Basic C++ code consists of fundamental syntax and commands to create simple programs, such as a "Hello, World!" example that demonstrates output to the console. Here’s a simple snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Your C++ Environment
Choosing a Compiler
To get started with basic C++ code, you'll first need to choose a compiler. A compiler converts your C++ code into executable programs. Some popular compilers include:
- GCC (GNU Compiler Collection): Widely used on UNIX-like systems and available for other operating systems.
- Clang: Known for its fast compilation time and better diagnostics.
- MSVC (Microsoft Visual C++): The standard compiler for Windows development, integrated into Visual Studio.
Installation details for each compiler may vary, so be sure to follow the official documentation for setup instructions that suit your operating system.
IDE vs Text Editors
When it comes to writing C++ code, you have the option of using an Integrated Development Environment (IDE) or simpler text editors.
- IDEs, such as Code::Blocks, Visual Studio, and Eclipse, offer powerful features like code suggestion, debugging tools, and project management, making them ideal for larger projects.
- Alternatively, simple text editors like VS Code and Sublime Text can be used for writing basic C++ code snippets efficiently, especially when you're starting out.
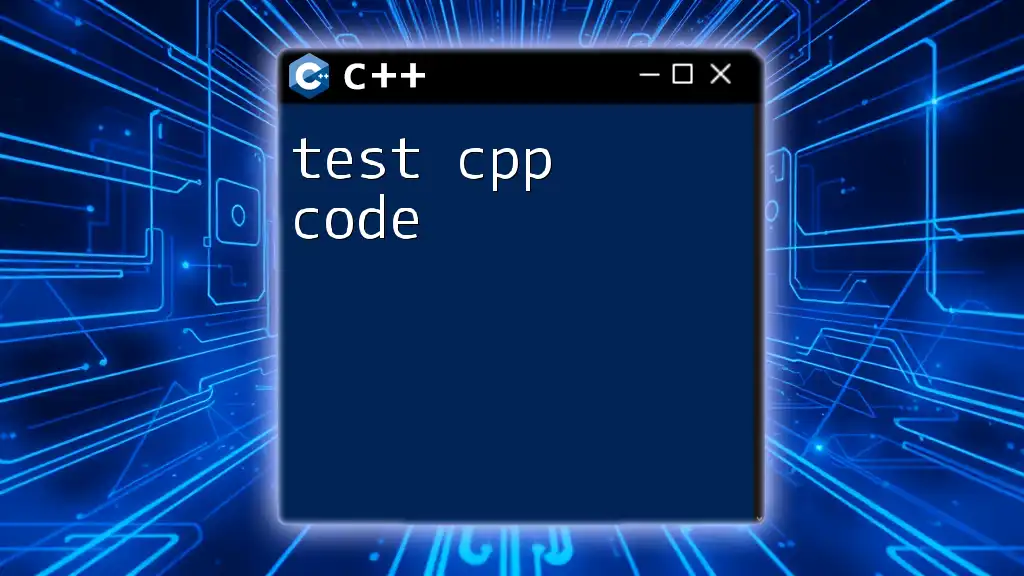
Basic Syntax of C++
Structure of a C++ Program
Every C++ program has a basic structure that consists of several key components, including headers and the main function. Below is a simple example that illustrates this:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `#include <iostream>` allows you to use input and output streams in your program. `int main()` is where the execution of the program begins, and `std::cout` is used to print output to the console.
Comments in C++
Using comments is essential for making your code understandable. C++ supports two types of comments:
- Single-line comments start with `//`, which tells the compiler to ignore the rest of the line.
- Multi-line comments are encapsulated within `/` and `/`, allowing you to comment out larger blocks of text.
Example:
// This is a single-line comment
/*
This is a
multi-line comment
*/
Adding comments helps both yourself and others understand the intent and functionality of your code better.
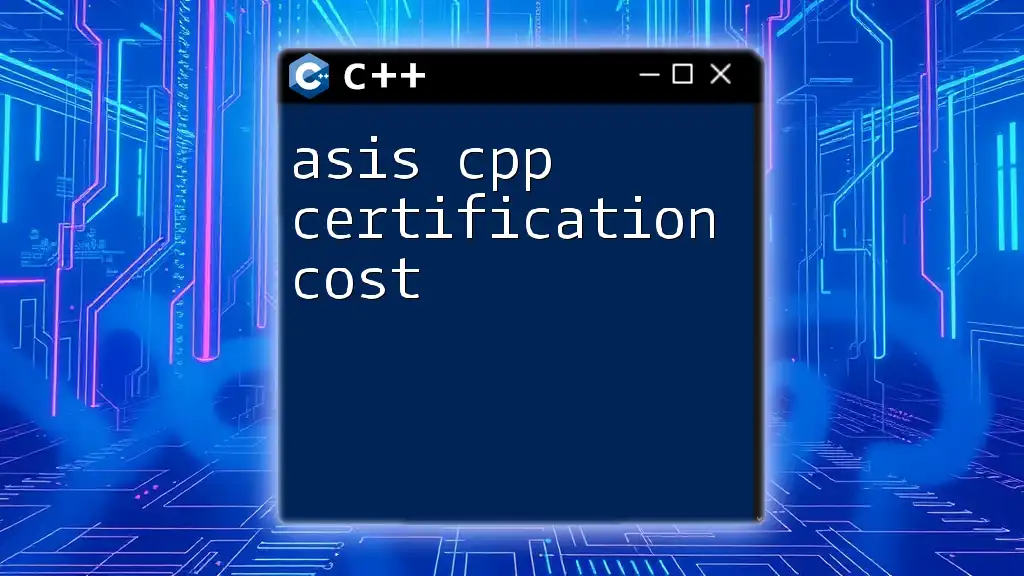
Variables and Data Types
Understanding Variables
A variable in C++ is a named storage that can hold a value. Variables must be declared before they are used in the program. It's important to follow naming conventions like using lower camel case for readability.
Common Data Types
C++ has several fundamental data types. Here are some of the most commonly used:
- int: Stores integer values.
- float: Used for single-precision floating-point numbers.
- char: Represents a single character.
- std::string: A sequence of characters used for text.
- bool: Represents boolean values (true or false).
Example of declaring variables in these types:
int age = 30;
float height = 5.9;
char initial = 'A';
std::string name = "John Doe";
bool isStudent = false;
Understanding data types is crucial in C++ as it impacts how data is stored and manipulated.
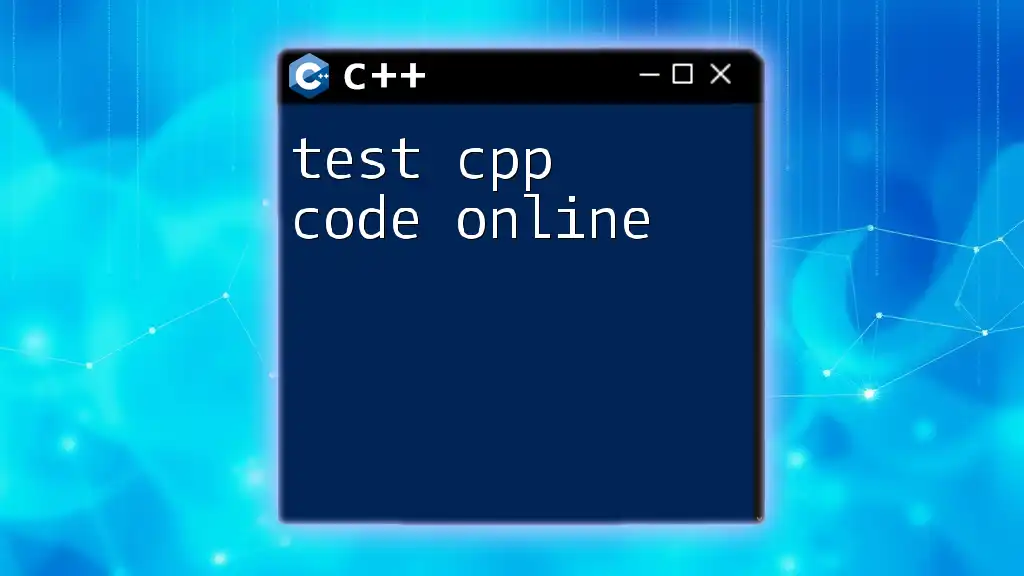
Control Structures
Conditional Statements
Conditional statements allow you to execute code based on certain conditions. The two main types are:
- If Statements: Used to test conditions.
- Switch Statements: Useful for multiple possible outcomes.
Example using an if statement:
if (age > 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Not an Adult" << std::endl;
}
Example with a switch statement:
switch(initial) {
case 'A':
std::cout << "Initial is A" << std::endl;
break;
// More cases...
}
Loops
Loops are a fundamental concept in programming that allow you to execute a block of code multiple times. The two primary types in C++ are:
- For Loop: Great for iterating when the number of iterations is known.
- While Loop: Useful when the number of iterations is unknown.
Example of a for loop:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
Example of a while loop:
int j = 0;
while (j < 5) {
std::cout << j << std::endl;
j++;
}
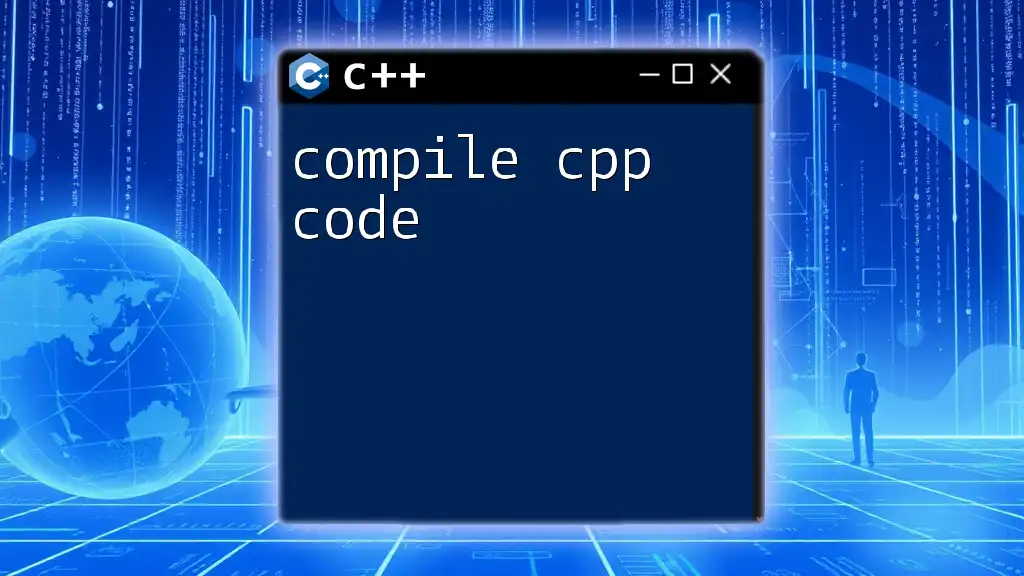
Functions
Defining and Calling Functions
Functions in C++ are blocks of code that perform a specific task and can be called multiple times throughout your program. They help in organizing code and reducing redundancy. Here’s an example of a simple function:
int add(int a, int b) {
return a + b;
}
int main() {
std::cout << add(5, 3) << std::endl; // Outputs: 8
}
Function Overloading
C++ allows you to have multiple functions with the same name as long as they have different parameter types or numbers of parameters. This feature is called function overloading. Here’s a brief example:
float add(float a, float b) {
return a + b;
}
int add(int a, int b) {
return a + b;
}
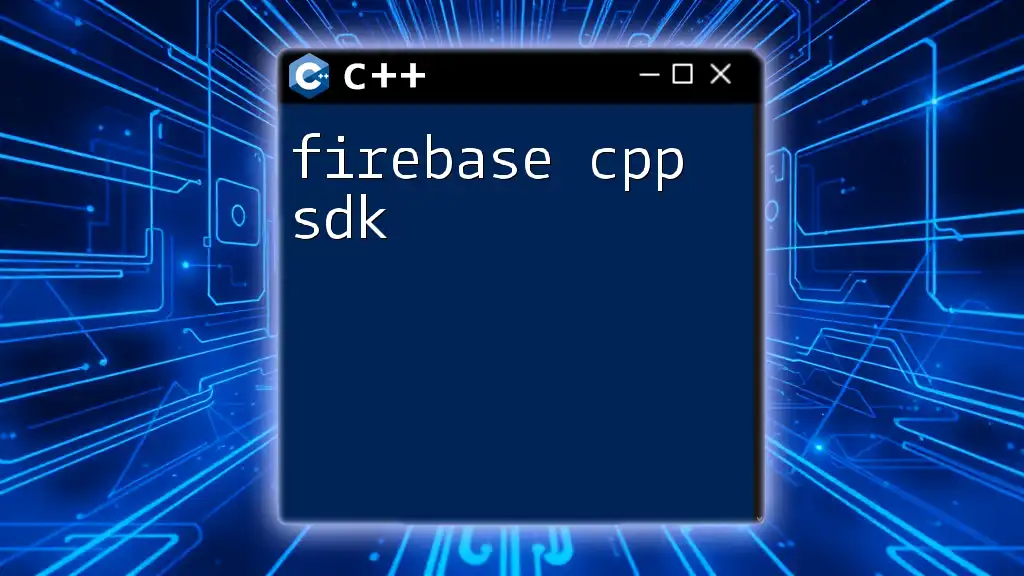
Input and Output
Basic Input and Output
Interacting with users typically requires the ability to accept input and provide output. In C++, you can use `std::cin` for input and `std::cout` for output. Here is a simple input/output example:
int number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "You entered: " << number << std::endl;
This code snippet prompts the user to enter a number, reads the input, and then displays it back to the user.
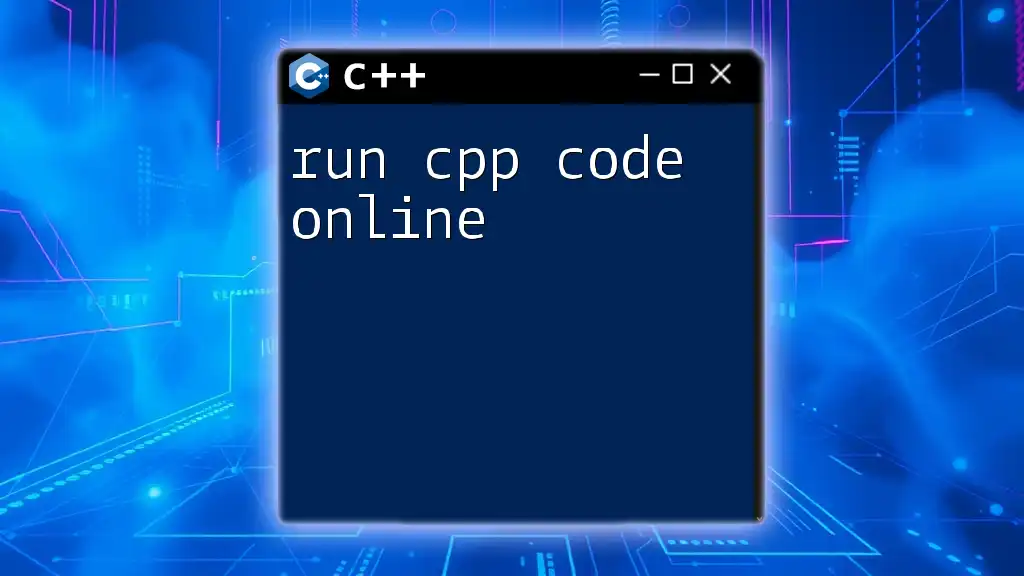
Conclusion
Recap of Key Points
Understanding basic C++ code is essential for anyone looking to start programming in this powerful language. The basics involve setting up your environment, mastering syntax, working with variables, control structures, functions, and handling input and output.
Next Steps in Learning C++
Once you've grasped these fundamentals, consider progressing to more advanced topics such as object-oriented programming, templates, and the Standard Template Library (STL). Engage with online communities, explore tutorials, and practice coding regularly to strengthen your skills in C++.