In C++, a `std::vector` can be used in combination with `std::unique` and `std::erase` to remove duplicate elements, resulting in a unique collection of values.
Here’s a code snippet demonstrating this:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 2, 3, 4, 4, 5};
std::sort(vec.begin(), vec.end());
auto last = std::unique(vec.begin(), vec.end());
vec.erase(last, vec.end());
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is a dynamic array provided by the Standard Template Library (STL) that can grow and shrink in size, allowing for a flexible approach to data management. Unlike traditional arrays, which have a fixed size defined at compile time, vectors can adjust their capacities at runtime. This flexibility makes them a preferred choice in many coding scenarios.
Importance of Uniqueness in Vectors
When working with vectors, ensuring that the elements are unique is often vital. Duplicate entries can lead to incorrect calculations, inefficient algorithms, and potential data integrity issues. For example, if you are counting occurrences or storing unique identifiers, having duplicates would skew your results.
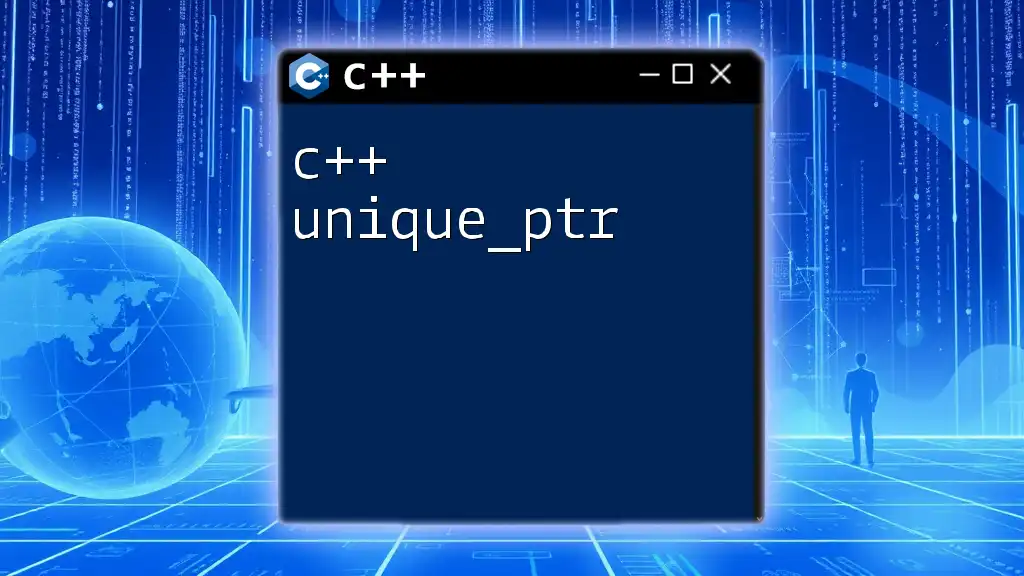
Understanding C++ Containers
Overview of C++ Standard Template Library (STL)
The STL provides a powerful set of tools for managing data through various built-in containers, algorithms, and iterators. Among these containers, vectors stand out due to their simplicity and dynamic size.
Why Use Vectors?
Vectors offer several advantages:
- Dynamic Sizing: They can grow and shrink based on the number of elements, which eliminates the need for manual memory management.
- Performance: For random access and iteration, vectors provide access times comparable to arrays while benefitting from enhanced functionalities.
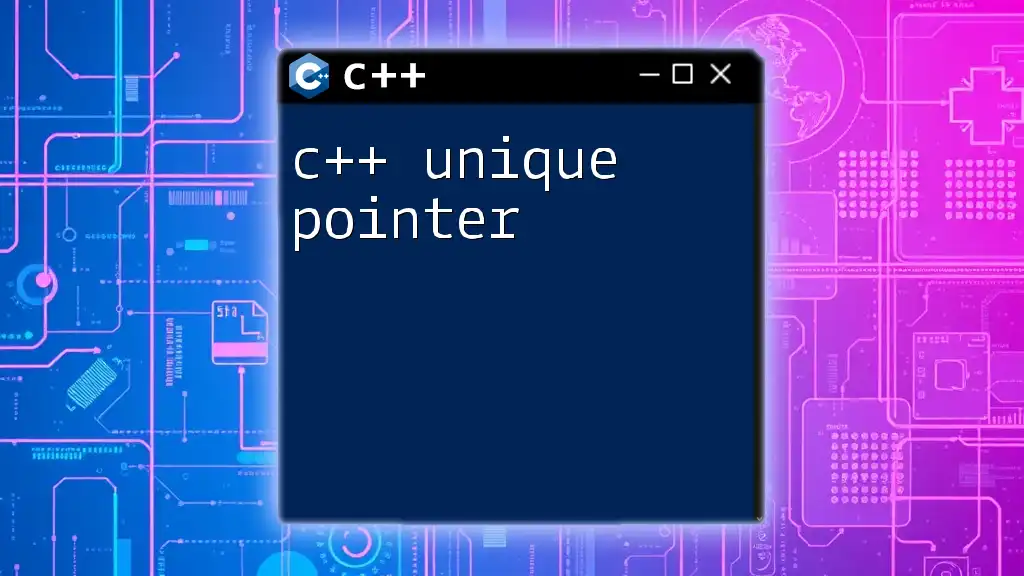
Creating and Initializing C++ Vectors
How to Create a Vector
Creating a vector in C++ is as simple as including the vector header and using the syntax `std::vector<Type> name;`. Here’s an example of creating a vector of integers:
#include <iostream>
#include <vector>
std::vector<int> myVector; // Creating an empty vector of integers
Initializing Vectors with Multiple Values
You can initialize vectors with a list of values, which allows for quick data entry. Here's how it can be done:
std::vector<int> myVector = {1, 2, 3, 4, 5}; // Initializer list
Reserve vs. Resize
Using `reserve()` allocates space for a certain number of elements without changing the size of the vector, while `resize()` changes the size of the vector.
myVector.reserve(10); // Reserves space for 10 elements
myVector.resize(5); // Resizes the vector to hold 5 elements
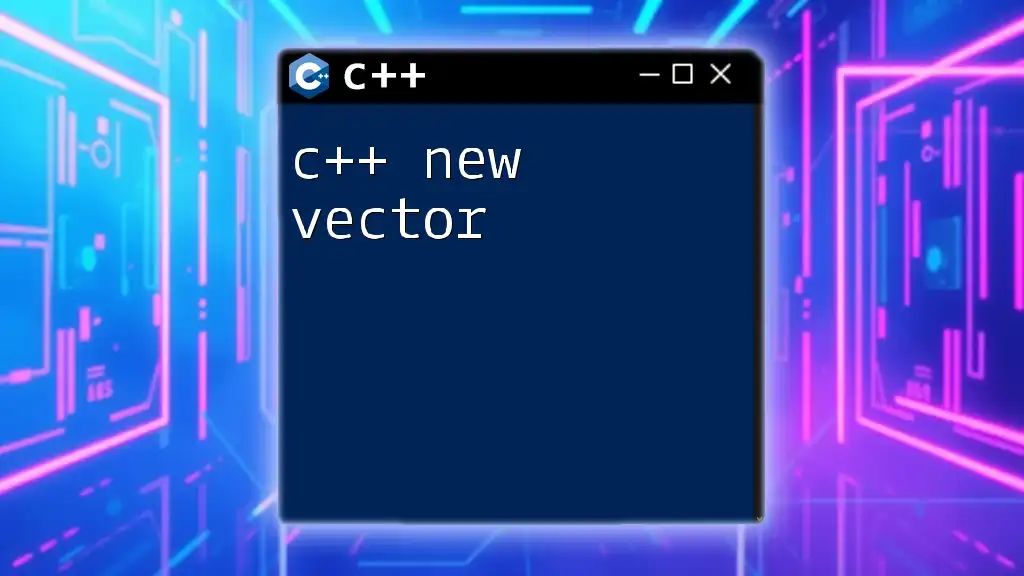
The Need for Unique Elements in a C++ Vector
Common Scenarios Requiring Uniqueness
In many applications, ensuring that a vector holds only unique elements can be crucial. Examples include:
- Eliminating Duplicate User IDs: In databases, duplicate entries can lead to unauthorized access or data corruption.
- Performance Optimization: Algorithms that rely on unique datasets can run more efficiently without unnecessary comparisons.
Algorithms and Utilities for Uniqueness
C++ provides various algorithms that can help achieve uniqueness. Notably, `std::unique` and `std::sort` can be employed effectively to handle duplicates in vectors.
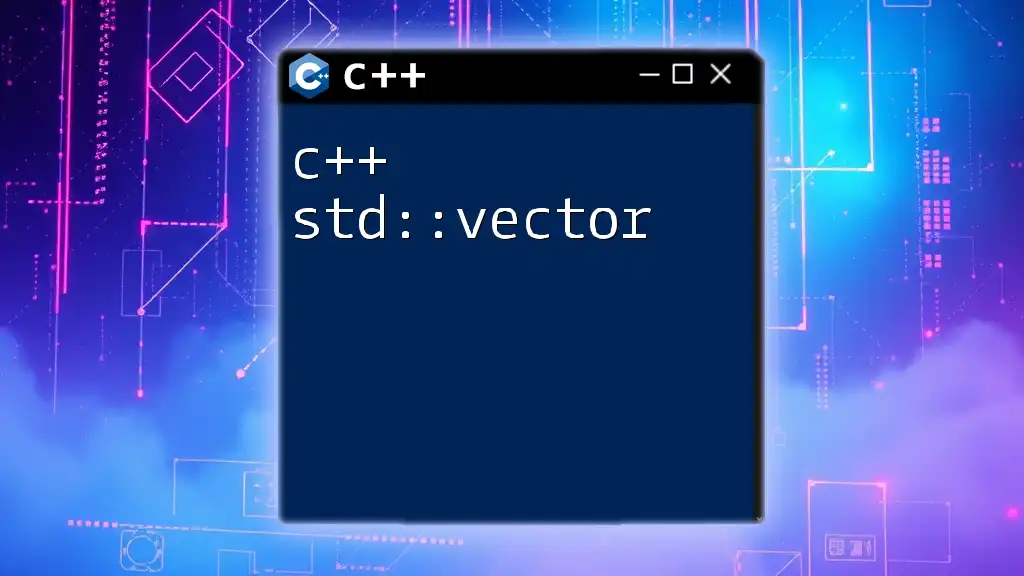
Making a C++ Vector Unique
Step-by-Step Guide to Ensure Uniqueness
Sorting the Vector
Before removing duplicates, it’s essential to sort the vector. This step ensures that duplicates are adjacent, making it easy to filter them out later.
std::sort(myVector.begin(), myVector.end()); // Sorting the vector
Applying std::unique
Once sorted, `std::unique` can be used to reorder the vector such that unique elements appear before the end of the vector range. It returns an iterator to the new end of the vector:
auto last = std::unique(myVector.begin(), myVector.end());
Resizing the Vector
After applying `std::unique`, the vector will still maintain its original size. To remove the extra elements, you need to resize it:
myVector.resize(std::distance(myVector.begin(), last)); // Resizing to new length
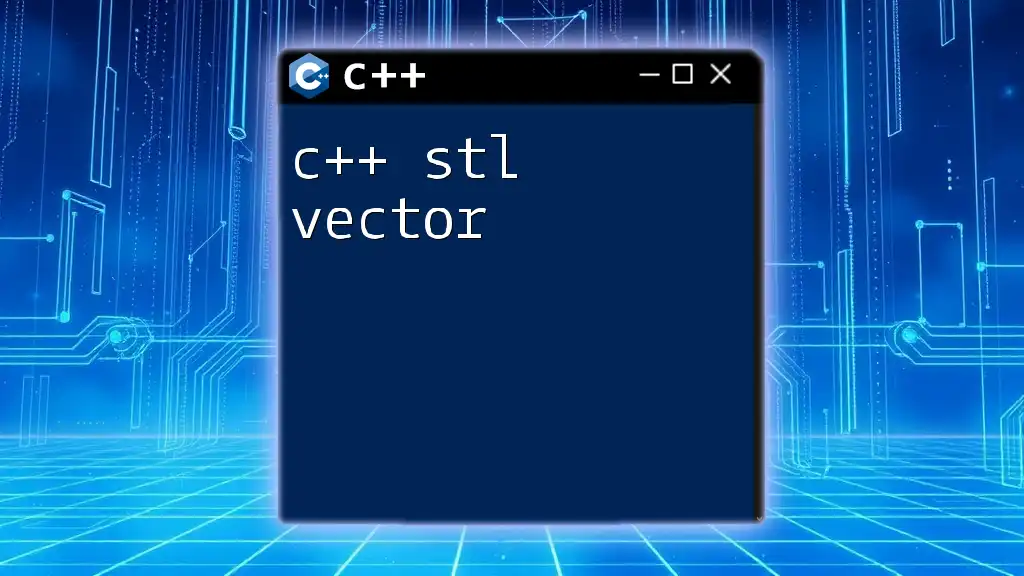
Advanced Techniques for Unique Vectors
Using std::unordered_set
For scenarios where performance is critical, `std::unordered_set` can be employed since it automatically handles uniqueness while allowing for fast insertion and lookup times.
#include <unordered_set>
std::unordered_set<int> uniqueSet(myVector.begin(), myVector.end());
Combining std::vector with std::set
Another option is to convert the vector into a set, which inherently stores only unique elements. This method can be beneficial if you plan to frequently add or check for duplicates.
std::set<int> uniqueSet(myVector.begin(), myVector.end());
std::vector<int> uniqueVector(uniqueSet.begin(), uniqueSet.end()); // Back to vector
Functional Programming Approach
Leveraging lambda functions, you can filter out duplicates directly during insertion or processing.
std::vector<int> uniqueVector;
std::copy_if(myVector.begin(), myVector.end(), std::back_inserter(uniqueVector),
[&uniqueSet](int n) { return uniqueSet.insert(n).second; });
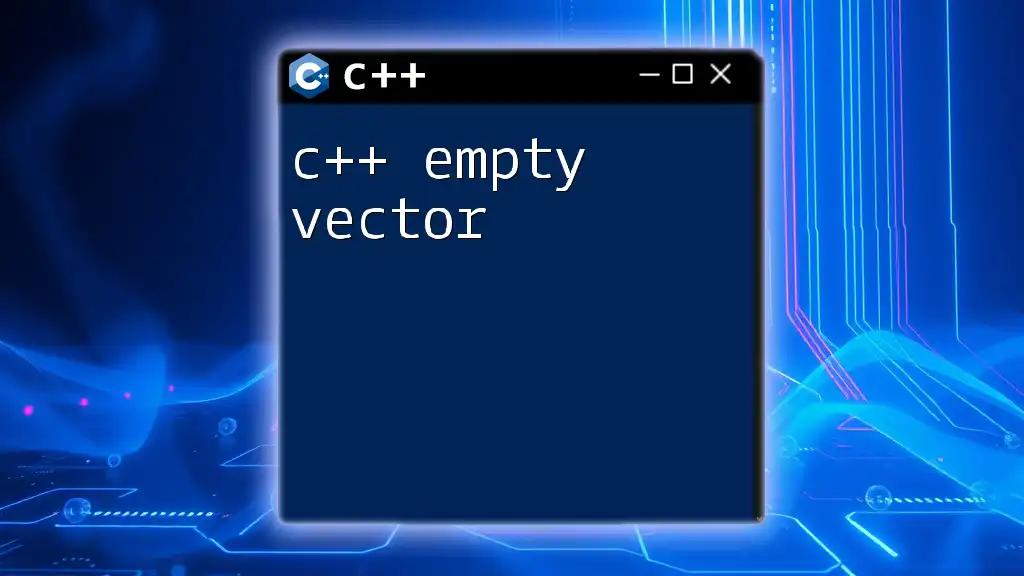
Performance Considerations
Time Complexity of Uniqueness Operations
Sorting a vector has a time complexity of O(n log n), while `std::unique` runs in O(n). Thus, ensuring uniqueness with these operations is relatively efficient.
Trade-offs Between Various Data Structures
When choosing between vectors, sets, or maps, consider your specific needs:
- Vectors are ideal if you need ordered data and random access.
- Sets are more suitable for scenarios where frequent insertions and uniqueness are paramount.
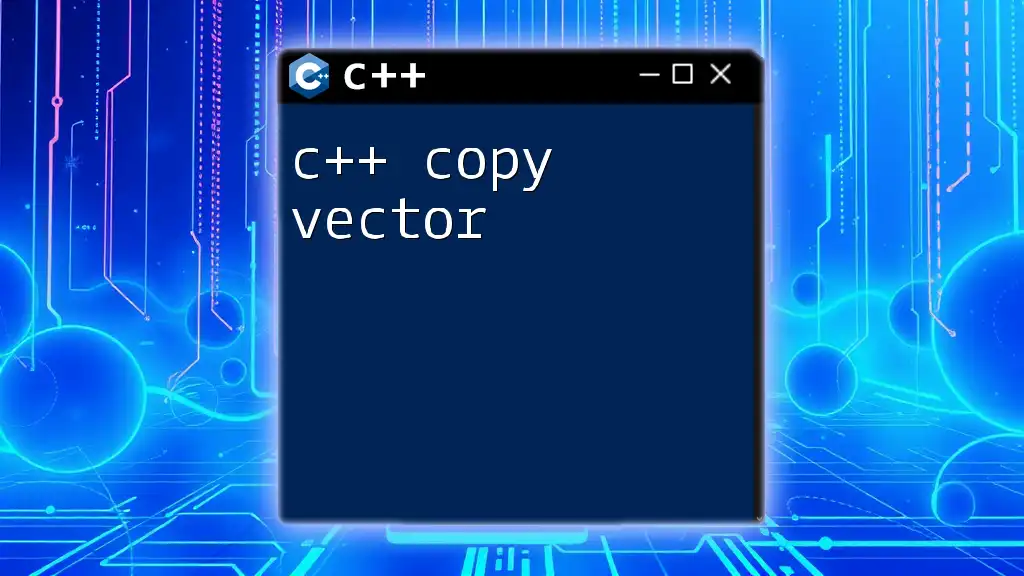
Real-Life Use Cases
Example Applications of Unique Vectors
Uniqueness is a common requirement. For instance, in web development, tracking unique page visits or managing unique usernames can be critical for maintaining system integrity.
Project Ideas
Consider creating a small project that validates user inputs to ensure uniqueness in a list or managing a music playlist that avoids duplicate songs.
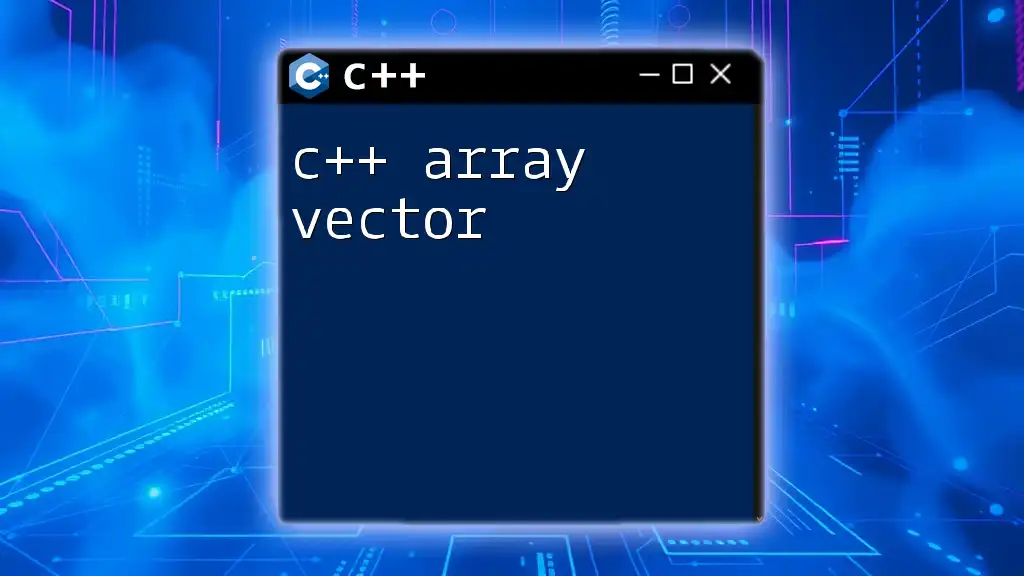
Conclusion
The significance of maintaining uniqueness within a C++ vector cannot be overstated. By utilizing the appropriate STL algorithms and data structures, you can ensure efficient management of your data, leading to improved performance and reliability in your applications. Embrace the concepts and techniques discussed here, and feel free to experiment with your projects to reinforce your understanding of C++ unique vector functionality.