Compiling C++ code involves converting your human-readable C++ source files into executable machine code using a compiler like g++, which can be done with a simple command line instruction.
g++ -o output_program source_file.cpp
Understanding C++ Compilation
What is Compilation?
Compilation is the process of translating source code written in C++ into machine code that the computer's processor can understand and execute. This involves several crucial stages:
-
Preprocessing: This initial stage processes directives that begin with `#`, such as `#include` or `#define`. The preprocessor handles directives before passing the code to the compiler, making it ready for compilation.
-
Compilation: The core compilation stage takes the preprocessed code and converts it into assembly language specific to the target architecture. This step includes syntax checking, type checking, and generating an intermediate object code.
-
Assembly: In this stage, the assembler translates the assembly language code into machine code, producing an object file with a `.o` or `.obj` extension.
-
Linking: Finally, the linker combines the object files into a single executable. It resolves references between files and includes libraries needed for functions not defined in the code.
Each stage plays a vital role in producing a functioning executable, and understanding this flow is crucial for effective C++ programming.
Common C++ Compilers
Several compilers are widely used to compile C++ code, each with distinct advantages:
-
GCC (GNU Compiler Collection): A popular, open-source compiler that supports multiple programming languages, including C++. It is known for its speed and cross-platform compatibility.
-
Clang: Another open-source compiler that boasts fast compilation times and excellent tools for static analysis. Clang is often favored in development environments for its helpful error messages.
-
MSVC (Microsoft Visual C++): This is the default compiler for Windows development. It is tightly integrated with Visual Studio and tailored for Windows applications, making it particularly popular among Windows developers.
Each compiler has its own set of features and optimizations, so the choice often depends on the project requirements and development environment.
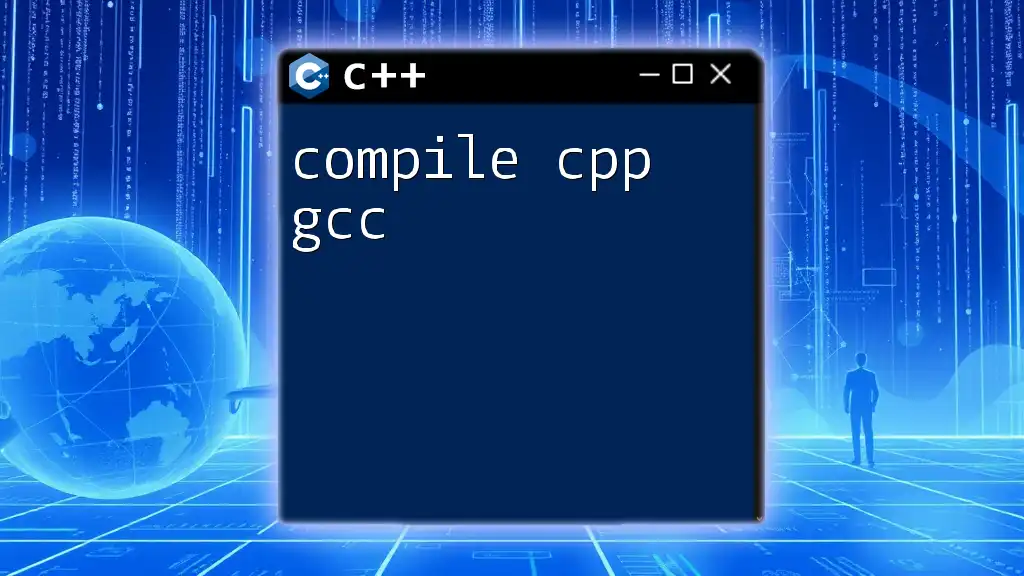
Environment Setup
Installing a C++ Compiler
To compile CPP code successfully, one must first install a suitable compiler. Here’s how to set up GCC on different platforms:
-
Windows:
- Download the MinGW (Minimalist GNU for Windows) installer and select the `g++` component.
- Follow the installation prompts.
- Ensure MinGW’s `bin` directory is added to the system’s PATH.
-
macOS:
- Install Xcode from the App Store, which includes the Clang compiler.
- Alternatively, install the Command Line Tools with the command `xcode-select --install`.
-
Linux:
- Use the package manager relevant to your distribution. For example:
sudo apt install g++
- Use the package manager relevant to your distribution. For example:
Setting Up an Integrated Development Environment (IDE)
An Integrated Development Environment (IDE) can greatly enhance productivity while coding in C++. An IDE provides features like syntax highlighting, code completion, and debugging tools.
Recommended IDEs include:
- Visual Studio: Ideal for Windows users, it offers a rich set of tools and integrations.
- Code::Blocks: A cross-platform and extensible IDE for C++.
- CLion: A powerful IDE from JetBrains that supports CMake and has intelligent coding assistance.
Guidelines to Configure the IDE:
- Install your chosen IDE.
- Set it to recognize the installed C++ compiler, often found in the settings or preferences menu.
- Create a new project to organize your code files easily.
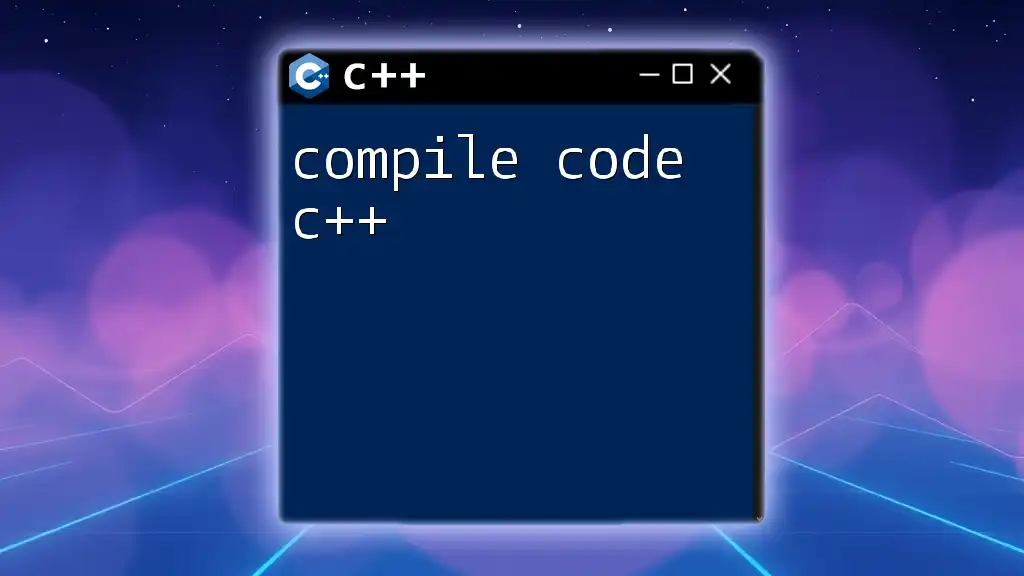
How to Compile a Program in C++
Creating a Simple C++ Program
Let's start with a basic example—a simple "Hello World" program. Here’s what the code looks like:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Explanation of the code components:
- `#include <iostream>`: This header allows use of input and output streams.
- `using namespace std;`: This line avoids the need to prefix standard library functions with `std::`.
- `int main()`: The entry point for every C++ program.
- `cout << "Hello, World!" << endl;`: Outputs "Hello, World!" to the console, followed by a newline.
- `return 0;`: Indicates that the program finished successfully.
Compiling from the Command Line
Using GCC
To compile CPP code with GCC, follow these steps:
- Open your terminal or command prompt.
- Navigate to the directory where your C++ source file is located.
- Execute the compile command:
Here, `-o hello_world` specifies the name of the output file, and `hello.cpp` is your source file name.g++ -o hello_world hello.cpp
Upon successful compilation, an executable named `hello_world` will be created. You can run it with:
./hello_world
Using Clang
Compiling with Clang follows similar steps. Use the command:
clang++ -o hello_world hello.cpp
Both commands translate the source code into executables that you can run in the terminal.
Linking Multiple Source Files
When working on larger projects, it's common to separate code into multiple files for better organization. For instance, if you have the following structure:
- `main.cpp`
- `functions.h`
- `functions.cpp`
You can link them together with GCC like so:
g++ -o program main.cpp functions.cpp
This command compiles all specified source files and creates an executable called `program`.
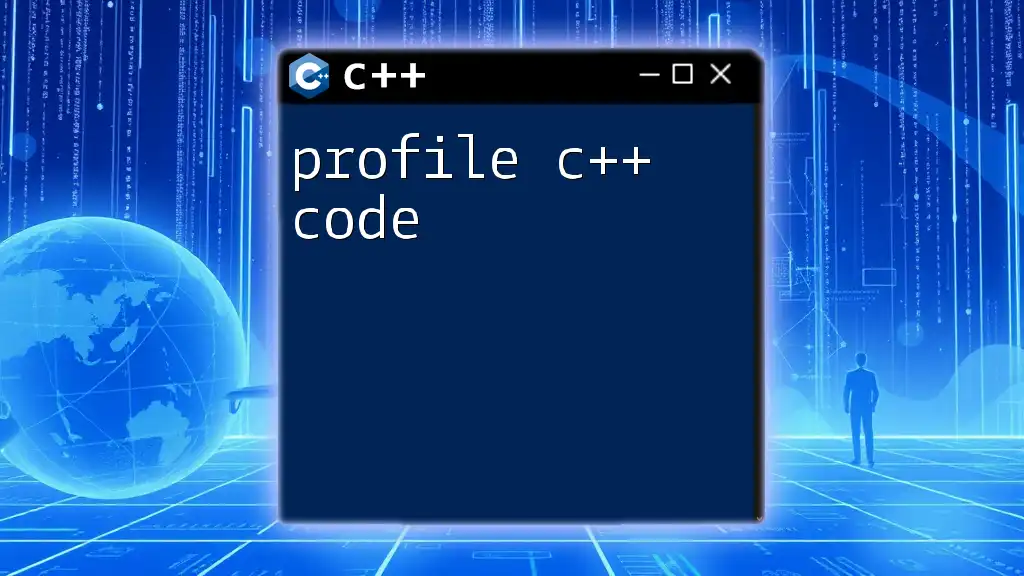
Troubleshooting Compilation Errors
Common Compilation Errors
When you compile CPP code, you may encounter a range of compilation errors. Here are a few common ones:
- Syntax Errors: These occur when code is not written according to C++ syntax rules—missing semicolons, brackets, or incorrect keywords.
- Undefined References: This error arises when the program calls a function that hasn’t been defined or linked properly.
Debugging Compilation Issues
To effectively debug errors, consider:
- Reading the error messages carefully—they often indicate the file and line number where the problem lies.
- Using a debugger (like GDB) to step through the code and understand where things go wrong.
- Regularly isolating code changes to identify when an error is introduced.
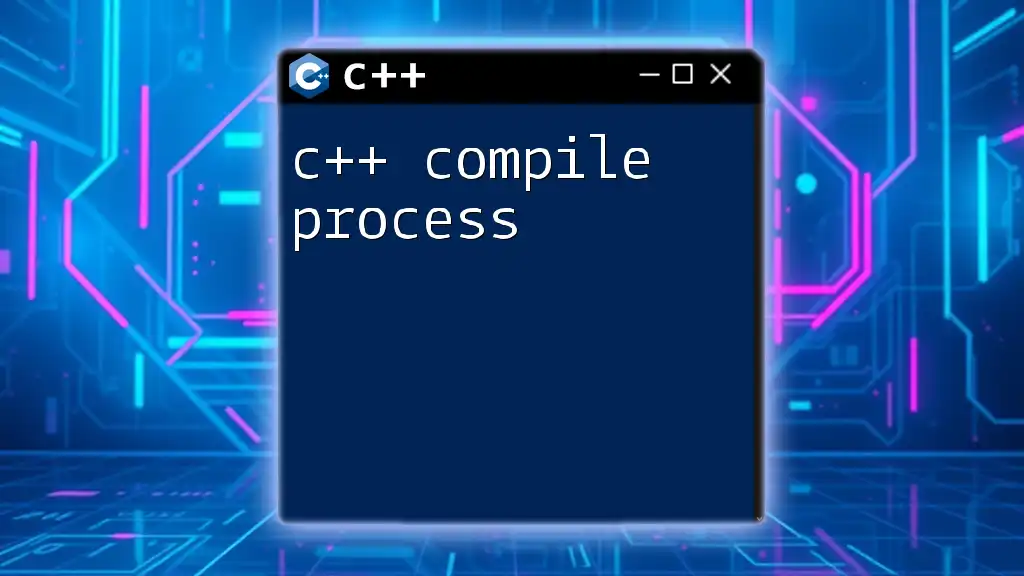
Advanced Compilation Techniques
Using Makefiles
As your C++ projects grow, managing multiple source files manually can become cumbersome. A Makefile can streamline the build process. Here’s a basic structure for a Makefile:
all: program
program: main.o functions.o
g++ -o program main.o functions.o
main.o: main.cpp
g++ -c main.cpp
functions.o: functions.cpp
g++ -c functions.cpp
clean:
rm *.o program
The commands `-c` will compile source files into object files, and the `clean` command will help remove them when needed.
Optimizing C++ Compilation
To enhance performance, consider adding optimization flags during the compilation process. Using flags like `-O2` or `-O3` will enable different optimization levels:
g++ -O2 -o optimized_program main.cpp
This can significantly improve the runtime efficiency of your program, especially in computationally intensive applications.
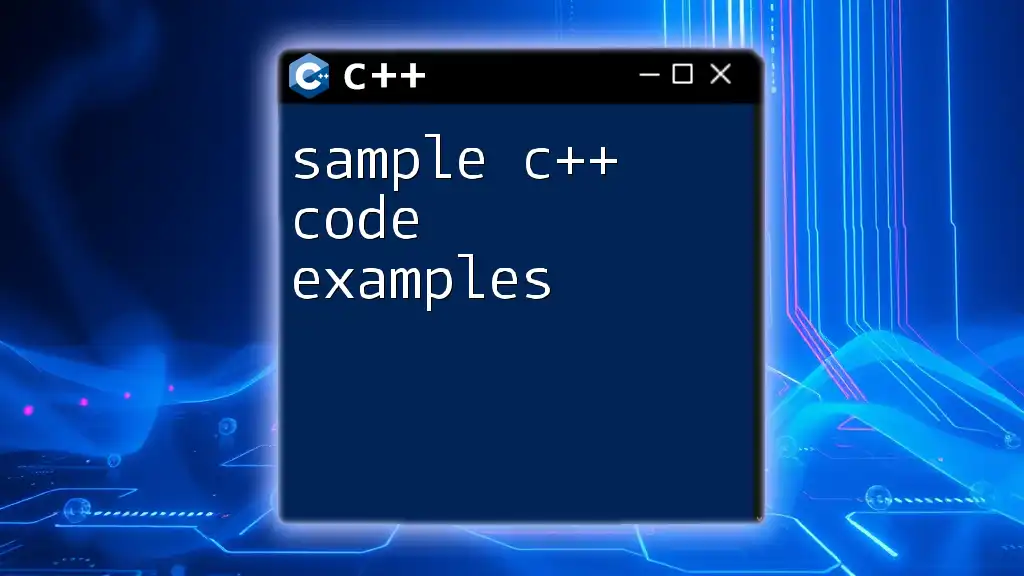
Best Practices in Compiling C++ Code
Organizing Your Codebase
Maintaining a well-structured codebase is crucial for simplifying the compilation process.
- Use clear, descriptive names for your files and directories to convey their purpose.
- Separate header files (`.h`) from implementation files (`.cpp`) to promote modularity.
- Group related functionalities into appropriate files to facilitate easier navigation.
Keeping Compilers Up-to-Date
Regular updates to your compiler can introduce new features, optimizations, and security improvements. To check for updates:
-
GCC: Use your package manager:
sudo apt update && sudo apt upgrade g++
-
Clang: Similar processes apply depending on how it was installed.
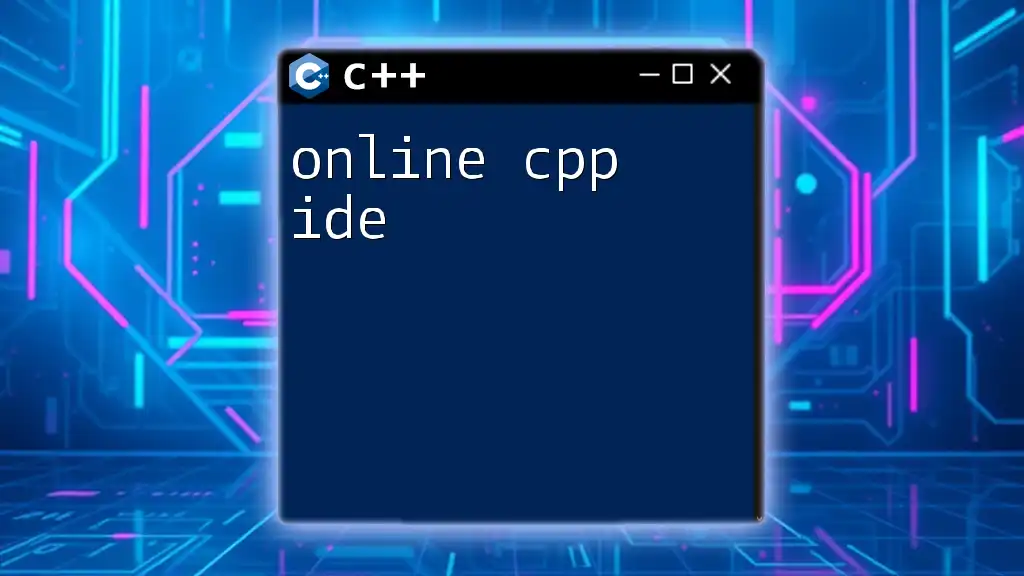
Conclusion
In this guide, we've explored the essential steps to successfully compile CPP code. From understanding the compilation process to utilizing modern tools and practices, you're now equipped with the knowledge necessary for effective C++ development. Practice compiling various projects, experiment with different compilers, and enhance your skills as you become more familiar with the nuances of C++.
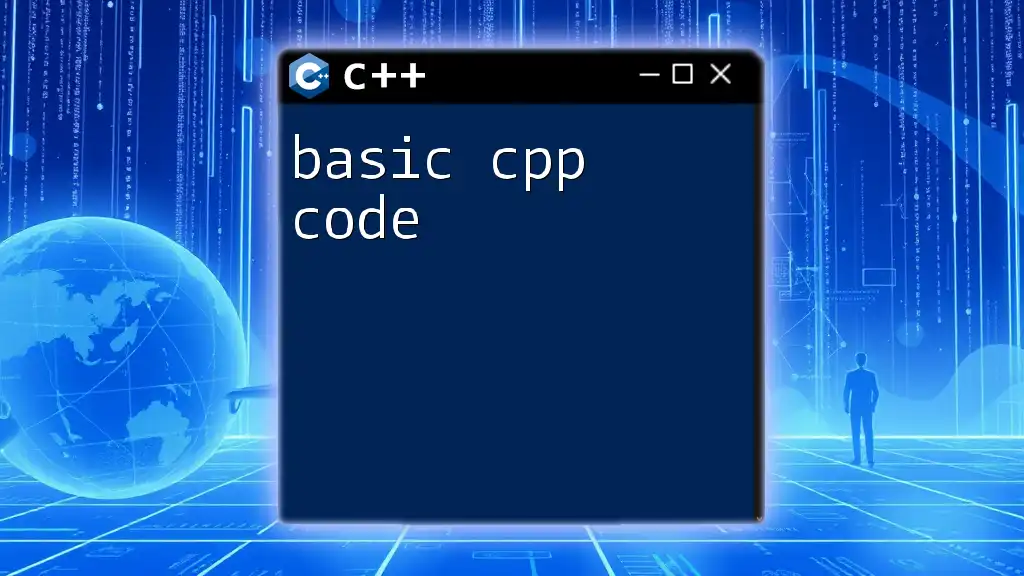
Additional Resources
To deepen your understanding, consider exploring these resources:
- Books: Titles like "Effective C++" and "The C++ Programming Language."
- Websites: C++ official documentation, forums like Stack Overflow, and community teaching platforms like Codecademy.
- Community Support: Engage with forums or local study groups to share knowledge and troubleshoot issues.
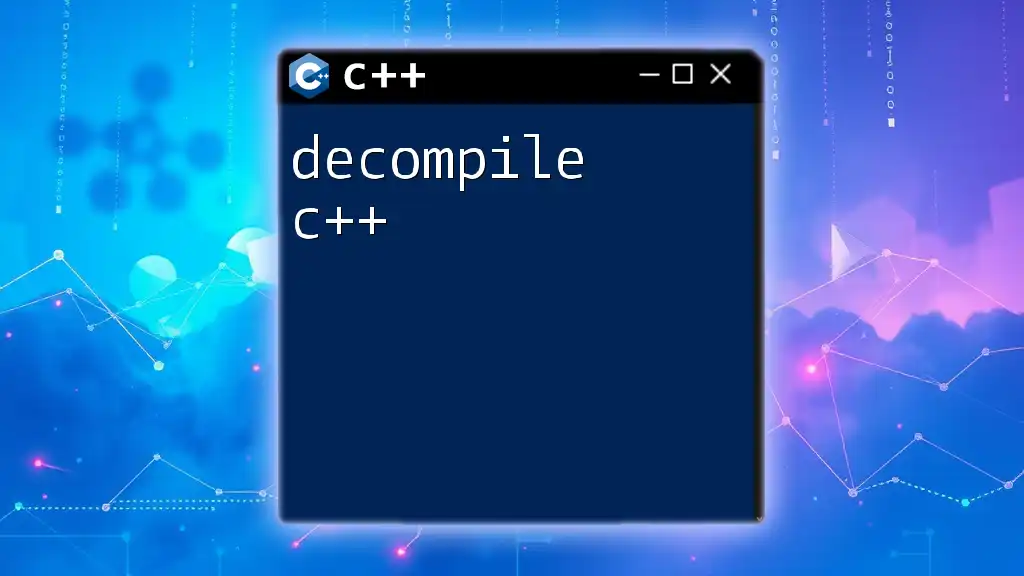
FAQs
What do I do if my program won’t compile?
Double-check syntax, ensure all dependencies are included, and read error messages carefully to identify the issue.
Can I compile C++ code on online platforms?
Yes, there are several online compilers available, such as repl.it and OnlineGDB, which allow you to run simple C++ programs without local setup.
How do I run my compiled C++ program?
After compilation, use the command `./your_program` in the terminal to execute your program's output file.