Secure coding in C and C++ involves implementing practices that help prevent vulnerabilities such as buffer overflows, ensuring memory safety, and validating inputs to protect against attacks.
Here’s a simple example demonstrating secure string handling using `snprintf` to prevent buffer overflow:
#include <cstdio>
#include <cstring>
int main() {
char buffer[10];
const char* input = "Hello, secure coding!";
// Secure way to copy a string into a buffer, preventing overflow
snprintf(buffer, sizeof(buffer), "%s", input);
printf("%s\n", buffer);
return 0;
}
Understanding Security Vulnerabilities
Common Vulnerabilities in C and C++
When discussing secure coding in C and C++, it is crucial to understand the common vulnerabilities that plague these languages. Key vulnerabilities include:
- Buffer Overflow: This occurs when data exceeds the buffer's capacity, leading to data corruption and potential code execution.
- Use After Free: This vulnerability arises when a program continues to use memory after it has been freed, leading to undefined behavior and potential exploitation.
- Integer Overflow/Underflow: This can happen when calculations exceed the largest (or smallest) representable integer value, leading to unexpected behavior.
- Format String Vulnerability: This occurs when user-controlled data is used in format strings, potentially allowing attackers to read memory and execute arbitrary code.
How Vulnerabilities Lead to Attacks
Understanding how these vulnerabilities lead to threats is vital for implementing secure coding in C and C++. For example, a buffer overflow can allow an attacker to overwrite critical data or even execute arbitrary code. An infamous case is the Morris Worm, which exploited a buffer overflow vulnerability in the early days of the internet, showcasing the dire repercussions of insecure coding.
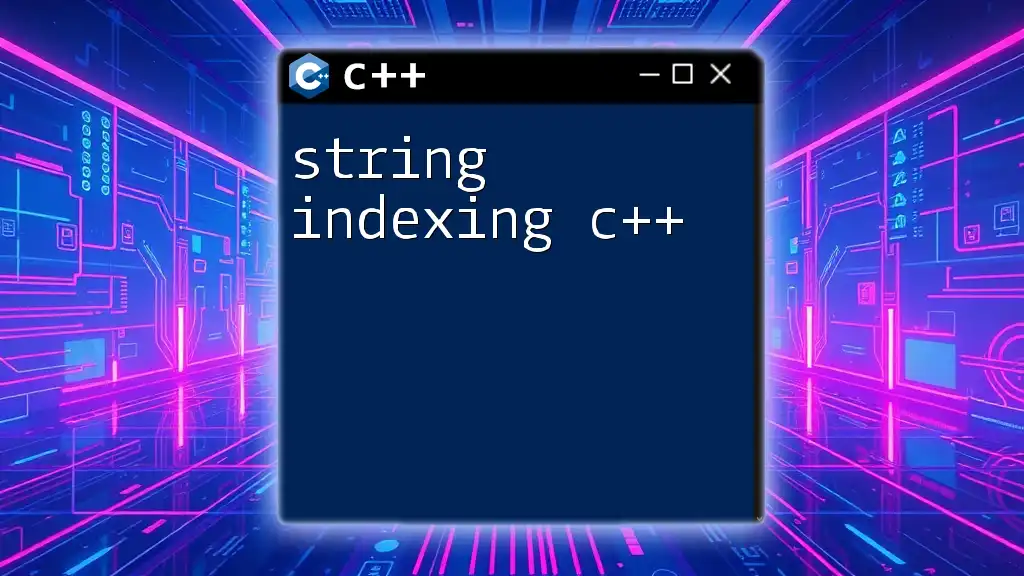
Best Practices for Secure Coding
Input Validation
One of the cornerstones of secure coding in C and C++ is input validation. Relying on user input without validation is a recipe for disaster. Ensure that inputs meet specific criteria before processing them. Techniques for validating user inputs include:
- Whitelisting: Accept only known-good inputs.
- Blacklisting: Reject known-bad inputs, although this is generally less secure.
For example, using a regular expression can help validate a username input as follows:
bool isValidInput(const std::string &input) {
std::regex validPattern("^[a-zA-Z0-9]+$");
return std::regex_match(input, validPattern);
}
Memory Management
Memory management is another critical aspect of secure coding in C and C++. Improper memory allocation and deallocation can lead to vulnerabilities, such as use after free. Always ensure that your memory operations are safe; for example:
void safeMemoryAllocation() {
char *buffer = nullptr;
buffer = (char*)malloc(100 * sizeof(char));
if (buffer == nullptr) {
// Handle memory allocation failure
}
// Use the buffer safely
free(buffer); // Always free allocated memory
}
By checking for NULL after memory allocation, you guard against potential crashes due to failed allocations.
Avoiding Buffer Overflows
Preventing buffer overflows is critical for securing applications. This can be achieved by using safer string functions rather than vulnerable alternatives. For instance:
void safeStringCopy(char *dst, const char *src, size_t size) {
strncpy(dst, src, size - 1);
dst[size - 1] = '\0'; // Ensure null termination
}
Utilizing safer functions helps mitigate the risk associated with buffer overflows and enhances the security of your applications.
Using Safe Libraries
When developing software, it is important to utilize well-established libraries that focus on security. For instance, libraries like OpenSSL for cryptography and Poco for networking offer layers of abstraction that help secure your code against common threats.
Code Reviews and Static Analysis
Code reviews are essential in identifying and mitigating vulnerabilities. Peer reviews allow another set of eyes to evaluate your code for potential risks before deployment. Complementing this process with static code analysis tools, such as cppcheck or Bandit, provides additional security layers by automatically scanning for vulnerabilities.
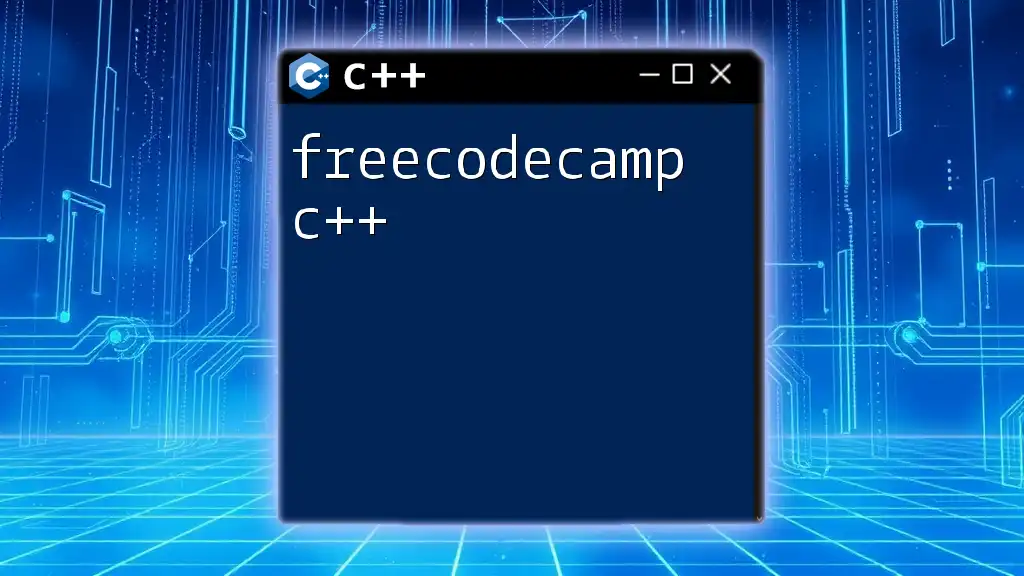
Secure Coding Guidelines for C and C++
Language-Specific Guidelines
C Language Guidelines
When practicing secure coding in C and C++, adhere to language-specific guidelines. In C, it’s essential to handle pointers with care, ensuring their correct initialization and lifetime management. The use of standard libraries for string manipulation whenever possible can significantly reduce risks.
C++ Language Guidelines
C++ provides abstractions such as RAII (Resource Acquisition Is Initialization) that help manage memory more securely. Use smart pointers, like `std::unique_ptr` and `std::shared_ptr`, to manage resource deallocation automatically, thus reducing memory leaks and vulnerabilities related to manual memory management.
The Role of Compiler Flags
Compiler flags are tools that help enforce secure coding practices. Leveraging flags like `-Wall`, `-Wextra`, and `-Wformat` during your build process can spotlight potential security issues right from the start, prompting developers to address warnings before they lead to vulnerabilities.
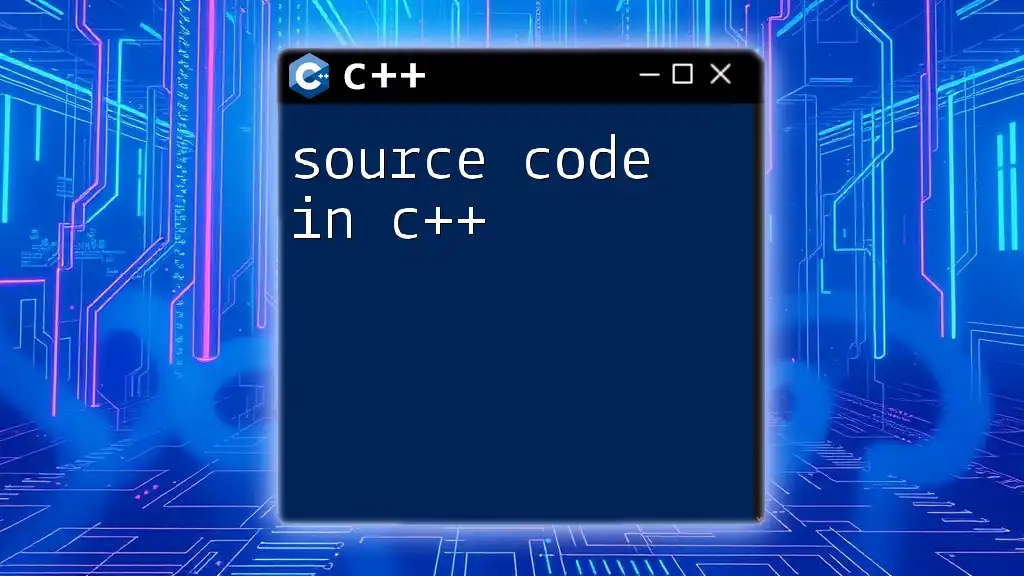
Testing for Security
Understanding Fuzz Testing
Fuzz testing is a technique used to uncover vulnerabilities by inputting randomly generated data into applications. This method can help identify buffer overflows, crashes, and memory leaks. Tools such as AFL (American Fuzzy Lop) and libFuzzer are great resources for implementing fuzz testing, providing extensive coverage of your application’s inputs.
Conducting Penetration Testing
Penetration testing mimics an attacker's attempt to exploit vulnerabilities within a system. This proactive security measure helps identify weaknesses before malicious actors do. Effective penetration testing involves preparation, planning, reconnaissance, scanning, exploitation, and reporting.
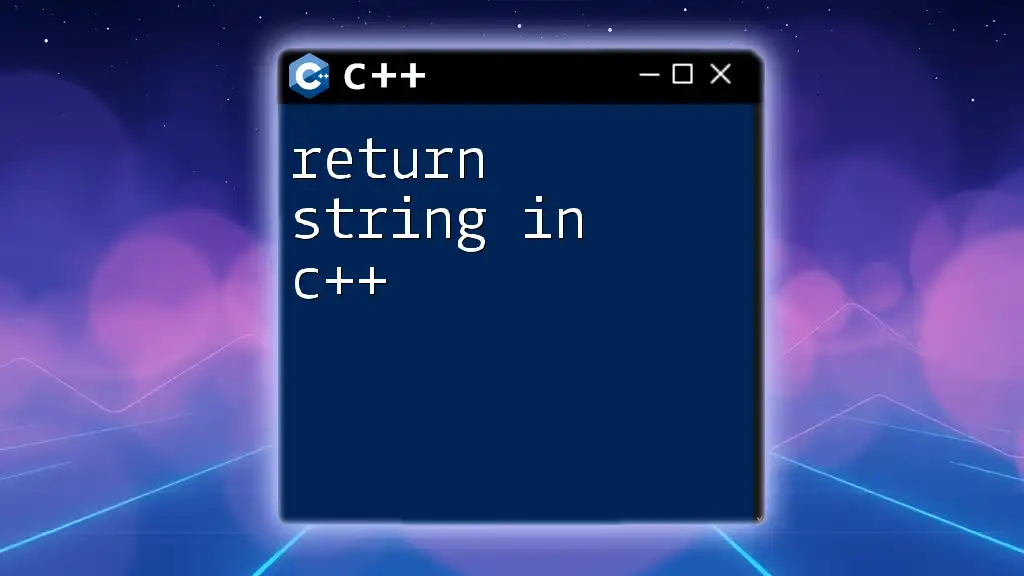
The Future of Secure Coding
Evolving Threat Landscape
As technology advances, so do security threats. Understanding the evolving threat landscape is crucial for developing resilient applications. Regular updates and patches should be part of your development culture to address new vulnerabilities as they arise.
Continuous Learning and Improvement
Fostering a culture of security awareness within development teams enhances the security posture of applications. Continuous learning through training, workshops, and resource sharing is vital for keeping up with the latest best practices in secure coding in C and C++.
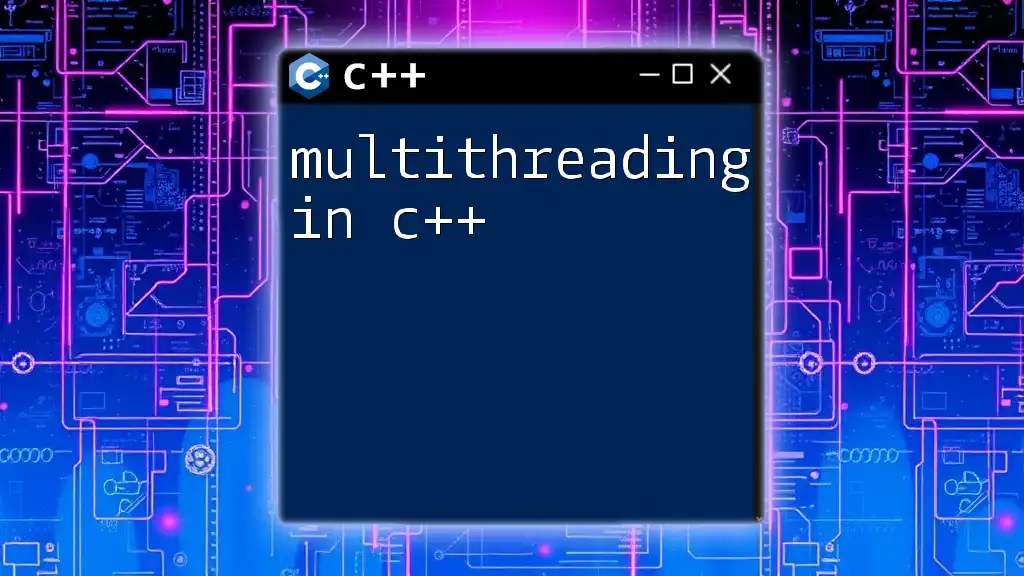
Conclusion
Adopting secure coding in C and C++ is imperative for building robust applications that stand the test of time and threats. Implementing best practices, understanding vulnerabilities, and continually improving your knowledge will equip you to write secure applications that protect both your software and its users. As developers, let’s prioritize security and commit to creating safer code.
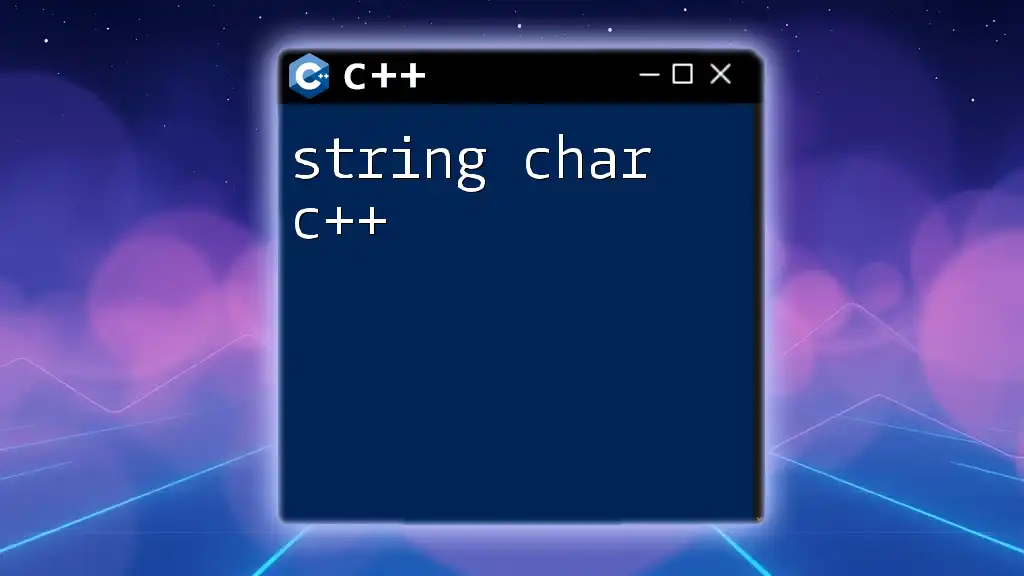
References
- “C and C++ Secure Coding.” Secure Coding Guidelines.
- “Static Code Analysis Tools.” cppcheck Official Documentation.
- “Fuzz Testing Frameworks.” The American Fuzzy Lop (AFL) Project.
This detailed guide serves as a resource to help developers understand and implement secure coding practices effectively in C and C++.