Dev C++ is primarily a Windows-based IDE, so Mac users can use alternative options like Code::Blocks or Xcode to write and compile C++ code efficiently; however, if you wish to use Dev C++ on a Mac, you can leverage virtualization or Wine to run it.
Here’s a simple C++ code snippet for beginners:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Dev C++ for Mac
Why Dev C++?
Dev C++ is a powerful Integrated Development Environment (IDE) designed primarily for Windows, which facilitates the development of C and C++ applications. Its intuitive interface and robust features make it a popular choice among programmers. However, for Mac users, accessing this tool can be challenging. Understanding its features is vital to appreciate why such an IDE can be beneficial, even if alternative options exist. Key capabilities include syntax highlighting, project management, and debugging tools.
Installation Steps
Download and Installation
Unfortunately, Dev C++ is not natively supported on macOS. However, you can still enjoy its benefits by utilizing Windows emulation tools such as Wine. Always ensure you are downloading from trusted sources to avoid potential security risks.
WineHQ as an Emulator
Wine is an impressive compatibility layer that enables Mac users to run Windows applications without needing a Windows license. Here’s a basic guide to installing Wine:
- Visit the WineHQ website and download the latest version of Wine for macOS.
- Follow the installation instructions provided on the site. This typically involves dragging the Wine application into your Applications folder and potentially running additional configuration commands in the terminal.
Installing Dev C++ using Wine
Follow these steps to install Dev C++:
- Download the Dev C++ installer from a reliable source.
- Open the terminal and navigate to the directory where you downloaded the installer.
- Use Wine to run the installer with the following command:
Replace `devcpp_x.x_setup.exe` with the actual filename of the downloaded installer.wine devcpp_x.x_setup.exe
- Follow the on-screen instructions to complete the installation.
During installation, you may encounter prompts or errors related to dependencies; these can often be resolved by following the detailed instructions provided by Wine.
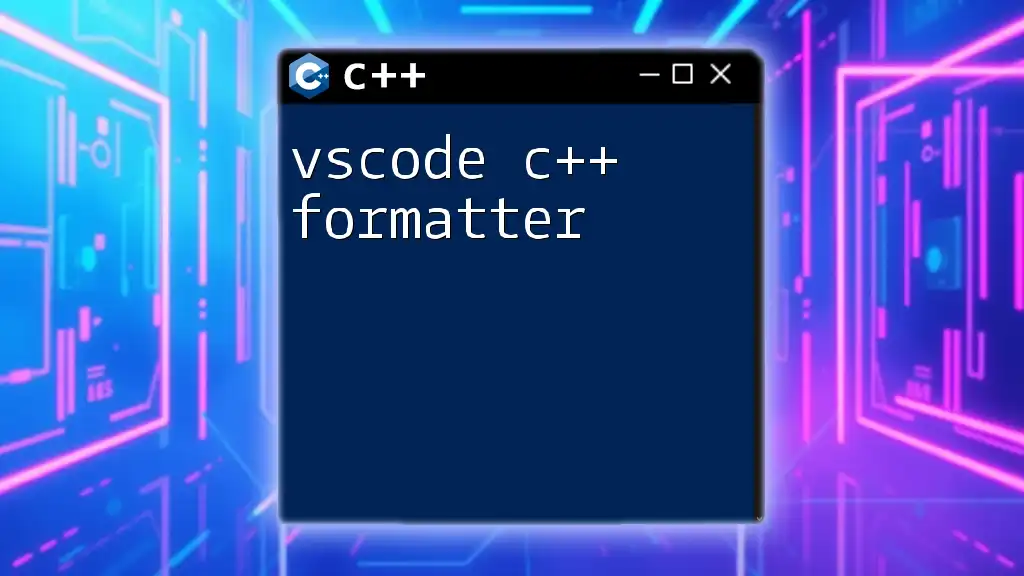
Alternatives to Dev C++ on Mac
Popular C++ IDEs compatible with Mac
Xcode
Xcode is the official IDE for macOS development. It offers comprehensive tools for C/C++ programming and is tightly integrated with Apple's ecosystem.
- Advantages: Free and highly versatile, with extensive support and documentation.
- Disadvantages: Can be overwhelming for beginners due to its numerous features and complexities.
Code::Blocks
Code::Blocks is an open-source, cross-platform IDE designed for C/C++ programming.
- Features: It includes customizable build tools, debugging capabilities, and support for multiple compilers.
- Installation: Download the macOS version from the Code::Blocks website and follow installation guides similar to Dev C++.
CLion
CLion is a powerful IDE developed by JetBrains, known for its intelligent coding assistance and robust coding features.
- Why Choose CLion?: It provides advanced code completion, refactoring, and navigation tools.
- Benefits and Constraints: As a paid option, you may need to invest in it unless you qualify for academic discounts.
Comparative Analysis of IDEs
In choosing an IDE, consider:
- Performance: Evaluate how well each IDE runs on Mac hardware.
- Usability: Read user reviews or forums for personal experiences.
- Features: Assess whether the features meet your C++ programming needs.
- Community Support: Check for active online communities where you can get help and resources.
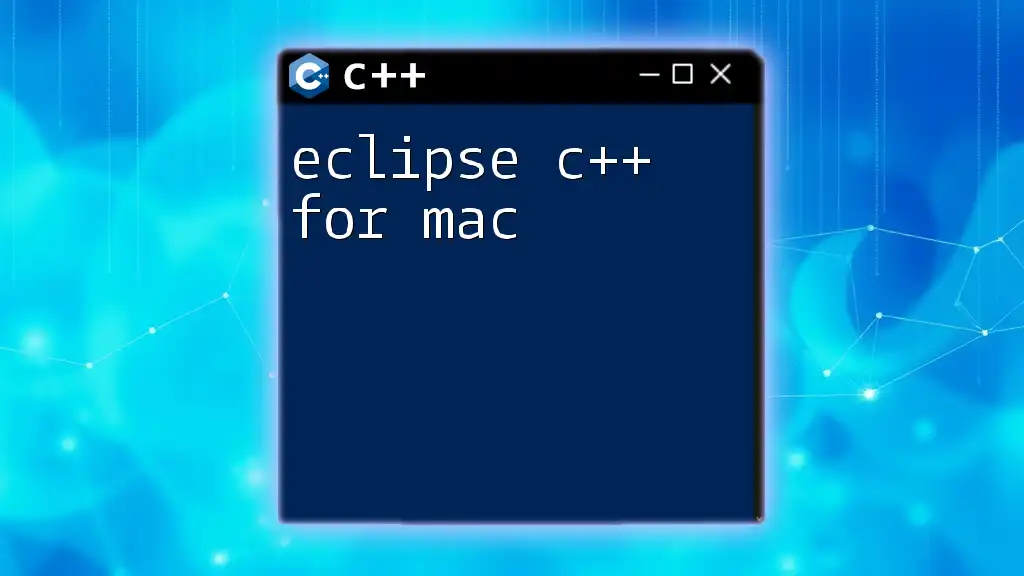
Writing Your First C++ Program in Dev C++
Creating a New Project
When you launch Dev C++, you can create a new project by navigating to the "File" menu and selecting "New Project." Configure the project settings, including its name and location. Note that effectively managing your projects is crucial for larger applications:
- Select the type of project (Console Application is recommended for beginners).
- Set your compiler to the one you prefer, typically the default MinGW compiler that comes bundled with Dev C++.
Sample Code Example
Let’s write a simple "Hello World" program. This is the quintessential first program for any programming language, serving as a useful introduction to the basics.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This code does the following:
- `#include <iostream>`: Incorporates the standard input-output library.
- `using namespace std;`: Allows you to use standard library functions without the `std::` prefix.
- `cout << "Hello, World!" << endl;`: Outputs "Hello, World!" to the console, followed by a newline.
- `return 0;`: Indicates that the program has executed successfully.
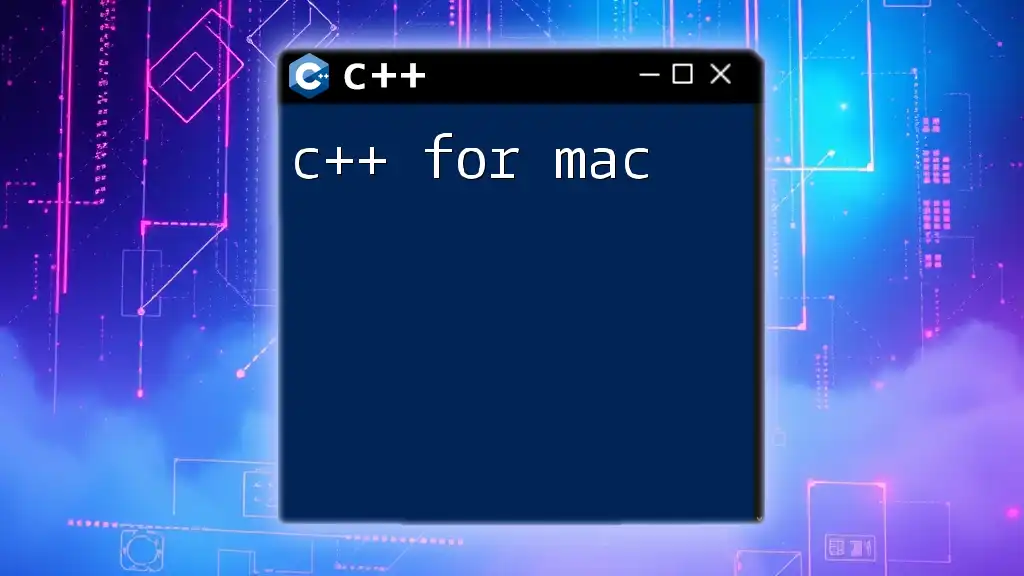
Compiling and Running Your Program
Compilation Process in Dev C++
After writing your code, it's time to compile it. Click on the "Execute" menu, then select "Compile." Dev C++ will translate your C++ code into executable machine code.
However, you may encounter compilation errors; these are generally indicated in the output window. Pay close attention to line numbers and error messages, as they guide you in debugging issues in your code.
Running the Executable
Once your code compiles without errors, go back to the "Execute" menu and click on "Run." The output console should display your "Hello, World!" message. Failure to see the expected output usually indicates a problem in compilation; review the output messages closely to identify any errors or warnings.
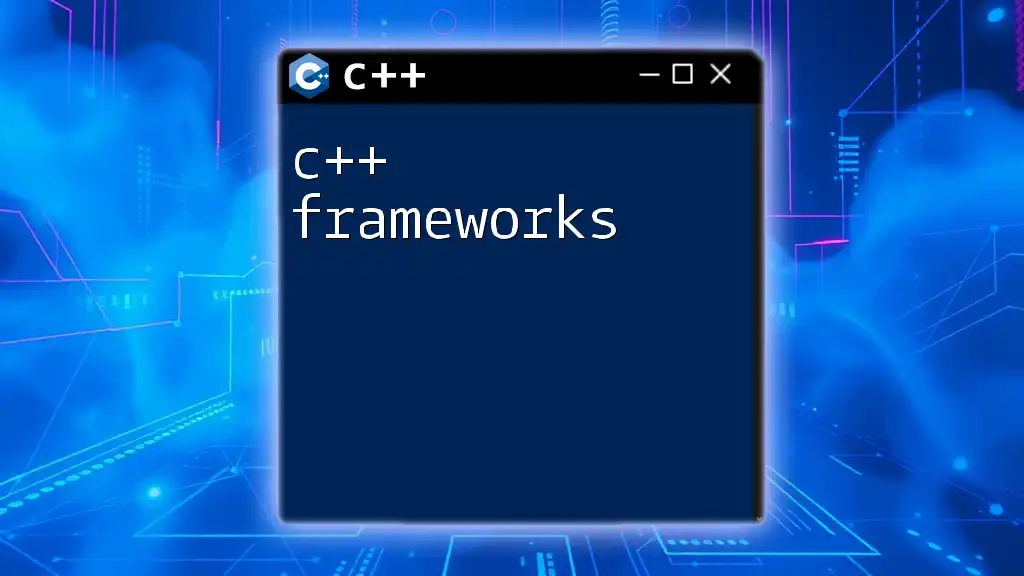
Debugging C++ Code in Dev C++
Importance of Debugging
Debugging is an essential part of programming. It allows you to identify and rectify errors in your code before deployment. Neglecting debugging can lead to significant issues later in application development.
Debugging Techniques
Dev C++ integrates several powerful debugging tools:
- Debugging Commands: Learn commands like "Step Over," "Step Into," and "Continue." These help navigate through the code execution step-by-step.
- Watchpoint and Breakpoints: Set breakpoints in your code to pause execution at specific points. This functionality helps inspect variables and program flow.
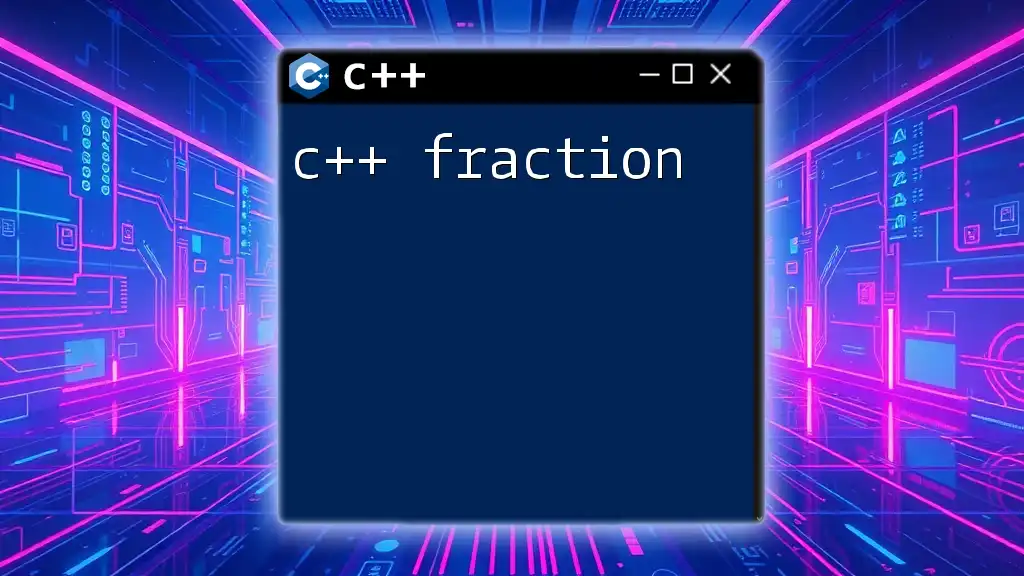
Best Practices for C++ Programming
Code Organization and Structure
Organizing your code clearly is crucial. Use meaningful variable names and consistent formatting styles. This practice not only helps you but also makes your code easier for others to read and understand.
- Comments: Include comments in your code to explain complex segments or logic.
- Functions: Modularize code by breaking down tasks into separate functions. This practice enhances readability and maintainability.
Efficient Resource Management
Managing memory allocation is vital in C++. Be sure to:
- Use smart pointers to handle dynamic memory safely.
- Familiarize yourself with key concepts like `new`, `delete`, and destructors to prevent memory leaks.
Example of a Well-Structured Program
Here’s an illustration of some best practices implemented in a C++ program:
#include <iostream>
#include <vector>
using namespace std;
void printVector(const vector<int>& vec) {
for (int num : vec) {
cout << num << " ";
}
cout << endl;
}
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
printVector(numbers);
return 0;
}
In this example, the code utilizes a function to print elements of a vector, demonstrating modular design, clarity, and efficiency.
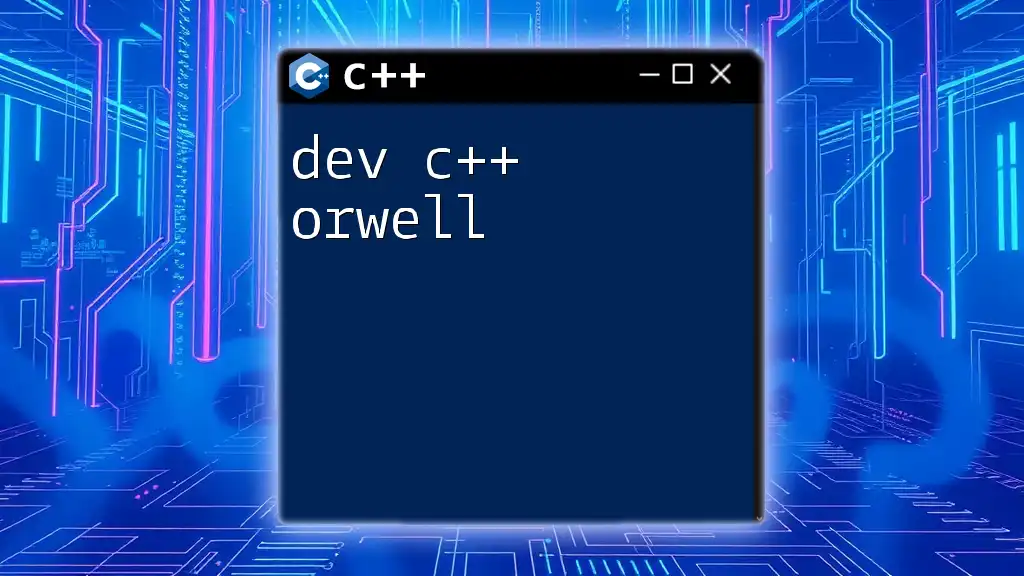
Conclusion
Exploring the intricacies of Dev C++ for MacBook may pose unique challenges, yet the potential for effective C++ programming is immense. Look beyond the hurdles, utilize emulation tools, or consider alternative IDE options. Embrace the learning curve and experiment with its functionalities to become proficient in C/C++ programming.
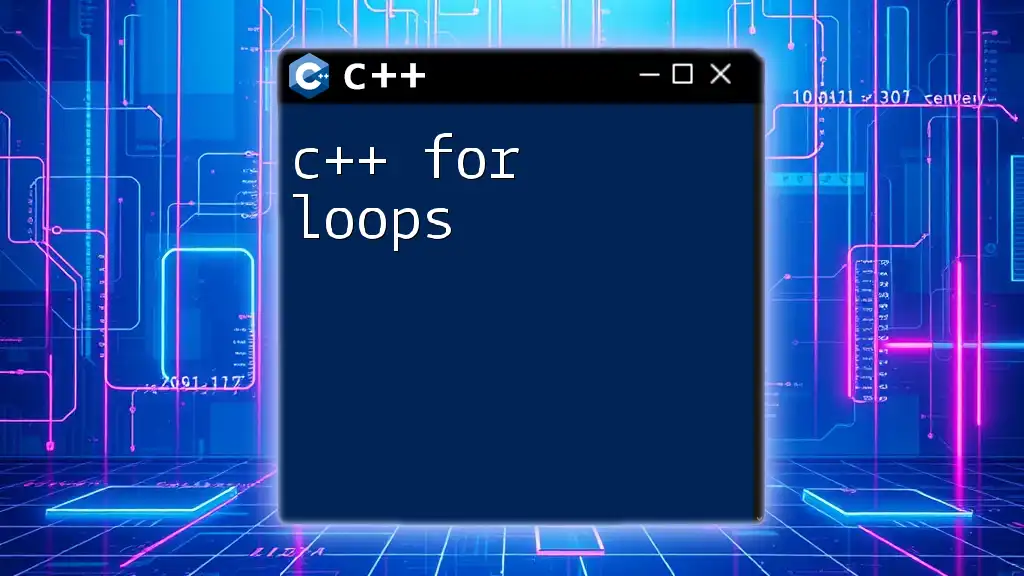
Additional Resources
To optimize your learning, consider delving into books, online courses, and tutorials focused on C++. Engage with active communities on forums where you can exchange knowledge and get tips from seasoned programmers. Moreover, keep an eye on the official C++ documentation and updates to stay informed about the evolving landscape of the language.