In C++, an object is a user-defined data type that combines data and functions that operate on that data, encapsulating both into a single entity.
Here’s a simple example:
#include <iostream>
using namespace std;
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog; // Creating an object of the Dog class
myDog.bark(); // Calling the bark function on the object
return 0;
}
What is an Object in C++?
An object in C++ is a foundational component of Object-Oriented Programming (OOP), which is a programming paradigm that organizes software design around data, or objects, rather than functions and logic. An object can be defined as an instance of a class, encompassing state and behavior.
Key Characteristics of Objects
-
State (Attributes): This refers to the data stored in an object, representing its properties. In the context of a class, attributes are typically declared as variables.
-
Behavior (Methods): This represents the actions that can be performed by the object. Methods are functions defined within the class that allow interaction with the object's state.
What is an Object in C++: A Deeper Look
Objects are meant to represent real-world entities and concepts, encapsulating both data and functions that manipulate that data. For instance, consider a `Car` class. Each `Car` object would have specific attributes like `color`, `model`, and `year`, also encapsulating behaviors like `drive()` and `stop()`.
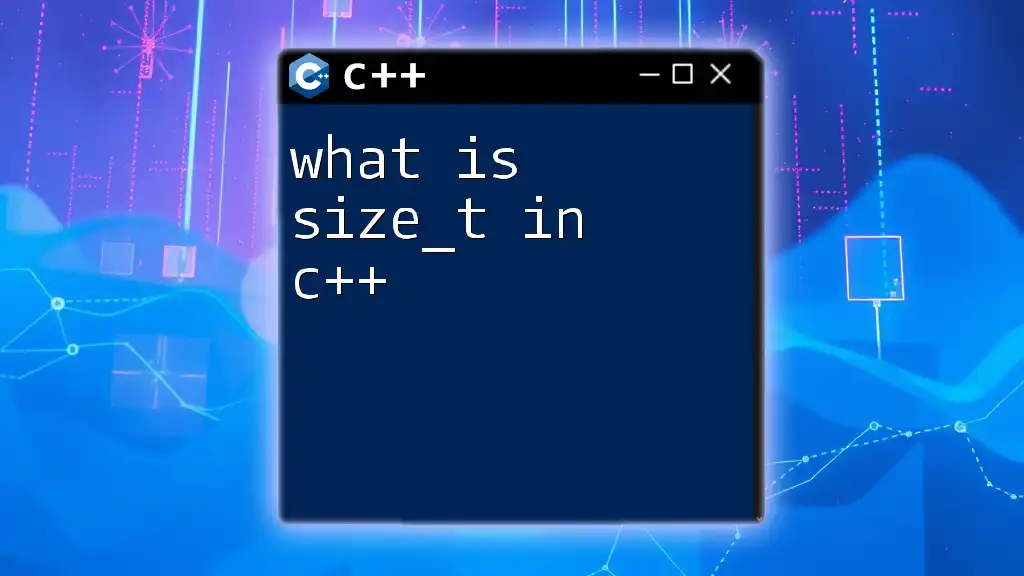
Understanding Classes and Objects
What is a Class?
In C++, a class is a blueprint for creating objects. It defines variables and functions that specify the properties and behaviors of the objects that can be instantiated. The syntax for defining a class is straightforward:
class Dog {
public:
string breed;
void bark() {
cout << "Woof!" << endl;
}
};
In this example, the `Dog` class has an attribute (`breed`) and a method (`bark()`) that represents its behavior.
Creating Objects from Classes
Once a class is defined, you can create objects (instances) of that class.
Dog myDog;
myDog.breed = "Labrador";
myDog.bark(); // Output: Woof!
Here, `myDog` is an object of the `Dog` class, and we are able to set its `breed` attribute as well as call its `bark()` method.
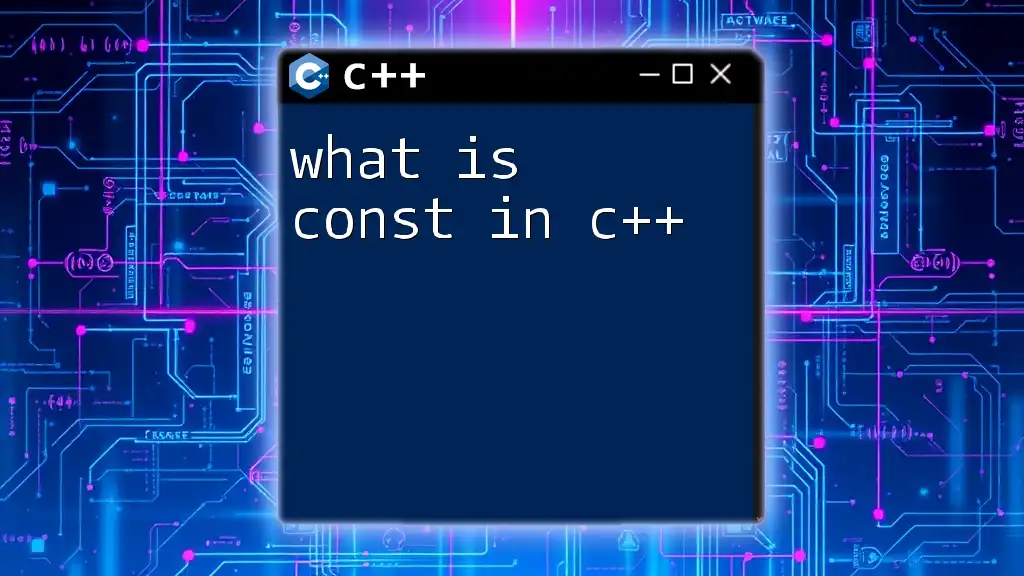
The Significance of Objects in C++
Objects in C++ provide several benefits, leveraging the principles of OOP.
Encapsulation
Encapsulation is a concept that restricts direct access to some of the object's components, which can prevent unintended interference and misuse. This is done through the use of access specifiers: `public`, `private`, and `protected`.
Consider the following example:
class Employee {
private:
string name; // Cannot be accessed directly outside this class
public:
void setName(string empName) {
name = empName;
}
string getName() {
return name;
}
};
Here, the `name` attribute is private, protecting it from external modifications, while public methods allow controlled access to it.
Inheritance
Inheritance allows a class (the child class) to inherit the attributes and methods from another class (the parent class). This promotes code reuse.
class Animal {
public:
void eat() {
cout << "Eating..." << endl;
}
};
class Cat : public Animal {
public:
void meow() {
cout << "Meow!" << endl;
}
};
The `Cat` class is inheriting from the `Animal` class, allowing it to access the `eat()` method while also incorporating its specific behavior with the `meow()` method.
Polymorphism
Polymorphism enables objects to be treated as instances of their parent class, allowing for method overriding. This provides flexibility in invoking methods.
class Shape {
public:
virtual void draw() {
cout << "Drawing Shape" << endl;
}
};
class Circle : public Shape {
public:
void draw() override {
cout << "Drawing Circle" << endl;
}
};
In this example, the `draw()` method in the `Shape` class is overridden in the `Circle` class. When you call `draw()` on a `Circle` object, the overridden method is executed, demonstrating polymorphism in action.
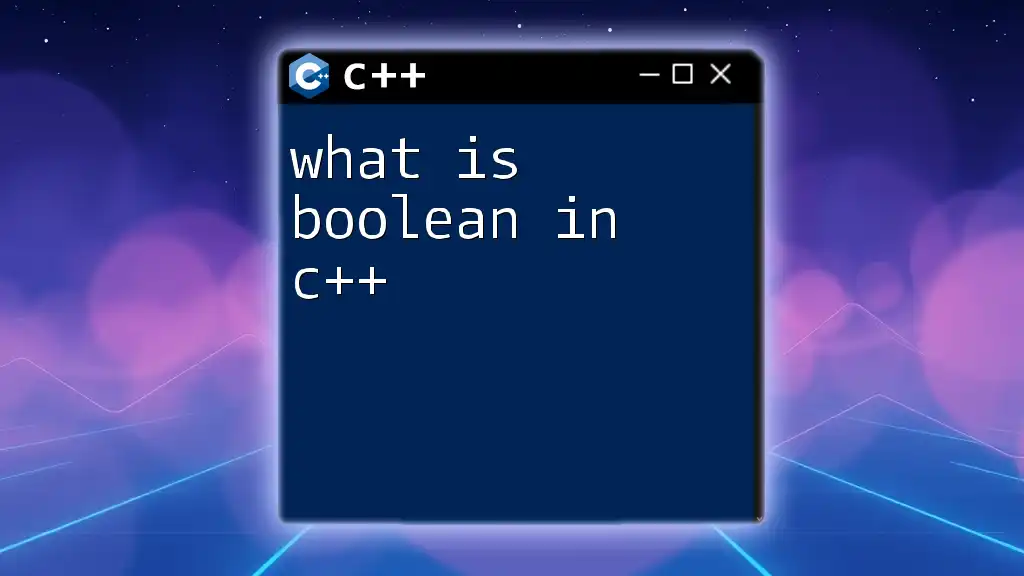
The Benefits of Using Objects in C++
Modularity
Objects allow developers to break down complex systems into smaller, manageable modules. Each object can be designed to perform a specific function or set of functions, making the code modular and easier to maintain.
Code Reusability
Once a class is defined, it can be reused to create multiple objects without having to rewrite the same code. This not only saves time but also helps ensure consistency across the application. For instance, you can create several `Dog` objects without redefining the `Dog` class.
Flexibility
Object-oriented programming enhances flexibility. With classes and objects, you can easily update parts of your code without affecting the rest. For instance, if you wanted to add a new method to the `Dog` class, it would not require significant changes elsewhere in your code.
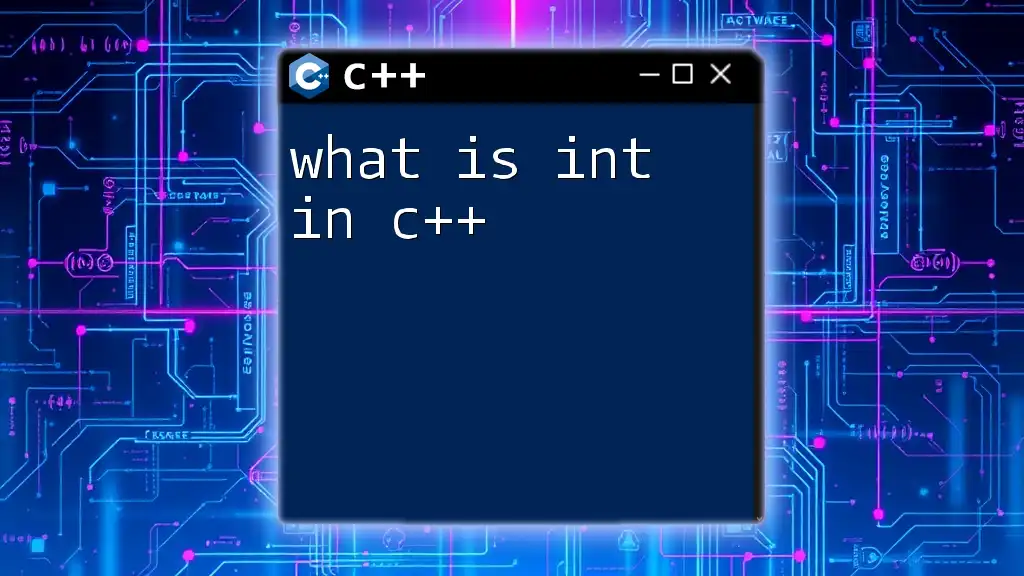
Common Object Operations
Instantiation
Instantiation is the creation of an object from a class. It can be done as follows:
Dog myDog; // myDog is an instance of the Dog class
Accessing Attributes and Methods
To access an object's attributes or methods, use the dot operator (`.`):
myDog.breed = "Beagle"; // Setting attribute
cout << "My dog's breed is: " << myDog.breed << endl; // Accessing attribute
myDog.bark(); // Calling method
Destruction
Destruction is the process of deallocating memory previously assigned to an object. C++ provides constructors and destructors to manage this.
class Example {
public:
Example() { cout << "Constructor Called!" << endl; }
~Example() { cout << "Destructor Called!" << endl; }
};
When an `Example` object goes out of scope, the destructor is automatically called, freeing up resources.
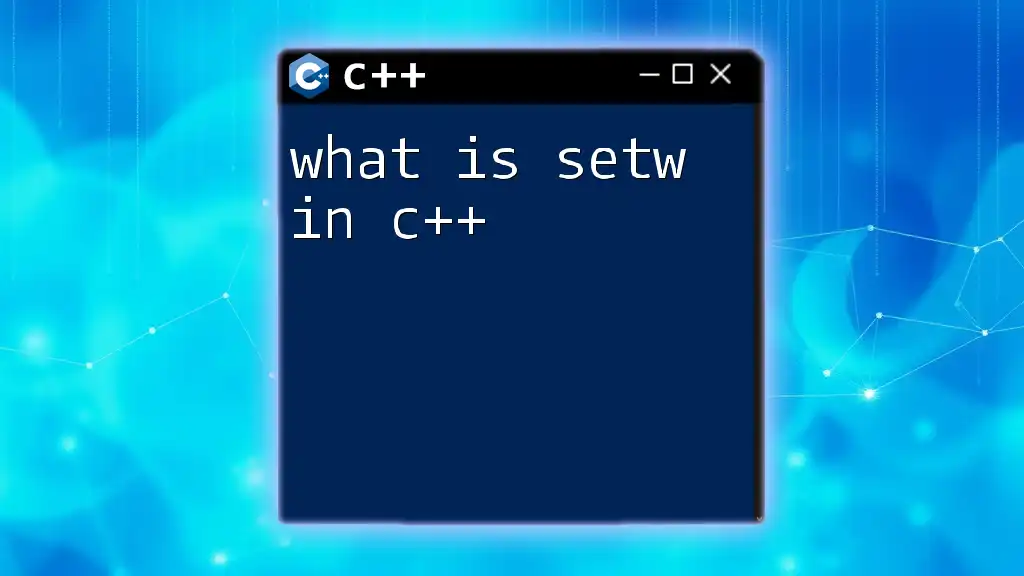
Conclusion
Understanding what is the object in C++ is crucial for leveraging the capabilities of object-oriented programming. Objects encapsulate both data and behavior, allowing developers to create modular, reusable, and flexible code. With this solid foundation in objects, you can delve deeper into the realm of C++ programming and enhance your skills significantly.
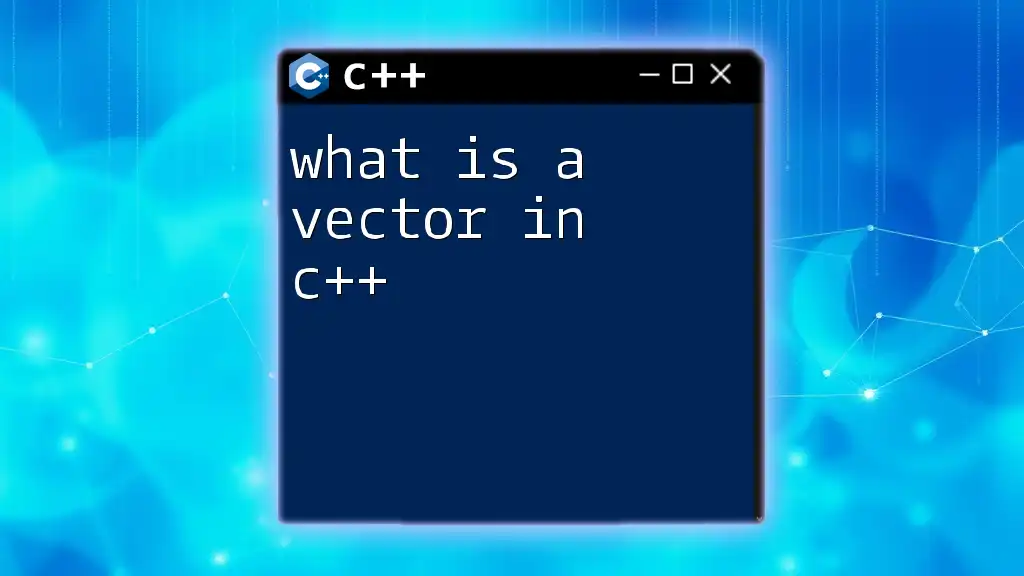
Call to Action
To further your understanding of C++ and object-oriented programming, explore more resources, sign up for courses, or start coding projects that utilize the concepts discussed in this article. Practice is key to mastering C++.
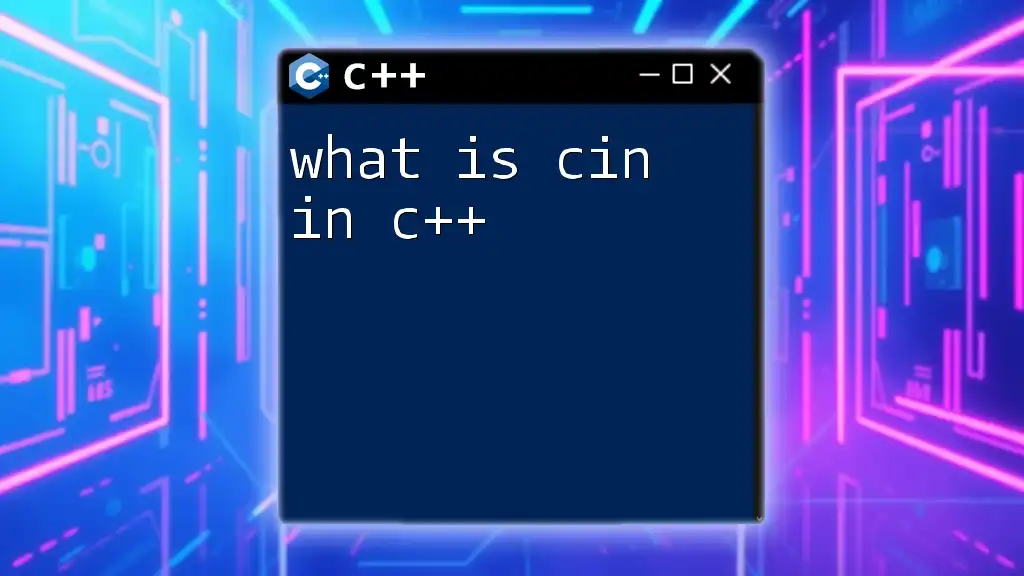
Additional Resources
For additional reading and hands-on practice, consider exploring recommended books, online courses, and community forums. Engaging with a community of C++ programmers can enhance your learning experience and open new opportunities.