The main differences between C++ versions, such as C++98, C++11, C++14, C++17, and C++20, primarily revolve around the introduction of new features, improved syntax, and enhanced standard libraries that facilitate modern programming practices.
Here's a code snippet showcasing a feature introduced in C++11—a range-based for loop:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
Evolution of C++
The History of C++
C++ was initially developed by Bjarne Stroustrup at Bell Labs in the early 1980s as an enhancement to the C programming language. The language was designed to incorporate object-oriented features, making it robust and versatile. Since its inception, C++ has undergone several revisions that have added important features, addressed bugs, and improved usability.
What is a C++ Standard?
The C++ standard, governed by the ISO (International Organization for Standardization), serves as the authoritative reference for the language's specifications. Each version of C++ results from collaborative efforts by a committee of experts and community members. Understanding these standards is crucial for developers to ensure compatibility and to leverage the latest features in their coding practices.
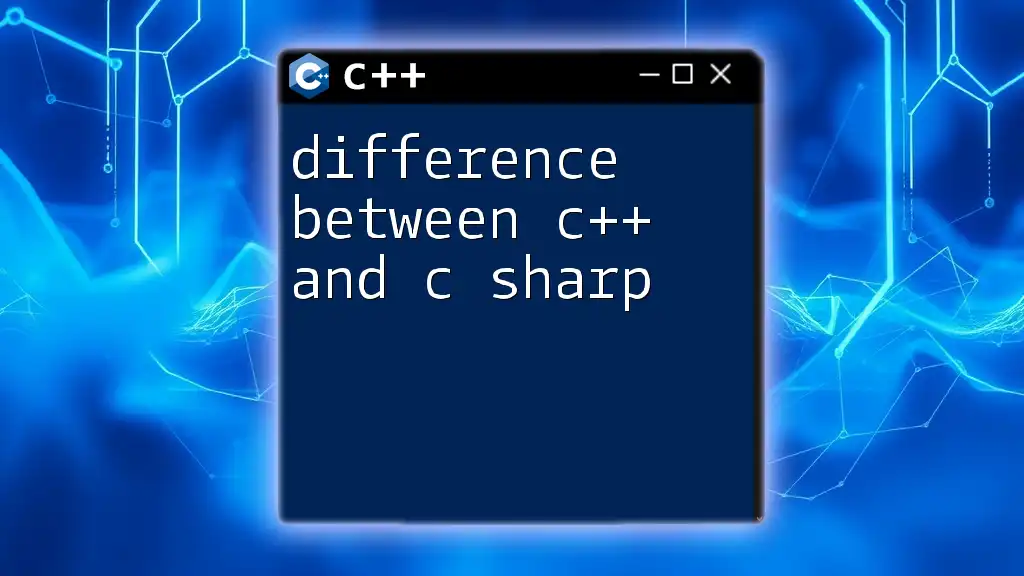
Major Versions of C++
C++98
Overview
C++98 is the first standardized version of C++, formalized in 1998. It laid the groundwork for numerous features that shaped modern C++ programming.
Key Features
Among the most significant additions were the Standard Template Library (STL), namespaces, and exception handling. These features enhanced code organization, reusability, and robustness in handling runtime errors.
Code Example
Here's a simple illustration of C++98 features using STL for a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3};
for (int n : nums) {
std::cout << n << std::endl;
}
return 0;
}
C++03
Overview
C++03, a minor standard published in 2003, primarily addressed inconsistencies and clarifications in the C++98 standard. It did not introduce any significant features but corrected various defects and enhanced library support.
Key Improvements
The revisions improved template specificity and fixed multiple issues found in C++98. These refinements ensured better performance and easier use of the language.
Code Snippet Highlight
One of the clarifications made in C++03 involved template specialization, allowing developers to write more efficient and clearer code.
template<typename T>
class Example {
// C++03 allows some enhancements on templates
};
C++11
Overview
C++11 marked a transformative phase in the language's evolution, introducing a wealth of new features that extended C++'s capabilities significantly.
Key Features
Prominent additions include the auto keyword, range-based for loops, lambda expressions, and enhanced support for multithreading through the inclusion of standard thread libraries. These changes made C++ more versatile and developer-friendly.
Code Examples
The introduction of lambda expressions simplified the syntax required for defining functions on-the-fly:
auto add = [](int a, int b) { return a + b; };
std::cout << add(3, 4) << std::endl; // Outputs: 7
C++14
Overview
C++14, released in 2014, built upon the features of C++11 with primarily incremental improvements aimed at enhancing usability.
Key Features
C++14 included features such as generic lambdas, which allowed lambda expressions to accept any type of arguments, and introduced std::make_unique for better memory management. The restrictions on the use of `constexpr` were also relaxed, allowing for more expressions to be evaluated at compile-time.
Code Example
Here's an example of the flexibility provided by generic lambdas:
auto lambda = [](auto a, auto b) { return a + b; };
std::cout << lambda(1, 2.5) << std::endl; // Outputs: 3.5
C++17
Overview
C++17 continued the trend of introducing substantial features that revamped the programming experience, focusing on performance and usability.
Key Features
The standard added std::optional, std::variant, and a filesystem library, with support for parallel algorithms. These features help developers manage data and file interactions more efficiently.
Code Example
The use of `std::optional` allows for cleaner code when a function may or may not return a value:
#include <optional>
std::optional<int> maybeGetInt(bool getIt) {
if (getIt) return 42;
return std::nullopt;
}
C++20
Overview
C++20 is another landmark version that introduced many game-changing features, streamlining development and enhancing performance.
Key Features
This version unveiled concepts, ranges, and modules, along with support for coroutines and the spaceship operator for three-way comparisons. These enhancements greatly simplify the management of types and streamline data processing in programs.
Code Example
Here’s how the spaceship operator simplifies syntax for comparisons:
#include <compare>
struct Point {
int x, y;
auto operator<=>(const Point&) const = default;
};
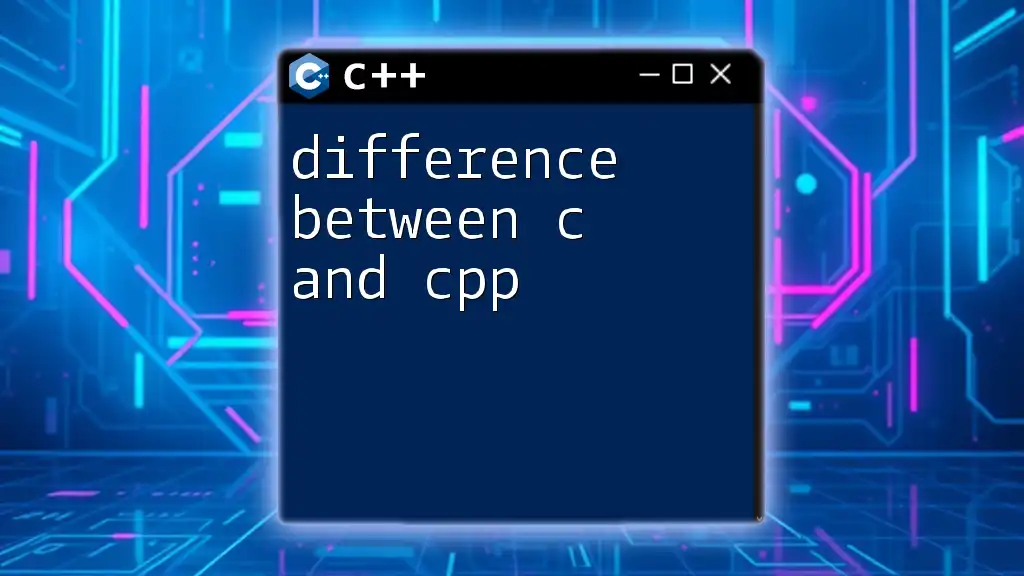
Future of C++
Upcoming Features
C++23 and Beyond
The community eagerly anticipates C++23, which is expected to introduce new features aimed at further improving code efficiency and developer productivity. Features under discussion may include expanded support for compile-time programming and better standard libraries.
Potential impact on developers
Understanding the differences between C++ versions is crucial for developers striving to write modern and efficient code. The ongoing evolution of the language also encourages continual learning and adaptation.
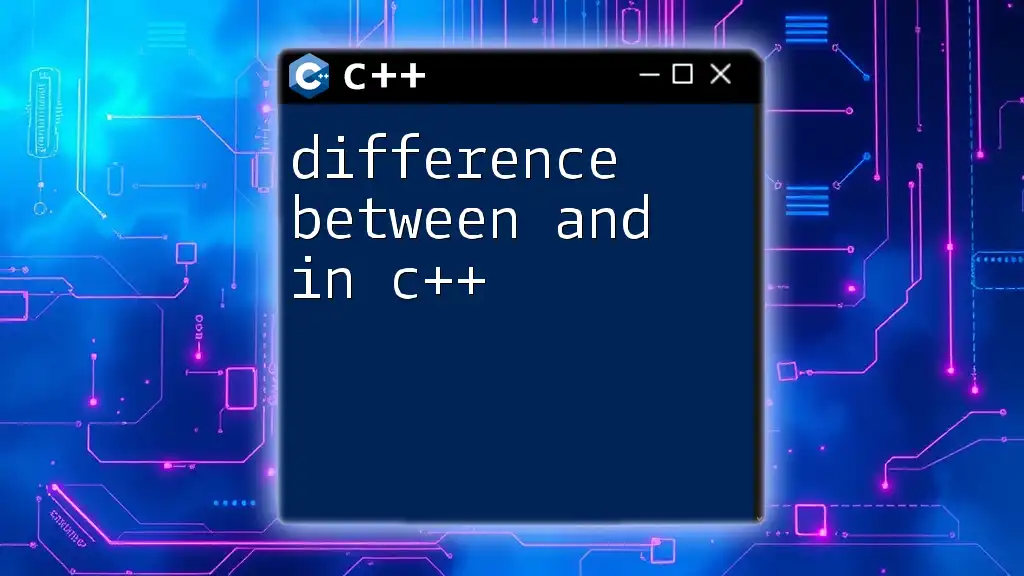
Conclusion
In summary, the difference between C++ versions extends beyond merely added features; it reflects a transformation in how developers interact with the language. Each version has built upon its predecessors to enhance usability, performance, and capabilities. Staying informed about these differences can significantly impact coding practices and project outcomes.
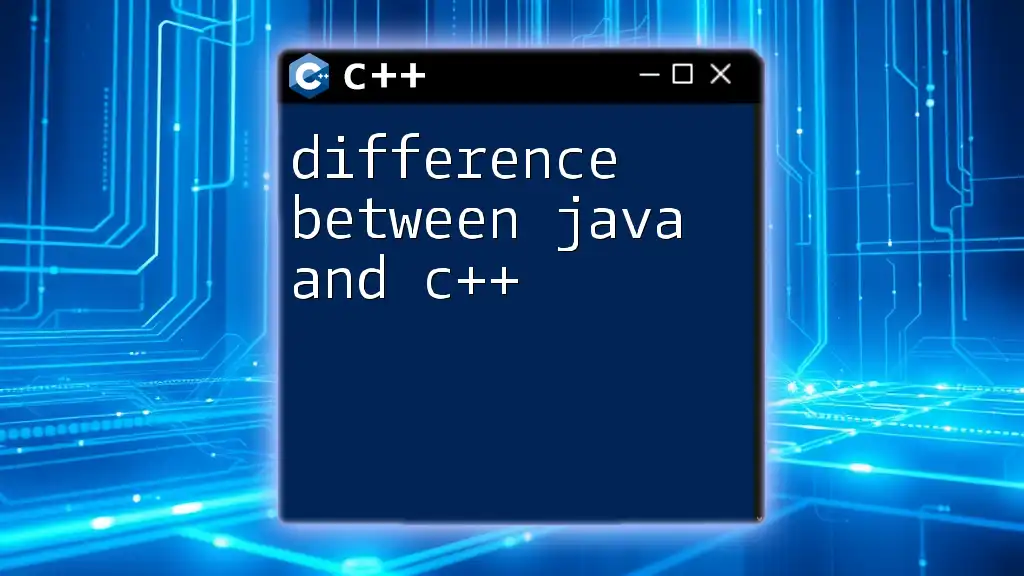
Call to Action
If you wish to deepen your understanding of C++ and stay updated with the latest trends, we invite you to join our community. Subscribe for regular updates and tutorials designed to enhance your coding skills in C++.
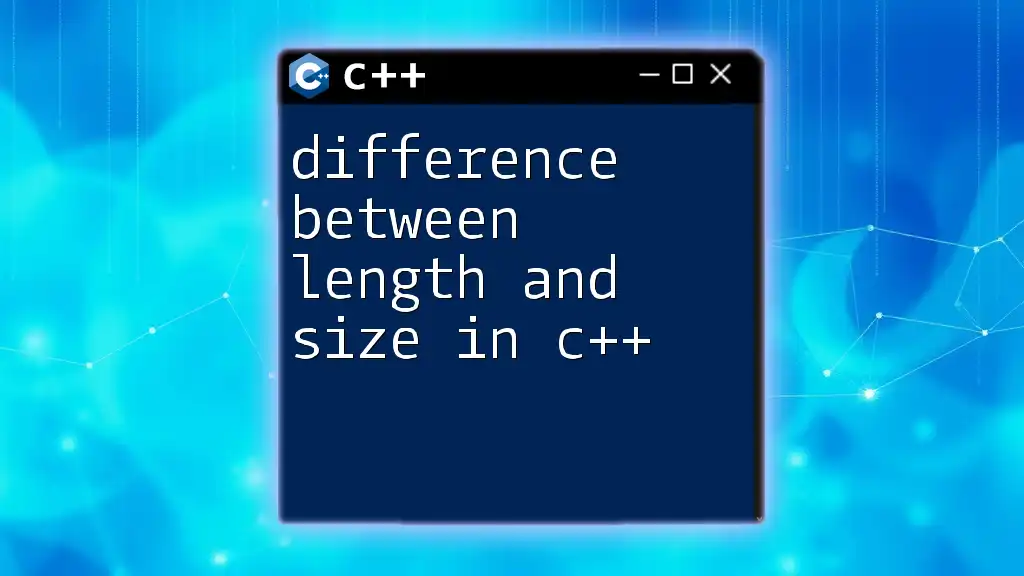
Additional Resources
Explore recommended books and trusted websites dedicated to C++ learning. Join forums and community groups where developers discuss updates, share insights, and collaborate on projects. Consider working on practical exercises tailored to the various C++ versions discussed to solidify your understanding of the differences and improvements over time.