"Dev-C++ (Orwell) is a free integrated development environment (IDE) for C and C++ programming that is popular for its simplicity and ease of use, particularly for beginners."
Here's a sample code snippet demonstrating a basic Hello World program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Dev C++?
Understanding Dev C++
Dev C++ is an integrated development environment (IDE) designed specifically for programming in C and C++. It is aimed primarily at beginners and those looking for a lightweight and efficient tool for coding. Originally developed by Orwell, this IDE has garnered popularity due to its simplicity and effectiveness, making it an excellent choice for both novice and experienced programmers.
Features of Dev C++
One of the standout features of Dev C++ is its user-friendly interface, which makes the coding process smooth. Key features include:
- Syntax Highlighting: This enhances readability by visually distinguishing keywords, comments, and strings.
- Code Completion: Dev C++ can anticipate what the user is trying to type and offers suggestions, which speeds up coding and reduces errors.
- Integrated Debugging Tools: Debugging is made easier with built-in tools that help users identify and fix errors quickly.
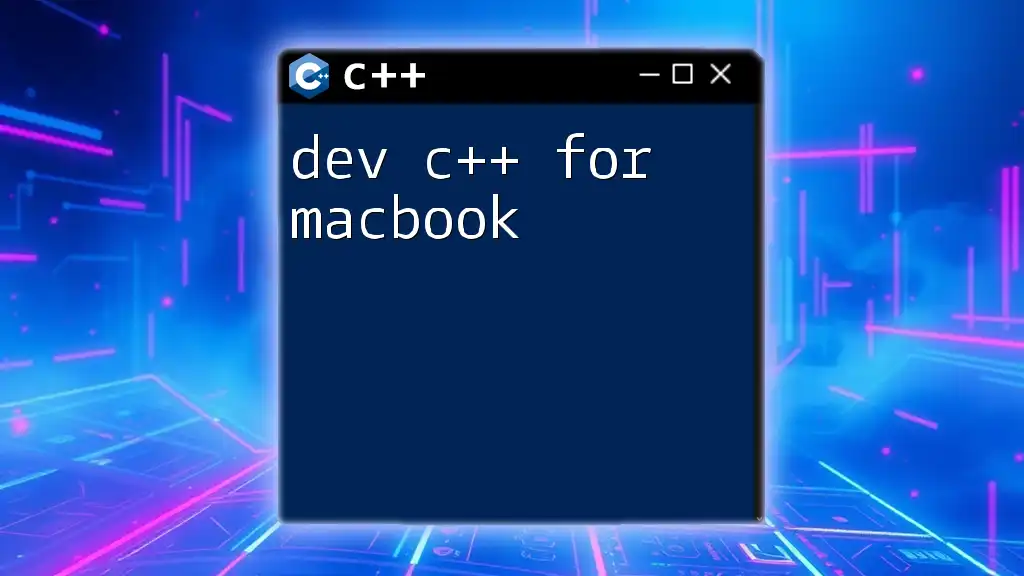
Setting Up Dev C++ (Orwell)
System Requirements
Before installation, ensure your system meets the necessary specifications. The following are the minimum requirements:
- OS: Windows 7 or later
- RAM: At least 1 GB
- Disk Space: 100 MB free space
For optimal performance, consider the recommended requirements:
- OS: Windows 10 or later
- RAM: 4 GB or more
- Disk Space: 500 MB free space
Downloading Dev C++
To get started, download Dev C++ from its official website. The download process is straightforward:
- Navigate to the official Dev C++ website.
- Look for the download link for "Dev C++ (Orwell)".
- Click the link to start the download.
Installation Process
Once downloaded, installing Dev C++ is simple. Follow these steps:
- Locate the downloaded file and double-click it to start the installation wizard.
- Follow the prompts to agree to the license terms and specify the installation directory.
- Complete the installation, which should take just a few minutes.
For users who prefer command-line interfaces, you may find alternate instructions to install Dev C++ via package managers in specialized forums.
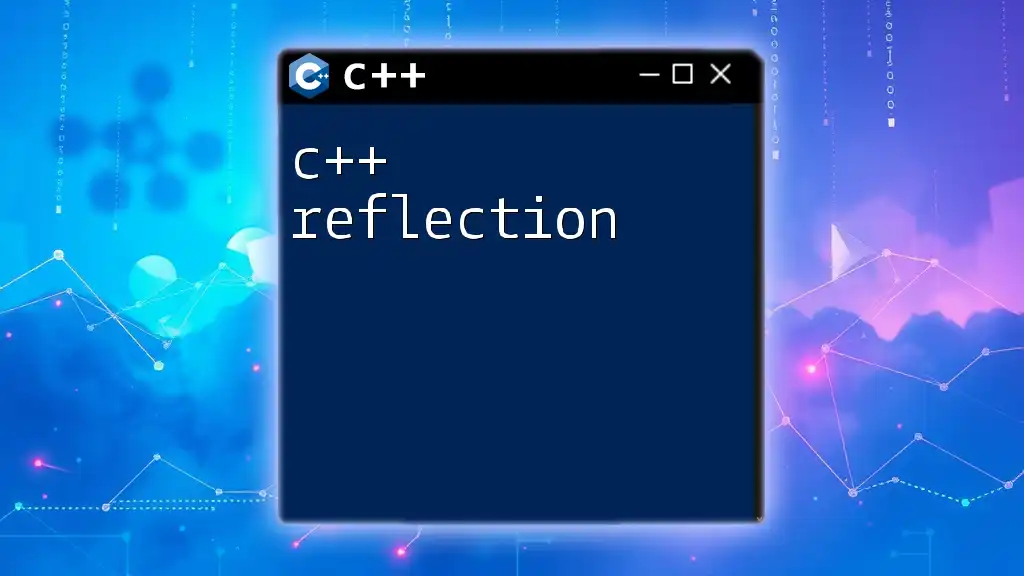
Navigating the Dev C++ Interface
Introduction to the Interface
Upon launching Dev C++, you'll notice a sleek interface divided into multiple sections. The editor where you write code occupies the largest area, while the console displays output and error messages. The toolbar contains essential commands for building, running, and debugging your code.
Creating a New Project
To begin coding, you need to create a new project. This is how you can set up a simple C++ project:
- Go to File > New > Project.
- Select "C++ Project" from the options provided.
- Name your project and choose a location for it.
Example: Creating a “Hello World” Project
You can start with a simple "Hello World" program. Enter the following code in the editor:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program introduces the basics of input and output in C++.
Managing Projects and Files
As your projects grow, organization becomes critical. Dev C++ allows you to structure projects using source files, header files, and resource files. Create folders within your project directory to keep related files together, which aids in maintaining an efficient workflow.
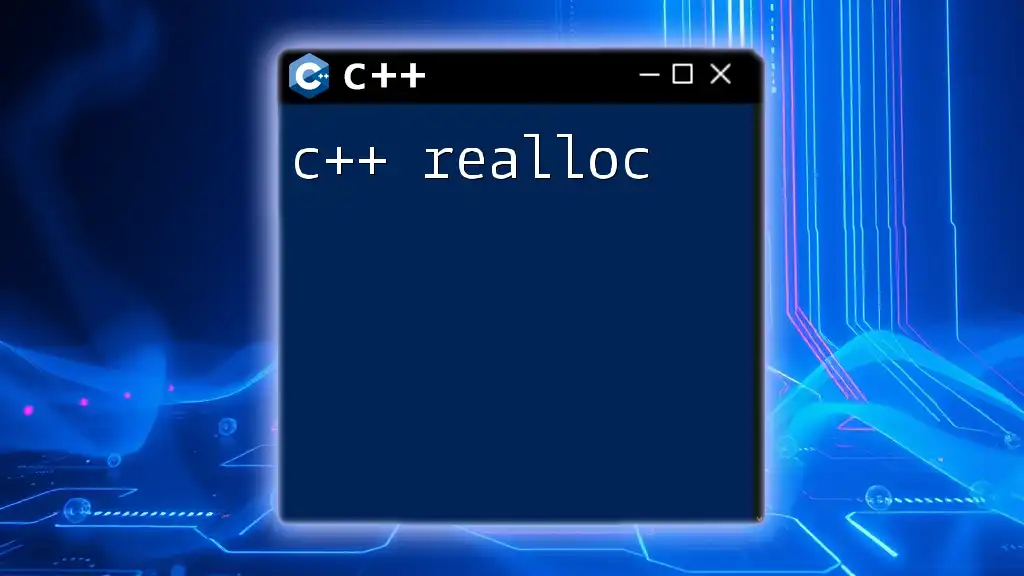
Writing Code in Dev C++
Basic Syntax in C++
Familiarity with C++ syntax is essential for effective coding. Here are the components you should know:
- Data Types: C++ supports various data types, including integers (`int`), floating-point numbers (`float`, `double`), and characters (`char`).
- Variables: Assign values to variables using the assignment operator (`=`). For instance:
int age = 25;
Editor Features
Dev C++ enhances the coding experience with several editor features:
- Syntax Highlighting helps you quickly identify different elements of your code.
- Auto-completion reduces typing time by suggesting methods, classes, and keywords as you type.
You can customize these features via the settings menu for a more personal experience.
Code Snippets and Templates
To improve efficiency, create code snippets for commonly used functions. This allows you to reuse code without rewriting it. For instance, a frequently used function for calculating the square of a number could look like this:
int square(int number) {
return number * number;
}
Using templates also boosts productivity by streamlining the creation of new files or functions.
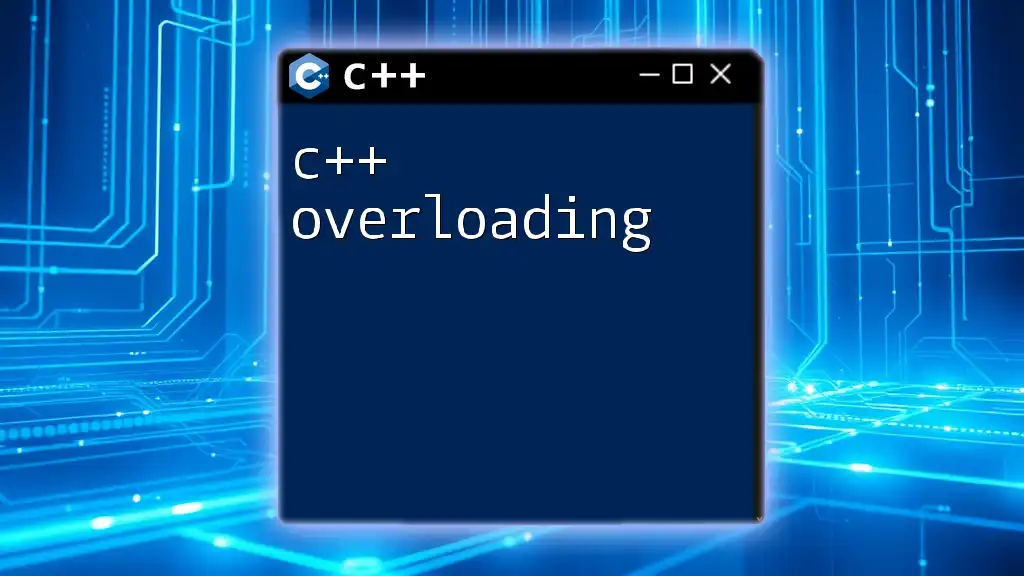
Compiling and Running C++ Programs
Understanding Compilation
Compilation is the process of converting your C++ source code into machine code, making it executable. This is a crucial step in programming, as errors can only manifest during this phase.
Compiling a Program in Dev C++
To compile your C++ program, follow these steps:
- Ensure your code is free from syntax errors.
- Click on Execute > Compile or press `F9`.
- Pay attention to the console for any error messages or warnings.
Running Your Program
After successful compilation, running your program can be achieved by clicking on Execute > Run or by pressing `Ctrl + F10`. You should see the output displayed in the console.
Example: Running the "Hello World" Project
If you've followed the earlier steps correctly and run the "Hello World" program, the expected output should be:
Hello, World!
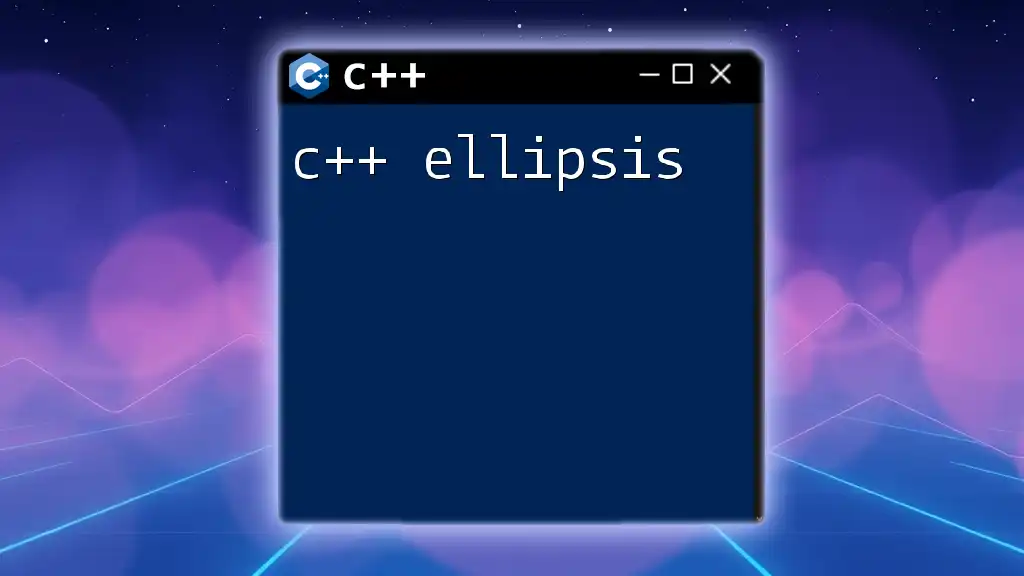
Debugging in Dev C++
Introducing the Debugger
Debugging is a critical part of the programming process, and Dev C++ provides built-in debugging tools to assist you. A debugger allows you to identify runtime errors, logical errors, and other issues that may arise when your program runs.
Setting Breakpoints
You can set breakpoints, which are markers that allow you to pause execution at specific lines of code. This enables you to inspect variable values and the program’s state at that point.
Using the Debugging Tools
To utilize the debugging tools effectively, follow these steps:
- Set breakpoints by double-clicking in the left margin next to the line numbers.
- Start debugging by clicking Debug > Start/Continue.
- Use commands like "Step Into" to advance through your code line by line, and "Watch" to monitor specific variables.
Example: If you have a logical error in a loop, setting a breakpoint can help you inspect the variable's value at each iteration.
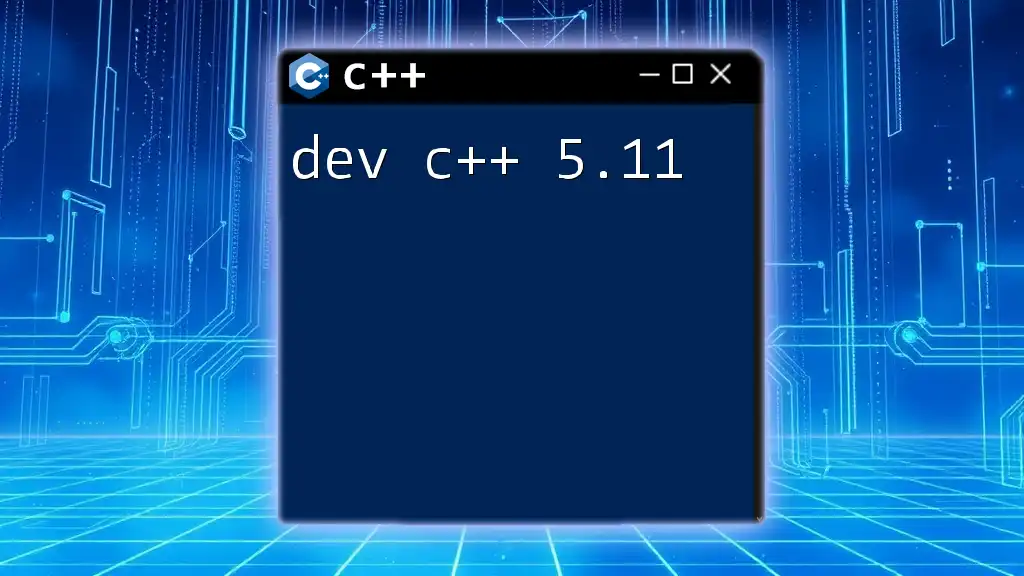
Best Practices for Using Dev C++
Code Organization
For larger projects, code organization is essential. Group related files in subfolders and use descriptive naming conventions for files and variables. This not only simplifies navigation but also improves code readability.
Version Control
Version control systems, like Git, are invaluable tools for managing changes in your project. Integrating version control into your workflow allows you to track changes and collaborate with others effectively. Dev C++ does not support version control natively, so consider using external tools.
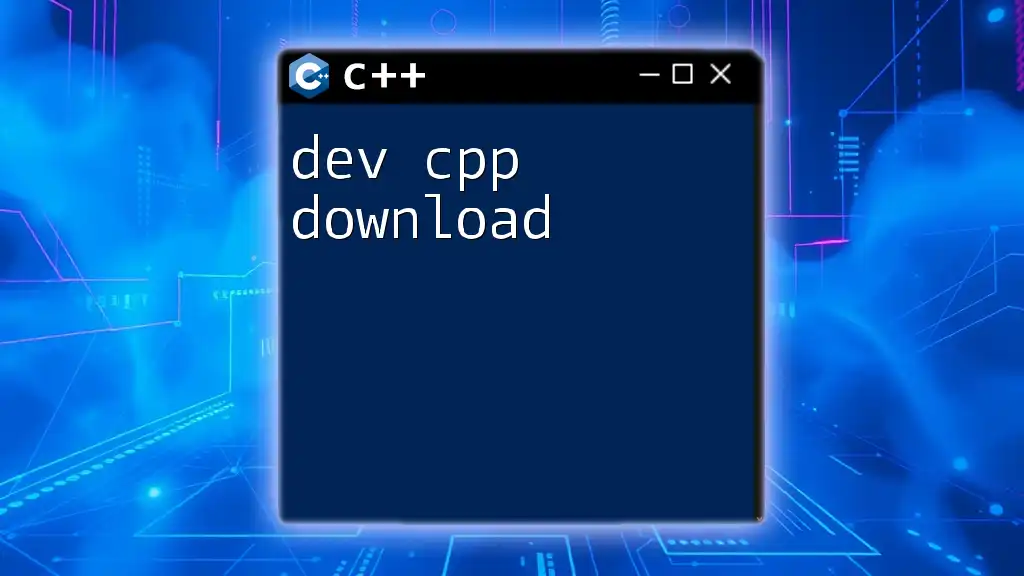
Troubleshooting Common Issues
Compilation Errors
Many new users encounter compilation errors at first. Common errors include:
- Syntax Errors: Misspelled keywords or misplaced punctuation. Always double-check your code for such mistakes.
- Missing Libraries: Ensure you include all necessary libraries for your project. For example, if you are using functions from the `<iostream>` library, include it at the top of your code.
Runtime Errors
Runtime errors occur during program execution. Common issues include:
- Null Pointer Exceptions: Attempting to access an uninitialized pointer.
- Infinite Loops: Ensure your loop conditions will terminate. Use debugging tools to step through your code.
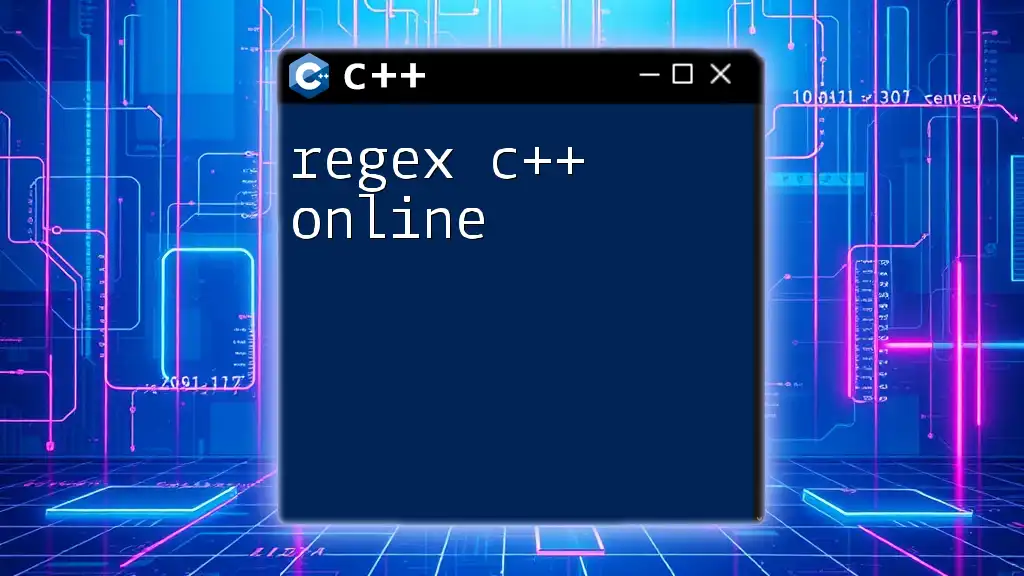
Conclusion
Dev C++ (Orwell) stands out as a valuable tool for C++ programming, especially for beginners. Its ease of use, combined with essential features, provides a solid foundation for learning and developing C++ projects. With practice and the application of best practices discussed, users can enhance their programming skills and tackle more complex challenges in C++. Embrace the experience and enjoy the journey of coding in C++.
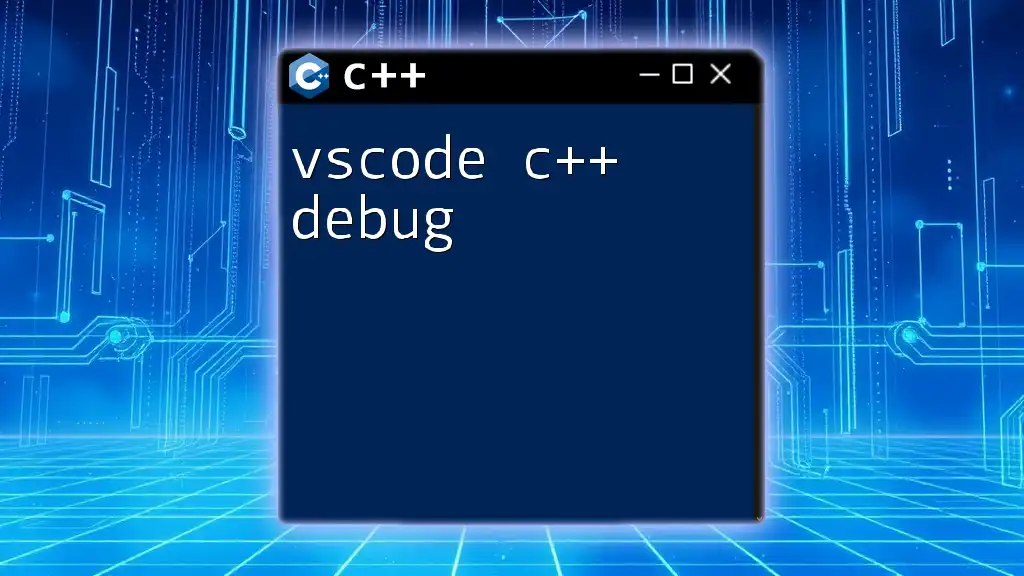
Additional Resources
For further learning, refer to additional tutorials, official documentation, and community forums dedicated to C++ programming and Dev C++. Engaging with others in the programming community can greatly enhance your understanding and provide valuable insights and support as you progress in your coding endeavors.