The basics of C++ involve understanding fundamental concepts such as variables, data types, loops, and functions, which allow you to write efficient and structured code.
Here’s a simple code snippet demonstrating a basic C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful programming language developed by Bjarne Stroustrup in the late 1970s as an extension of the C language. It introduced significant features like object-oriented programming, which allow developers to create complex data structures and programs that are more manageable and reusable. C++ has become a cornerstone in various fields: from system software and game development to applications in finance and telecommunications. Its performance and efficiency are notable, making it a preferred choice for high-performance applications.
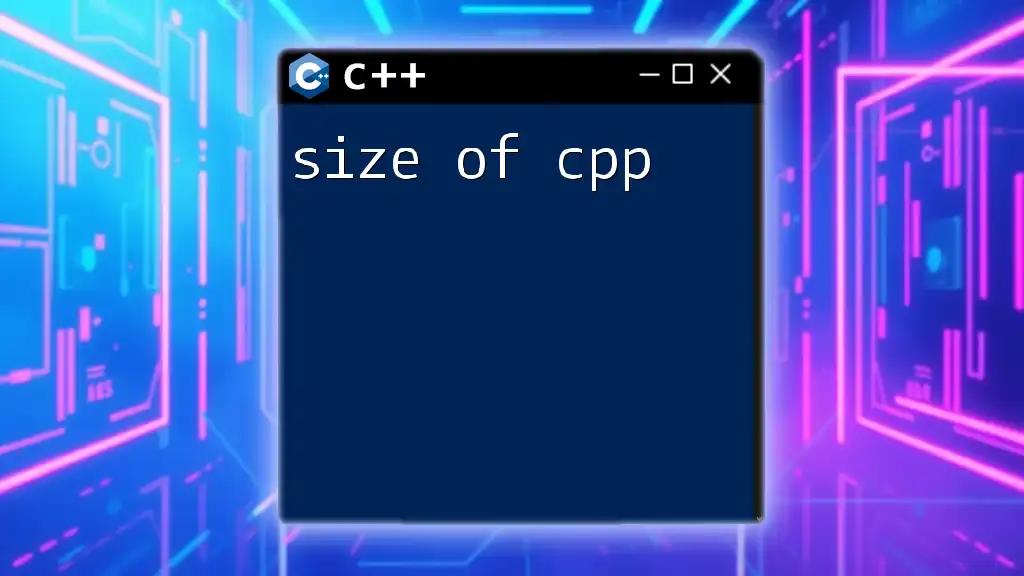
Why Learn C++?
Learning C++ opens numerous doors for developers. Here are some compelling reasons:
- Performance Advantages: C++ offers fine control over system resources, enabling optimization that is crucial in resource-constrained environments.
- Object-Oriented Features: With the ability to encapsulate data and functions, C++ promotes code reusability through inheritance, polymorphism, and encapsulation.
- Versatility and Portability: C++ programs can run on various platforms without significant changes, making it easier for developers to target multiple systems.
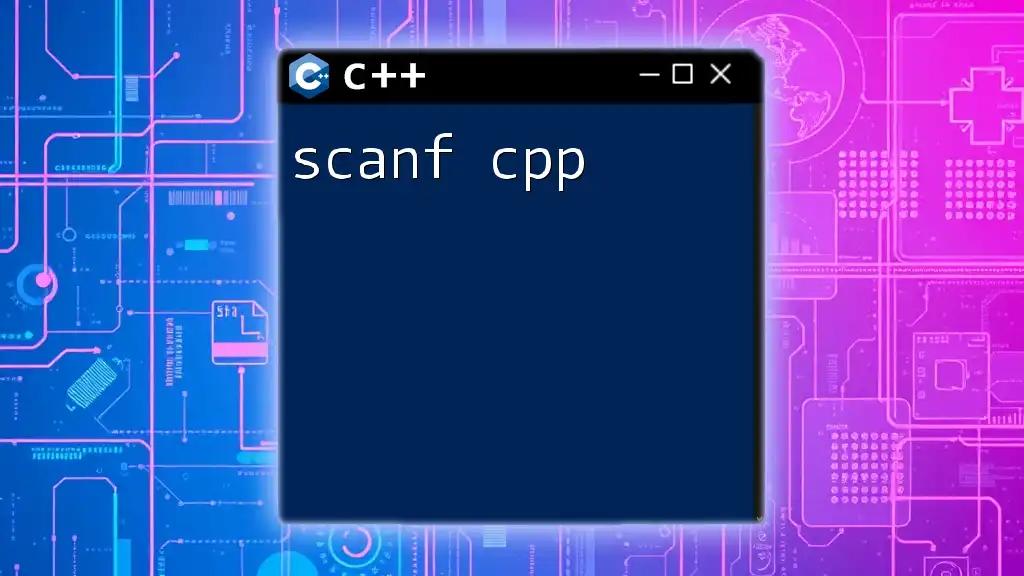
Setting Up the Development Environment
Choosing the Right IDE
An Integrated Development Environment (IDE) is essential for coding efficiently in C++. Popular choices include Visual Studio, Code::Blocks, and CLion. Each IDE offers unique features such as code auto-completion, debugging tools, and project management capabilities. Select one that aligns with your workflow and preferences to enhance your coding experience.
Installing a Compiler
A C++ compiler translates your code into executable programs. Common compilers include GCC and Clang. Follow the installation instructions specific to your operating system, which are available in their respective documentation. A correctly configured compiler is crucial for successful code compilation and execution.
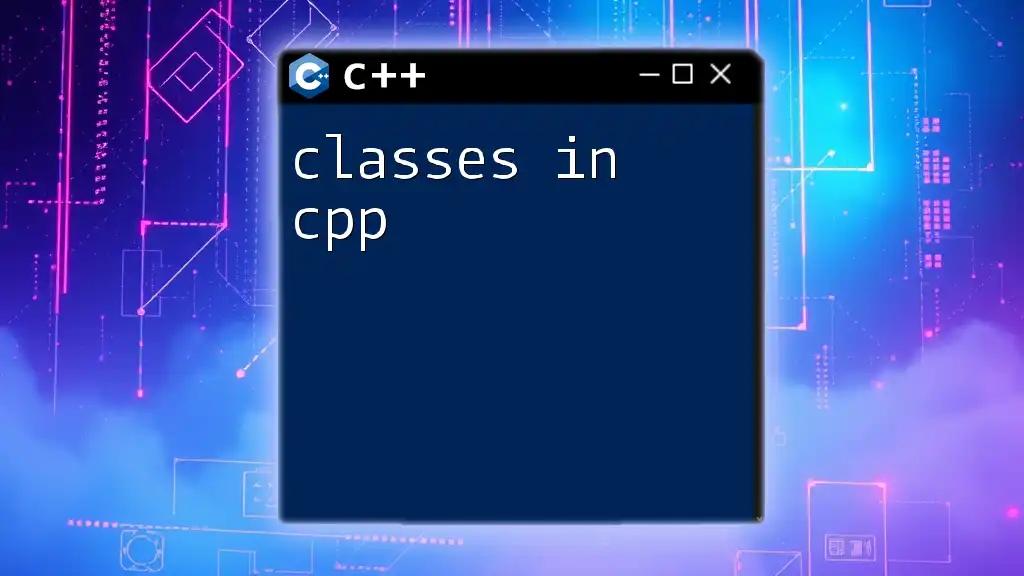
Basic Syntax and Structure
The Structure of a C++ Program
Understanding the basic structure of a C++ program is essential before diving into coding. Every C++ program typically consists of:
- Header Files: These include libraries for functionality (e.g., `#include <iostream>`).
- Main Function: This is where the execution of the program begins, represented as `int main()`.
- Statements: These perform operations such as outputting text or performing calculations.
Here’s an example of a simple C++ program that outputs "Hello, World!":
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Comments in C++
Comments are an important part of programming. They allow programmers to add explanations or notes to the code, enhancing readability. In C++, comments can be single-line (using `//`) or multi-line (using `/* ... */`). Adding comments is a good practice, especially in complex code, as it helps others (and yourself) understand the purpose and logic behind various code sections.
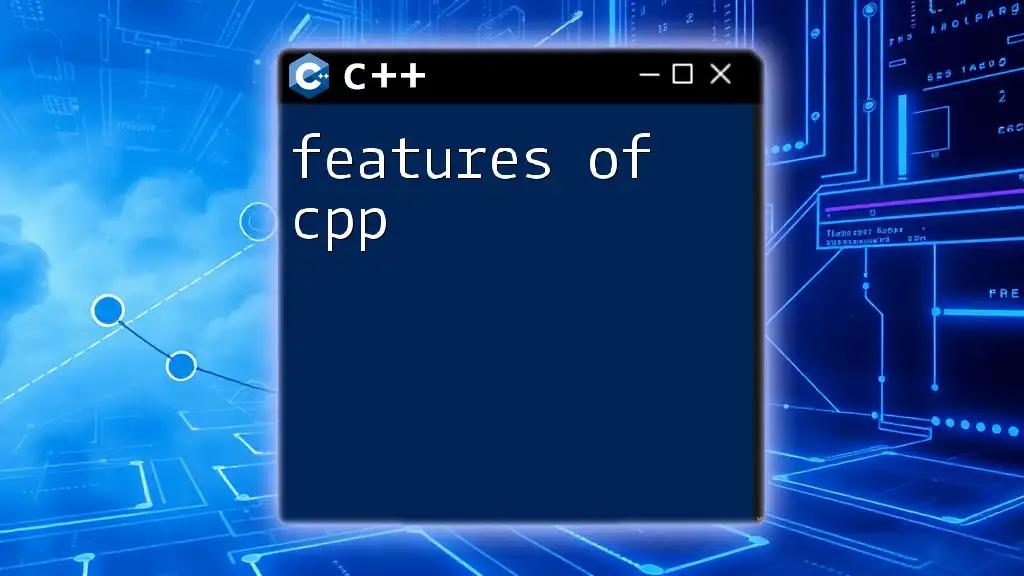
Data Types and Variables
Fundamental Data Types
C++ offers several built-in data types that serve as the primary building blocks for data manipulation. Some of the fundamental data types include:
- int: Represents integer values (e.g., `int age = 30;`).
- float: Represents single-precision floating-point numbers (e.g., `float score = 95.5;`).
- double: Provides double-precision floating-point numbers for more accuracy (e.g., `double cost = 19.99;`).
- char: Represents single characters (e.g., `char initial = 'A';`).
- bool: Represents boolean values, either `true` or `false` (e.g., `bool isValid = true;`).
Declaring and Initializing Variables
Declaring and initializing variables in C++ is straightforward. A variable must be declared before it can be used, specifying its data type followed by its name. Initialization occurs when you assign a value to the variable at the time of declaration. For example:
int age = 30;
double height = 5.9;
char initial = 'A';
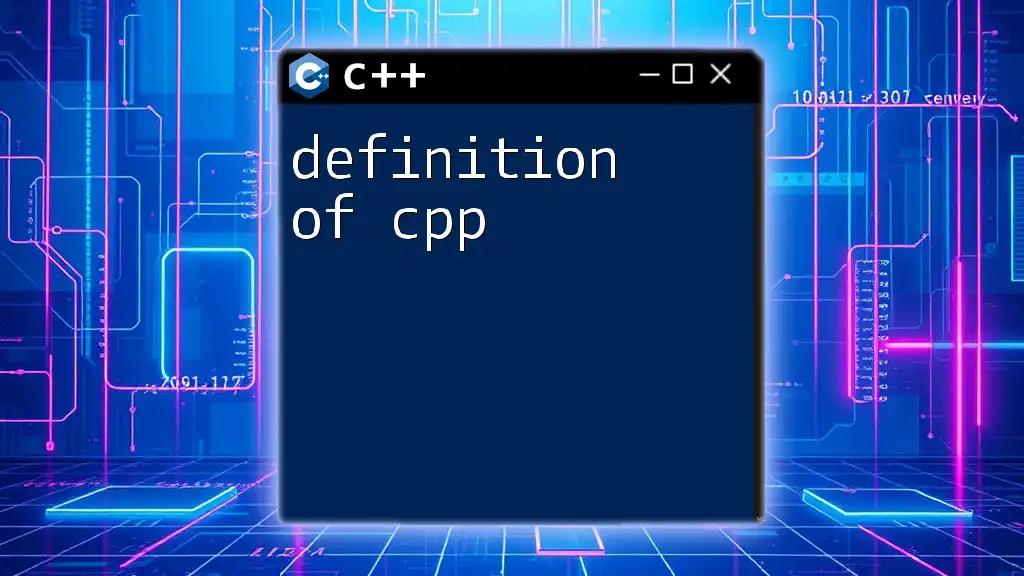
Control Structures
Conditional Statements
Conditional statements allow your program to take different actions based on varying conditions. The most common way to implement this in C++ is through if-else statements. Consider the following example:
if (age >= 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
In this case, the program checks whether the variable `age` is 18 or greater. If true, it outputs "Adult"; otherwise, it prints "Minor".
Loops
Loops are vital for performing repetitive tasks in programming. C++ supports several types of loops, including:
-
For Loop: A common structure for iterating a specific number of times:
for (int i = 0; i < 5; i++) { std::cout << i << std::endl; }
-
While Loop: Executes a block of code as long as a specified condition is true:
int count = 0; while (count < 5) { std::cout << count << std::endl; count++; }
-
Do-While Loop: Similar to the while loop, but it guarantees that the code block will execute at least once:
int number; do { std::cout << "Enter a number: "; std::cin >> number; } while (number < 0);
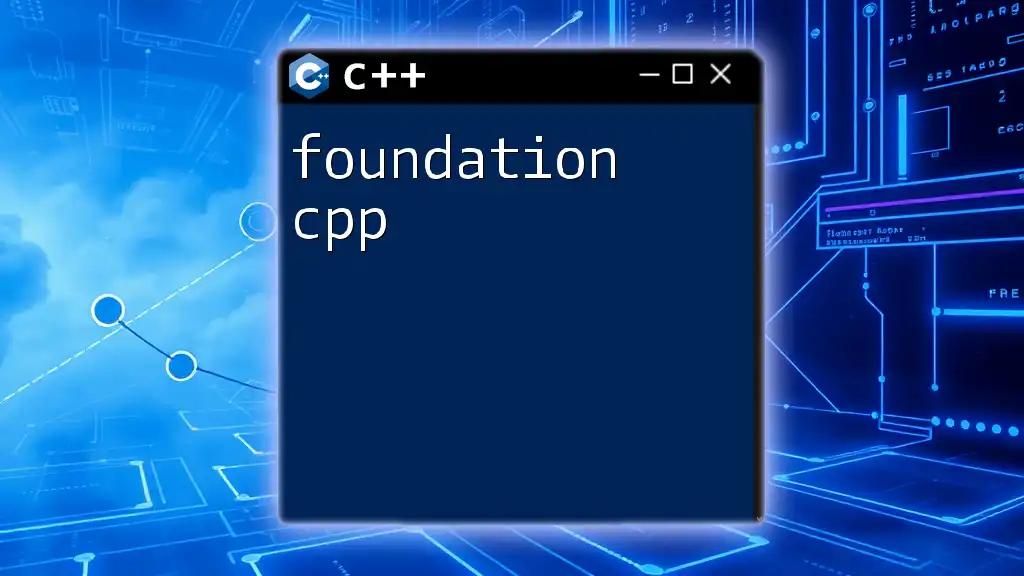
Functions
Declaring and Defining Functions
Functions are reusable blocks of code designed to perform specific tasks. A function must be declared before it can be used in your program. The general syntax for function declaration consists of a return type, function name, and parameters. Here’s how you can declare and define a simple function:
int add(int a, int b) {
return a + b;
}
In this example, the function `add` takes two integers as parameters and returns their sum.
Function Parameters and Return Types
Understanding function parameters and return types is crucial for effectively using functions. Parameters allow you to pass information into functions, while return types specify the type of value a function will return. Here’s an example that demonstrates both concepts:
double multiply(double x, double y) {
return x * y;
}
In this case, the function `multiply` accepts two double values and returns their product.
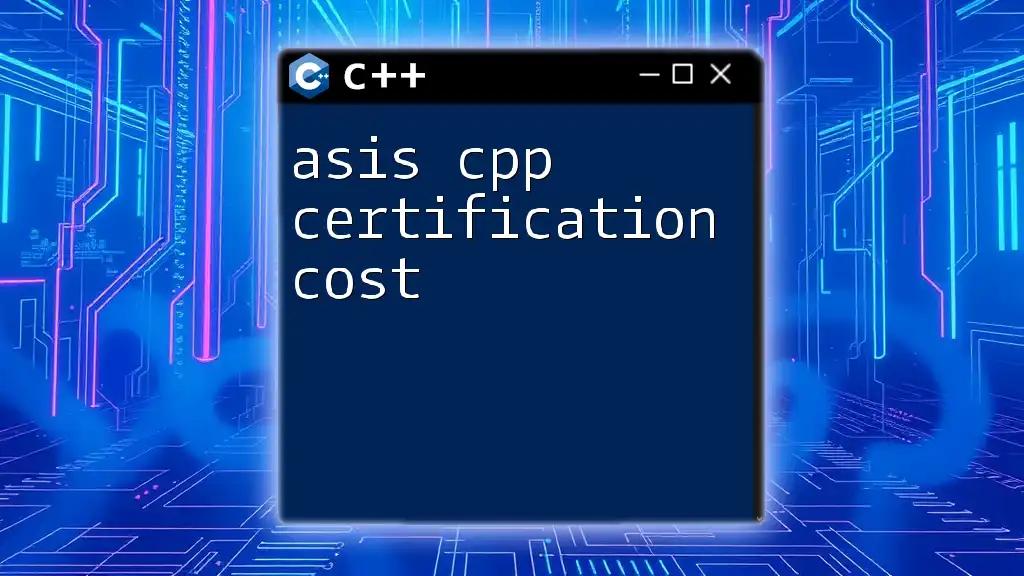
Object-Oriented Programming Concepts
Understanding Classes and Objects
C++ is fundamentally an object-oriented programming language, allowing developers to create classes as blueprints for objects. A class encapsulates data and functions that operate on that data. Here’s a simple example of how to define a class and create an object:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
In this code, we create a class `Dog` with a member function `bark`. We then instantiate the `Dog` object and call its method.
Inheritance and Polymorphism
Inheritance allows a class to inherit properties and behaviors from another class, promoting code reusability. For instance, if we have a base class `Animal`, we can create derived classes like `Dog` and `Cat`.
Polymorphism enables using functions with different implementations through a common interface. It allows a program to decide at runtime which method to invoke based on the object's type.
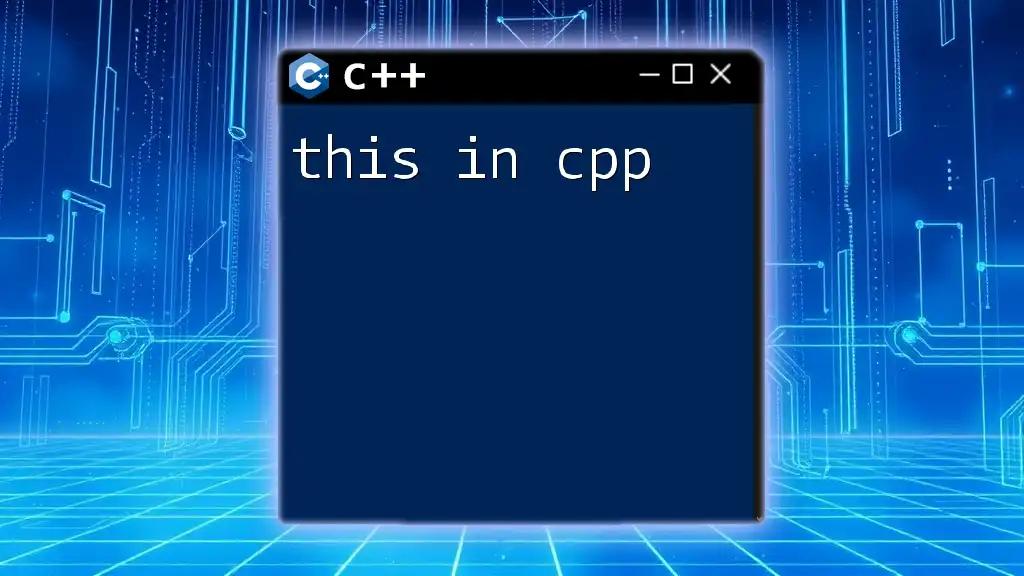
Standard Template Library (STL)
Overview of STL
The Standard Template Library (STL) is a powerful collection of classes and functions in C++. It provides ready-to-use data structures like lists, vectors, and maps, along with algorithms for searching and manipulating these structures. STL promotes code efficiency and reduces the need for custom implementations.
Common STL Containers
Some commonly used STL containers include:
- Vector: A dynamic array that can grow in size as needed. You can easily add, remove, and access elements. Here’s how to use a vector:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4};
for (int number : numbers) {
std::cout << number << std::endl;
}
return 0;
}
- List: A doubly linked list that allows fast insertions and deletions.
- Map: A collection of key-value pairs that provides fast retrieval based on keys.
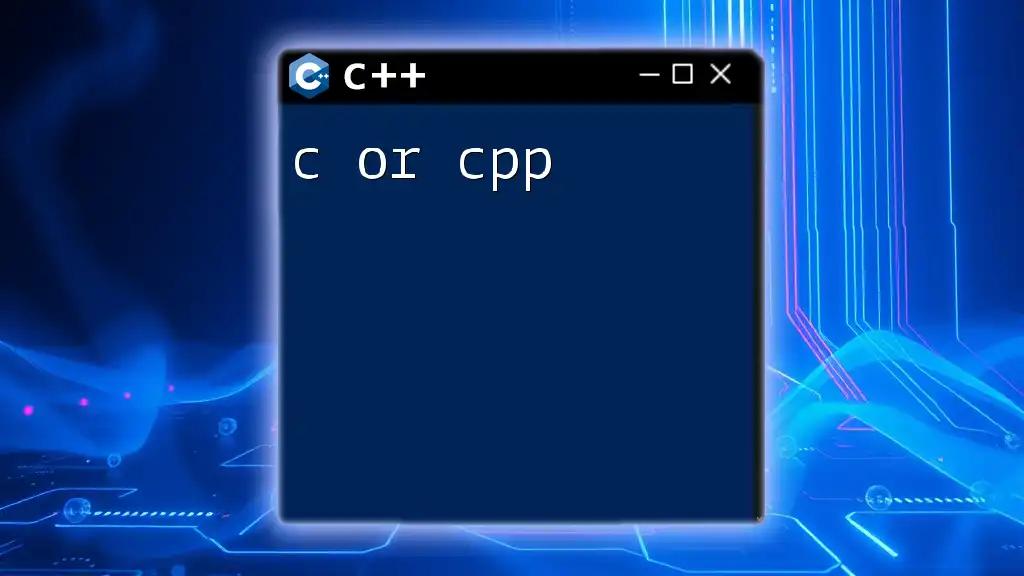
Best Practices in C++ Programming
Writing Readable Code
Writing clear and maintainable code is critical to becoming a successful developer. Naming conventions (e.g., using meaningful variable names, following camelCase or snake_case) make code easier to read. Additionally, proper code formatting (like consistent indentation and spacing) significantly enhances clarity.
Error Handling
Robust error handling is essential for writing reliable applications. C++ provides mechanisms for catching and handling exceptions. Consider the following try-catch block:
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
In this example, when an error is thrown, the program will catch it and display an appropriate message, preventing a complete halt of execution.
Code Optimization Techniques
Optimizing code improves performance and efficiency. Techniques include minimizing resource usage, using appropriate data structures, and adopting algorithms with lower computational complexity. Profiling tools can help identify bottlenecks in the code.
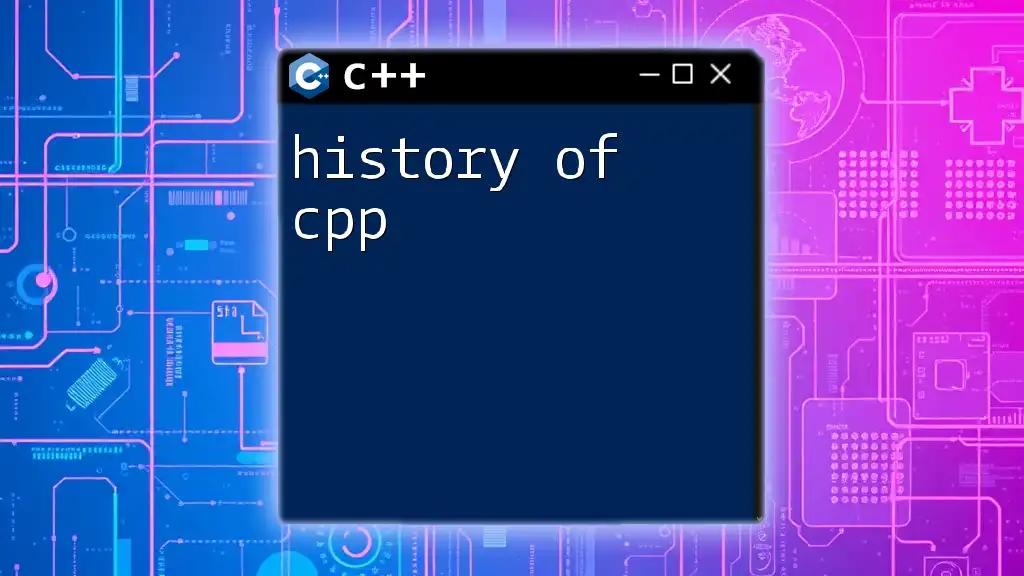
Conclusion
In summary, this guide has covered the basics of C++. From understanding data types and control structures to delving into functions and object-oriented programming, you are now equipped with foundational knowledge that is essential for further exploration of C++.
As you embark on your journey with C++, practical application is crucial—practice writing code, experiment with examples, and explore more advanced concepts. The programming community is vast, and numerous resources are available to deepen your understanding. Happy coding!