C and C++ are both powerful programming languages, with C being a procedural language focused on low-level system programming, while C++ extends C by adding object-oriented features and abstractions for building complex applications.
Here's a simple code snippet in C++ that demonstrates a basic class definition:
#include <iostream>
class Greetings {
public:
void sayHello() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
Greetings greet;
greet.sayHello();
return 0;
}
What is C?
History of C
C was developed in the early 1970s by Dennis Ritchie at Bell Labs. It was originally designed to implement the Unix operating system and has since become one of the most widely used programming languages. Throughout its evolution, C has inspired numerous derivative languages and has played a significant role in software development.
Key Features of C
Low-Level Access: C gives programmers direct access to memory through pointers. This capability is crucial for system-level programming, offering the ability to manipulate memory and hardware directly, which can lead to high performance but requires careful management.
Portability: One of the main strengths of C is its portability; programs written in C can be compiled and run on different types of computer systems with minimal modification. This is largely due to the standardized syntax defined in the ANSI C specification.
Efficiency: C is known for its efficiency in execution and runtime performance. Its straightforward syntax and low-level capabilities make it ideal for applications where resource constraints are a key consideration, such as in embedded systems or operating systems.
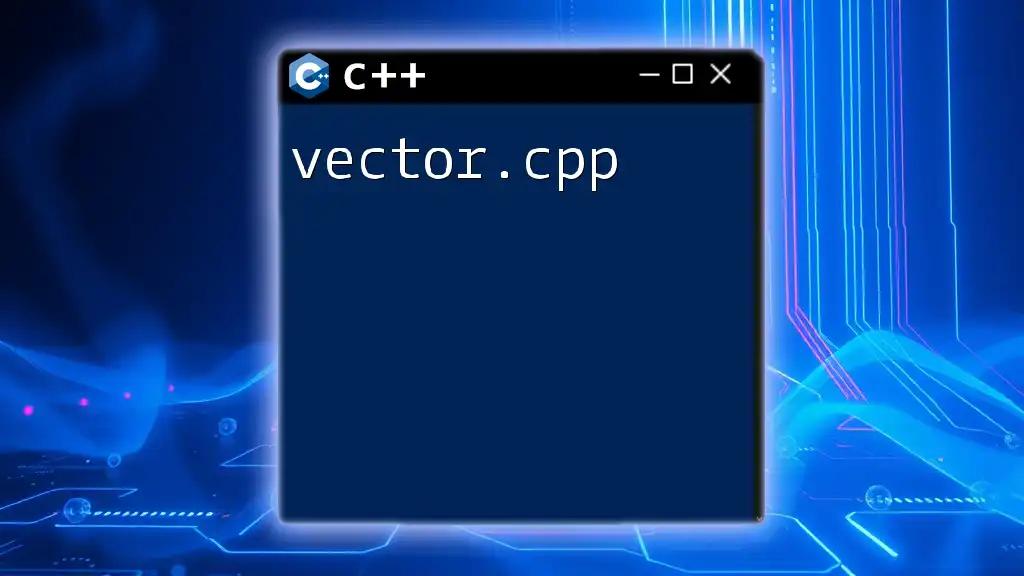
What is C++?
History of C++
C++ was created by Bjarne Stroustrup in the late 1970s as an extension of the C programming language. It introduced features like classes and object-oriented programming to enhance the language's capabilities, making it suitable for larger, more complex software projects.
Key Features of C++
Object-Oriented Programming (OOP): C++ supports OOP principles such as encapsulation, inheritance, and polymorphism. This allows developers to create modular and reusable code, which is critical for maintaining larger codebases. For example, classes in C++ can encapsulate data and functions, allowing for better organization and maintainability.
Generic Programming: With the introduction of templates, C++ allows developers to write generic and reusable code. This feature promotes type safety without compromising performance, making it easier to work with different data types using the same code framework.
Standard Template Library (STL): One of C++'s most powerful features is the STL, which provides a collection of template classes and functions for data structures and algorithms. This library enables developers to write efficient and performance-optimized code with ease.
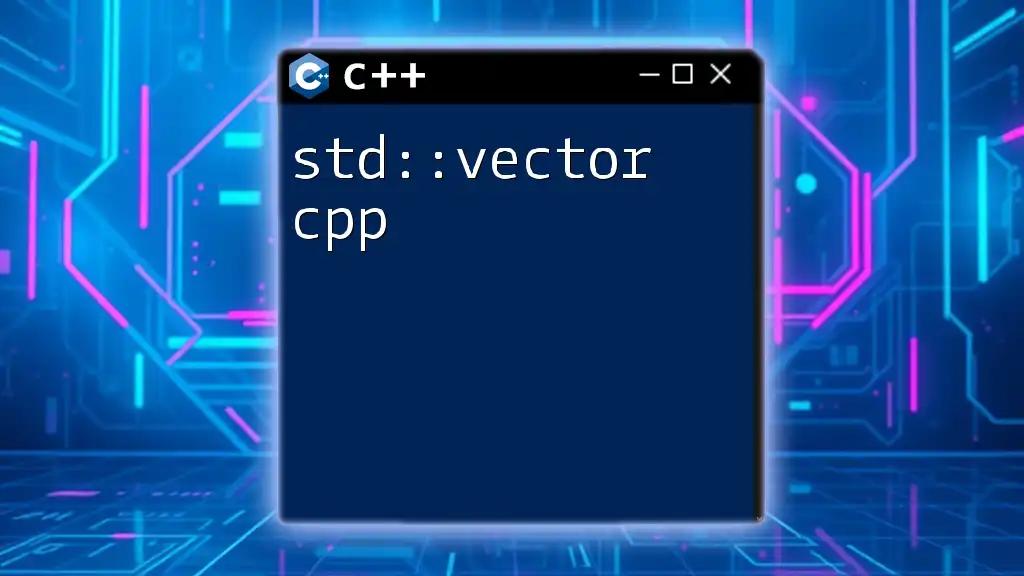
C vs C++: A Side-by-Side Comparison
Syntax Differences
Function Declarations: While both C and C++ share a similar syntax, there are subtle differences. In C++, you might declare a function using the keyword `inline` for inline functions, a concept not present in C. Understanding these nuances can be vital when migrating from one language to the other.
Memory Management: In C, memory management is often manually handled using `malloc` and `free`, providing full control but also introducing the risk of memory leaks. In contrast, C++ introduces the concept of constructors and destructors, allowing for better resource management and automatic cleanup through RAII (Resource Acquisition Is Initialization).
Programming Paradigms
Procedural Programming in C: C is typically described as a procedural programming language. It follows a linear approach, focusing on functions and procedures to operate on data.
Object-Oriented Programming in C++: In contrast, C++ is a multi-paradigm language that supports both procedural and object-oriented programming. This versatility allows developers to choose the best approach for their specific problem domain.
Performance Considerations
When it comes to performance, both languages have their strengths. C is often preferred for applications requiring maximum speed and minimal overhead, such as operating systems and embedded systems. On the other hand, C++ provides optimizations that can enhance performance through advanced features like operator overloading and inline functions, making it suitable for complex applications like video games and graphics engines.
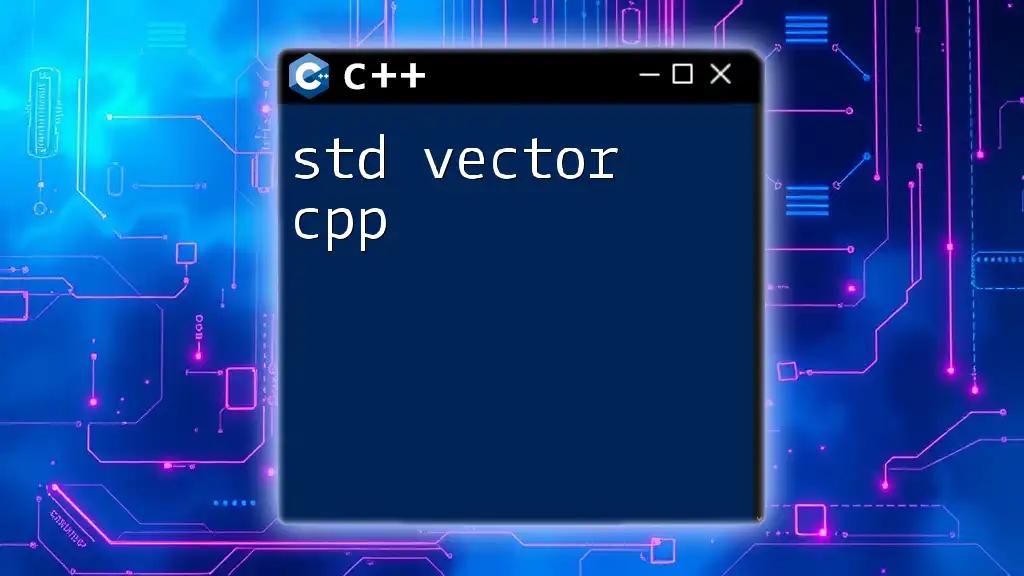
Use Cases for C
System Programming
C excels in system programming tasks, such as crafting operating systems or developing software that interacts directly with hardware. Its low-level access to memory allows for fine-tuned optimization and control over system resources.
Game Development
Although C++ is more commonly associated with game development, C finds its place in various game engines that prioritize performance. For instance, several game consoles use C due to its efficiency and low overhead.
Legacy Systems
Many legacy systems were built using C, necessitating ongoing maintenance and support. Understanding C is crucial for developers who need to update or integrate with these systems efficiently.
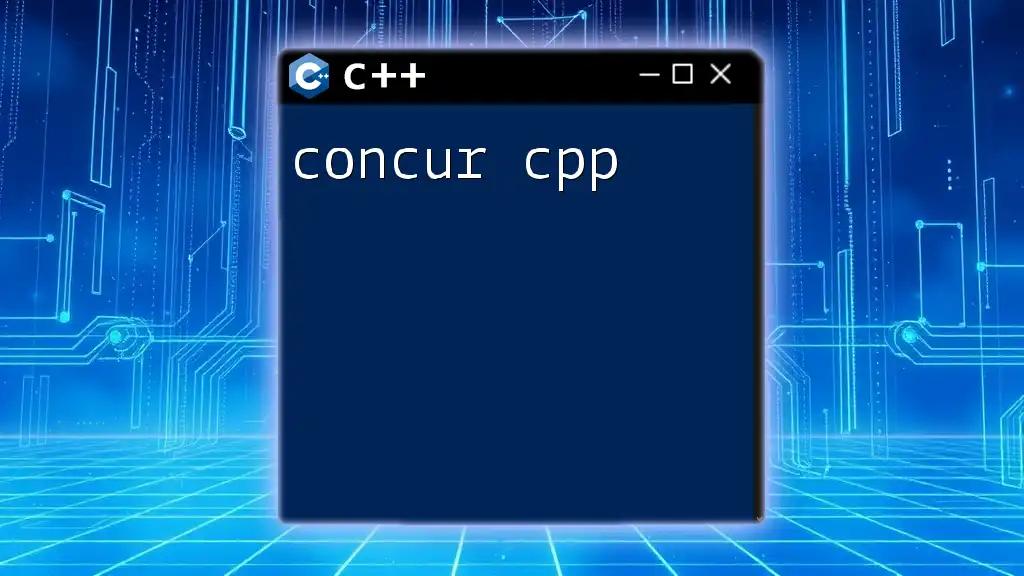
Use Cases for C++
Application Development
C++ shines in application development, particularly for desktop applications where OOP can significantly ease the design and maintenance process. GUI frameworks like Qt leverage C++ features for building user-friendly interfaces.
Game Development
C++ plays a dominant role in modern game development, particularly with engines such as Unreal Engine. Its OOP features streamline code organization, making it easier to develop and manage complex game systems.
High-Performance Applications
Applications that require high computational power—such as those used in finance, simulations, or scientific computing—benefit substantially from C++'s performance-oriented features, allowing developers to build efficient solutions that handle significant workloads.
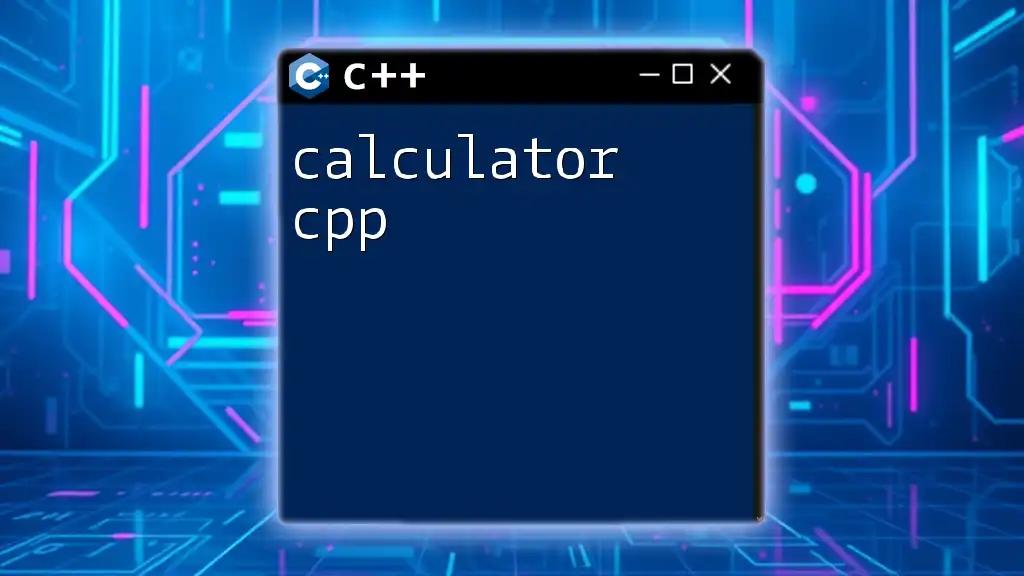
How to Choose Between C and C++
Project Requirements
The primary consideration should always be the project requirements. If the project demands low-level hardware manipulation or system programming, C is typically the preferred choice.
Team Expertise
Assess the skills and expertise of your development team. If the majority are well-versed in C++, utilizing its advanced features may lead to better productivity and code quality. Conversely, a team skilled in C might produce faster results when writing system-level code.
Performance Needs
Consider the performance requirements of your application. If absolute speed and minimal overhead are critical, C may be the better choice. However, if the project benefits from OOP features while still needing performance, C++ is likely the ideal candidate.
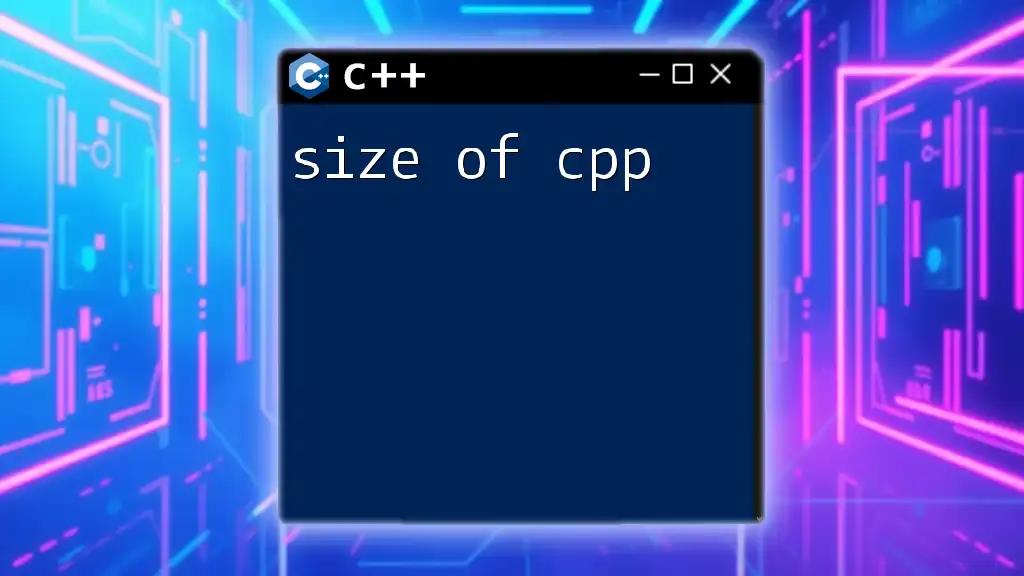
Getting Started with C and C++
Tools and IDEs
Some recommended Integrated Development Environments (IDEs) for C and C++ development include Code::Blocks, Visual Studio, and Eclipse. These tools offer features like code completion, debugging, and project management, enhancing the development experience.
First Steps in Coding
Initiating your programming journey involves writing a simple "Hello, World!" program. Here’s how you do it in both C and C++:
// C
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
// C++
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Both snippets achieve the same goal but demonstrate the syntactical differences between the languages. Understanding these differences is essential for mastering either C or C++.

Conclusion
In summary, the choice between C or C++ should be guided by the specific needs of your project and the expertise of your team. C offers unparalleled performance and access for system-level programming, while C++ excels in application development and complex systems thanks to its object-oriented features. As you continue to explore and develop your programming skills, consider diving into both languages, leveraging their distinct strengths to elevate your development toolkit.

Additional Resources
Books
To deepen your understanding of C and C++, consider reading classic texts like "The C Programming Language" by Brian Kernighan and Dennis Ritchie, and "The C++ Programming Language" by Bjarne Stroustrup.
Online Courses
Platforms such as Coursera, Udacity, and edX provide structured online courses tailored for both C and C++ learners, ranging from beginner to advanced levels.
Community and Forums
Engagement with programming communities such as Stack Overflow, Reddit, or dedicated forums can enhance your learning experience. Here, you can ask questions, share knowledge, and connect with fellow developers who share your interests.
Engage with these resources to sharpen your skills and knowledge in C or C++, and take the first steps toward becoming a proficient programmer.