C++ (pronounced "C plus plus") is a powerful, high-level programming language developed in the early 1980s by Bjarne Stroustrup as an extension of the C programming language, incorporating object-oriented features and supporting system and application software development.
Here's a simple code snippet demonstrating a basic C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Origins of C++
Emergence of the C Language
The journey of C++ begins with C, a programming language created by Dennis Ritchie in the early 1970s. C was designed to write system software for UNIX, showcasing both the power and simplicity necessary for effective programming at the time. Its structured programming paradigm and low-level capabilities made it immensely popular among developers.
Example: Here is a simple C program demonstrating the fundamental structure:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
While C provided solid foundations, it had its limitations, particularly in managing larger codebases that required abstraction and organization. This need for a higher level of abstraction gave rise to the subsequent evolution that is C++.
The Need for Abstraction
As software systems grew increasingly complex, developers encountered challenges when using C due to its procedural nature. The lack of support for Object-Oriented Programming (OOP) made it difficult to manage complexities inherent in large applications. Bjarne Stroustrup recognized this need while working at Bell Labs, leading to the creation of C++ in 1979.
C++ was designed to allow programmers to define data structures abstractly, incorporating features of C while adding OOP concepts like classes and inheritance. This offered a powerful way to represent real-world entities in code, making it a more natural fit for many types of software development.
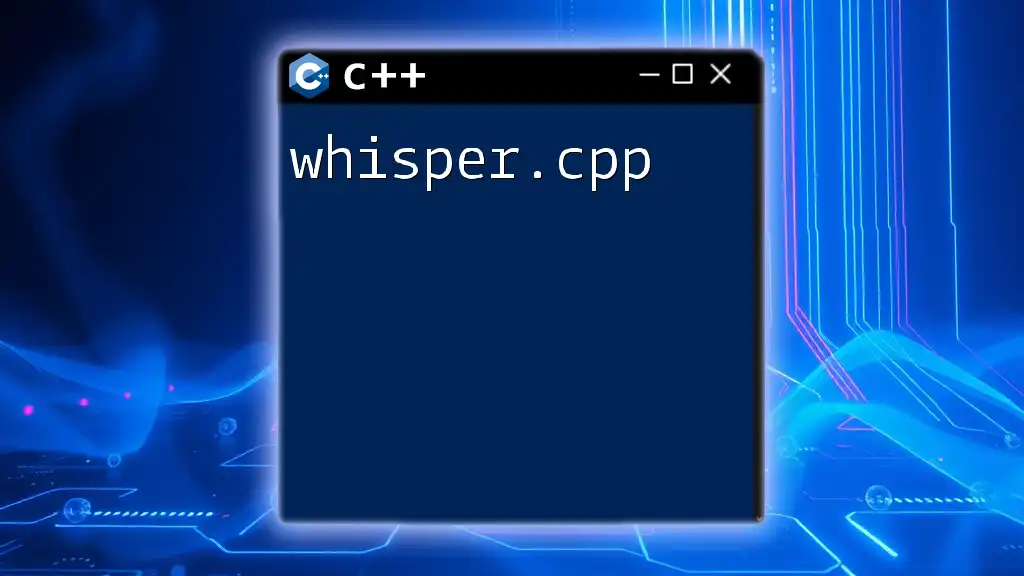
Key Milestones in the History of C++
The First Version: C with Classes
The first version of C++ was known as C with Classes, which introduced the concept of classes to the language. This marked a significant shift, allowing developers to group data and functions that operate on that data together, encapsulating them in objects.
Example: Here’s a simple implementation of a class in C++:
class Dog {
public:
void bark() {
printf("Woof!\n");
}
};
int main() {
Dog myDog;
myDog.bark(); // Outputs: Woof!
return 0;
}
This encapsulation promotes better organization and modularity in software designs, making code more maintainable and flexible.
C++ 2.0 and the Expansion of Features
By 1989, C++ evolved into C++ 2.0, which introduced several enhanced features. Notable amongst these was the support for multiple inheritance, allowing a class to inherit from more than one base class. This was a significant advancement in allowing developers to create more complex relationships between classes.
Additionally, C++ 2.0 included features like function overloading, which allowed the same function name to be used for different purposes, depending on the parameter types. This streamlined coding and made it easier to read and maintain.
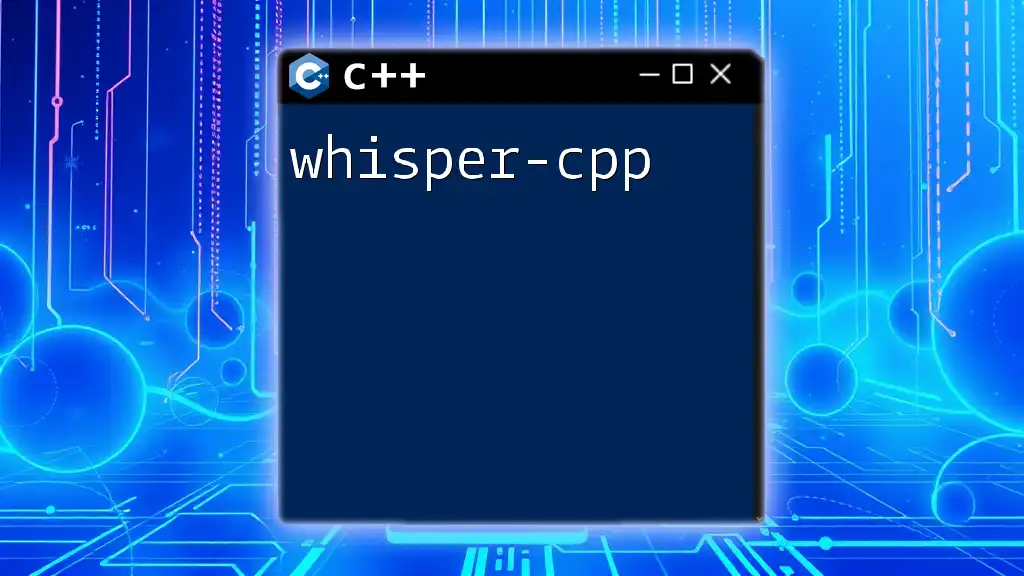
Standardization of C++
The C++ Standardization Committee
As C++ gained popularity, the need for a standardized version became apparent. In the early 1990s, the ISO C++ Committee was formed with the goal of defining a consistent and robust standard for the language. Their efforts culminated in the release of C++98 in 1998, a milestone that formalized the language and its features.
The First Standard: C++98
C++98 included the much-anticipated Standard Template Library (STL), which provided a set of common data structures and algorithms. The STL's introduction simplified many programming tasks by supplying pre-built components for handling commonly encountered problems.
Example: Here’s a simple demonstration of using the STL's vector:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
std::cout << number << " ";
}
return 0;
}
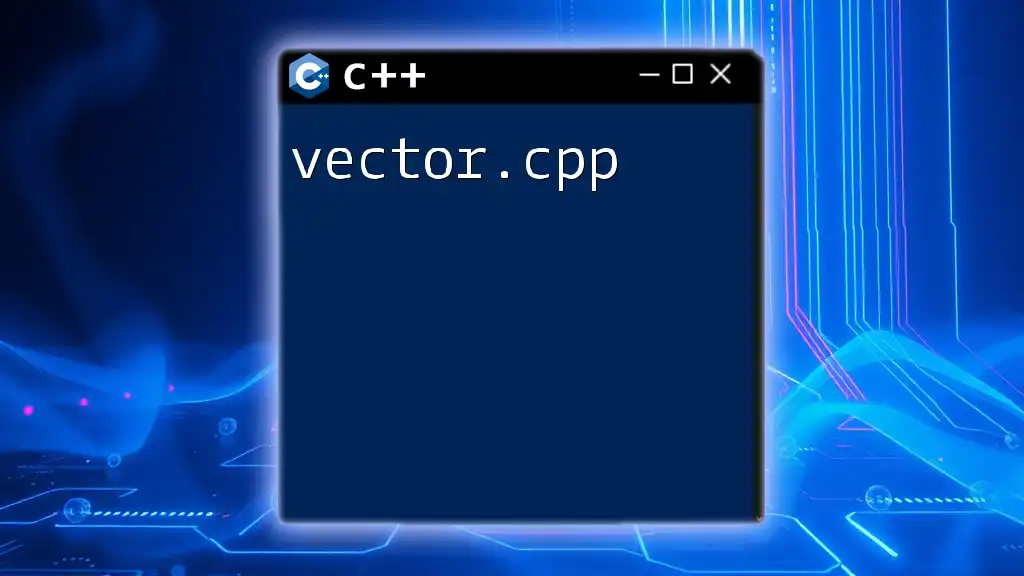
Evolution of C++: Major Updates and Their Impacts
C++03: Corrections and Improvements
Following C++98, the community recognized the need for minor corrections and clarifications, which led to the release of C++03. While it didn’t introduce major new features, it consolidated and polished existing functionalities, ensuring consistency across implementations.
C++11: A Significant Leap Forward
In 2011, C++ underwent a revolutionary update with the release of C++11. This version added numerous features that significantly enhanced the language:
- Lambda Expressions: These allow function objects to be defined inline, improving expressiveness and readability.
- Auto Keyword: This keyword enables automatic type deduction of variables, simplifying code.
Example: Here’s a basic use of lambda expressions in C++11:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int n) { std::cout << n << " "; });
return 0;
}
The introduction of smart pointers in C++11 also revolutionized memory management, allowing better control and automatic resource deallocation.
C++14 and 17: Fine Tuning the Language
The evolution continued with C++14, which introduced several enhancements like generic lambdas, and further improved with C++17, notable for features such as std::optional.
Example: An illustration of std::optional:
#include <iostream>
#include <optional>
std::optional<int> findValue(bool found) {
if (found) {
return 42;
}
return std::nullopt; // Represents no value
}
int main() {
auto result = findValue(true);
if (result) {
std::cout << *result << std::endl; // Outputs: 42
}
return 0;
}
These updates marked a significant shift towards modern programming practices, making C++ more seamless and enjoyable to work with.
C++20 and Beyond: The Future of C++
Recently, C++20 was released, bringing groundbreaking features such as concepts, which allow developers to specify template constraints. Also introduced were ranges, which simplify operations on collections, and coroutines that enable asynchronous programming more intuitively.
The community surrounding C++ continues to thrive as open-source contributions flourish and new libraries emerge, propelling C++ toward future innovations.
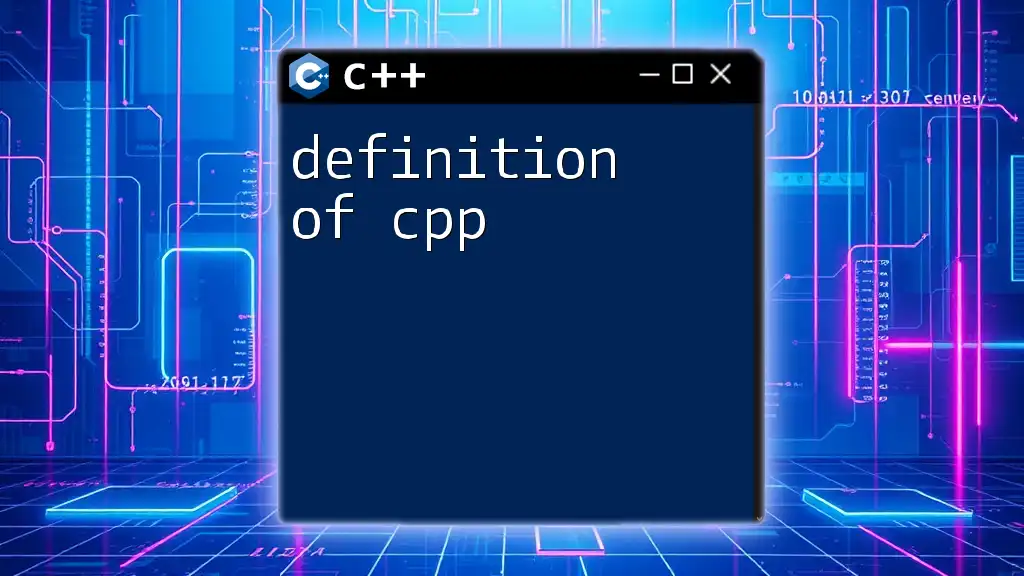
C++ in the Programming Ecosystem
Current Usage and Popularity
Today, the history of C++ is marked not just by its longevity but by its relevance in contemporary programming. Its versatility allows it to thrive in various domains, including game development, embedded systems, and high-performance computing.
Statistics indicate that C++ remains one of the most popular programming languages, consistently ranking high in developer surveys. It’s widely embraced for its performance capabilities and robustness, making it an ideal choice for resource-intensive applications.
C++ vs. Other Languages
When compared to languages like Python, Java, and Rust, C++ stands out due to its unique blend of low-level capabilities and high-level abstractions. This combination enables fine control over system resources, which can be crucial for performance-critical applications. However, it comes with complexities that sometimes necessitate a longer learning curve.
When to Choose C++: It is particularly advantageous for projects requiring high performance, such as game engines, real-time simulation, and extensive system programming.
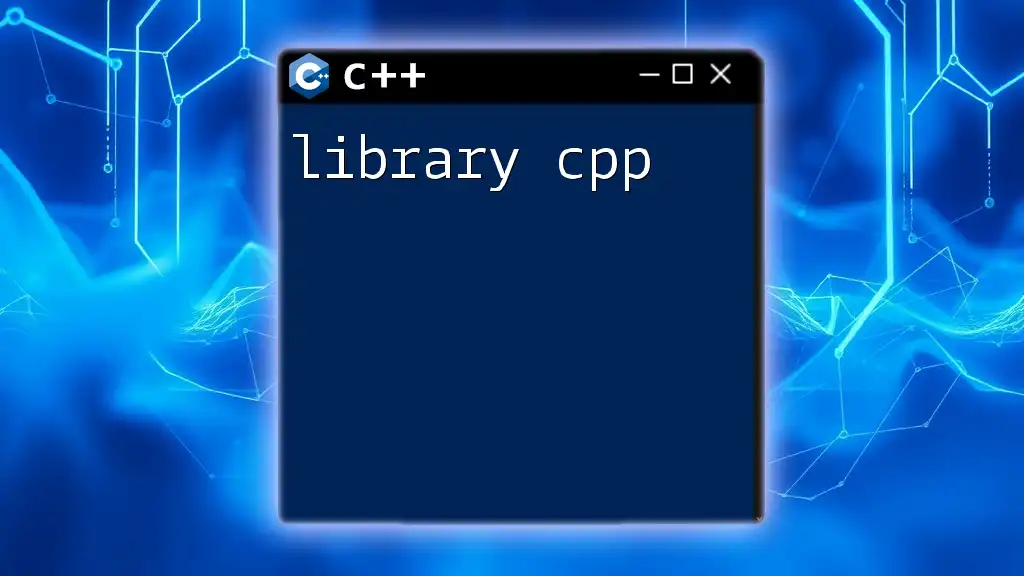
Conclusion
Understanding the history of C++ provides insights not only into the evolution of a powerful programming language but also into the philosophies that shaped modern software development practices. From its origins in the early days of computing to its status as a cornerstone of today's programming landscape, C++ remains a critical tool for developers around the globe.
As the language evolves, mastering its features and commands will empower you to tackle complex challenges in software development with confidence and efficiency.