To download and set up Eclipse for C++ development, you can visit the official Eclipse website and choose the "Eclipse IDE for C/C++ Developers" package.
// Example C++ code to display "Hello, World!" in Eclipse
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Eclipse?
Overview of Eclipse
Eclipse is a powerful Integrated Development Environment (IDE) that caters to a diverse range of programming languages, including C++. Prominent for its flexibility and extensibility, Eclipse allows developers to create applications with optimized ease. Its robust features include a sophisticated code editor, built-in debugger, and comprehensive build tools that streamline the software development process. This makes it an ideal choice for both beginners and seasoned developers alike.
Benefits of Using Eclipse for C++
Using Eclipse for C++ development presents numerous advantages:
-
Customizable Interface: Eclipse provides a highly customizable interface that adapts to different programming styles. You can modify toolbars and layouts to suit your workflow for enhanced productivity.
-
Rich Plugin Ecosystem: With a wide array of plugins available, users can easily extend the functionality of their IDE. This ensures that you can build your development environment tailored specifically to your needs.
-
Strong Community Support: A vast and active community surrounds Eclipse, offering forums, documentation, and plugins that ensure developers can find solutions to their queries and learn from shared experiences.
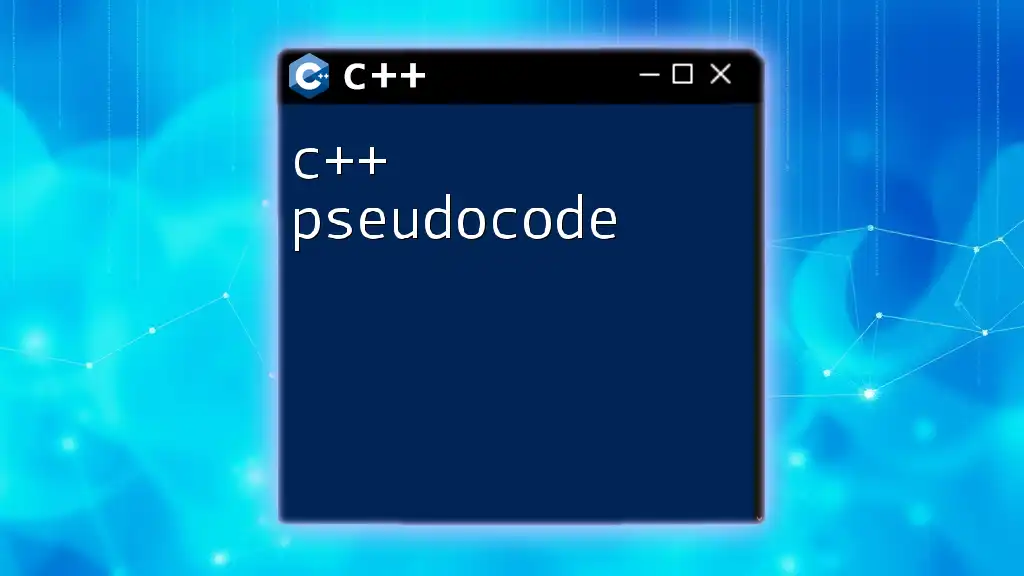
How to Download Eclipse for C++
Minimum System Requirements
Before diving into the C++ Eclipse download, it's essential to ensure your system meets the minimum requirements to run Eclipse effectively:
- Operating Systems: Windows 10 or later, macOS (various versions), or a recent Linux distribution.
- RAM: At least 4 GB, though 8 GB or more is recommended for optimal performance.
- Disk Space: A minimum of 1 GB of free disk space to install the IDE and additional space for your projects.
Steps to Download Eclipse for C++
To download Eclipse for C++, follow these steps:
-
Visit the Official Eclipse Website: Directly navigate to the [Eclipse downloads page](https://www.eclipse.org/downloads/).
-
Select the Right Eclipse Package: Look for the package titled "Eclipse IDE for C/C++ Developers." This version includes all the essential tools and plugins for C++ programming, ensuring a smooth development experience.
Download Process
After selecting the appropriate Eclipse package, proceed with the following steps:
-
Click on the Download Link: This will redirect you to the latest release for your operating system.
-
Choose Your Operating System: Make sure to select "Windows," "macOS," or "Linux," depending on what you're using.
-
Download the Installer File: Begin downloading the installer by clicking the relevant link. Ensure you have a stable internet connection for a smooth download.
Alternative Download Options
In addition to the official website, Eclipse is often available through repositories and package managers. For instance:
-
GitHub: Certain versions of Eclipse packages can be found on GitHub, which may offer quicker access for developers familiar with Git.
-
Direct Download of Compressed Files: For more advanced users, downloading a compressed file (like .zip or .tar.gz) gives you the option to manually install Eclipse without an installer.
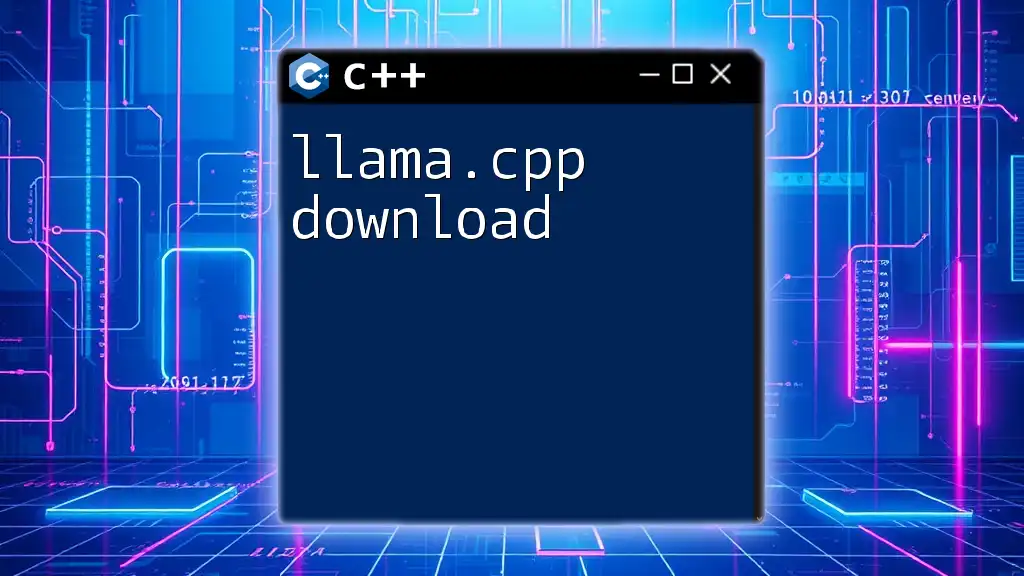
Installing Eclipse for C++
Installation Steps
Once you have successfully downloaded the installer, follow these steps to install Eclipse:
-
Run the Installer: Locate the downloaded installer and double-click to launch it.
-
Specify Installation Directory: Choose a directory where you want Eclipse to be installed. If you have a preferred location, feel free to direct the installer there.
-
Complete the Installation Process: Follow the on-screen instructions to finish the installation. Ensure that you grant any permissions requested by the installer to allow it to run seamlessly.
Initial Configuration
After installing Eclipse, setting up the IDE for your projects is crucial:
-
Configuring Workspace Settings: Upon running Eclipse for the first time, you'll be prompted to select a workspace location. This folder is where your projects will be stored, so it's best to choose a clean directory. You can change this later if needed.
-
Importance of Workspace Folder: A well-organized workspace can enhance your development experience by maintaining easy access to all your projects.
Installing Necessary Plugins
To maximize the functionality of Eclipse for C++, you should install the required plugins, with the C/C++ Development Tools (CDT) being the primary one. Here's how:
-
Accessing Eclipse Marketplace: In Eclipse, navigate to `Help` > `Eclipse Marketplace`.
-
Searching for CDT: Use the search bar to look for "C/C++ Development Tools". Follow the prompts to install the plugin.
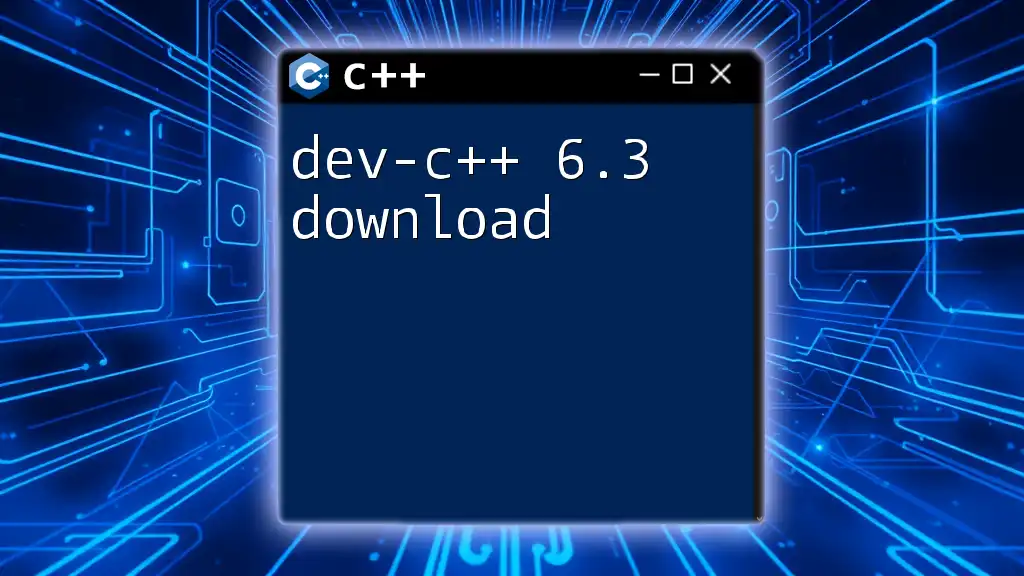
First Steps in Eclipse for C++
Creating a New C++ Project
Once Eclipse is set up, you can create your first C++ project:
-
Open Eclipse and Select "New Project": Go to `File` > `New` > `C Project`.
-
Choosing Project Type: Select a suitable project type, such as "Executable" or "Static Library," and click `Next`.
-
Name Your Project: Enter a meaningful name for your project that corresponds to the task at hand.
Writing Your First C++ Program
Now, let’s write a simple C++ program— a classic "Hello World" example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Compiling and Running C++ Programs
To compile and run your C++ program:
-
Building Your Project: Click on `Project` > `Build Project`. Eclipse will compile your code. If there are errors, check the console for error messages and fix them accordingly.
-
Running the Program: Once your project builds successfully, click the green run button (play icon) in the toolbar. This will execute your program, and you should see "Hello, World!" printed in the console.
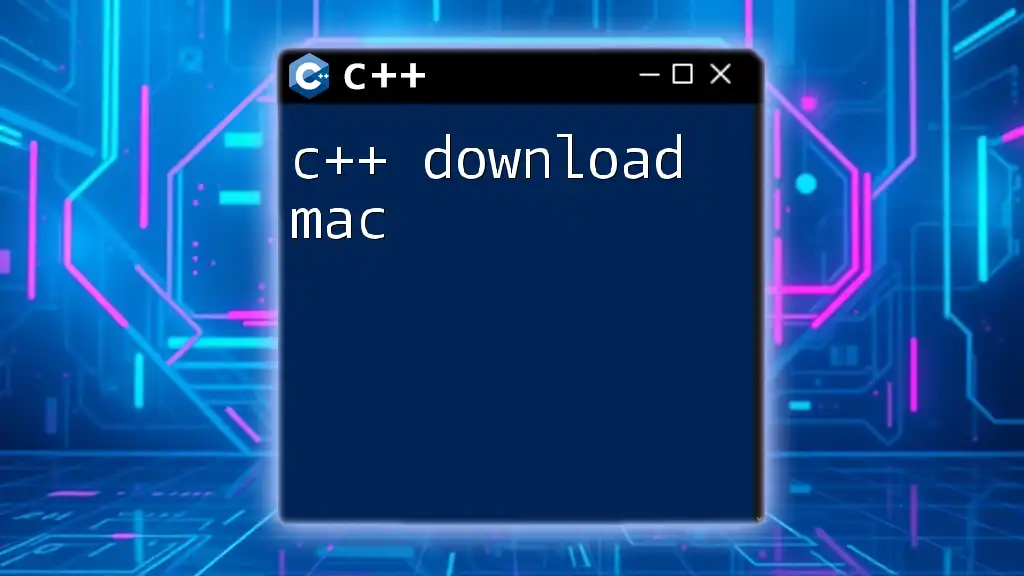
Troubleshooting Common Issues
Installation Issues
During the installation process, you may encounter several common problems:
- Installation Fails: This may occur if your system does not meet the minimum requirements or if there are permission issues. Ensure your user account has administrative privileges and consult the official Eclipse FAQs if issues persist.
Configuration Problems
Configuration issues can hinder your programming experience. Here are tips to resolve them:
- Path Issues: If you have installed compilers, ensure their paths are correctly set in Eclipse under `Preferences` > `C/C++` > `Build` > `Environment`.
Performance Improvements
Improving Eclipse's performance can significantly enhance your programming experience:
-
Memory Settings: Open the `eclipse.ini` file in your Eclipse installation directory. Adjust memory settings, increasing the `-Xms` and `-Xmx` values to allocate more memory.
-
Workspace Management: Regularly clean your workspace by removing unused projects and organizing your files effectively.
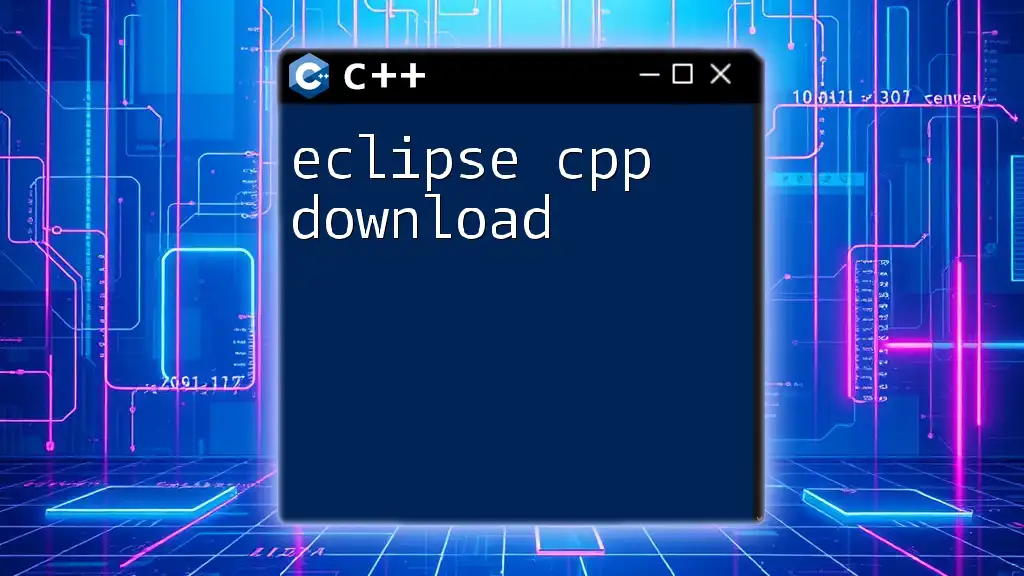
Conclusion
Downloading and setting up Eclipse for C++ development is essential for any programmer looking to streamline their workflow. With its powerful features and extensive plugin support, Eclipse remains a top choice among developers, enabling them to code efficiently. Start exploring its capabilities today and delve deeper into the world of C++ programming.
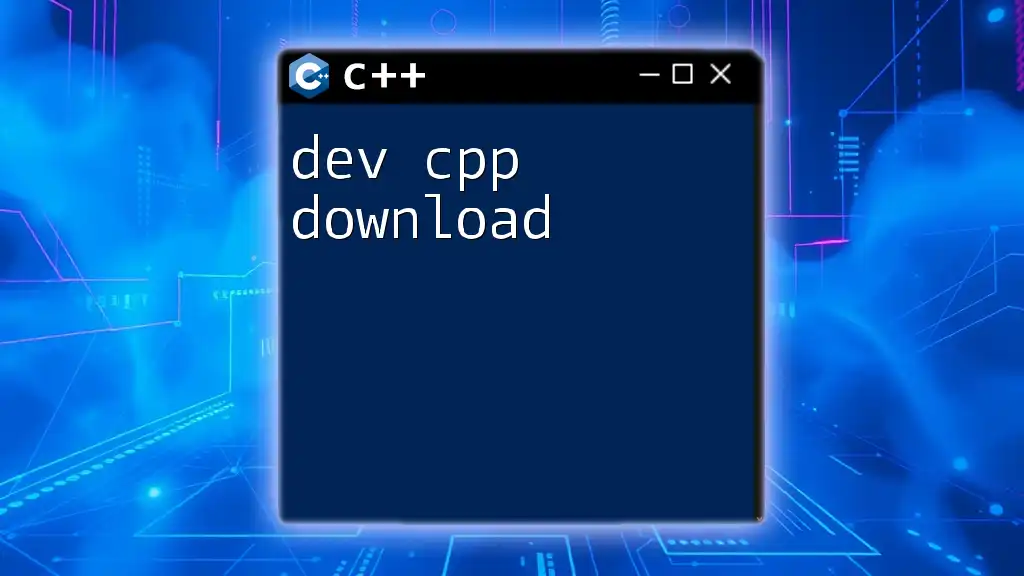
Call to Action
If you found this guide helpful, consider joining our community or subscribing for additional resources and tutorials on C++ and Eclipse to further enhance your development skills.
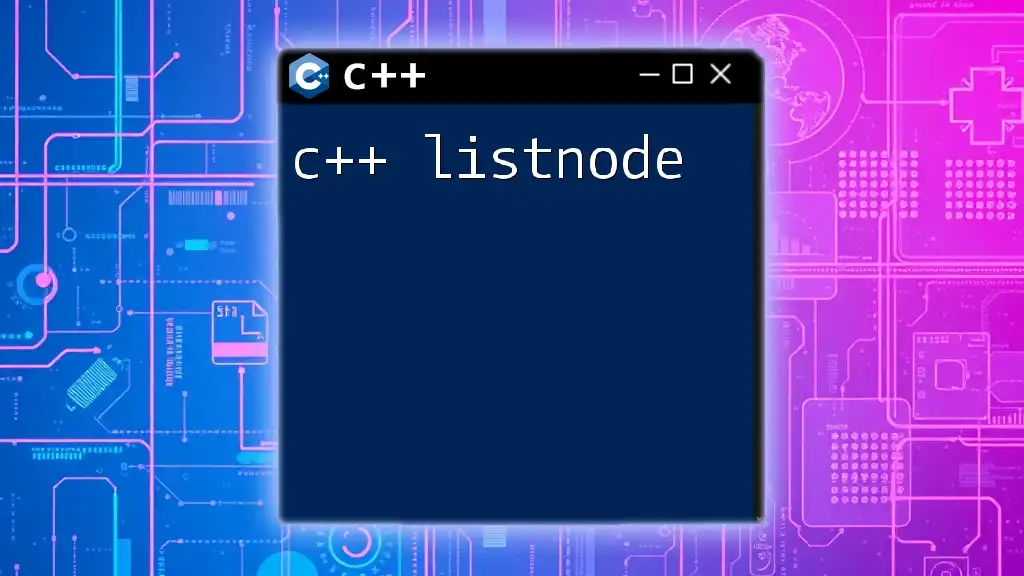
Additional Resources
For further exploration, check out the official Eclipse documentation, engage in community forums, and view other learning materials that can facilitate your journey in mastering C++ development.