To download and install C++ on Windows 11, you can obtain the latest version of Microsoft Visual Studio by visiting the official website and following the installation instructions.
// Example C++ code to print "Hello, World!" after setup
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ and Its Uses
What is C++?
C++ is a powerful and versatile programming language created by Bjarne Stroustrup in the early 1980s. It provides support for both procedural and object-oriented programming paradigms, allowing developers to create efficient and high-performance applications. Key features of C++ include:
- Rich set of libraries that support various functionalities.
- Low-level memory manipulation capabilities, which gives developers fine control over system resources.
- Type safety and the ability to create user-defined types.
Use Cases for C++
C++ is widely utilized across numerous domains, making it a great language to learn and master. Common applications include:
- Game Development: Many leading game engines, such as Unreal Engine, utilize C++ for its performance and memory control.
- System/Software Development: Operating systems and software applications often rely on C++ for system-level programming.
- Embedded Systems: C++ is essential in developing software for hardware devices due to its efficiency.
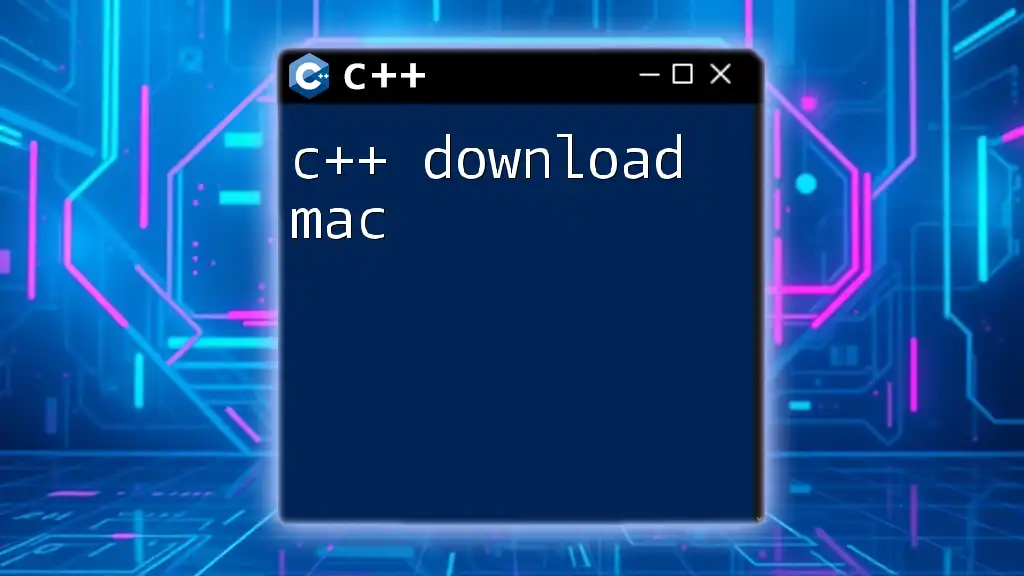
Preparing Your Windows 11 System for C++
System Requirements
Before proceeding with a C++ download for Windows 11, it is essential to ensure your system meets the necessary requirements.
- Minimum Requirements: A modern CPU, at least 4 GB of RAM, and 2 GB of free disk space are generally sufficient for basic programming.
- Recommended Specifications: For a smoother development experience, consider an 8 GB RAM configuration or more, along with a solid-state drive (SSD).
Installing Windows Update
A crucial first step before downloading C++ is to ensure your Windows 11 is fully updated. Regular updates not only provide security patches but also improve system performance.
- Open Settings.
- Select Windows Update.
- Click on Check for updates and install any pending updates.
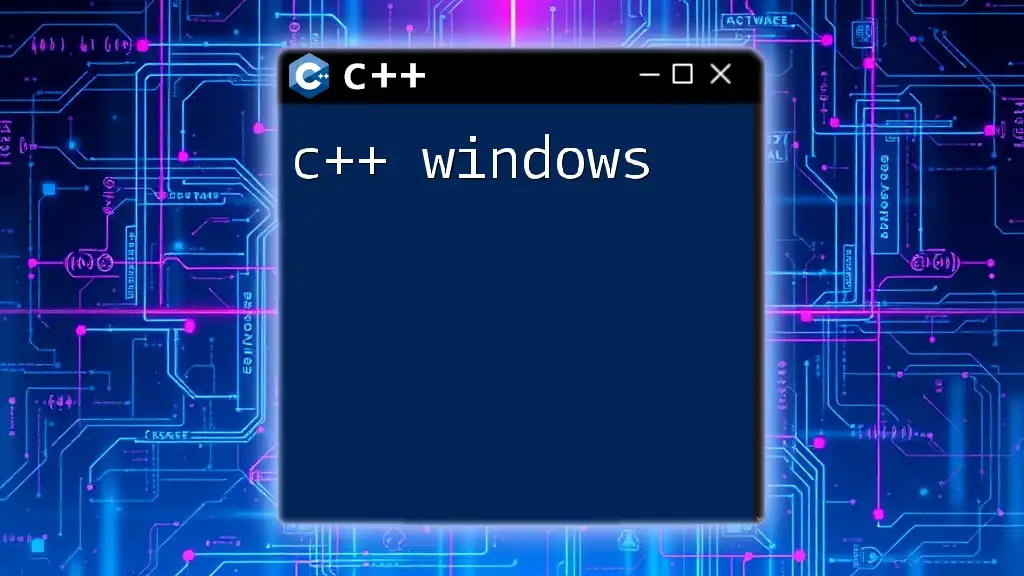
Downloading a C++ Compiler for Windows 11
What is a Compiler?
A compiler translates the source code written in C++ into machine code that your computer can execute. Choosing a good compiler is critical, as it impacts the efficiency and performance of your applications.
Popular C++ Compilers for Windows 11
Microsoft Visual Studio Community Edition
Visual Studio is one of the most popular IDEs for C++ development. It's free for individual developers, students, and open-source projects.
- Visit the [Visual Studio Download Page](https://visualstudio.microsoft.com/vs/community/).
- Click on Download and run the installer.
- Choose the Desktop Development with C++ workload and click Install.
Example setup process: Once installed, you can create your first program using Visual Studio. Begin by opening Visual Studio and selecting Create a new project. Choose Console App, name it, and write the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Code::Blocks
Code::Blocks is an open-source IDE that is lightweight and user-friendly.
- Go to the [Code::Blocks official website](http://www.codeblocks.org/downloads/26).
- Download the binary release that includes MinGW and follow the installation prompts.
Example setup process: Open Code::Blocks, create a new project, and select Console Application. Name your project and enter the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
MinGW (Minimalist GNU for Windows)
MinGW is a minimalist development environment for native Microsoft Windows applications.
- Visit the [MinGW website](https://osdn.net/projects/mingw/releases/).
- Download the latest version of the installer and follow the prompts.
Example setup process: After installing MinGW, ensure you add the MinGW bin directory to your system PATH for ease of use in command-line operations. Create a simple C++ file, and use the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
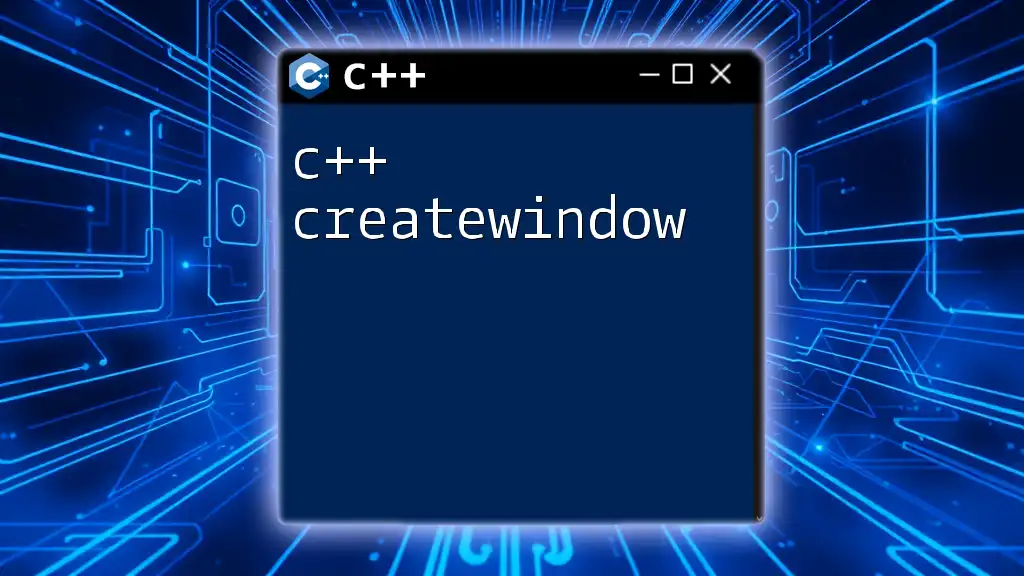
Setting Up Your C++ Development Environment
Configuring the Compiler
Once you have downloaded your C++ compiler, proper configuration is vital to ensure smooth operation.
Visual Studio Configuration
In Visual Studio, open the Tools menu and navigate to Options. Check the settings for C++ and configure paths, additional include directories, and library directories as needed.
Code::Blocks Configuration
In Code::Blocks, go to Settings > Compiler. Ensure that the selected compiler is correctly set up and that the paths point to your MinGW installation.
MinGW Configuration
After downloading MinGW, open the Environment Variables settings on Windows. Under System Variables, find the Path variable and add the path to the MinGW `bin` directory. This enables you to use the `g++` command from any command prompt.
Writing Your First C++ Program
To write your first C++ program, open your chosen IDE, create a new file, and include the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This basic program outputs "Hello, World!" to the console and demonstrates the foundational structure of a C++ program.
Compiling Your C++ Program
In Visual Studio, click on the Build > Build Solution option, while in Code::Blocks, navigate to Build > Build. For MinGW, you can compile your program through the command prompt by navigating to the folder containing your file and using:
g++ -o HelloWorld HelloWorld.cpp
Replace `HelloWorld.cpp` with the name of your file. This command generates an executable file named HelloWorld, which you can run in the console.
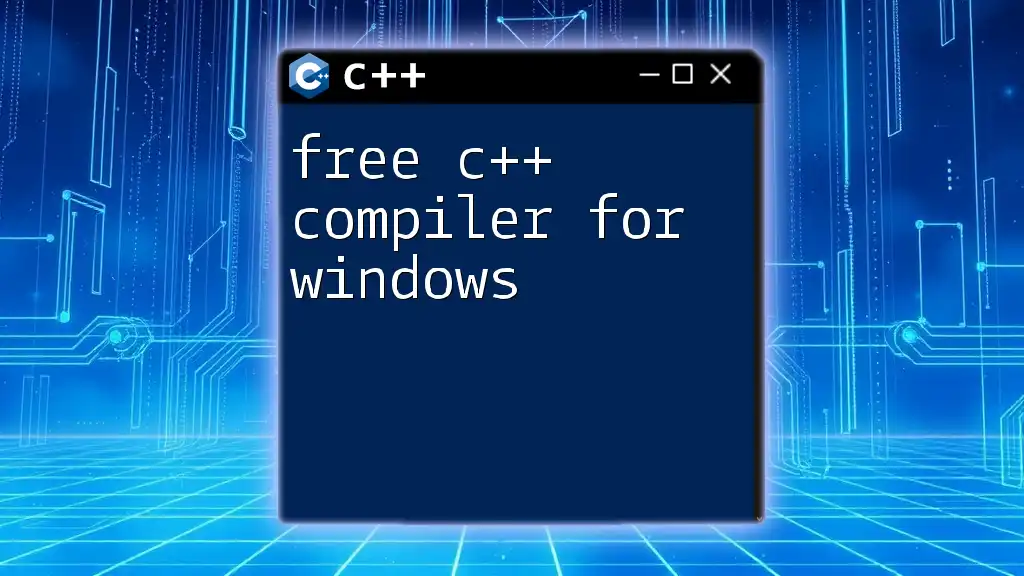
Additional Tools for C++ Development
Debugging Tools
Debugging is an integral part of software development. Familiarizing yourself with debugging tools in your IDE can tremendously improve your coding efficiency. Tools like gdb (available with MinGW) and built-in debuggers in Visual Studio and Code::Blocks help you identify and fix errors quickly.
Version Control
Understanding the significance of version control can take your C++ project management to the next level. Tools like Git allow you to track changes, collaborate with others, and revert back to previous code versions. Installing Git on Windows can be done easily, and utilizing platforms like GitHub can further enhance your coding experience and encourage collaboration.
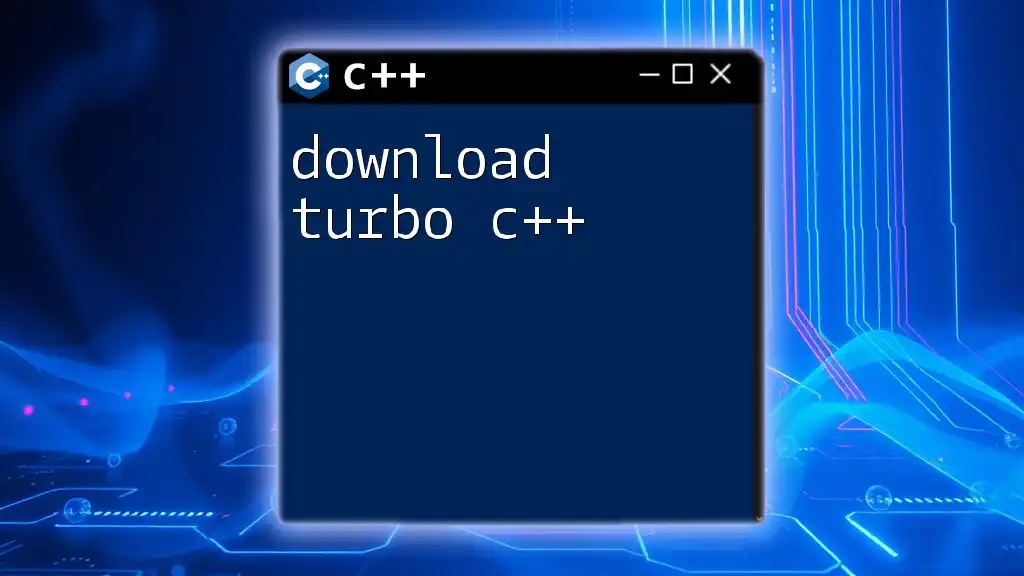
Resources for Learning C++
Online Tutorials and Courses
As you embark on your C++ journey, leveraging online resources is crucial. Websites like Codecademy, Coursera, and freeCodeCamp offer comprehensive tutorials that can help you grasp the fundamentals and advance your skills.
Communities and Forums
Participating in programming forums and communities is highly beneficial. Websites like Stack Overflow and Reddit’s r/cpp provide a platform to ask questions, share knowledge, and connect with fellow C++ enthusiasts.
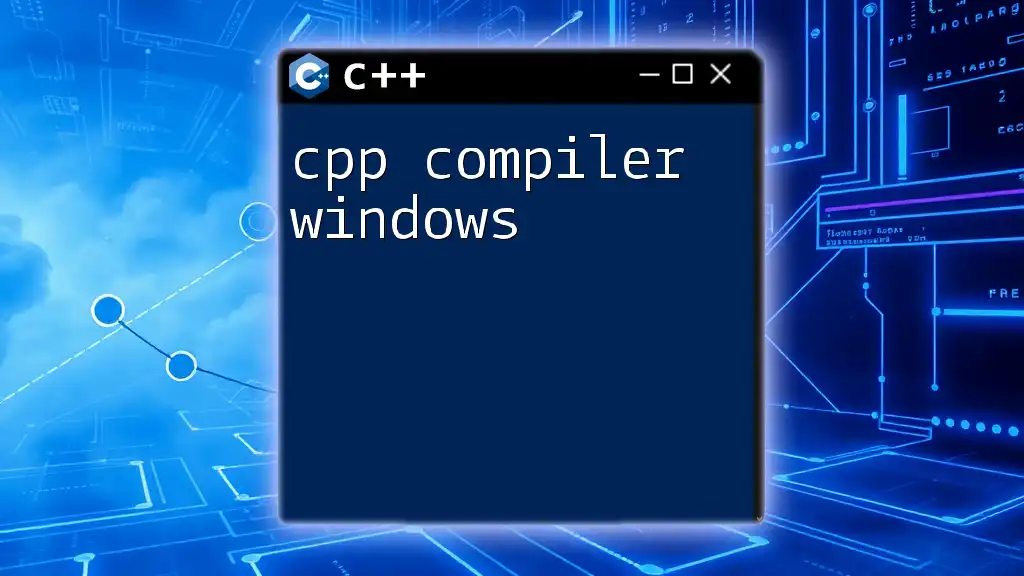
Conclusion
Downloading and setting up C++ on Windows 11 is a straightforward process that opens up a world of programming possibilities. By following the steps outlined, you'll be well on your way to creating your own programs and applications. As you continue learning, utilize the ample resources and communities available to sharpen your skills. Get coding!
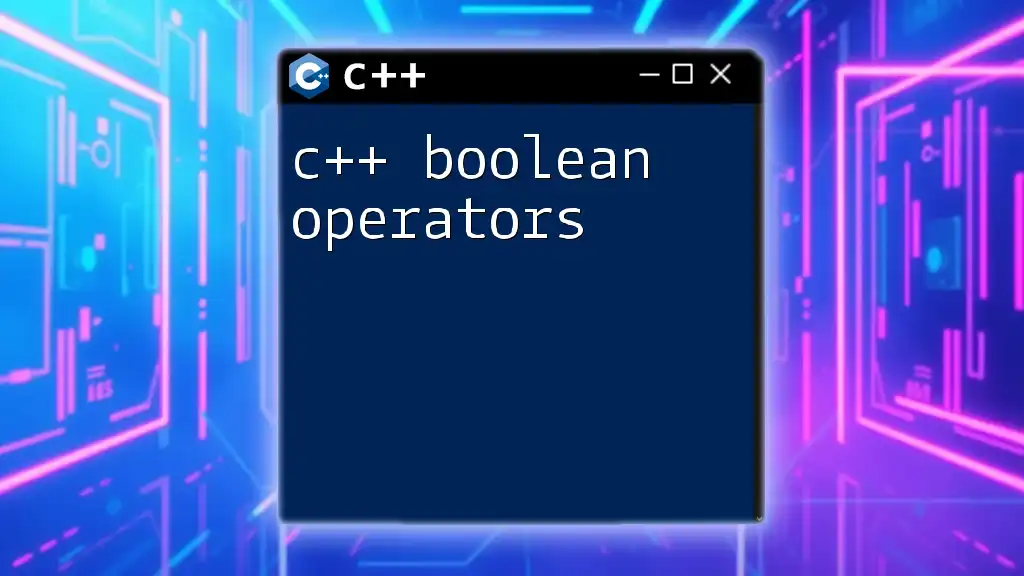
Call to Action
Now that you are equipped with the knowledge to download C++ for Windows 11, don’t hesitate to subscribe for more tips and insights on mastering C++. Share your first C++ program with us in the comments and let’s embark on this journey together!