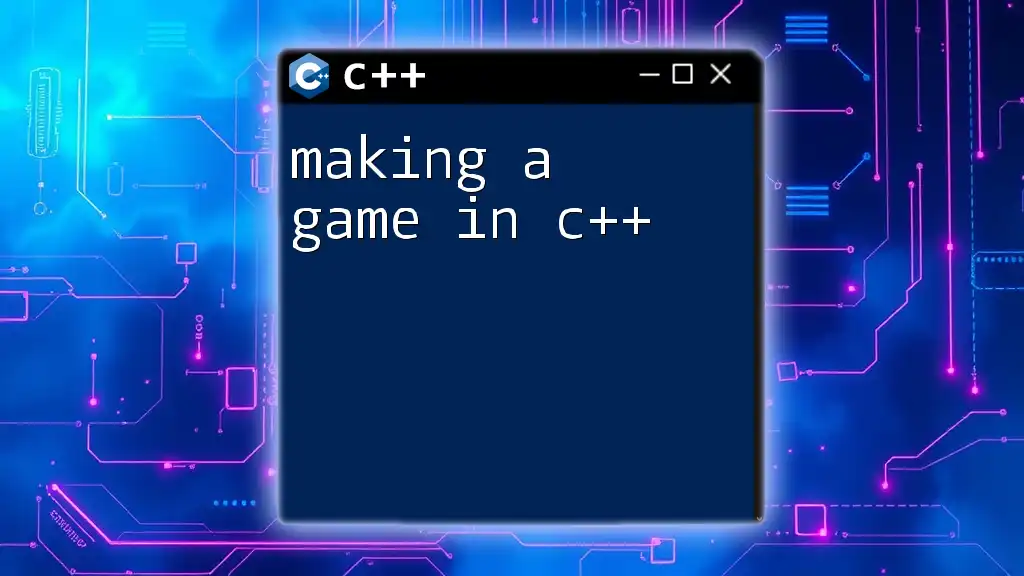
Sound and Music Integration
Incorporating Audio Effects
Sound can greatly enhance the gaming experience, providing feedback and atmosphere. Integrating audio using a library like SFML is straightforward. Here’s an example of how to add sound effects:
sf::SoundBuffer buffer;
buffer.loadFromFile("sound.wav");
sf::Sound sound(buffer);
sound.play();
Proper use of audio can immerse players further into the world you've created, making it feel more alive.
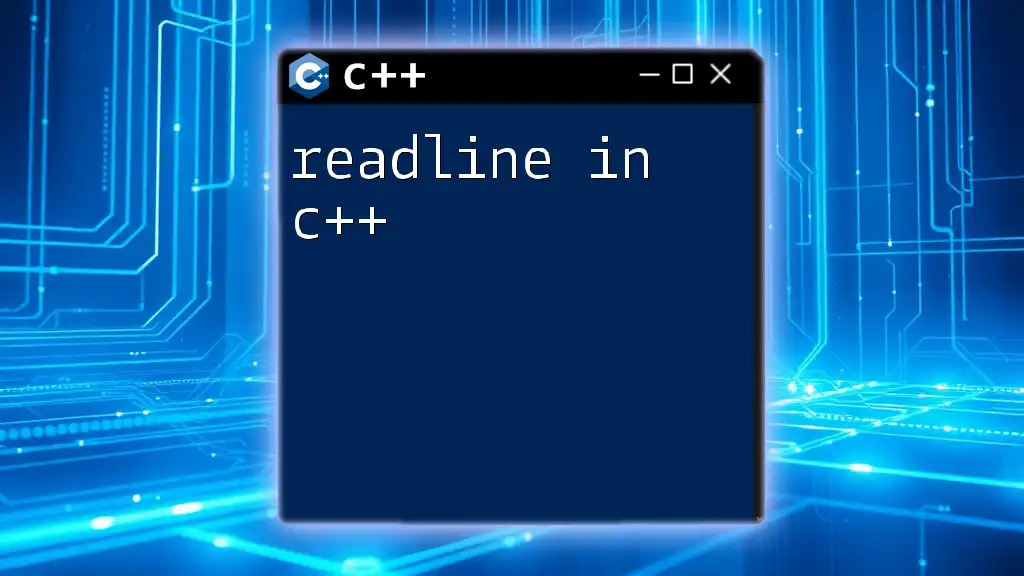
Testing and Debugging Your Game
Testing Strategies
Testing is vital in game development; it ensures the game functions as intended. Playtesting provides insight into gameplay mechanics and areas needing improvement.
Debugging Techniques
Utilizing debugging tools within your IDE can significantly simplify the identification of issues. Here’s a common issue you might face, along with how to handle it:
if (player.x < 0) { // Check for out of bounds
player.x = 0; // Fix the position
}
This snippet ensures the player cannot move out of the game boundaries, enhancing gameplay experience.
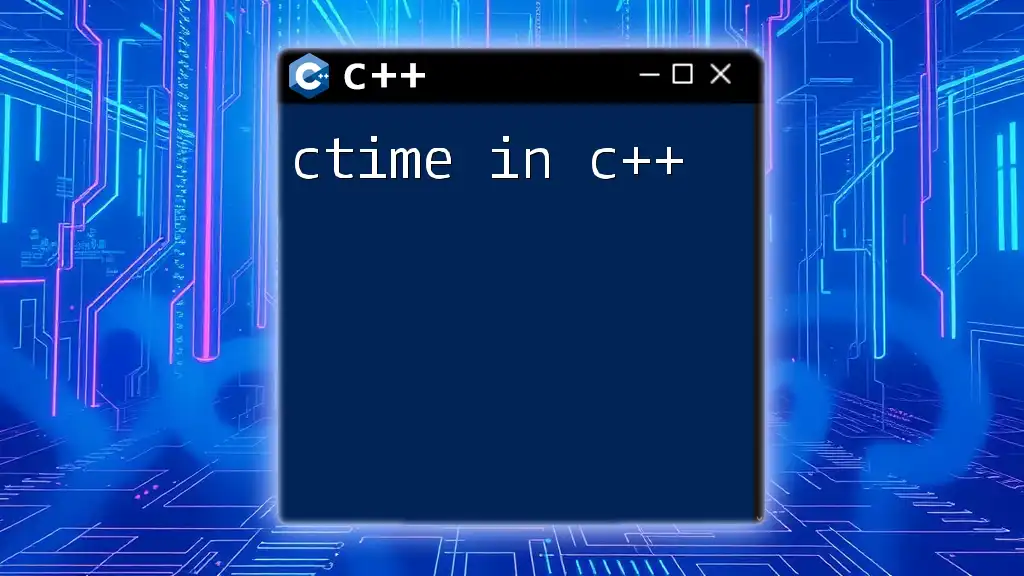
Polishing and Finalizing Your Game
Adding Finishing Touches
Once the core functionalities are in place, focus on polishing your game. Consider adding visual elements to enhance the user interface (UI), polishing graphics, and refining gameplay mechanics. These details can make a significant difference in player satisfaction.
Building the Game for Distribution
After finalizing your game, it's time to compile it for different platforms. Make sure to test on each platform to ensure a consistent experience. Several distribution platforms, such as Steam and itch.io, are excellent channels for publishing your game.
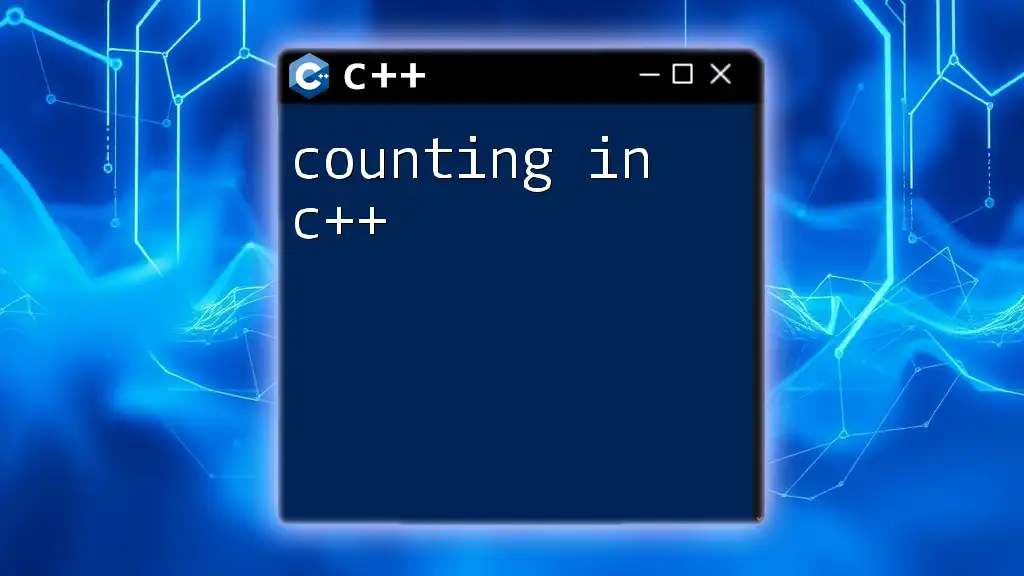
Conclusion
Creating games in C++ can be an exciting and rewarding venture. By understanding the fundamentals, setting up a proper development environment, and applying structured coding practices, you can bring your game ideas to life. The possibilities are endless, and with perseverance and creativity, you can develop compelling game experiences that resonate with players. Continue to explore, learn, and practice, as the world of game development is vast and ever-evolving.
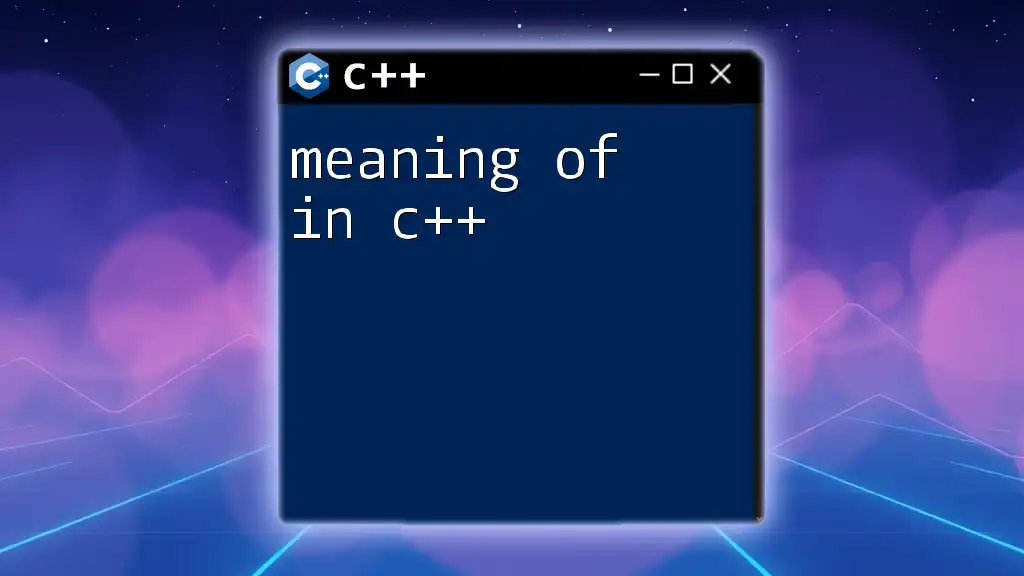
Additional Resources
To deepen your understanding of C++ game development, consider exploring the following resources: recommended books, online communities, and sample code repositories on platforms like GitHub. Engaging with other developers can provide invaluable insights and inspiration as you embark on this journey.