A C++ for loop can be effectively used to iterate through elements in a vector, enabling efficient access and manipulation of its contents. Here's a simple example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.size(); i++) {
std::cout << numbers[i] << " ";
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a sequence container that encapsulates dynamic size arrays. Unlike traditional arrays, which have a fixed size, vectors can grow and shrink as needed, offering greater flexibility and convenience in managing collections of data.
Vectors are part of the Standard Template Library (STL) and provide several built-in functions and capabilities. Key advantages of using vectors include:
- Dynamic sizing: Vectors can change in size during program execution.
- Automatic memory management: Vectors handle memory allocation and deallocation automatically.
- Easy to use: They come with useful member functions for common operations.
Creating a Vector
To create a vector in C++, you need to include the vector header and specify its data type. Here's how you can declare and initialize a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers; // Declaring an empty vector of integers
std::vector<int> initializedNumbers = {1, 2, 3, 4, 5}; // Initialized with values
return 0;
}
In the example above, the first vector `numbers` is declared but not initialized, while `initializedNumbers` is created with predefined values.
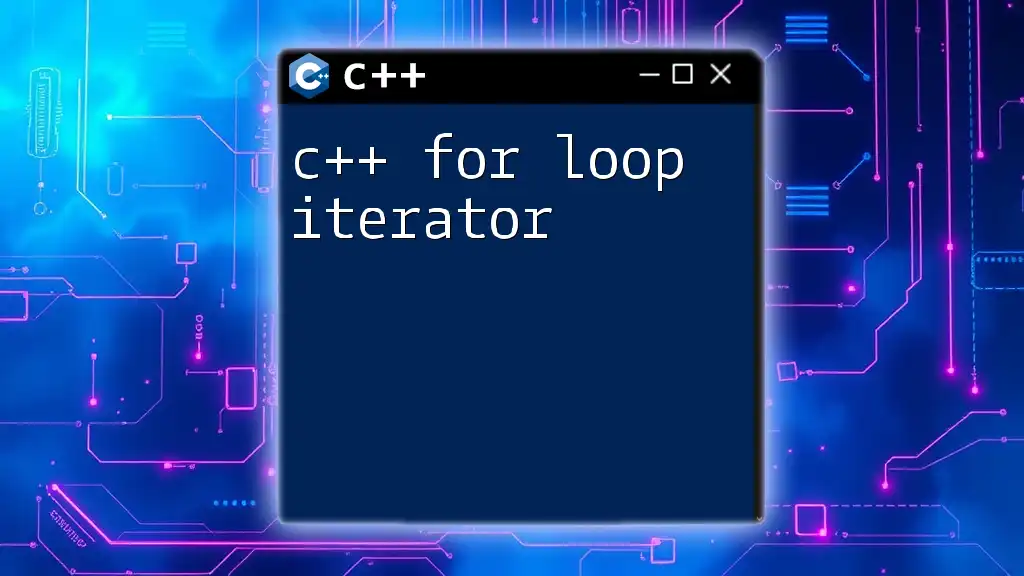
What is a For Loop?
Understanding Loops in C++
Loops are an essential part of programming, allowing you to execute a block of code multiple times. In C++, there are several types of loops, including:
- for loops: Best suited for situations where the number of iterations is known.
- while loops: Used when the number of iterations is not known in advance.
- do-while loops: Similar to while loops, but guarantees that the code executes at least once.
The For Loop Syntax
The basic syntax of a for loop in C++ includes three main components: initialization, condition, and increment. Here’s how it looks:
for (initialization; condition; increment) {
// code to execute
}
- Initialization: Sets the starting point of the loop.
- Condition: Determines whether to continue executing the loop.
- Increment: Updates the loop control variable.
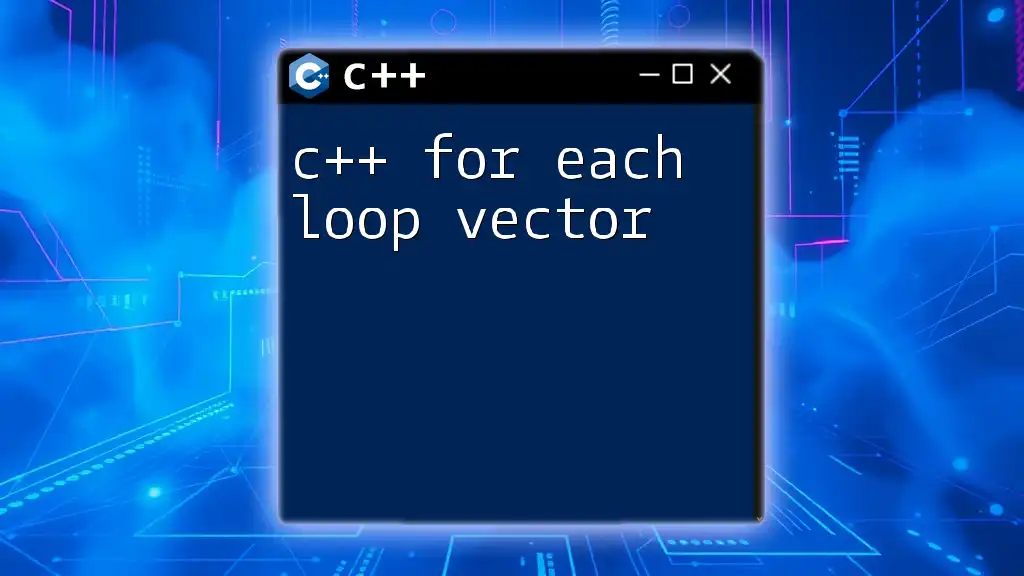
Looping Through a Vector in C++
How to Iterate Through a Vector
Iterating through a vector is vital for accessing and manipulating its elements. This process enables you to read, modify, or delete vector items as needed. Understanding how to do so efficiently can greatly enhance your programming effectiveness.
Using a For Loop to Iterate Through a Vector
Basic For Loop Example
To iterate through a vector using a basic for loop, you can use the index to access elements directly. Here’s an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
for (size_t i = 0; i < numbers.size(); ++i) {
std::cout << "Element at index " << i << ": " << numbers[i] << std::endl;
}
return 0;
}
In this example, the loop runs from `0` to `numbers.size() - 1`, printing each element of the vector.
Enhanced For Loop (C++11 and Later)
Since C++11, there's an enhanced for loop (also known as the range-based for loop) that simplifies the iteration process:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
for (int num : numbers) {
std::cout << "Element: " << num << std::endl;
}
return 0;
}
This structure eliminates the need for indices and makes your code cleaner and more readable. Each element in `numbers` is accessed directly.
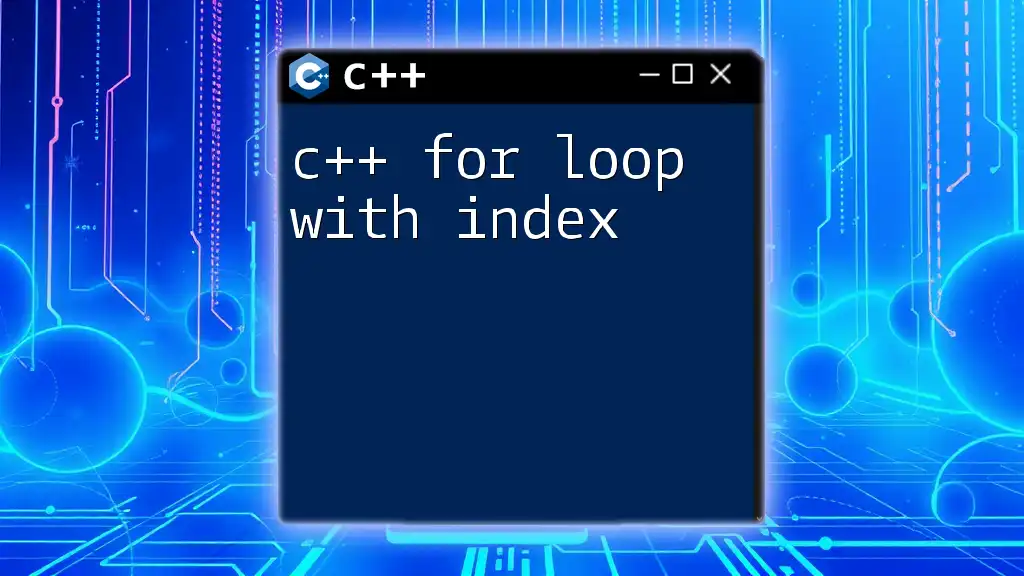
Advanced Techniques for Iterating Through Vectors
Using Iterators
What are Iterators?
Iterators are powerful tools that allow you to traverse container elements without exposing the underlying structure. They serve as a generalized pointer, providing a uniform way to access different data structures.
Example of Iterating Using Iterators
You can easily iterate through vectors using iterators, much like this:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
for (std::vector<int>::iterator it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << "Element: " << *it << std::endl;
}
return 0;
}
In this snippet, the iterator `it` starts at `numbers.begin()` and continues until it reaches `numbers.end()`, dereferencing `it` to access the elements.
Nested For Loops with Vectors
When working with multi-dimensional vectors (such as 2D vectors), nested loops are essential. Here’s how you can iterate through a 2D vector:
#include <iostream>
#include <vector>
int main() {
std::vector<std::vector<int>> matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
for (size_t i = 0; i < matrix.size(); ++i) {
for (size_t j = 0; j < matrix[i].size(); ++j) {
std::cout << "Element at (" << i << ", " << j << "): " << matrix[i][j] << std::endl;
}
}
return 0;
}
This nested loop iterates through each row and column, printing out each element in the 2D vector.
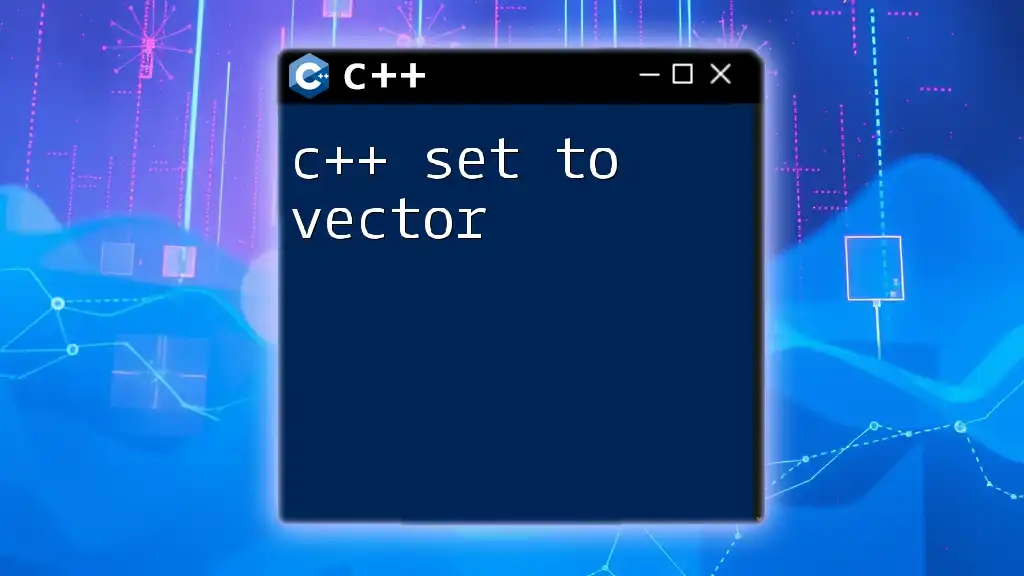
Practical Examples
Real-world Application Scenarios
Vector iteration is critical in numerous real-world applications. For example, loop through a vector of customer orders to compute the total income generated during a sales period or iterate through data points for statistical analysis.
Code Example: Summing Elements of a Vector
Here's how you can write a program to sum the elements in a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int sum = 0;
for (int num : numbers) {
sum += num;
}
std::cout << "Sum of elements: " << sum << std::endl;
return 0;
}
This code iterates through `numbers`, adding each element to the `sum` variable and printing the result.
Code Example: Finding Maximum/Minimum Values
Finding the maximum and minimum values in a vector is another typical use case:
#include <iostream>
#include <vector>
#include <limits>
int main() {
std::vector<int> numbers = {3, 5, 2, 8, 6};
int max = std::numeric_limits<int>::min();
int min = std::numeric_limits<int>::max();
for (int num : numbers) {
if (num > max) max = num;
if (num < min) min = num;
}
std::cout << "Maximum value: " << max << std::endl;
std::cout << "Minimum value: " << min << std::endl;
return 0;
}
In this example, you initialize `max` and `min` with the lowest and highest possible integer values, respectively, before checking and updating them during the iteration.
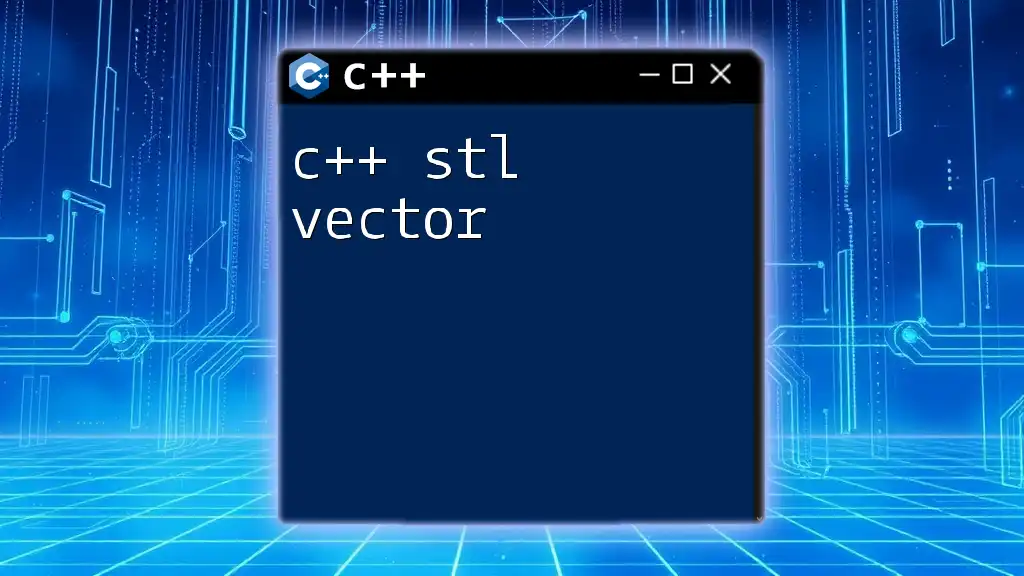
Best Practices for Looping Through Vectors
Efficient Memory Management
When using vectors, it’s crucial to manage memory effectively. Vectors automatically handle memory, but if you manipulate large amounts of data, be cautious about resizing, as this can affect performance. Always consider using `reserve()` to preallocate memory if you anticipate a significant number of elements.
Optimizing Loop Performance
Optimizing loops is essential for efficient code. Here are a few tips:
- Avoid unnecessary computations within loops. Perform calculations outside of the loop when possible.
- Use range-based for loops over traditional for loops when accessing elements, as they are often more efficient and concise.
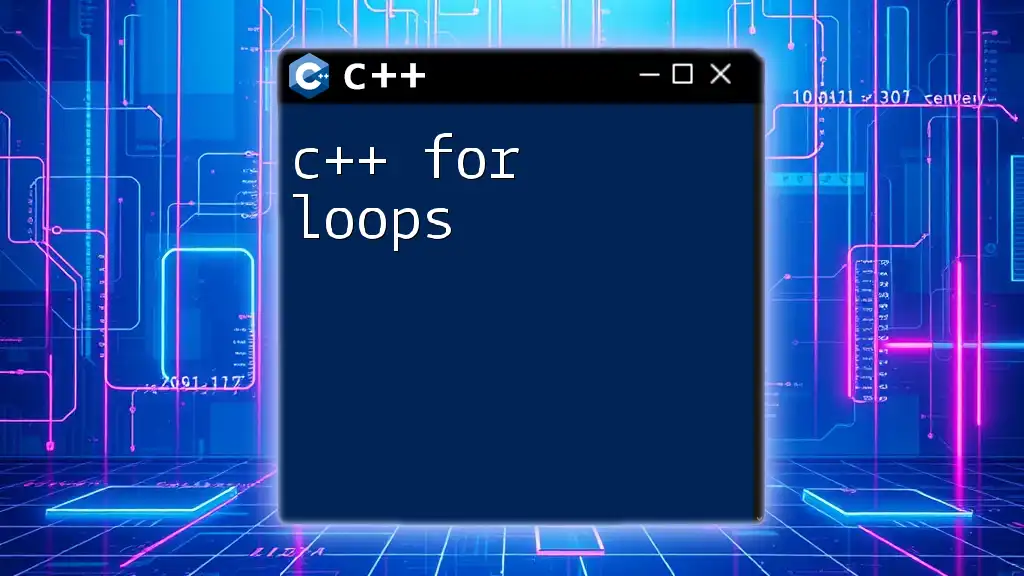
Common Mistakes to Avoid
Off-by-One Errors
One common issue in loops is the off-by-one error, which occurs when the loop runs one iteration too few or too many. Always verify your loop's termination condition, especially when using indices.
Modifying Vectors While Iterating
Modifying a vector during iteration can lead to unexpected behavior, such as skipping elements or crashing the program. If you need to remove elements, consider iterating in reverse or using a separate collection to store elements to be removed.
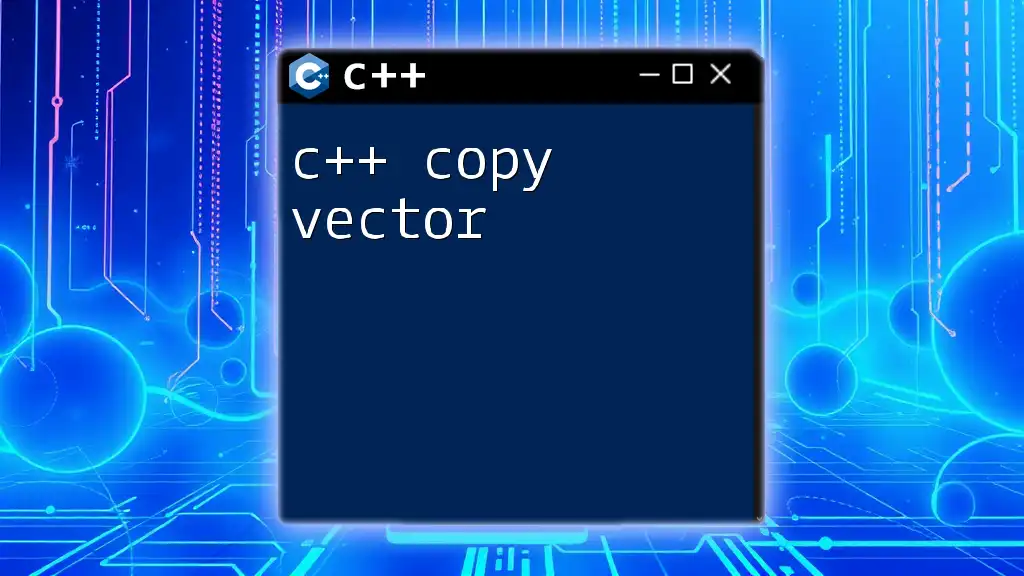
Conclusion
Understanding how to effectively use c++ for loop vector is a fundamental skill in C++ programming. The ability to iterate through vectors efficiently opens up numerous possibilities in handling data collections.
Practice by manipulating vectors in your coding projects to fully grasp these concepts. As you become more comfortable with for loops and vectors, you’ll unlock advanced techniques for data handling in your applications.
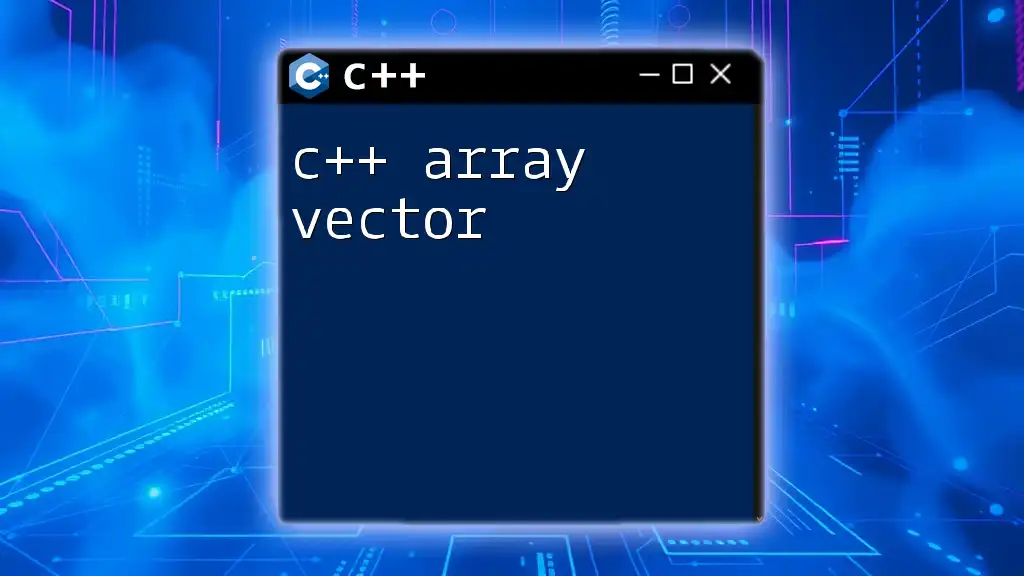
Additional Resources
Books and Tutorials
For further learning, consider exploring:
- "C++ Primer" by Stanley Lippman
- Online platforms like Codecademy, Udacity, and LeetCode for interactive tutorials.
Community and Forums
Join C++ coding communities such as Stack Overflow or Reddit's r/cpp to seek help and share knowledge.
Practice Problems
Engage with coding challenges and exercises focused on vectors and loops to strengthen your understanding and proficiency in C++.